The Art of Effective Code Commenting: A Comprehensive Guide
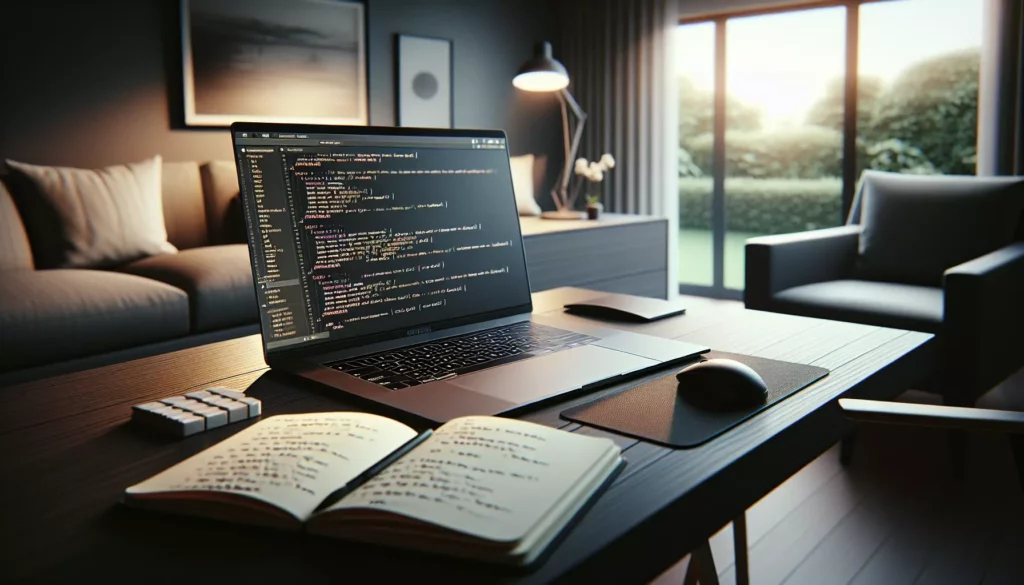
In the world of programming, writing clean and efficient code is only half the battle. The other crucial aspect that often separates good programmers from great ones is the ability to write effective comments. Comments are essential for making your code more readable, maintainable, and understandable, not just for others but also for your future self. In this comprehensive guide, we’ll explore the art of effective code commenting, providing you with valuable tips and best practices to elevate your coding skills.
Why Are Code Comments Important?
Before diving into the tips, let’s briefly discuss why code comments are so crucial:
- Improved Readability: Comments help other developers (and you) understand the code’s purpose and functionality more quickly.
- Easier Maintenance: Well-commented code is easier to update, debug, and maintain over time.
- Knowledge Transfer: Comments serve as a form of documentation, helping to transfer knowledge within a team or to future maintainers.
- Code Review Assistance: Good comments can make the code review process smoother and more efficient.
- Learning Tool: For beginners, reading well-commented code can be an excellent way to learn programming concepts and best practices.
15 Tips for Effective Code Commenting
1. Comment on the Why, Not the What
One of the most common mistakes in code commenting is explaining what the code does instead of why it does it. The code itself should be clear enough to show what it’s doing. Your comments should focus on the reasoning behind the implementation, any constraints, or important considerations.
Bad example:
// Increment i by 1
i++;
Good example:
// Increment the loop counter to move to the next array element
i++;
2. Keep Comments Concise and Clear
While it’s important to provide enough information, overly verbose comments can be just as problematic as no comments at all. Aim for clarity and conciseness in your comments. Use simple language and avoid unnecessary jargon.
Bad example:
// This function takes two parameters, a and b, which are both integers.
// It then proceeds to add these two integers together using the addition
// operator and returns the result of this mathematical operation.
int add(int a, int b) {
return a + b;
}
Good example:
// Adds two integers and returns the sum
int add(int a, int b) {
return a + b;
}
3. Use Proper Grammar and Spelling
Treat your comments as you would any professional writing. Use correct grammar, spelling, and punctuation. This not only makes your comments more readable but also demonstrates attention to detail and professionalism.
4. Update Comments Along with Code
As your code evolves, make sure to update the associated comments. Outdated comments can be misleading and potentially more harmful than no comments at all. Whenever you modify code, review and update the relevant comments to ensure they remain accurate.
5. Use TODO Comments for Temporary Solutions
When you need to implement a quick fix or a temporary solution, use TODO comments to mark areas that require further attention or improvement. This helps you and your team keep track of pending tasks.
// TODO: Implement proper error handling for network failures
try {
// API call here
} catch (Exception e) {
System.out.println("Error occurred");
}
6. Leverage Documentation Comments
Many programming languages support special documentation comments that can be used to generate automatic documentation. For example, in Java, you can use Javadoc comments, while in Python, you can use docstrings. These are particularly useful for APIs and libraries.
