15 Essential Habits for New Programmers to Cultivate Success
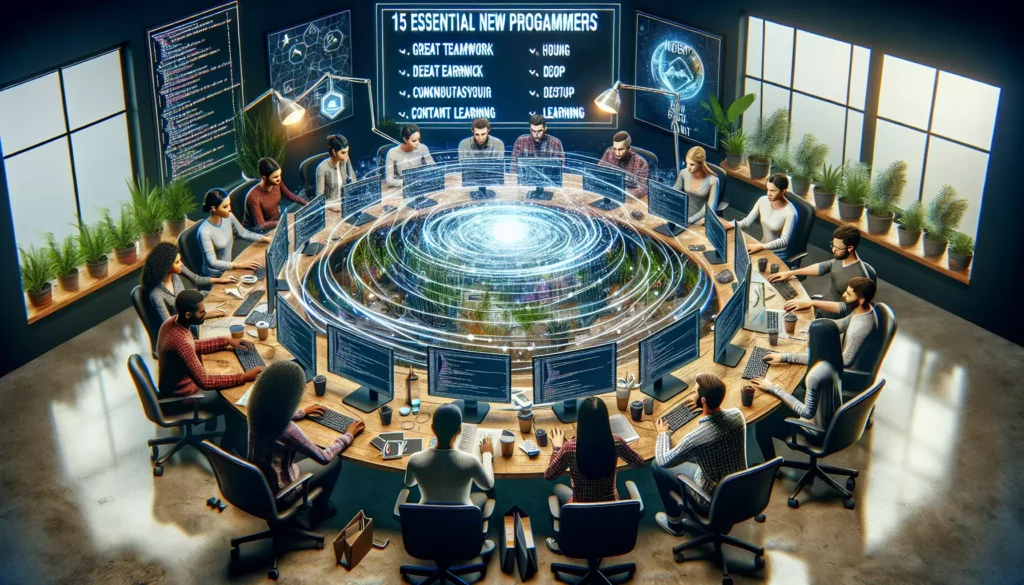
Embarking on a journey into the world of programming can be both exciting and daunting. As a new programmer, developing the right habits early on can significantly impact your growth, efficiency, and overall success in the field. In this comprehensive guide, we’ll explore 15 essential habits that every aspiring coder should cultivate to build a strong foundation for their programming career.
1. Practice Consistent Coding
One of the most crucial habits for new programmers is to code consistently. Just like any skill, programming improves with regular practice. Set aside dedicated time each day, even if it’s just for 30 minutes, to write code. This consistent practice helps reinforce concepts, improves muscle memory for syntax, and keeps your skills sharp.
Consider following the “Code Every Day” challenge, where you commit to writing code for at least an hour every day for 100 days. This habit can dramatically accelerate your learning and help you build a robust coding routine.
2. Read and Understand Code Written by Others
Reading code is just as important as writing it. Make it a habit to regularly explore open-source projects, study code snippets, and analyze solutions to coding challenges. This practice exposes you to different coding styles, problem-solving approaches, and best practices used by experienced developers.
Platforms like GitHub are treasure troves of code to study. Start by exploring popular repositories in languages you’re learning, and try to understand how the code works. This habit will not only improve your comprehension skills but also introduce you to new techniques and coding patterns.
3. Document Your Code
Developing a habit of properly documenting your code from the beginning is invaluable. Clear and concise documentation helps you understand your own code when you revisit it later and makes it easier for others to comprehend and collaborate on your projects.
Practice writing meaningful comments, creating README files for your projects, and using clear variable and function names. Here’s a simple example of well-documented code:
# Function to calculate the factorial of a number
def factorial(n):
"""
Calculate the factorial of a given number.
Args:
n (int): The number to calculate factorial for
Returns:
int: The factorial of the input number
"""
if n == 0 or n == 1:
return 1
else:
return n * factorial(n-1)
# Example usage
result = factorial(5)
print(f"The factorial of 5 is: {result}")
4. Learn to Debug Effectively
Debugging is an essential skill for any programmer. Develop the habit of systematically approaching and solving errors in your code. Instead of getting frustrated, see debugging as an opportunity to learn and improve your understanding of the code and the programming language.
Start by learning to use debugging tools provided by your IDE or code editor. Practice using breakpoints, stepping through code, and inspecting variables. Additionally, get comfortable with reading and interpreting error messages – they often contain valuable information about what went wrong and where.
5. Embrace Version Control
Version control systems like Git are integral to modern software development. Make it a habit to use version control for all your projects, even personal ones. This practice not only helps you track changes and collaborate with others but also serves as a safety net, allowing you to experiment without fear of breaking your code irreversibly.
