How to Communicate Your Thought Process Clearly: A Comprehensive Guide for Programmers
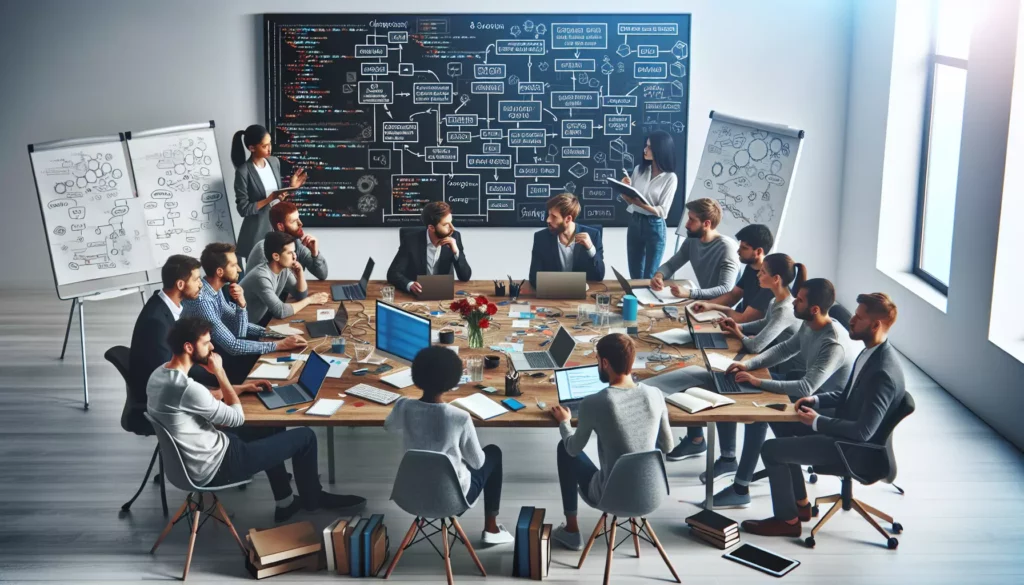
In the world of programming and software development, being able to communicate your thought process clearly is just as important as writing efficient code. Whether you’re collaborating with team members, explaining your solution during a technical interview, or documenting your work for future reference, the ability to articulate your ideas effectively can make a significant difference in your career. This comprehensive guide will explore various strategies and techniques to help you communicate your thought process clearly, with a focus on coding and problem-solving scenarios.
1. Understanding the Importance of Clear Communication
Before diving into specific techniques, it’s crucial to recognize why clear communication of your thought process is so valuable:
- Collaboration: In team environments, clear communication facilitates better collaboration and reduces misunderstandings.
- Problem-solving: Articulating your thoughts helps you organize your ideas and often leads to better solutions.
- Interviews: During technical interviews, explaining your approach can be just as important as arriving at the correct solution.
- Documentation: Clear communication in comments and documentation makes your code more maintainable and easier for others to understand.
- Learning: By explaining concepts to others, you reinforce your own understanding and identify areas for improvement.
2. Start with a High-Level Overview
When communicating your thought process, it’s often helpful to begin with a high-level overview before diving into the details. This approach provides context and helps your audience understand the big picture.
Tips for providing a high-level overview:
- Summarize the problem or task in your own words
- Outline the main steps or components of your solution
- Highlight any key algorithms or data structures you plan to use
- Mention any assumptions or constraints you’re working with
For example, if you’re explaining your approach to solving a coding problem, you might start like this:
"To solve this problem of finding the longest palindromic substring, I'm planning to use a dynamic programming approach. The main idea is to build a table that stores whether substrings are palindromes, starting with the smallest substrings and working our way up to the full string. I'll then use this table to find the longest palindromic substring. The time complexity of this solution will be O(n^2), where n is the length of the input string."
3. Break Down Complex Ideas into Smaller Parts
Complex problems or solutions can be overwhelming when presented all at once. Breaking them down into smaller, more manageable parts makes your thought process easier to follow and understand.
