Mastering the Art of Coding Interviews: A Comprehensive Guide
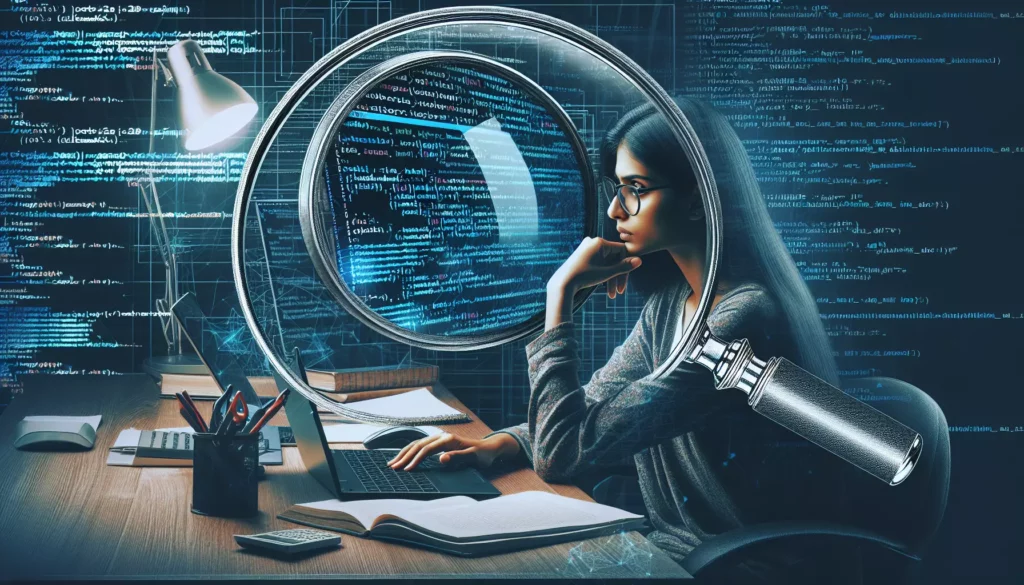
In today’s competitive tech industry, mastering coding interviews is a crucial skill for aspiring software engineers and developers. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech firm, being well-prepared for coding interviews can make all the difference in landing your dream job. This comprehensive guide will walk you through the essential steps to excel in coding interviews, from understanding the basics to tackling advanced algorithms and data structures.
1. Understanding the Coding Interview Process
Before diving into the specifics of preparation, it’s important to understand what coding interviews typically entail. Most technical interviews follow a similar structure:
- Introduction and brief discussion about your background
- One or more coding problems to solve
- Discussion of your approach and solution
- Questions about your code, potential optimizations, and edge cases
- Time for you to ask questions about the company or role
The coding portion is usually the most critical part of the interview, where you’ll be asked to solve algorithmic problems or implement specific functionalities. This is where your problem-solving skills and coding proficiency will be put to the test.
2. Essential Topics to Master
To succeed in coding interviews, you need to have a solid grasp of fundamental computer science concepts and data structures. Here are the key areas to focus on:
2.1. Data Structures
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
2.2. Algorithms
- Sorting (e.g., Quicksort, Mergesort)
- Searching (e.g., Binary Search)
- Recursion and Dynamic Programming
- Graph Algorithms (e.g., BFS, DFS)
- Greedy Algorithms
2.3. Big O Notation and Time Complexity
Understanding time and space complexity is crucial for optimizing your solutions and discussing trade-offs with your interviewer. Make sure you can analyze the efficiency of your algorithms using Big O notation.
3. Developing Problem-Solving Skills
Coding interviews are not just about memorizing algorithms; they’re about demonstrating your problem-solving abilities. Here’s a step-by-step approach to tackling coding problems:
- Understand the problem: Carefully read the question and ask clarifying questions if needed.
- Identify the inputs and outputs: Determine what data you’re working with and what result is expected.
- Break down the problem: Divide the problem into smaller, manageable sub-problems.
- Plan your approach: Outline a high-level strategy before diving into coding.
- Implement your solution: Write clean, readable code to solve the problem.
- Test your code: Consider edge cases and test your solution with various inputs.
- Optimize: Look for ways to improve the efficiency of your solution.
4. Practice, Practice, Practice
The key to mastering coding interviews is consistent practice. Here are some effective ways to hone your skills:
4.1. Coding Platforms
Utilize online coding platforms that offer a wide range of problems and allow you to practice in a simulated interview environment. Some popular options include:
- LeetCode
- HackerRank
- CodeSignal
- AlgoExpert
4.2. Mock Interviews
Participate in mock interviews to get comfortable with the interview process and receive feedback on your performance. You can use platforms like:
- Pramp
- InterviewBit
- Interviewing.io
4.3. Coding Projects
Work on personal coding projects to apply your skills in real-world scenarios and build a portfolio to showcase your abilities.
