The Role of Big O Notation in Coding Interviews: Mastering Algorithmic Efficiency
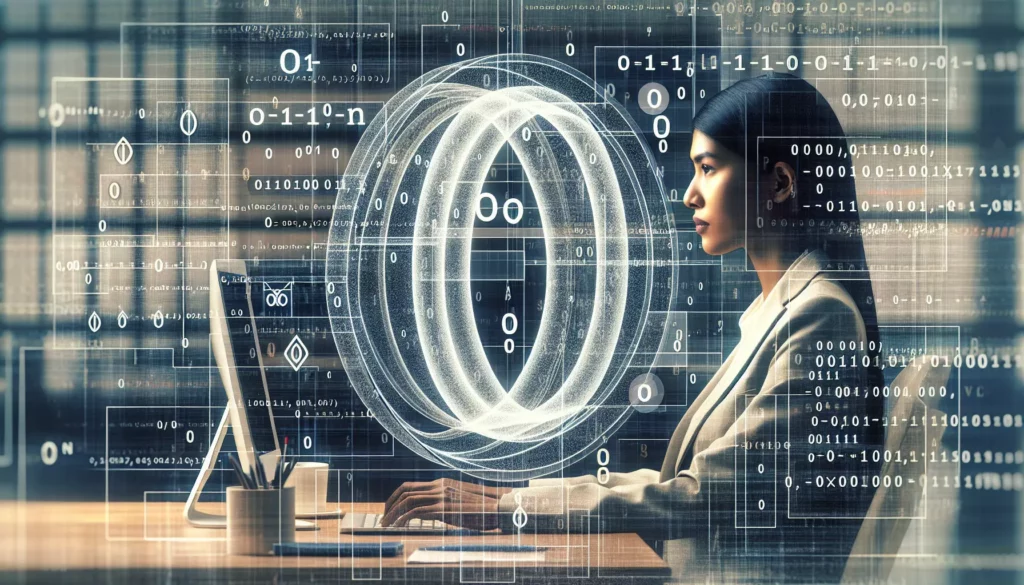
In the world of software engineering and computer science, few concepts are as ubiquitous and essential as Big O notation. Whether you’re a fresh graduate preparing for your first coding interview or a seasoned developer looking to join one of the FAANG (Facebook, Amazon, Apple, Netflix, Google) companies, understanding Big O notation is crucial. This powerful tool allows us to analyze and compare the efficiency of algorithms, making it an indispensable part of any coding interview preparation.
In this comprehensive guide, we’ll dive deep into the role of Big O notation in coding interviews, exploring its importance, how to use it effectively, and strategies to optimize your algorithmic thinking. By the end of this article, you’ll have a solid grasp of Big O notation and be better equipped to tackle complex coding challenges in your next interview.
What is Big O Notation?
Before we delve into its role in coding interviews, let’s start with a clear definition of Big O notation. Big O notation is a mathematical notation used in computer science to describe the performance or complexity of an algorithm. Specifically, it describes the worst-case scenario, or the maximum time an algorithm will take to complete as the input size grows.
The “O” in Big O notation stands for “Order of,” which refers to the order of magnitude of complexity. It provides an upper bound on the growth rate of an algorithm’s running time or space usage in relation to the input size.
For example, when we say an algorithm has a time complexity of O(n), we mean that its execution time grows linearly with the input size. If the input size doubles, the execution time will also roughly double.
Why is Big O Notation Important in Coding Interviews?
Understanding Big O notation is crucial in coding interviews for several reasons:
- Measuring Efficiency: Big O notation allows interviewers to assess how well you can analyze and optimize algorithms. It’s not just about solving the problem; it’s about solving it efficiently.
- Comparing Algorithms: It provides a standardized way to compare different algorithms and their performance, helping you choose the best solution for a given problem.
- Scalability: In real-world applications, algorithms need to perform well with large datasets. Big O notation helps predict how an algorithm will perform as the input size grows.
- Problem-Solving Skills: Analyzing time and space complexity demonstrates your ability to think critically about code and consider trade-offs between different approaches.
- Industry Standard: Big O notation is widely used in the tech industry, especially at FAANG companies, making it an essential skill for any aspiring software engineer.
