How to Improve Your Coding Challenge Performance: A Comprehensive Guide
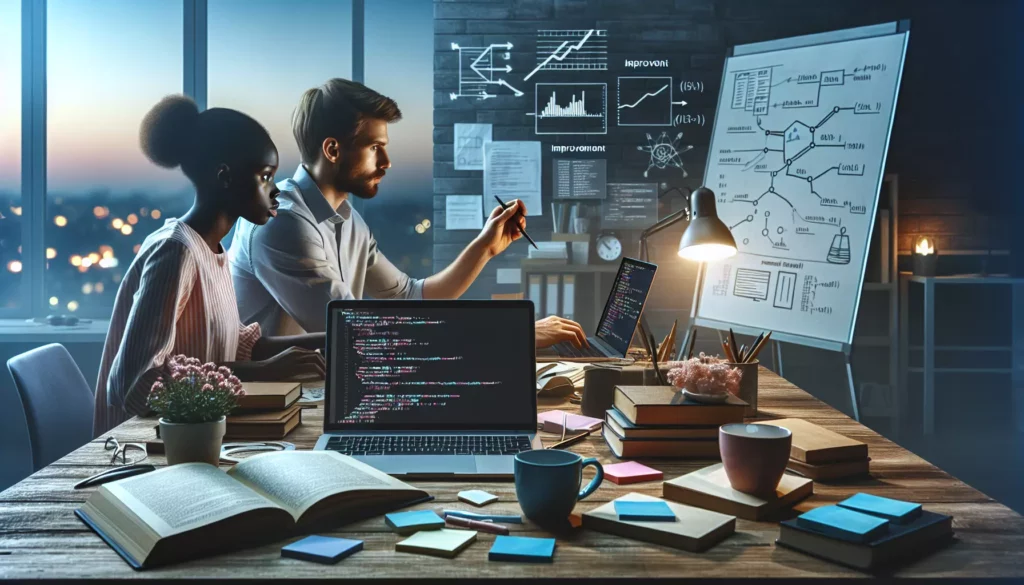
In today’s competitive tech landscape, mastering coding challenges has become an essential skill for aspiring programmers and seasoned developers alike. Whether you’re preparing for technical interviews at top tech companies or simply looking to sharpen your problem-solving abilities, improving your performance in coding challenges can significantly boost your career prospects. This comprehensive guide will explore various strategies, techniques, and resources to help you excel in coding challenges and take your programming skills to the next level.
Understanding the Importance of Coding Challenges
Before diving into improvement strategies, it’s crucial to understand why coding challenges matter. These challenges serve multiple purposes:
- They assess your problem-solving skills
- They evaluate your ability to write clean, efficient code
- They test your knowledge of data structures and algorithms
- They simulate real-world programming scenarios
- They help companies identify top talent during the hiring process
By excelling in coding challenges, you demonstrate your technical prowess and increase your chances of landing coveted positions at leading tech companies, often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google) or MANGA (Microsoft, Amazon, Netflix, Google, Apple).
1. Develop a Strong Foundation in Data Structures and Algorithms
The cornerstone of success in coding challenges is a solid understanding of data structures and algorithms. These fundamental concepts form the building blocks of efficient problem-solving in computer science. To improve your foundation:
- Study common data structures like arrays, linked lists, stacks, queues, trees, and graphs
- Master basic algorithms such as sorting, searching, and graph traversal
- Understand time and space complexity analysis (Big O notation)
- Practice implementing these concepts from scratch in your preferred programming language
Resources like AlgoCademy offer structured courses and interactive tutorials to help you build this crucial foundation. Their step-by-step approach and AI-powered assistance can guide you through complex topics, ensuring a thorough understanding of these core concepts.
2. Practice Regularly with Online Coding Platforms
Consistent practice is key to improving your coding challenge performance. Numerous online platforms offer a wide range of problems to solve, catering to various difficulty levels and topics. Some popular platforms include:
- LeetCode
- HackerRank
- CodeSignal
- Codewars
- Project Euler
Set aside dedicated time each day or week to solve problems on these platforms. Start with easier problems and gradually progress to more challenging ones. This consistent practice will help you become more comfortable with different problem types and improve your problem-solving speed.
3. Learn and Apply Problem-Solving Techniques
Developing a structured approach to problem-solving can significantly enhance your coding challenge performance. Some effective techniques include:
- Understand the problem thoroughly before coding
- Break down complex problems into smaller, manageable sub-problems
- Identify patterns and similarities to problems you’ve solved before
- Consider multiple approaches and evaluate their trade-offs
- Use pseudocode to outline your solution before implementation
AlgoCademy’s interactive coding tutorials often incorporate these problem-solving techniques, guiding you through the thought process of tackling complex challenges step by step.
