Mastering Coding Katas: Sharpen Your Programming Skills and Ace Technical Interviews
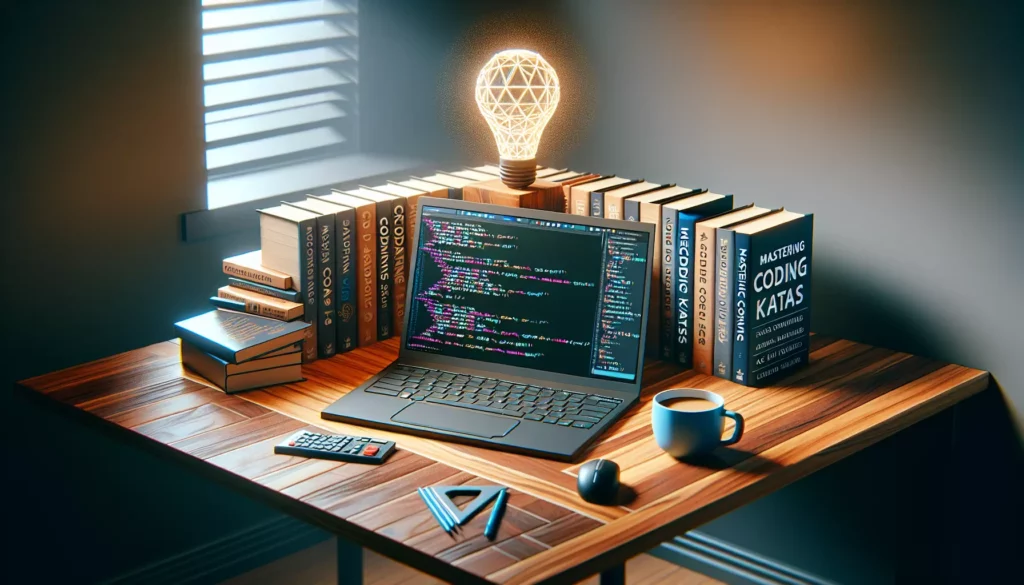
In the ever-evolving world of software development, staying sharp and continuously improving your coding skills is crucial. Whether you’re a beginner looking to establish a solid foundation or an experienced developer preparing for technical interviews at top tech companies, one powerful technique stands out: coding katas. In this comprehensive guide, we’ll explore what coding katas are, how they can benefit your programming journey, and how to effectively incorporate them into your learning routine.
What Are Coding Katas?
Coding katas are small, focused programming exercises designed to help developers hone their skills through repetition and practice. The term “kata” originates from martial arts, where it refers to a sequence of movements that are practiced repeatedly to perfect form and technique. Similarly, coding katas involve solving specific programming problems or implementing particular algorithms to reinforce coding patterns, improve problem-solving abilities, and enhance overall programming proficiency.
These exercises typically have the following characteristics:
- Well-defined problem statements
- Small scope, usually solvable within an hour or less
- Focus on a particular programming concept or technique
- Encourage clean code and best practices
- Can be repeated multiple times to reinforce learning
The Benefits of Practicing Coding Katas
Incorporating coding katas into your learning routine can provide numerous benefits for programmers at all levels. Let’s explore some of the key advantages:
1. Skill Reinforcement and Muscle Memory
Just as musicians practice scales or athletes drill specific movements, coding katas help programmers build muscle memory for common coding patterns and techniques. By repeatedly solving similar problems, you internalize efficient approaches and best practices, making them second nature when tackling real-world programming challenges.
2. Problem-Solving Practice
Coding katas expose you to a wide variety of programming problems, helping you develop and refine your problem-solving skills. As you work through different katas, you’ll learn to break down complex problems into smaller, manageable steps and apply appropriate algorithms and data structures to solve them efficiently.
3. Clean Code and Best Practices
Many coding katas emphasize writing clean, readable, and maintainable code. By focusing on these aspects in a controlled environment, you’ll develop good coding habits that transfer to your larger projects. This includes proper naming conventions, code organization, and adherence to principles like DRY (Don’t Repeat Yourself) and SOLID.
4. Language and Framework Familiarity
Coding katas provide an excellent opportunity to practice and become more proficient in specific programming languages or frameworks. By solving katas in different languages, you can improve your syntax knowledge, discover language-specific features, and gain a deeper understanding of each language’s strengths and idioms.
5. Interview Preparation
For those aiming to land positions at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), coding katas are invaluable for interview preparation. Many technical interviews involve solving algorithmic problems on the spot, and practicing katas can help you become more comfortable with this format while expanding your problem-solving toolkit.
6. Continuous Learning and Growth
The tech industry is constantly evolving, and coding katas provide a structured way to stay up-to-date with new concepts, algorithms, and best practices. By regularly challenging yourself with diverse katas, you ensure continuous learning and growth throughout your career.
How to Get Started with Coding Katas
Now that we understand the benefits of coding katas, let’s explore how you can incorporate them into your learning routine:
1. Choose a Platform
Several online platforms offer coding katas and programming challenges. Some popular options include:
- LeetCode
- HackerRank
- CodeWars
- Exercism
- Project Euler
These platforms provide a wide range of problems, from beginner to advanced levels, and often include features like leaderboards, discussion forums, and solution comparisons.
2. Start with Beginner-Friendly Katas
If you’re new to coding katas, begin with simpler problems to build confidence and familiarize yourself with the format. Look for katas tagged as “easy” or “beginner” on your chosen platform. Some examples of beginner-friendly katas include:
- FizzBuzz
- Reverse a String
- Calculate Factorial
- Find the Largest Number in an Array
3. Establish a Regular Practice Routine
Consistency is key when it comes to improving your coding skills. Set aside dedicated time for kata practice, such as 30 minutes to an hour each day. You can also try the “Code Kata of the Day” approach, where you solve one new kata every day to maintain a steady learning pace.