Common Big O Notations and Their Meanings
To effectively use Big O notation in coding interviews, you need to be familiar with the most common complexity classes. Here are the key ones to remember:
- O(1) – Constant Time: The algorithm takes the same amount of time regardless of the input size. Example: Accessing an array element by index.
- O(log n) – Logarithmic Time: The algorithm’s time increases logarithmically as the input size grows. Example: Binary search in a sorted array.
- O(n) – Linear Time: The algorithm’s time increases linearly with the input size. Example: Traversing an array once.
- O(n log n) – Linearithmic Time: Common in efficient sorting algorithms. Example: Merge sort, quicksort (average case).
- O(n^2) – Quadratic Time: The algorithm’s time increases quadratically with the input size. Example: Nested loops, bubble sort.
- O(2^n) – Exponential Time: The algorithm’s time doubles with each addition to the input. Example: Recursive calculation of Fibonacci numbers.
- O(n!) – Factorial Time: The algorithm’s time grows factorially with the input size. Example: Generating all permutations of a set.
Understanding these complexity classes and being able to identify them in your code is crucial for success in coding interviews.
Analyzing Time Complexity: A Practical Approach
When asked to analyze the time complexity of an algorithm during a coding interview, follow these steps:
- Identify the input: Determine what variable represents the size of the input (usually denoted as ‘n’).
- Count the operations: Look for loops, recursive calls, and other operations that depend on the input size.
- Determine the growth rate: Focus on the dominant term as the input size approaches infinity.
- Simplify: Drop constants and lower-order terms.
Let’s practice with an example:
def find_max(arr):
max_val = arr[0]
for num in arr:
if num > max_val:
max_val = num
return max_val
Analyzing this function:
- The input size is the length of the array ‘arr’, let’s call it ‘n’.
- We have a single loop that iterates through each element once.
- The number of operations grows linearly with the input size.
- The time complexity is O(n).
Space Complexity: The Other Half of the Equation
While time complexity often takes center stage, space complexity is equally important in coding interviews. Space complexity refers to the amount of memory an algorithm uses relative to the input size.
When analyzing space complexity, consider:
- Variables created
- Data structures used
- Recursive call stack
Let’s look at an example:
def create_squared_array(n):
result = []
for i in range(n):
result.append(i * i)
return result
The space complexity of this function is O(n) because it creates an array that grows linearly with the input size ‘n’.
Common Pitfalls and How to Avoid Them
When dealing with Big O notation in coding interviews, be aware of these common mistakes:
- Ignoring constants: While we drop constants in the final notation, they can be important when comparing algorithms with the same Big O complexity.
- Focusing only on time complexity: Don’t forget to consider space complexity as well.
- Overcomplicating the analysis: Start with a simple analysis and refine if necessary.
- Misidentifying nested loops: Be careful with nested loops; they often lead to quadratic time complexity.
- Forgetting about best and average cases: While Big O represents the worst case, knowing best and average cases can be valuable.
Optimizing Algorithms: From Theory to Practice
Understanding Big O notation is just the first step. The real challenge in coding interviews is using this knowledge to optimize your algorithms. Here are some strategies:
- Use appropriate data structures: Choosing the right data structure can significantly improve your algorithm’s efficiency. For example, using a hash table for quick lookups instead of repeatedly searching an array.
- Avoid unnecessary work: Look for opportunities to reduce redundant calculations or iterations.
- Divide and conquer: Break down complex problems into smaller, manageable parts. This often leads to more efficient solutions.
- Caching and memoization: Store results of expensive operations to avoid recalculating them.
- Two-pointer technique: This can often reduce the time complexity from O(n^2) to O(n) in array-related problems.
Let’s see an optimization example:
def has_duplicate(arr):
# Original approach: O(n^2)
for i in range(len(arr)):
for j in range(i+1, len(arr)):
if arr[i] == arr[j]:
return True
return False
def has_duplicate_optimized(arr):
# Optimized approach: O(n)
seen = set()
for num in arr:
if num in seen:
return True
seen.add(num)
return False
The optimized version uses a set for O(1) lookups, reducing the overall time complexity from O(n^2) to O(n).
