The Importance of Design Patterns in Coding: A Comprehensive Guide
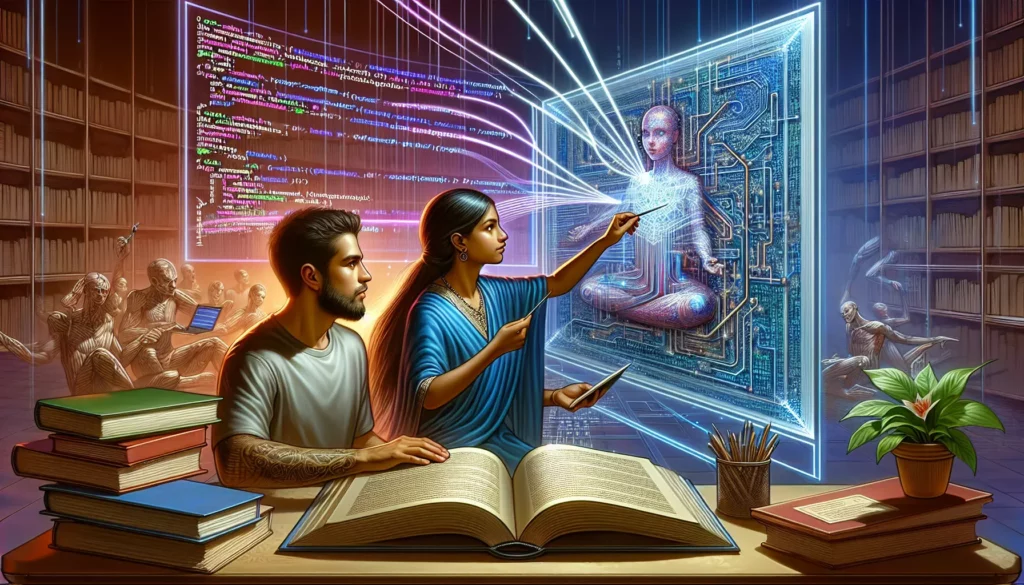
In the ever-evolving world of software development, mastering the art of writing efficient, maintainable, and scalable code is crucial. One of the key concepts that help developers achieve these goals is the use of design patterns. In this comprehensive guide, we’ll explore the importance of design patterns in coding, their benefits, and how they can significantly improve your programming skills.
What Are Design Patterns?
Design patterns are reusable solutions to common problems that occur in software design. They are not specific code implementations but rather templates or blueprints that can be applied to various programming challenges. These patterns have been developed and refined over time by experienced software developers to address recurring issues in software development.
The concept of design patterns was popularized by the book “Design Patterns: Elements of Reusable Object-Oriented Software” by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, collectively known as the “Gang of Four” (GoF). This seminal work introduced 23 classic design patterns that are still widely used today.
The Importance of Design Patterns in Coding
Now that we have a basic understanding of what design patterns are, let’s delve into why they are so important in the world of coding:
1. Promotes Best Practices
Design patterns encapsulate best practices developed by experienced programmers over many years. By using these patterns, developers can benefit from the collective wisdom of the software development community. This leads to more robust, efficient, and maintainable code.
2. Provides a Common Vocabulary
Design patterns give developers a shared language to discuss complex software designs. Instead of explaining a solution in detail, a developer can simply mention the name of a design pattern, and other team members will immediately understand the general structure and intent of the code.
3. Speeds Up Development Process
By providing tried-and-tested solutions to common problems, design patterns can significantly speed up the development process. Developers don’t need to reinvent the wheel for every new project; they can apply these patterns to solve similar problems across different applications.
4. Improves Code Readability and Maintainability
When used correctly, design patterns can make code more organized and easier to understand. This improved structure makes it easier for other developers (or even the original developer after some time) to read, maintain, and modify the code.
5. Facilitates Scalability
Many design patterns are specifically created to make software systems more flexible and scalable. They allow for easier addition of new features or modifications to existing ones without requiring a complete overhaul of the system.
6. Enhances Software Architecture
Design patterns often form the building blocks of larger architectural patterns. Understanding and using design patterns can help developers create more robust and flexible software architectures.
7. Encourages Code Reusability
Design patterns promote the creation of reusable components. This not only saves time but also reduces the likelihood of errors, as these components have been tested and refined over time.
Common Design Patterns
While there are numerous design patterns, they are typically categorized into three main types:
1. Creational Patterns
These patterns deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. Some examples include:
- Singleton Pattern
- Factory Method Pattern
- Abstract Factory Pattern
- Builder Pattern
- Prototype Pattern
2. Structural Patterns
Structural patterns are concerned with how classes and objects are composed to form larger structures. Examples include:
- Adapter Pattern
- Bridge Pattern
- Composite Pattern
- Decorator Pattern
- Facade Pattern
3. Behavioral Patterns
These patterns are about identifying common communication patterns between objects and realizing these patterns. Some examples are:
- Observer Pattern
- Strategy Pattern
- Command Pattern
- State Pattern
- Template Method Pattern
Implementing Design Patterns: A Practical Example
To better understand how design patterns work in practice, let’s look at an example of the Singleton pattern, one of the simplest and most commonly used design patterns.