Strategies for breaking down complex ideas:
- Use a step-by-step approach
- Group related concepts or operations
- Explain each component or function separately
- Use analogies or real-world examples to illustrate abstract concepts
For instance, when explaining a complex algorithm, you might break it down like this:
"Let's break down the merge sort algorithm into three main steps:
1. Divide: We'll split the array into two halves recursively until we have subarrays of size 1.
2. Conquer: We'll sort these small subarrays.
3. Combine: We'll merge the sorted subarrays back together to form the final sorted array.
Now, let's look at each step in more detail..."
4. Use Visual Aids and Diagrams
Visual representations can be incredibly powerful when communicating complex ideas or algorithms. They can help illustrate relationships, flow of control, and data structures in a way that words alone cannot.
Types of visual aids that can be helpful:
- Flowcharts for algorithmic processes
- Tree diagrams for hierarchical data structures
- Box and arrow diagrams for system architecture
- State diagrams for finite state machines
- Timelines for explaining time complexities
When using visual aids, remember to:
- Keep them simple and focused on the key ideas
- Explain each component of the diagram
- Use consistent notation and color coding
- Reference the diagram throughout your explanation
5. Use Pseudocode Before Actual Code
Pseudocode can be an excellent tool for communicating your thought process, especially when explaining algorithms or complex procedures. It allows you to focus on the logic and structure of your solution without getting bogged down in language-specific syntax.
Benefits of using pseudocode:
- It’s easier to understand for non-programmers or those unfamiliar with the specific programming language
- It allows you to express your ideas at a higher level of abstraction
- It’s quicker to write and modify than actual code
- It helps identify logical errors before writing the actual implementation
Here’s an example of how you might use pseudocode to explain a binary search algorithm:
function binarySearch(array, target):
left = 0
right = length of array - 1
while left <= right:
mid = (left + right) / 2
if array[mid] == target:
return mid
else if array[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 // Target not found
6. Provide Examples and Edge Cases
Concrete examples can significantly enhance understanding, especially when dealing with abstract concepts or complex algorithms. Additionally, discussing edge cases demonstrates a thorough understanding of the problem and solution.
Tips for using examples effectively:
- Start with a simple, straightforward example
- Gradually increase complexity to illustrate different aspects of your solution
- Use realistic data that reflects actual use cases
- Walk through the example step-by-step, explaining each operation
Addressing edge cases:
- Identify potential edge cases (e.g., empty input, maximum values, etc.)
- Explain how your solution handles these cases
- Discuss any necessary error handling or input validation
For example, when explaining a function that finds the maximum element in an array:
"Let's walk through an example. Consider the array [3, 7, 1, 9, 4, 2]. Our function would iterate through each element, keeping track of the maximum value seen so far. It would return 9 as the result.
Now, let's consider some edge cases:
1. Empty array: We should handle this by returning an error or a special value.
2. Array with one element: In this case, that single element is the maximum.
3. Array with all equal elements: Any element can be returned as the maximum.
4. Array with negative numbers: Our algorithm works for this case without modification."
7. Explain Time and Space Complexity
When communicating your thought process, especially in the context of algorithm design or optimization, it’s crucial to discuss the time and space complexity of your solution. This demonstrates a deeper understanding of efficiency and scalability considerations.
Key points to cover when discussing complexity:
- Explain the Big O notation for both time and space complexity
- Break down the complexity analysis for different parts of your solution
- Discuss any trade-offs between time and space efficiency
- Compare your solution’s complexity to alternative approaches
Here’s an example of how you might explain the complexity of a solution:
"The time complexity of this solution is O(n log n) due to the sorting step, where n is the number of elements in the input array. The subsequent linear scan through the sorted array takes O(n) time, but this is dominated by the sorting complexity.
In terms of space complexity, we're using O(1) extra space as we're sorting the array in-place. If we weren't allowed to modify the input array, we'd need O(n) extra space to create a copy for sorting.
An alternative approach using a hash table would have O(n) time complexity but would require O(n) extra space. Our current solution trades some time efficiency for better space efficiency."
8. Use Analogies and Real-World Comparisons
Analogies and real-world comparisons can be powerful tools for explaining complex or abstract concepts. They help bridge the gap between technical ideas and everyday experiences, making your explanations more relatable and memorable.
