99 Effective Ways to Stay Organized While Coding: Boost Your Productivity and Efficiency
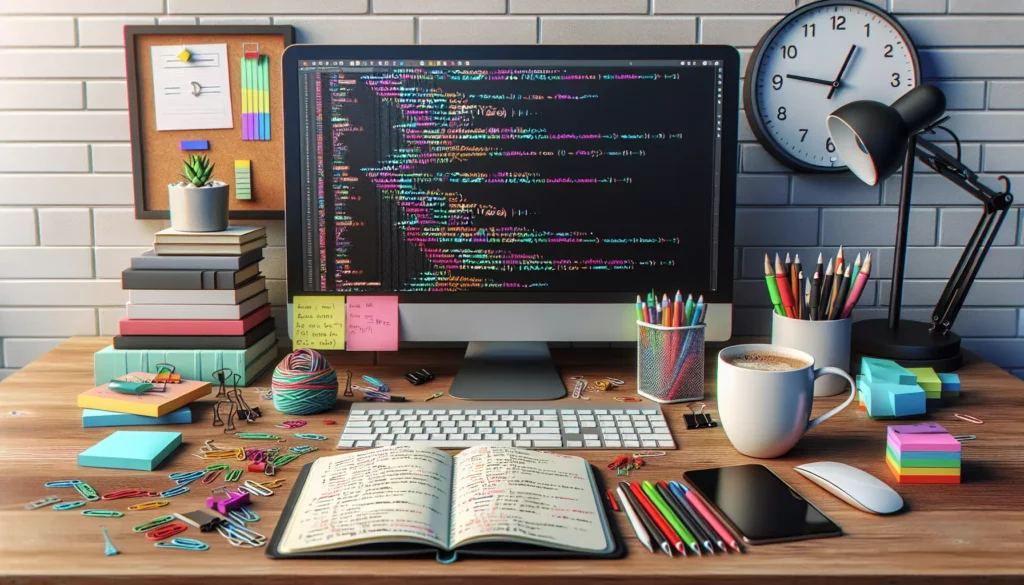
In the fast-paced world of software development, staying organized is crucial for maintaining productivity, reducing errors, and delivering high-quality code. Whether you’re a beginner just starting your coding journey or an experienced developer working on complex projects, having a well-organized approach to your work can make a significant difference in your success. In this comprehensive guide, we’ll explore 99 effective ways to stay organized while coding, helping you streamline your workflow and become a more efficient programmer.
1. Establish a Consistent Folder Structure
Creating a logical and consistent folder structure for your projects is fundamental to staying organized. Here are some tips:
- Use descriptive folder names that clearly indicate their contents
- Separate source code, documentation, and resources into distinct directories
- Create subfolders for different components or modules of your project
- Use version control systems like Git to manage your project structure
2. Implement Version Control
Version control is essential for tracking changes, collaborating with others, and maintaining a history of your code. Consider these practices:
- Use Git or another version control system for all your projects
- Commit frequently with descriptive commit messages
- Create branches for different features or experiments
- Use pull requests for code reviews and merging changes
3. Follow Consistent Naming Conventions
Adhering to naming conventions makes your code more readable and easier to maintain:
- Use descriptive and meaningful names for variables, functions, and classes
- Follow language-specific conventions (e.g., camelCase for JavaScript, snake_case for Python)
- Be consistent with abbreviations and acronyms
- Avoid using single-letter variable names except for simple loop counters
4. Write Clear and Concise Comments
Well-written comments can greatly improve code readability and organization:
- Use comments to explain complex logic or algorithms
- Write descriptive function and class docstrings
- Avoid redundant comments that simply restate the code
- Update comments when you modify the corresponding code
5. Utilize Code Formatting Tools
Consistent code formatting enhances readability and reduces cognitive load:
- Use an automated code formatter like Prettier or Black
- Configure your IDE to format code on save
- Adhere to language-specific style guides (e.g., PEP 8 for Python)
- Use consistent indentation and spacing throughout your codebase
6. Implement Modular Design
Breaking your code into smaller, reusable modules improves organization and maintainability:
- Create separate modules or classes for distinct functionalities
- Use dependency injection to manage relationships between modules
- Follow the Single Responsibility Principle (SRP) for each module
- Implement interfaces or abstract classes to define common behaviors
7. Use Task Management Tools
Keeping track of tasks and priorities is crucial for staying organized:
- Utilize project management tools like Trello or Jira
- Create a to-do list for each coding session
- Use the Pomodoro Technique to manage your time effectively
- Prioritize tasks based on importance and urgency
8. Implement Continuous Integration and Deployment (CI/CD)
CI/CD practices help maintain code quality and streamline the development process:
- Set up automated testing for your codebase
- Use tools like Jenkins, Travis CI, or GitHub Actions for CI/CD
- Implement code linting as part of your CI pipeline
- Automate deployment processes to reduce manual errors
9. Organize Your Development Environment
A well-organized development environment can significantly boost productivity:
- Use virtual environments to manage project-specific dependencies
- Set up your IDE with relevant extensions and plugins
- Customize your workspace layout for optimal efficiency
- Use keyboard shortcuts to speed up common tasks
10. Document Your Code and Processes
Comprehensive documentation is key to maintaining an organized codebase:
- Write a README file for each project explaining its purpose and setup
- Document your API endpoints and their expected inputs/outputs
- Create diagrams to visualize complex system architectures
- Maintain a changelog to track significant changes in your project
11. Implement Code Reviews
Regular code reviews help maintain code quality and organization:
- Establish a code review process for all significant changes
- Use pull requests to facilitate code reviews
- Provide constructive feedback and suggestions for improvement
- Ensure code adheres to project standards and best practices
12. Use Design Patterns
Implementing design patterns can help organize complex codebases:
- Familiarize yourself with common design patterns (e.g., Singleton, Factory, Observer)