4. Optimize Your Code for Efficiency
Writing correct code is just the first step; optimizing it for efficiency is equally important in coding challenges. To improve your code’s performance:
- Analyze the time and space complexity of your solutions
- Look for opportunities to reduce redundant operations
- Use appropriate data structures to minimize time complexity
- Consider edge cases and handle them efficiently
- Learn and apply optimization techniques specific to your programming language
Practice refactoring your solutions to achieve better runtime and memory usage. Many coding platforms provide performance metrics, allowing you to compare your solution against others and identify areas for improvement.
5. Master Your Preferred Programming Language
While problem-solving skills are language-agnostic, having a deep understanding of your chosen programming language can give you a significant advantage in coding challenges. To master your language:
- Study the language’s syntax, features, and best practices in depth
- Familiarize yourself with the standard library and built-in functions
- Learn language-specific optimizations and tricks
- Practice implementing common algorithms and data structures in your language
- Stay updated with the latest language updates and features
For example, if you’re using Python, you might want to master list comprehensions, generators, and the collections module to write more concise and efficient code.
6. Simulate Real Interview Conditions
To prepare for coding interviews effectively, it’s essential to simulate real interview conditions during your practice sessions. Here’s how:
- Set a timer for each problem to mimic time constraints
- Practice explaining your thought process out loud as you solve problems
- Use a whiteboard or pen and paper instead of an IDE for some practice sessions
- Conduct mock interviews with friends or use platforms that offer this service
- Practice handling follow-up questions and optimizing your initial solutions
AlgoCademy and similar platforms often provide features that simulate interview conditions, helping you become more comfortable with the pressure and constraints of real coding interviews.
7. Learn from Others and Collaborate
Improving your coding skills doesn’t have to be a solitary journey. Engaging with the programming community can accelerate your learning:
- Join coding forums and discussion groups
- Participate in coding competitions or hackathons
- Contribute to open-source projects
- Review and learn from others’ solutions to coding challenges
- Explain concepts to others to reinforce your own understanding
Platforms like AlgoCademy often have community features or forums where you can interact with other learners, share insights, and learn from diverse perspectives.
8. Develop a Growth Mindset
Approaching coding challenges with a growth mindset can significantly impact your performance and learning trajectory. Embrace the following principles:
- View challenges as opportunities for growth rather than tests of your abilities
- Embrace failure as a learning experience
- Focus on the process of problem-solving, not just the outcome
- Celebrate small improvements and milestones
- Stay persistent and maintain a positive attitude, even when faced with difficult problems
Remember that even experienced programmers encounter challenges they can’t immediately solve. The key is to learn from each experience and continuously improve.
9. Utilize AI-Powered Learning Tools
Artificial Intelligence has revolutionized the way we learn and practice coding. AI-powered tools can provide personalized guidance, instant feedback, and adaptive learning experiences. Here’s how you can leverage AI to improve your coding challenge performance:
- Use AI-powered code completion tools to learn efficient coding patterns
- Engage with AI tutors for personalized explanations of complex concepts
- Utilize AI-generated practice problems tailored to your skill level
- Analyze AI-provided insights on your coding habits and areas for improvement
- Explore AI-powered debugging assistants to identify and fix errors more efficiently
Platforms like AlgoCademy often incorporate AI technology to enhance the learning experience, providing real-time assistance and personalized learning paths.
10. Review and Reflect on Your Progress
Regular self-assessment and reflection are crucial for continuous improvement. To track and analyze your progress:
- Keep a log of the problems you’ve solved and your approach to each
- Regularly review your past solutions and look for ways to improve them
- Identify patterns in the types of problems you find challenging
- Set specific, measurable goals for improvement (e.g., solving X medium difficulty problems per week)
- Periodically reassess your strengths and weaknesses
Many coding platforms and learning tools offer progress tracking features. Utilize these to gain insights into your performance trends and areas needing focus.
Implementing Effective Coding Practices
As you work on improving your coding challenge performance, it’s essential to develop and maintain good coding practices. These habits will not only help you in challenges but also make you a better programmer overall:
Write Clean and Readable Code
Even under time pressure, strive to write clean, well-organized code. This includes:
- Using meaningful variable and function names
- Maintaining consistent indentation and formatting
- Breaking down complex functions into smaller, manageable pieces
- Adding comments to explain non-obvious logic
Here’s an example of clean code in Python:
def is_palindrome(s: str) -> bool:
"""
Check if a string is a palindrome.