The Singleton pattern ensures that a class has only one instance and provides a global point of access to that instance. This can be useful in scenarios where you need to coordinate actions across a system, such as managing a shared resource or a central data store.
Here’s a simple implementation of the Singleton pattern in Python:
class Singleton:
_instance = None
def __new__(cls):
if cls._instance is None:
cls._instance = super(Singleton, cls).__new__(cls)
cls._instance.value = None
return cls._instance
def set_value(self, value):
self.value = value
def get_value(self):
return self.value
# Usage
s1 = Singleton()
s1.set_value(42)
s2 = Singleton()
print(s2.get_value()) # Output: 42
In this example, no matter how many times we create a Singleton object, we always get the same instance. This ensures that there’s only one global instance of the class, which can be accessed from anywhere in the code.
Best Practices for Using Design Patterns
While design patterns are powerful tools, it’s important to use them judiciously. Here are some best practices to keep in mind:
1. Understand the Problem First
Before applying a design pattern, make sure you fully understand the problem you’re trying to solve. Don’t use a pattern just because it’s popular or because you’re familiar with it.
2. Keep It Simple
Sometimes, a simple solution is better than a complex design pattern. Don’t overcomplicate your code by forcing a pattern where it’s not needed.
3. Consider the Context
The effectiveness of a design pattern can vary depending on the context of your project. Consider factors like the programming language, the specific requirements of your project, and your team’s expertise.
4. Combine Patterns
Often, the best solutions come from combining multiple design patterns. Don’t be afraid to mix and match patterns to create the most effective solution for your specific problem.
5. Document Your Use of Patterns
When you use a design pattern, make sure to document it in your code comments or project documentation. This helps other developers (or your future self) understand the reasoning behind your design decisions.
Design Patterns and Modern Software Development
As software development practices evolve, so do the applications and interpretations of design patterns. In modern software development, design patterns continue to play a crucial role, but their use has adapted to new paradigms and technologies:
1. Microservices Architecture
In the world of microservices, design patterns are applied not just within individual services, but also in how these services interact. Patterns like API Gateway, Circuit Breaker, and Saga are specifically tailored for microservices architectures.
2. Functional Programming
While many classic design patterns were conceived with object-oriented programming in mind, they’re being reinterpreted and adapted for functional programming paradigms. For instance, the Strategy pattern in OOP can be implemented simply as a higher-order function in functional programming.
3. Reactive Programming
Reactive programming introduces new patterns for dealing with data streams and propagation of change. The Observer pattern, for example, is fundamental to many reactive programming implementations.
4. Cloud-Native Development
Cloud-native development has given rise to new patterns for designing scalable, resilient, and easily deployable applications. Patterns like the Sidecar, Ambassador, and Leader Election are particularly relevant in cloud environments.
Learning Design Patterns
For developers looking to improve their skills and write better code, learning design patterns is an excellent investment of time. Here are some strategies for mastering design patterns:
1. Study the Classics
Start with the classic GoF patterns. While some may seem dated, understanding these patterns provides a solid foundation for learning more modern patterns.
2. Practice Implementation
Don’t just read about patterns; implement them in your preferred programming language. This hands-on experience will help you understand when and how to apply these patterns in real-world scenarios.
3. Analyze Existing Code
Look for design patterns in open-source projects or frameworks you use. This will give you insight into how experienced developers apply these patterns in production code.
4. Join Developer Communities
Participate in online forums, attend meetups, or join coding groups where you can discuss design patterns with other developers. This can provide valuable insights and different perspectives on pattern usage.
5. Keep Learning
The world of software development is constantly evolving. Stay updated with new patterns that emerge to address modern development challenges.
Conclusion
Design patterns are an essential tool in a developer’s toolkit. They provide proven solutions to common problems, promote best practices, and can significantly improve the quality of your code. By understanding and correctly applying design patterns, you can write more efficient, maintainable, and scalable software.
However, it’s important to remember that design patterns are not a silver bullet. They should be used judiciously, always keeping in mind the specific needs of your project and the principle of keeping things as simple as possible.
As you continue your journey in software development, make it a point to learn and understand various design patterns. Practice implementing them in your projects, and over time, you’ll develop an intuition for when and how to apply them effectively. This knowledge will not only make you a better programmer but also a more valuable team member in any software development project.
Remember, the goal is not to use design patterns everywhere, but to know them well enough to apply the right pattern in the right situation. As you gain experience, you’ll find that design patterns become an invaluable part of your problem-solving toolkit, helping you create more robust, flexible, and maintainable software solutions.