- Apply appropriate patterns to solve recurring design problems
- Document the use of design patterns in your code
- Avoid overusing patterns when simpler solutions suffice
13. Implement Error Handling and Logging
Proper error handling and logging contribute to a well-organized codebase:
- Use try-catch blocks to handle exceptions gracefully
- Implement a logging system to track errors and important events
- Use descriptive error messages to aid in debugging
- Create custom exception classes for specific error scenarios
14. Optimize Your Workspace
A clean and organized physical workspace can improve focus and productivity:
- Keep your desk clutter-free and organized
- Use a dual-monitor setup for increased screen real estate
- Invest in ergonomic equipment to reduce physical strain
- Create a comfortable and distraction-free environment
15. Utilize Code Snippets and Templates
Reusable code snippets and templates can save time and maintain consistency:
- Create a library of commonly used code snippets
- Use code snippet managers or IDE features to organize snippets
- Develop project templates for quick setup of new projects
- Share useful snippets and templates with your team
16. Implement Unit Testing
Comprehensive unit testing helps maintain code quality and organization:
- Write unit tests for all significant functions and methods
- Use test-driven development (TDD) when appropriate
- Organize tests in a logical structure mirroring your codebase
- Automate test execution as part of your development workflow
17. Use Static Code Analysis Tools
Static code analysis tools can help identify potential issues and maintain code quality:
- Integrate tools like SonarQube or ESLint into your development process
- Configure analysis rules to match your project’s coding standards
- Address issues and warnings identified by static analysis tools
- Use code coverage tools to ensure comprehensive test coverage
18. Implement Code Refactoring
Regular code refactoring helps maintain a clean and organized codebase:
- Identify and eliminate code smells
- Extract reusable code into separate functions or classes
- Simplify complex conditionals and loops
- Use automated refactoring tools provided by your IDE
19. Utilize Project Management Methodologies
Adopting project management methodologies can improve overall organization:
- Implement Agile methodologies like Scrum or Kanban
- Use sprint planning and retrospectives to organize work
- Break down large tasks into smaller, manageable user stories
- Regularly review and adjust your development process
20. Implement Continuous Learning
Staying up-to-date with best practices and new technologies is crucial for maintaining an organized approach:
- Allocate time for learning new programming languages and tools
- Attend coding workshops and conferences
- Participate in online coding communities and forums
- Read programming books and blogs regularly
21. Use Code Generation Tools
Code generation tools can help maintain consistency and reduce repetitive tasks:
- Utilize code generators for boilerplate code
- Use scaffolding tools to create project structures
- Implement custom code generators for project-specific needs
- Ensure generated code adheres to your coding standards
22. Implement Database Version Control
Keeping your database schema organized is crucial for project maintainability:
- Use database migration tools to manage schema changes
- Version control your database scripts alongside your code
- Document database schema changes in your project changelog
- Implement database seeds for consistent development environments
23. Utilize Code Metrics
Monitoring code metrics can help identify areas for improvement and maintain code quality:
- Track metrics like cyclomatic complexity and code duplication
- Use tools like CodeClimate or SonarQube to analyze code quality
- Set thresholds for acceptable metric values
- Regularly review and address issues identified by code metrics
24. Implement Pair Programming
Pair programming can improve code quality and knowledge sharing:
- Schedule regular pair programming sessions with team members
- Rotate pairs to spread knowledge across the team
- Use pair programming for complex problem-solving and design decisions
- Implement remote pair programming tools for distributed teams
25. Use Mind Mapping Tools
Mind mapping can help organize thoughts and plan complex projects:
- Use tools like MindMeister or XMind to create visual project maps
- Break down large projects into manageable components
- Use mind maps for brainstorming sessions
- Share mind maps with team members for better collaboration
26. Implement Code Ownership
Establishing code ownership can improve accountability and organization:
- Assign primary owners to different modules or components
- Implement a code stewardship model for shared responsibilities
- Document code ownership in project documentation