Tips for using analogies effectively:
- Choose analogies that are familiar to your audience
- Ensure the analogy accurately represents the key aspects of the concept
- Explain where the analogy breaks down or has limitations
- Use multiple analogies to illustrate different aspects of a complex idea
For example, when explaining the concept of recursion:
"Recursion is like solving a big problem by breaking it down into smaller versions of the same problem. It's similar to the process of looking up a word in a dictionary. If you don't understand a word in the definition, you look up that word, and so on, until you reach a point where you understand all the words. Then you work your way back up to understand the original word.
In programming, instead of a person doing this manually, the function calls itself with a simpler version of the problem until it reaches a base case that can be solved directly. Then it combines these solutions to solve the original problem."
9. Practice Active Listening and Encourage Questions
Effective communication is a two-way street. While explaining your thought process, it’s crucial to practice active listening and encourage questions from your audience. This approach ensures that your explanation is understood and allows you to clarify any points of confusion.
Strategies for encouraging interaction:
- Pause periodically to check for understanding
- Ask if there are any questions after explaining each major point
- Encourage your audience to rephrase your explanation in their own words
- Be open to alternative viewpoints or suggestions
For example, you might say:
"I've just explained the basic concept of hash tables. Does anyone have any questions about how they work or why we use them? Can someone summarize the key advantages of hash tables that we've discussed?"
10. Tailor Your Communication to Your Audience
The effectiveness of your communication largely depends on how well you can adapt your explanation to your audience’s level of knowledge and experience. Whether you’re speaking to a fellow senior developer, a junior programmer, or a non-technical stakeholder, your approach should be tailored accordingly.
Tips for audience-specific communication:
- Assess your audience’s background and familiarity with the topic
- Adjust your level of technical detail based on their expertise
- Use appropriate terminology and explain jargon if necessary
- Focus on aspects that are most relevant to your audience’s interests or needs
For instance, when explaining a new feature to different stakeholders:
For developers: "We've implemented the new search functionality using an inverted index data structure, which allows for efficient full-text search with a time complexity of O(k + log n) for k search terms and n documents."
For non-technical stakeholders: "The new search feature we've added will make it much faster for users to find relevant information. It uses advanced techniques that allow it to quickly scan through large amounts of text and return accurate results, even as we add more content to the system."
11. Use Version Control Analogies for Explaining Code Changes
When discussing code changes or the evolution of a solution, using analogies from version control systems can be very effective. This approach is particularly useful when explaining refactoring processes or iterative improvements to an algorithm.
Version control concepts that can be used as analogies:
- Commits: Representing distinct stages or versions of your solution
- Branches: Illustrating different approaches or variations of an algorithm
- Merging: Combining ideas or integrating optimizations
- Diffs: Highlighting specific changes or improvements between versions
For example, when explaining how you optimized an algorithm:
"Let's think of our algorithm optimization process like a series of Git commits. Our initial commit was a brute force approach with O(n^2) time complexity. In the next commit, we introduced memoization, which improved our average-case performance but didn't change the worst-case complexity.
Our latest commit represents a significant refactor where we switched to a dynamic programming approach. This 'merge' of our previous ideas with the new DP concept resulted in an O(n) time complexity solution. If we look at the 'diff' between our initial and final versions, we can see how we've traded space for time by using an additional array to store intermediate results."
12. Utilize the Feynman Technique
The Feynman Technique, named after the renowned physicist Richard Feynman, is a powerful method for explaining complex ideas. It involves explaining a concept in simple terms as if you were teaching it to someone with no background in the subject. This approach not only helps others understand but also reinforces your own understanding.
Steps of the Feynman Technique:
- Choose the concept you want to explain
- Explain it to an imaginary 12-year-old (or write it down in simple language)
- Identify gaps in your explanation or understanding
- Review and simplify (or try analogies for) the difficult parts
Here’s an example of using the Feynman Technique to explain binary search:
"Imagine you're trying to find a specific page in a big book. You could start at the beginning and flip through each page until you find it, but that would take a long time. Instead, you might open the book to the middle, see if your page number is higher or lower, and then only look in that half of the book. You keep doing this, cutting the search area in half each time, until you find your page.
That's basically how binary search works with numbers. It's a way of finding a specific number in a sorted list by repeatedly dividing the search area in half. It's much faster than checking every single number, especially when you have a really long list."