Big O Notation in Real-World Scenarios
While understanding Big O notation is crucial for coding interviews, its importance extends far beyond that. In real-world software development, especially at FAANG companies, efficient algorithms can make the difference between a system that scales gracefully and one that crumbles under load.
Consider these scenarios:
- Search Engines: Google’s search algorithm needs to process billions of web pages quickly. An algorithm with poor time complexity would make this impossible.
- Social Media Feeds: Facebook’s news feed algorithm must efficiently sort and display posts from thousands of friends and pages in real-time.
- E-commerce Recommendations: Amazon’s recommendation system analyzes vast amounts of data to suggest products. An inefficient algorithm would lead to slow page loads and lost sales.
- Streaming Services: Netflix’s content delivery and recommendation systems need to work seamlessly for millions of concurrent users.
In these cases, even small improvements in algorithmic efficiency can lead to significant performance gains and cost savings at scale.
Practicing Big O Analysis: Sample Interview Questions
To help you prepare for coding interviews, here are some sample questions focusing on Big O notation:
- Question: What’s the time complexity of searching for an element in a balanced binary search tree?
Answer: O(log n), as each comparison allows us to eliminate half of the remaining tree. - Question: Analyze the time and space complexity of the following function:
def fibonacci(n): if n <= 1: return n return fibonacci(n-1) + fibonacci(n-2)
Answer: Time complexity is O(2^n) due to the exponential number of recursive calls. Space complexity is O(n) due to the maximum depth of the recursion stack.
- Question: How would you optimize the following function, and what’s its current time complexity?
def find_sum_pair(arr, target): for i in range(len(arr)): for j in range(i+1, len(arr)): if arr[i] + arr[j] == target: return (arr[i], arr[j]) return None
Answer: Current time complexity is O(n^2). It can be optimized to O(n) using a hash table:
def find_sum_pair_optimized(arr, target): seen = {} for num in arr: if target - num in seen: return (num, target - num) seen[num] = True return None
Advanced Topics in Big O Notation
As you become more comfortable with basic Big O analysis, consider exploring these advanced topics:
- Amortized Analysis: This technique averages the time required to perform a sequence of data structure operations over all the operations performed. It’s particularly useful for data structures like dynamic arrays.
- Multi-Variable Time Complexity: Some algorithms have time complexities that depend on multiple input variables. For example, an algorithm that operates on a graph might have a complexity of O(V + E), where V is the number of vertices and E is the number of edges.
- Space-Time Trade-offs: Often, you can reduce time complexity by using more space, or vice versa. Understanding these trade-offs is crucial for optimizing algorithms in constrained environments.
- Lower Bounds and Big Omega Notation: While Big O provides an upper bound, Big Omega (Ω) notation provides a lower bound on the growth rate of an algorithm.
- NP-Completeness: Some problems are believed to have no polynomial-time solutions. Understanding NP-completeness can help you recognize when to look for approximate solutions or heuristics instead of exact algorithms.
Conclusion: Mastering Big O Notation for Interview Success
Big O notation is more than just a tool for analyzing algorithms—it’s a fundamental concept that underpins much of computer science and software engineering. In coding interviews, especially at top tech companies like FAANG, demonstrating a solid understanding of Big O notation can set you apart from other candidates.
Remember these key points:
- Big O notation describes the worst-case scenario for algorithm performance.
- It’s crucial for comparing algorithms and predicting scalability.
- Practice analyzing both time and space complexity.
- Use your understanding of Big O to optimize your solutions.
- Real-world applications often require balancing multiple factors, not just optimizing for the best Big O complexity.
As you prepare for your coding interviews, make analyzing and optimizing algorithms using Big O notation a core part of your practice routine. With time and effort, you’ll develop the intuition to quickly assess and improve the efficiency of your code—a skill that will serve you well both in interviews and in your career as a software engineer.
Remember, mastering Big O notation is a journey. Keep practicing, stay curious, and don’t hesitate to dive deeper into the mathematical foundations if you’re inclined. Your efforts will pay off not just in acing coding interviews, but in becoming a more thoughtful and efficient programmer overall.