- Rotate code ownership periodically to spread knowledge
27. Use Feature Flags
Feature flags can help manage the release of new features and maintain code organization:
- Implement a feature flag system in your codebase
- Use feature flags to toggle features on/off in different environments
- Gradually roll out new features using feature flags
- Clean up unused feature flags regularly
28. Implement Code Archiving
Properly archiving old or unused code helps maintain a clean codebase:
- Establish a process for identifying and archiving obsolete code
- Use version control tags to mark archived code versions
- Document the reasons for archiving code
- Maintain an archive of old projects for future reference
29. Use Dependency Management Tools
Proper dependency management is crucial for maintaining an organized project:
- Use package managers like npm, pip, or Maven
- Keep dependencies up-to-date with regular audits
- Use lock files to ensure consistent dependency versions
- Document project dependencies and their purposes
30. Implement Code Style Guides
Adhering to a consistent code style improves readability and maintainability:
- Establish a code style guide for your project or team
- Use automated tools to enforce code style rules
- Include code style checks in your CI/CD pipeline
- Regularly review and update your code style guide
31. Use Project Boards
Project boards help visualize work and manage tasks effectively:
- Utilize tools like GitHub Projects or Trello for task management
- Create columns for different stages of work (e.g., To Do, In Progress, Done)
- Use labels or tags to categorize tasks
- Regularly update and review your project board
32. Implement Code Modularity
Writing modular code improves organization and reusability:
- Break down complex functions into smaller, focused functions
- Use classes and objects to encapsulate related functionality
- Implement interfaces to define clear contracts between modules
- Use dependency injection to manage module relationships
33. Utilize Code Documentation Tools
Automated documentation tools can help maintain up-to-date documentation:
- Use tools like Doxygen or Sphinx to generate documentation from code comments
- Implement automated documentation generation in your CI/CD pipeline
- Regularly review and update generated documentation
- Host documentation on easily accessible platforms
34. Implement Code Reviews
Regular code reviews help maintain code quality and organization:
- Establish a code review process for all significant changes
- Use pull requests to facilitate code reviews
- Provide constructive feedback and suggestions for improvement
- Ensure code adheres to project standards and best practices
35. Use Time Tracking Tools
Tracking your time can help improve productivity and organization:
- Use time tracking tools like Toggl or RescueTime
- Set time limits for different tasks or project components
- Analyze your time usage to identify areas for improvement
- Use time tracking data to estimate future project timelines
36. Implement Code Annotations
Code annotations can provide valuable metadata and improve code organization:
- Use annotations to mark deprecated code or methods
- Implement custom annotations for project-specific needs
- Use annotations to generate documentation or configure frameworks
- Document the purpose and usage of custom annotations
37. Use Code Profiling Tools
Profiling tools can help identify performance bottlenecks and optimize code:
- Use profiling tools to analyze code execution and resource usage
- Identify and optimize performance-critical sections of code
- Implement regular performance testing as part of your development process
- Document performance optimizations and their impact
38. Implement Code Contracts
Code contracts help define and enforce expected behavior:
- Use pre-conditions and post-conditions to define function contracts
- Implement invariants to maintain object state consistency
- Use code contract libraries or language features when available
- Document code contracts in function and class documentation
39. Use Diagrams and Visualizations
Visual representations can help organize complex systems and algorithms:
- Create UML diagrams to visualize class relationships
- Use flowcharts to document complex processes or algorithms
- Implement sequence diagrams to illustrate system interactions
- Use tools like PlantUML or draw.io for diagram creation
40. Implement Code Generators
Custom code generators can help maintain consistency and reduce repetitive tasks:
- Create code generators for repetitive boilerplate code
- Use template engines to generate code from predefined templates
- Implement code generators as part of your build process
- Document the usage and configuration of custom code generators
41. Use Code Complexity Metrics
Monitoring code complexity helps maintain a manageable codebase:
- Use tools to measure cyclomatic complexity and cognitive complexity
- Set thresholds for acceptable complexity levels
- Refactor complex code to improve readability and maintainability
- Include complexity metrics in code review processes
42. Implement Continuous Documentation
Keeping documentation up-to-date is crucial for maintaining an organized project:
- Update documentation as part of your development process
- Use automated tools to generate API documentation
- Implement a documentation review process
- Use version control for documentation files
43. Use Code Smell Detection Tools
Identifying and addressing code smells helps maintain code quality:
- Use tools like SonarQube or ReSharper to detect code smells
- Address identified code smells through refactoring
- Educate team members on common code smells and how to avoid them
- Include code smell detection in your CI/CD pipeline
44. Implement Code Ownership Rotation
Rotating code ownership can improve knowledge sharing and code quality:
- Regularly rotate primary ownership of different modules or components
- Implement pair programming sessions during ownership transitions
- Document code ownership changes and reasons
- Use code ownership rotation as a learning opportunity for team members
45. Use Code Metrics Dashboards
Visualizing code metrics can help track progress and identify areas for improvement:
- Implement dashboards to display key code quality metrics
- Use tools like Grafana or Kibana to create custom dashboards
- Include metrics such as test coverage, code complexity, and bug counts
- Regularly review and act on insights from metric dashboards
46. Implement Code Reuse Strategies
Effective code reuse can improve efficiency and maintain consistency:
- Create reusable libraries for common functionality
- Implement a component-based architecture for frontend development
- Use design patterns to promote code reuse
- Document and share reusable code components within your team
47. Use Code Review Checklists
Checklists can help ensure thorough and consistent code reviews:
- Create a standard code review checklist for your team
- Include items such as code style, performance, and security considerations
- Customize checklists for different types of changes or components
- Regularly update and improve your code review checklists
48. Implement Code Deprecation Processes
Properly deprecating old code helps maintain a clean and organized codebase:
- Establish a process for identifying and marking deprecated code
- Use deprecation annotations or comments to flag outdated code
- Set timelines for removing deprecated code
- Communicate deprecations to team members and users
49. Use Code Formatting Hooks
Automated formatting hooks ensure consistent code style:
- Implement pre-commit hooks to format code before committing
- Use tools like husky to manage Git hooks
- Configure IDE settings to format code on save
- Ensure all team members use consistent formatting tools and settings
50. Implement Code Review Automation
Automating parts of the code review process can improve efficiency:
- Use tools like Reviewable or Gerrit for automated code review assignments
- Implement bots to perform initial code style and quality checks
- Automate the merging of approved changes
- Use code review analytics to identify bottlenecks in the review process
51. Use Code Dependency Visualization
Visualizing code dependencies can help manage complex codebases:
- Use tools like Graphviz or Dependency-cruiser to generate dependency graphs
- Analyze dependency graphs to identify circular dependencies
- Use visualizations to plan refactoring efforts
- Include dependency visualizations in project documentation
52. Implement Code Ownership Documentation
Clearly documenting code ownership improves accountability and organization:
- Maintain a CODEOWNERS file in your repository
- Use code comments to indicate ownership of specific functions or classes
- Include ownership information in project documentation
- Regularly review and update code ownership information
53. Use Code Snippet Libraries
Maintaining a library of code snippets can improve efficiency:
- Create a shared repository of commonly used code snippets
- Use tools like GitHub Gists or GitLab Snippets to manage snippets
- Categorize snippets for easy searching and retrieval
- Regularly review and update snippet libraries
54. Implement Code Review Metrics
Tracking code review metrics can help improve the review process:
- Monitor metrics such as review time, number of comments, and approval rate
- Use tools like ReviewBoard or GitHub Insights for code review analytics
- Set goals for improving code review efficiency and effectiveness
- Regularly discuss code review metrics with your team
55. Use Code Annotation Tools
Code annotation tools can improve code documentation and readability:
- Use tools like JSDoc or Sphinx to generate documentation from code annotations
