Understanding Time and Space Complexity in Interviews
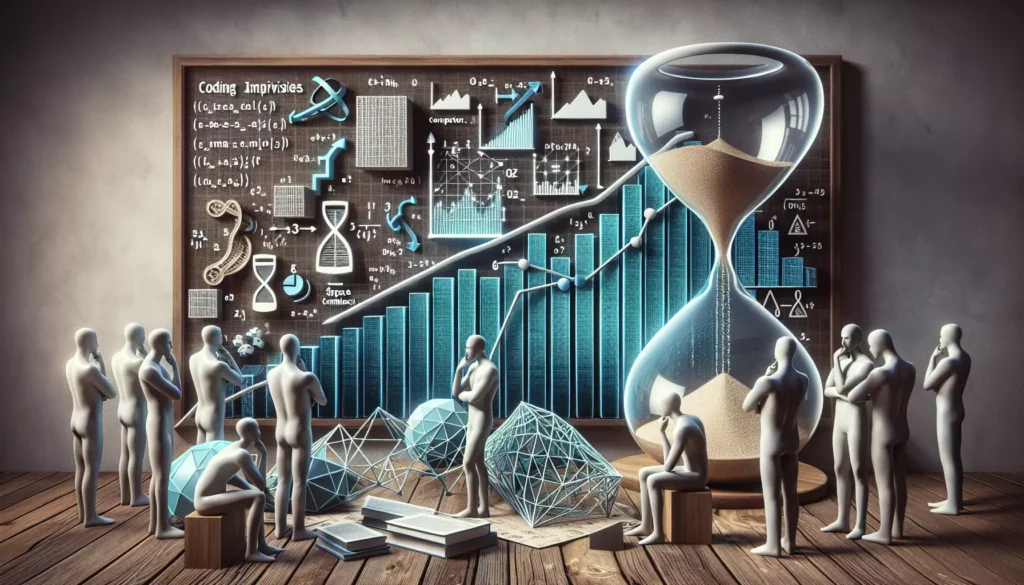
When preparing for technical interviews, especially those at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), understanding time and space complexity is crucial. These concepts are fundamental to analyzing the efficiency of algorithms and are frequently discussed during coding interviews. In this comprehensive guide, we’ll dive deep into time and space complexity, their importance in interviews, and how to effectively communicate your understanding to interviewers.
What are Time and Space Complexity?
Before we delve into the specifics, let’s define what time and space complexity mean in the context of computer science and algorithm analysis:
Time Complexity
Time complexity is a measure of how long an algorithm takes to run as the input size increases. It’s typically expressed using Big O notation, which describes the upper bound of the growth rate of an algorithm’s running time.
Space Complexity
Space complexity refers to the amount of memory an algorithm uses relative to the input size. Like time complexity, it’s often expressed using Big O notation to describe the worst-case scenario for memory usage.
Why are Time and Space Complexity Important in Interviews?
Understanding and effectively communicating time and space complexity during interviews is essential for several reasons:
- Demonstrates analytical skills: It shows you can think critically about algorithm efficiency.
- Proves problem-solving ability: It indicates you can optimize solutions for better performance.
- Showcases CS fundamentals: It reflects a solid grasp of computer science principles.
- Aligns with real-world scenarios: Efficient algorithms are crucial in production environments.
- Differentiates candidates: Strong complexity analysis can set you apart from other applicants.
Common Time Complexities
Let’s explore some common time complexities you’re likely to encounter in coding interviews, along with examples:
O(1) – Constant Time
Constant time complexity means the algorithm always takes the same amount of time, regardless of input size.
Example: Accessing an array element by index.
def get_element(arr, index):
return arr[index]
O(log n) – Logarithmic Time
Logarithmic time complexity often appears in algorithms that divide the problem in half each time.
Example: Binary search in a sorted array.
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
O(n) – Linear Time
Linear time complexity means the running time increases linearly with the input size.
Example: Finding the maximum element in an unsorted array.
def find_max(arr):
max_val = float('-inf')
for num in arr:
if num > max_val:
max_val = num
return max_val
O(n log n) – Linearithmic Time
This complexity often appears in efficient sorting algorithms.
Example: Merge sort algorithm.
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
O(n^2) – Quadratic Time
Quadratic time complexity often appears in algorithms with nested iterations over the input.
Example: Bubble sort algorithm.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
O(2^n) – Exponential Time
Exponential time complexity often appears in recursive algorithms that solve a problem of size N by recursively solving two smaller problems of size N-1.
Example: Recursive calculation of Fibonacci numbers.
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n - 1) + fibonacci(n - 2)
Common Space Complexities
Now let’s look at some common space complexities:
O(1) – Constant Space
Constant space complexity means the algorithm uses a fixed amount of memory, regardless of input size.