4. Focus on Understanding, Not Just Solving
While it’s satisfying to solve a kata quickly, the real value comes from understanding the problem and exploring different approaches. After solving a kata, take time to:
- Analyze your solution and identify areas for improvement
- Study other developers’ solutions to learn alternative approaches
- Reflect on the underlying concepts and algorithms used
5. Gradually Increase Difficulty
As you become more comfortable with basic katas, challenge yourself by tackling more complex problems. Look for katas that introduce new concepts or require more advanced algorithms and data structures. This progression will help you continue growing and expanding your skillset.
6. Implement Time Constraints
To simulate interview conditions and improve your problem-solving speed, try solving katas within specific time limits. Start with generous time frames and gradually reduce them as you become more proficient. This practice will help you manage time pressure during actual technical interviews.
Popular Types of Coding Katas
Coding katas cover a wide range of programming concepts and problem types. Here are some popular categories you’re likely to encounter:
1. String Manipulation
These katas involve working with strings, including tasks like reversing strings, finding substrings, or implementing string compression algorithms. Example kata: Implement a function that checks if a given string is a palindrome.
2. Array and List Operations
Katas in this category focus on manipulating arrays or lists, such as sorting, searching, or performing various transformations. Example kata: Implement a function to rotate an array by a given number of positions.
3. Mathematical Problems
These katas involve implementing mathematical algorithms or solving number-based problems. Example kata: Write a function to find the nth Fibonacci number.
4. Tree and Graph Algorithms
More advanced katas often involve working with tree or graph data structures, implementing traversal algorithms, or solving graph-based problems. Example kata: Implement a depth-first search algorithm for a binary tree.
5. Dynamic Programming
Dynamic programming katas focus on optimization problems that can be solved by breaking them down into smaller subproblems. Example kata: Implement the coin change problem to find the minimum number of coins needed to make a given amount.
6. Object-Oriented Design
Some katas emphasize object-oriented programming principles and design patterns. Example kata: Design and implement a simple deck of cards class with methods for shuffling and dealing.
Advanced Techniques for Mastering Coding Katas
As you become more experienced with coding katas, consider these advanced techniques to further enhance your skills:
1. Implement Multiple Solutions
For each kata, try to come up with multiple solutions using different approaches or algorithms. This practice will broaden your problem-solving toolkit and help you understand the trade-offs between different implementations.
2. Analyze Time and Space Complexity
For each solution you develop, analyze its time and space complexity using Big O notation. This will help you understand the efficiency of your algorithms and prepare you for discussions about optimization during technical interviews.
3. Write Unit Tests
Practice test-driven development (TDD) by writing unit tests for your kata solutions. This will improve your testing skills and ensure your implementations are correct and robust.
4. Refactor and Optimize
After implementing a working solution, spend time refactoring your code to improve its readability, efficiency, and maintainability. This practice will sharpen your code quality skills and help you write production-ready code.
5. Explain Your Solution
Practice explaining your solution out loud, as if you were presenting it to an interviewer. This will improve your communication skills and deepen your understanding of the problem-solving process.
6. Collaborate and Review
Engage with the coding kata community by participating in code reviews, discussing solutions with other developers, and contributing to open-source kata repositories. This collaboration will expose you to diverse perspectives and approaches.
Integrating Coding Katas with AlgoCademy
AlgoCademy, as a comprehensive coding education platform, provides an ideal environment for incorporating coding katas into your learning journey. Here’s how you can leverage AlgoCademy’s features to maximize the benefits of coding katas:
1. AI-Powered Assistance
Take advantage of AlgoCademy’s AI-powered assistance to get hints and guidance when you’re stuck on a kata. This feature can help you learn new problem-solving techniques and overcome challenges without getting frustrated.
2. Step-by-Step Guidance
Use AlgoCademy’s step-by-step guidance feature to break down complex katas into manageable steps. This approach will help you develop a structured problem-solving process that you can apply to more challenging problems in the future.
3. Interactive Coding Environment
Practice your coding katas directly in AlgoCademy’s interactive coding environment. This allows you to write, run, and debug your code in real-time, simulating a realistic development experience.
4. Progress Tracking
Utilize AlgoCademy’s progress tracking features to monitor your improvement over time. Set goals for the number of katas you want to complete each week and track your success rate to stay motivated and identify areas for improvement.
5. Curated Learning Paths
Follow AlgoCademy’s curated learning paths that incorporate coding katas alongside other learning resources. This holistic approach ensures you’re developing a well-rounded skill set that combines theoretical knowledge with practical coding experience.
6. Community Engagement
Participate in AlgoCademy’s community forums to discuss kata solutions, share insights, and learn from other learners. This collaborative environment can enhance your learning experience and expose you to diverse problem-solving approaches.
Conclusion
Coding katas are a powerful tool for improving your programming skills, preparing for technical interviews, and staying sharp in the ever-evolving world of software development. By incorporating regular kata practice into your learning routine, you’ll develop stronger problem-solving abilities, write cleaner code, and build the confidence needed to tackle complex programming challenges.
Remember that the key to mastering coding katas is consistency and reflection. Make kata practice a regular part of your coding routine, challenge yourself with increasingly difficult problems, and always take time to analyze and learn from each solution you develop.
Whether you’re a beginner looking to establish a solid foundation or an experienced developer preparing for interviews at top tech companies, coding katas can play a crucial role in your journey to becoming a more skilled and confident programmer. So, pick a platform, choose your first kata, and start sharpening your coding skills today!