- Implement custom annotations for project-specific needs
- Use type annotations in dynamically-typed languages for improved clarity
- Include code annotations in your code review process
56. Implement Code Refactoring Sprints
Dedicating time to refactoring helps maintain code quality:
- Schedule regular refactoring sprints or dedicated time
- Prioritize areas of the codebase that need improvement
- Use refactoring as an opportunity for knowledge sharing
- Document major refactoring efforts and their impact
57. Use Code Coverage Tools
Monitoring code coverage helps ensure comprehensive testing:
- Implement code coverage tools in your testing process
- Set code coverage goals for your project
- Use code coverage reports to identify under-tested areas
- Include code coverage metrics in your CI/CD pipeline
58. Implement Code Review Templates
Templates can help standardize the code review process:
- Create templates for pull request descriptions
- Implement checklists for common review criteria
- Use templates to ensure all necessary information is provided
- Regularly update templates based on team feedback
59. Use Code Complexity Analysis
Analyzing code complexity helps identify areas for improvement:
- Use tools like SonarQube or Codacy for complexity analysis
- Set thresholds for acceptable complexity levels
- Address overly complex code through refactoring
- Include complexity analysis in your code review process
60. Implement Code Documentation Reviews
Reviewing documentation helps maintain its quality and relevance:
- Include documentation reviews in your code review process
- Verify that documentation is up-to-date with code changes
- Check for clarity and completeness in documentation
- Encourage team members to suggest documentation improvements
61. Use Code Smell Detectors
Automated tools can help identify potential code issues:
- Implement code smell detection tools in your development process
- Use tools like PMD or ESLint to detect common code smells
- Address identified code smells through refactoring
- Educate team members on common code smells and how to avoid them
62. Implement Code Review Pair Programming
Combining code reviews with pair programming can improve quality:
- Schedule pair programming sessions for complex code reviews
- Use screen sharing tools for remote pair review sessions
- Rotate pairs to spread knowledge across the team
- Document insights and decisions from pair review sessions
63. Use Code Metric Trending
Tracking code metrics over time helps identify trends:
- Implement tools to track code metrics over time
- Use trend analysis to identify areas of improvement or degradation
- Set goals for improving key metrics
- Regularly review metric trends with your team
64. Implement Code Review Feedback Loops
Establishing feedback loops improves the code review process:
- Encourage reviewers to provide timely and constructive feedback
- Implement a system for tracking and addressing review comments
- Follow up on review feedback to ensure issues are resolved
- Regularly solicit feedback on the review process itself
65. Use Code Duplication Detection
Identifying and addressing code duplication improves maintainability:
- Use tools like CPD or Simian to detect code duplication
- Set thresholds for acceptable levels of code duplication
- Refactor duplicated code into reusable functions or classes
- Include duplication detection in your CI/CD pipeline
66. Implement Code Review Rotations
Rotating code reviewers helps spread knowledge and fresh perspectives:
- Establish a system for rotating code review assignments
- Ensure each team member reviews different parts of the codebase
- Use review rotations as a learning opportunity for junior developers
- Balance workload and expertise when assigning reviews
67. Use Code Readability Metrics
Measuring code readability helps maintain clear and understandable code:
- Implement tools to measure code readability (e.g., Flesch-Kincaid readability tests)
- Set readability goals for your codebase
- Use readability metrics to identify areas for improvement
- Include readability considerations in your code review process
68. Implement Code Review Etiquette
Establishing review etiquette promotes constructive feedback:
- Create guidelines for providing respectful and constructive feedback
- Encourage reviewers to explain the reasoning behind their suggestions
- Promote a culture of learning and improvement through reviews
- Address any conflicts or misunderstandings promptly and professionally
69. Use Code Dependency Management
Effective dependency management helps maintain a stable codebase:
- Regularly update and audit project dependencies
- Use tools like npm audit or bundler-audit to check for security vulnerabilities
- Implement strategies for managing transitive dependencies
- Document reasons for specific dependency versions or constraints