Java example:
/**
* Calculates the area of a circle.
*
* @param radius The radius of the circle.
* @return The area of the circle.
* @throws IllegalArgumentException if radius is negative.
*/
public double calculateCircleArea(double radius) {
if (radius < 0) {
throw new IllegalArgumentException("Radius cannot be negative");
}
return Math.PI * radius * radius;
}
7. Use Inline Comments Sparingly
While inline comments (comments on the same line as code) can be useful, they should be used sparingly. They’re best reserved for clarifying complex lines of code that might not be immediately obvious.
int result = (a + b) * c; // Parentheses ensure addition happens before multiplication
8. Avoid Redundant Comments
Don’t state the obvious in your comments. If the code is self-explanatory, adding a comment might just add unnecessary clutter.
Bad example:
// Declare an integer variable named count and initialize it to 0
int count = 0;
9. Use Block Comments for Complex Logic
For complex algorithms or business logic, consider using block comments to explain the overall approach before diving into the code details.
/*
* Bubble Sort Algorithm:
* 1. Iterate through the array
* 2. Compare adjacent elements
* 3. Swap if they are in the wrong order
* 4. Repeat until no swaps are needed
*/
void bubbleSort(int[] arr) {
// Implementation here
}
10. Comment on Magic Numbers and Constants
When using magic numbers (hard-coded numerical values) in your code, always add a comment explaining what they represent. Better yet, replace magic numbers with named constants.
// Maximum allowed login attempts
private static final int MAX_LOGIN_ATTEMPTS = 3;
11. Use Comments to Mark Code Sections
In larger functions or files, use comments to separate and label different sections of your code. This improves readability and helps others navigate your code more easily.
// Initialize variables
int x = 0;
int y = 0;
// Main processing loop
while (condition) {
// Processing logic here
}
// Cleanup and resource release
closeResources();
12. Avoid Commenting Out Code
Instead of commenting out large blocks of code, consider using version control systems to manage different versions of your code. If you must keep old code around temporarily, use clear TODO comments explaining why the code is commented out and when it can be removed.
13. Use Descriptive Function and Variable Names
While this isn’t directly related to commenting, using descriptive names for functions and variables can reduce the need for comments. Clear, self-explanatory names can often convey the purpose of the code without additional explanation.
// Less clear, requires a comment
int d; // Number of days since last login
// More clear, self-explanatory
int daysSinceLastLogin;
14. Comment on Edge Cases and Exceptions
When your code handles specific edge cases or throws exceptions, make sure to comment on these scenarios. This helps other developers understand the full behavior of your code, including error handling.
/**
* Divides two numbers.
*
* @param dividend The number to be divided.
* @param divisor The number to divide by.
* @return The result of the division.
* @throws ArithmeticException if divisor is zero.
*/
public double divide(double dividend, double divisor) {
if (divisor == 0) {
throw new ArithmeticException("Cannot divide by zero");
}
return dividend / divisor;
}
15. Use Comments to Explain Regular Expressions
Regular expressions can be notoriously difficult to read. When using regex in your code, always add a comment explaining what the pattern is matching.
// Regex to validate email addresses
String emailRegex = "^[A-Za-z0-9+_.-]+@[A-Za-z0-9.-]+$";
The Role of Comments in Coding Education
In the context of coding education platforms like AlgoCademy, effective commenting plays a crucial role in the learning process. Here’s how:
- Learning by Example: When students encounter well-commented code in tutorials or examples, they not only learn the programming concepts but also absorb good commenting practices.
- Understanding Complex Algorithms: For advanced topics like algorithm implementation, comments can help break down complex logic into manageable chunks, making it easier for learners to grasp difficult concepts.
- Preparing for Technical Interviews: Many technical interviews involve discussing or writing code. Being able to effectively comment on your code during an interview demonstrates professionalism and clear communication skills.
- Collaborative Learning: In pair programming exercises or group projects, good commenting habits facilitate better collaboration and knowledge sharing among students.
- Code Review Skills: Learning to write and read good comments helps students develop critical code review skills, which are essential in professional software development.
Conclusion
Mastering the art of effective code commenting is a valuable skill that can significantly enhance your capabilities as a programmer. It’s not just about following rules, but about developing a mindset of clear communication through your code. As you progress in your coding journey, whether you’re using platforms like AlgoCademy or working on personal projects, make it a habit to write meaningful comments.
Remember, the goal of commenting is to make your code more understandable and maintainable. By following these tips and continuously refining your commenting skills, you’ll not only become a better programmer but also a more valuable team member in any software development project.
As you practice and improve your coding skills, pay attention to how experienced developers comment their code. Analyze open-source projects, participate in code reviews, and always be open to feedback on your commenting style. With time and practice, writing effective comments will become second nature, elevating the quality of your code and your overall proficiency as a software developer.