13. Document Your Thought Process in Code
While verbal and written explanations are important, documenting your thought process directly in your code is equally crucial. This practice not only helps others understand your code but also aids your future self when revisiting the code.
Best practices for documenting your thought process in code:
- Write clear and concise comments explaining the ‘why’ behind complex logic
- Use meaningful variable and function names that convey their purpose
- Include a high-level description of the algorithm or approach in the function/class documentation
- Document any assumptions, constraints, or edge cases handled by the code
- Use TODO comments to indicate areas for future improvement or optimization
Here’s an example of well-documented code that communicates the thought process:
/**
* Finds the longest palindromic substring in the given string.
* Uses dynamic programming approach with O(n^2) time and space complexity.
*
* @param s The input string
* @return The longest palindromic substring
*/
public String longestPalindrome(String s) {
int n = s.length();
boolean[][] dp = new boolean[n][n];
int start = 0, maxLength = 1;
// All substrings of length 1 are palindromes
for (int i = 0; i < n; i++) {
dp[i][i] = true;
}
// Check for substrings of length 2
for (int i = 0; i < n - 1; i++) {
if (s.charAt(i) == s.charAt(i + 1)) {
dp[i][i + 1] = true;
start = i;
maxLength = 2;
}
}
// Check for substrings of length 3 or more
for (int len = 3; len <= n; len++) {
for (int i = 0; i < n - len + 1; i++) {
int j = i + len - 1;
// A substring is a palindrome if its first and last characters match
// and the substring without these characters is also a palindrome
if (s.charAt(i) == s.charAt(j) && dp[i + 1][j - 1]) {
dp[i][j] = true;
if (len > maxLength) {
start = i;
maxLength = len;
}
}
}
}
return s.substring(start, start + maxLength);
}
14. Reflect on Your Problem-Solving Process
An often overlooked aspect of communicating your thought process is reflecting on how you arrived at your solution. This meta-cognitive approach not only helps others understand your reasoning but also improves your own problem-solving skills over time.
Key points to consider when reflecting on your process:
- Initial approach and why you chose it
- Challenges encountered and how you overcame them
- Alternative solutions considered and why they were rejected
- Lessons learned or insights gained during the process
- Areas for potential improvement or further optimization
For example, you might explain your thought process like this:
"When I first approached this problem of finding the k-th largest element in an unsorted array, I initially thought of sorting the entire array and then returning the k-th element from the end. However, I realized this would always have a time complexity of O(n log n) due to the sorting step, regardless of the value of k.
I then considered using a max heap, which would give us O(n log k) time complexity. This was better, but I felt we could do even better for the average case.
Eventually, I settled on using the QuickSelect algorithm, which has an average time complexity of O(n) and worst-case of O(n^2). The key insight was that we don't need to fully sort the array; we just need to ensure that the k-th largest element is in its correct position.
One challenge I faced was handling duplicate elements correctly. I overcame this by using a three-way partitioning scheme in the QuickSelect algorithm.
If I were to improve this further, I might consider implementing a hybrid approach that falls back to the heap solution for certain input sizes to guarantee O(n log k) worst-case performance while still achieving O(n) average-case complexity."
Conclusion
Effectively communicating your thought process is a vital skill for any programmer or software developer. It enhances collaboration, improves problem-solving abilities, and can significantly impact your performance in technical interviews and your overall career progression.
By employing the strategies discussed in this guide – from providing high-level overviews and breaking down complex ideas, to using visual aids and pseudocode, to reflecting on your problem-solving process – you can greatly improve your ability to articulate your thoughts and ideas.
Remember, clear communication is not just about explaining your solution, but also about conveying your reasoning, considering alternatives, and demonstrating a thorough understanding of the problem space. With practice and conscious effort, you can develop this skill and stand out as a clear and effective communicator in the world of programming.
As you continue to hone your communication skills, you’ll likely find that it not only helps others understand your work better but also enhances your own understanding and problem-solving capabilities. After all, as the saying goes, “If you can’t explain it simply, you don’t understand it well enough.” So, embrace the challenge of communicating your thought process clearly, and watch as it transforms both your coding and your career.