Example: In-place array reversal.
def reverse_array(arr):
left, right = 0, len(arr) - 1
while left < right:
arr[left], arr[right] = arr[right], arr[left]
left += 1
right -= 1
return arr
O(n) – Linear Space
Linear space complexity means the memory usage grows linearly with the input size.
Example: Creating a new array with the squares of input array elements.
def square_array(arr):
return [x**2 for x in arr]
O(n^2) – Quadratic Space
Quadratic space complexity often appears when creating a two-dimensional structure based on the input.
Example: Creating an adjacency matrix for a graph with n vertices.
def create_adjacency_matrix(n):
return [[0 for _ in range(n)] for _ in range(n)]
Analyzing Time and Space Complexity
When analyzing the time and space complexity of an algorithm, consider the following steps:
- Identify the input: Determine what constitutes the input and how it affects the algorithm’s performance.
- Count the operations: Identify the core operations and how they scale with input size.
- Consider the worst-case scenario: Focus on the upper bound of the algorithm’s performance.
- Simplify the expression: Drop constants and lower-order terms to get the Big O notation.
- Analyze space usage: Consider both auxiliary space (extra space used by the algorithm) and input space.
Communicating Complexity in Interviews
When discussing time and space complexity during an interview, follow these best practices:
- Be proactive: Mention complexity analysis without being prompted.
- Explain your reasoning: Walk the interviewer through your thought process.
- Use precise terminology: Employ Big O notation correctly.
- Consider all cases: Discuss best, average, and worst-case scenarios if relevant.
- Propose optimizations: If you identify areas for improvement, mention them.
Common Pitfalls to Avoid
When analyzing and discussing complexity, be aware of these common mistakes:
- Overlooking hidden loops: Be cautious of operations like string concatenation or certain built-in functions that may have hidden loops.
- Ignoring constant factors: While we often drop constants in Big O notation, very large constants can be significant in practice.
- Misunderstanding space complexity: Remember to consider both auxiliary space and input space.
- Overcomplicating analysis: Strive for the simplest correct Big O expression.
- Neglecting amortized analysis: Some data structures (like dynamic arrays) have operations with amortized time complexity that differs from their worst-case complexity.
Practice Problems
To reinforce your understanding of time and space complexity, try analyzing the following problems:
Problem 1: Two Sum
Given an array of integers and a target sum, find two numbers in the array that add up to the target.
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
Time Complexity: O(n) – We iterate through the array once.
Space Complexity: O(n) – In the worst case, we store all elements in the dictionary.
Problem 2: Merge Sorted Arrays
Merge two sorted arrays into a single sorted array.
def merge_sorted_arrays(arr1, arr2):
result = []
i, j = 0, 0
while i < len(arr1) and j < len(arr2):
if arr1[i] < arr2[j]:
result.append(arr1[i])
i += 1
else:
result.append(arr2[j])
j += 1
result.extend(arr1[i:])
result.extend(arr2[j:])
return result
Time Complexity: O(n + m), where n and m are the lengths of arr1 and arr2 respectively.
Space Complexity: O(n + m) for the result array.
Advanced Topics in Complexity Analysis
As you progress in your understanding of time and space complexity, consider exploring these advanced topics:
Amortized Analysis
Amortized analysis considers the average performance of a sequence of operations, rather than just the worst-case scenario for a single operation. This is particularly relevant for data structures like dynamic arrays (e.g., ArrayList in Java or list in Python) where occasional resizing operations are costly but infrequent.
Recursion and Master Theorem
The Master Theorem provides a cookbook method for solving recurrence relations that often arise when analyzing recursive algorithms. Understanding this theorem can greatly simplify the analysis of divide-and-conquer algorithms.
NP-Completeness
Some problems are inherently difficult and are believed not to have polynomial-time solutions. Understanding the basics of NP-completeness can help you recognize when you’re dealing with such problems and when to look for approximate solutions or heuristics.
Conclusion
Understanding time and space complexity is a crucial skill for any programmer, especially when preparing for technical interviews at top tech companies. By mastering these concepts, you’ll be better equipped to analyze and optimize your algorithms, communicate effectively about code efficiency, and tackle complex programming challenges.
Remember, practice is key. As you work through coding problems, make it a habit to consider and discuss the time and space complexity of your solutions. Over time, this analysis will become second nature, making you a stronger programmer and a more competitive candidate in technical interviews.
Keep learning, keep practicing, and don’t hesitate to dive deeper into the fascinating world of algorithm analysis and optimization. Your efforts will pay off not just in interviews, but throughout your career as a software developer.