5. Mastering Common Coding Patterns
Many coding interview questions follow certain patterns. Familiarizing yourself with these patterns can help you recognize and solve problems more efficiently. Here are some common patterns to study:
5.1. Two Pointers
This technique involves using two pointers to traverse an array or string, often moving in opposite directions. It’s useful for problems involving searching, reversing, or finding pairs in sorted arrays.
Example problem: Reverse a string in-place
def reverse_string(s):
left, right = 0, len(s) - 1
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
return s
# Example usage
input_string = list("hello")
print(reverse_string(input_string)) # Output: ['o', 'l', 'l', 'e', 'h']
5.2. Sliding Window
This pattern is used to perform operations on a specific window size of an array or string. It’s particularly useful for solving substring or subarray problems.
Example problem: Find the maximum sum subarray of size k
def max_sum_subarray(arr, k):
if len(arr) < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(k, len(arr)):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
# Example usage
arr = [1, 4, 2, 10, 23, 3, 1, 0, 20]
k = 4
print(max_sum_subarray(arr, k)) # Output: 39
5.3. Fast and Slow Pointers
This technique, also known as the “tortoise and hare” algorithm, uses two pointers moving at different speeds. It’s often used to detect cycles in linked lists or arrays.
Example problem: Detect a cycle in a linked list
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def has_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
# Example usage
# Create a linked list with a cycle
head = ListNode(1)
head.next = ListNode(2)
head.next.next = ListNode(3)
head.next.next.next = ListNode(4)
head.next.next.next.next = head.next # Create cycle
print(has_cycle(head)) # Output: True
5.4. Merge Intervals
This pattern deals with problems involving overlapping intervals. It’s useful for scheduling and time management problems.
Example problem: Merge overlapping intervals
def merge_intervals(intervals):
if not intervals:
return []
# Sort intervals based on start time
intervals.sort(key=lambda x: x[0])
merged = [intervals[0]]
for interval in intervals[1:]:
if interval[0] <= merged[-1][1]:
merged[-1][1] = max(merged[-1][1], interval[1])
else:
merged.append(interval)
return merged
# Example usage
intervals = [[1,3],[2,6],[8,10],[15,18]]
print(merge_intervals(intervals)) # Output: [[1,6],[8,10],[15,18]]
6. Advanced Topics
As you progress in your preparation, you may encounter more advanced topics in coding interviews. Here are some areas to explore:
6.1. System Design
For senior-level positions, you may be asked to design large-scale distributed systems. Key areas to study include:
- Scalability and load balancing
- Database design (SQL vs. NoSQL)
- Caching mechanisms
- Microservices architecture
6.2. Object-Oriented Design
Understanding OOP principles and being able to design classes and interfaces is crucial. Focus on:
- SOLID principles
- Design patterns
- UML diagrams
6.3. Concurrency and Multithreading
For certain roles, you may need to demonstrate knowledge of concurrent programming. Key concepts include:
- Thread synchronization
- Deadlocks and race conditions
- Parallel algorithms
7. Soft Skills and Communication
While technical skills are crucial, don’t underestimate the importance of soft skills in coding interviews. Here are some tips to improve your communication during the interview:
- Think out loud: Explain your thought process as you work through the problem.
- Ask clarifying questions: Don’t hesitate to seek clarification if something is unclear.
- Be open to feedback: If the interviewer provides hints or suggestions, be receptive and incorporate them into your solution.
- Stay calm under pressure: Practice staying composed even when faced with challenging problems.
- Show enthusiasm: Demonstrate your passion for coding and problem-solving.
8. Interview Day Preparation
As your interview day approaches, here are some final preparations to ensure you’re ready:
- Review your resume: Be prepared to discuss any projects or experiences you’ve listed.
- Research the company: Familiarize yourself with the company’s products, culture, and recent news.
- Prepare questions: Have thoughtful questions ready to ask your interviewer about the role and company.
- Set up your environment: If it’s a remote interview, test your audio, video, and coding environment in advance.
- Get a good night’s sleep: Ensure you’re well-rested and alert for the interview.
9. Post-Interview Follow-Up
After the interview, take these steps to leave a lasting positive impression:
- Send a thank-you email to your interviewer within 24 hours.
- Reflect on the interview experience and note areas for improvement.
- If you don’t hear back within the expected timeframe, follow up politely with the recruiter.
Conclusion
Mastering coding interviews is a journey that requires dedication, practice, and continuous learning. By focusing on core computer science concepts, honing your problem-solving skills, and practicing regularly, you can significantly improve your chances of success in technical interviews.
Remember that the goal of coding interviews is not just to test your knowledge but also to evaluate your thought process and problem-solving approach. Stay calm, communicate clearly, and approach each problem systematically. With the right preparation and mindset, you’ll be well-equipped to tackle even the most challenging coding interviews and land your dream job in the tech industry.
Keep practicing, stay curious, and never stop learning. The world of technology is constantly evolving, and so should your skills. Good luck with your coding interview preparation!