Args:
s (str): The input string to check.
Returns:
bool: True if the string is a palindrome, False otherwise.
"""
# Remove non-alphanumeric characters and convert to lowercase
cleaned_s = ''.join(char.lower() for char in s if char.isalnum())
# Compare the string with its reverse
return cleaned_s == cleaned_s[::-1]
# Example usage
print(is_palindrome("A man, a plan, a canal: Panama")) # Output: True
Test Your Code Thoroughly
Develop a habit of testing your code with various inputs, including edge cases:
- Test with normal cases, edge cases, and corner cases
- Consider empty inputs, very large inputs, and invalid inputs
- Use assertion statements to verify your code’s behavior
Here’s an example of how you might test the palindrome function:
def test_is_palindrome():
assert is_palindrome("racecar") == True
assert is_palindrome("hello") == False
assert is_palindrome("") == True
assert is_palindrome("A man, a plan, a canal: Panama") == True
assert is_palindrome("race a car") == False
assert is_palindrome("0P") == False
print("All tests passed!")
test_is_palindrome()
Optimize Incrementally
When tackling coding challenges, it’s often beneficial to start with a working solution and then optimize it:
- Implement a brute-force solution first
- Ensure the solution is correct by testing it
- Analyze the time and space complexity
- Identify bottlenecks and areas for improvement
- Refactor and optimize step by step
- Re-test after each optimization to ensure correctness
This approach allows you to have a working solution quickly and then improve it systematically.
Leveraging Advanced Data Structures and Algorithms
As you progress in your coding challenge journey, you’ll encounter problems that require more advanced data structures and algorithms. Familiarizing yourself with these can significantly boost your problem-solving capabilities:
Advanced Data Structures
- Trie (Prefix Tree): Efficient for string-related problems
- Segment Tree: Useful for range query problems
- Disjoint Set Union (Union-Find): Ideal for graph connectivity problems
- Fenwick Tree (Binary Indexed Tree): Efficient for range sum queries
- Red-Black Tree: Self-balancing binary search tree
Advanced Algorithms
- Dynamic Programming: For optimization problems with overlapping subproblems
- Dijkstra’s Algorithm: For finding shortest paths in weighted graphs
- A* Search: For pathfinding and graph traversal
- Kruskal’s and Prim’s Algorithms: For minimum spanning tree problems
- Topological Sorting: For scheduling problems and dependency resolution
Understanding these advanced concepts will enable you to tackle a wider range of problems efficiently. Platforms like AlgoCademy often provide in-depth tutorials and practice problems for these advanced topics, helping you master them step by step.
Preparing for Specific Types of Coding Challenges
Different companies and platforms may focus on various types of coding challenges. It’s beneficial to familiarize yourself with common categories:
1. Array and String Manipulation
These problems often involve techniques like two-pointer approach, sliding window, or prefix sums. Example problem: “Find the longest substring without repeating characters.”
2. Linked List and Tree Traversal
Understanding various traversal methods (in-order, pre-order, post-order for trees; iterative vs. recursive approaches) is crucial. Example problem: “Reverse a linked list” or “Find the lowest common ancestor in a binary tree.”
3. Dynamic Programming
Recognizing when to use DP and how to formulate the recurrence relation is key. Example problem: “Find the longest increasing subsequence in an array.”
4. Graph Algorithms
Familiarity with DFS, BFS, and more advanced algorithms like Dijkstra’s is important. Example problem: “Find the number of islands in a 2D grid.”
5. Bit Manipulation
Understanding bitwise operations can lead to highly efficient solutions for certain problems. Example problem: “Find the single number in an array where every element appears twice except for one.”
Conclusion: Continuous Learning and Improvement
Improving your coding challenge performance is an ongoing process that requires dedication, practice, and a willingness to learn. By following the strategies outlined in this guide and consistently challenging yourself, you can significantly enhance your problem-solving skills and coding abilities.
Remember that the journey of improvement is as important as the destination. Each problem you solve, each concept you master, and each challenge you overcome contributes to your growth as a programmer. Platforms like AlgoCademy are designed to support this journey, offering structured learning paths, interactive coding environments, and AI-powered assistance to help you reach your full potential.
As you continue to improve, don’t forget to apply your skills to real-world projects and contribute to the programming community. Share your knowledge, collaborate with others, and stay curious about new technologies and approaches. With persistence and the right resources, you’ll be well-equipped to tackle any coding challenge that comes your way, whether in interviews, competitions, or your professional career.
Happy coding, and may your algorithms always run in O(1) time!