Start by learning basic Git commands and workflows. Commit your changes regularly, use meaningful commit messages, and practice branching and merging. These skills will be invaluable as you progress in your programming career.
6. Continuously Learn and Stay Updated
The field of programming is constantly evolving, with new languages, frameworks, and tools emerging regularly. Cultivate a habit of continuous learning to stay relevant and expand your skill set. Set aside time each week to explore new technologies, read tech blogs, or work through tutorials on platforms like AlgoCademy.
Subscribe to programming newsletters, follow tech influencers on social media, and join online communities like Stack Overflow or Reddit’s programming subreddits. This habit of staying informed will help you adapt to the changing landscape of technology and discover new opportunities for growth.
7. Solve Coding Challenges Regularly
Engaging in coding challenges and puzzles is an excellent way to sharpen your problem-solving skills and learn new concepts. Make it a habit to solve at least one coding challenge every day. Platforms like LeetCode, HackerRank, and CodeWars offer a wide range of problems suitable for all skill levels.
This practice not only improves your coding skills but also prepares you for technical interviews, which often involve solving algorithmic problems. Start with easier challenges and gradually increase the difficulty as you improve.
8. Write Clean and Readable Code
Developing a habit of writing clean, well-organized code from the start will save you (and your future collaborators) a lot of time and headaches. Follow established coding standards and best practices for the language you’re using. This includes proper indentation, meaningful variable names, and breaking down complex functions into smaller, more manageable pieces.
Here’s an example of clean, readable code:
def calculate_total_price(items, tax_rate):
subtotal = sum(item.price for item in items)
tax = subtotal * tax_rate
total = subtotal + tax
return total
def apply_discount(total, discount_percentage):
discount = total * (discount_percentage / 100)
discounted_total = total - discount
return discounted_total
# Usage
item_list = [Item("Book", 10), Item("Pen", 2), Item("Notebook", 5)]
tax_rate = 0.08
discount_percentage = 10
total_price = calculate_total_price(item_list, tax_rate)
final_price = apply_discount(total_price, discount_percentage)
print(f"Final price after discount: ${final_price:.2f}")
9. Collaborate with Other Programmers
Programming is often a collaborative effort, especially in professional settings. Develop the habit of working with other programmers early on. Participate in open-source projects, join coding meetups, or find a coding buddy to work on projects together.
Collaboration exposes you to different perspectives, coding styles, and problem-solving approaches. It also helps you develop essential soft skills like communication, teamwork, and giving/receiving code reviews – all crucial for a successful programming career.
10. Plan Before You Code
While it’s tempting to dive straight into coding, developing a habit of planning your approach before writing any code can save you time and lead to better solutions. Take a few minutes to outline your approach, consider potential challenges, and break down the problem into smaller, manageable tasks.
Use tools like flowcharts, pseudocode, or simple bullet points to map out your solution. This habit of planning helps you think through the problem more thoroughly and often leads to more efficient and well-structured code.
11. Practice Code Refactoring
Refactoring is the process of restructuring existing code without changing its external behavior. Make it a habit to regularly review and refactor your code. This practice helps you improve code quality, efficiency, and readability over time.
Start by identifying repetitive code that can be consolidated into functions, simplifying complex logic, and ensuring your code follows the DRY (Don’t Repeat Yourself) principle. Regular refactoring not only improves your code but also deepens your understanding of programming concepts and best practices.
12. Learn to Use the Command Line
While graphical interfaces are user-friendly, many development tools and workflows rely on command-line interfaces. Develop a habit of using the command line for tasks like file manipulation, git operations, and running scripts. This skill will make you more efficient and give you greater control over your development environment.
Start by learning basic commands for navigating the file system, creating and deleting files and directories, and running programs. Gradually incorporate more advanced command-line tools into your workflow.
13. Write Tests for Your Code
Testing is a crucial aspect of software development that ensures your code works as expected and helps catch bugs early. Develop the habit of writing tests for your code, even for small projects or practice exercises.
Start with simple unit tests and gradually learn about different types of testing, such as integration tests and end-to-end tests. This habit not only improves the quality and reliability of your code but also helps you think more critically about edge cases and potential issues in your programs.
Here’s a simple example of a unit test in Python using the unittest
module:
import unittest
def add_numbers(a, b):
return a + b
class TestAddNumbers(unittest.TestCase):
def test_add_positive_numbers(self):
self.assertEqual(add_numbers(2, 3), 5)
def test_add_negative_numbers(self):
self.assertEqual(add_numbers(-1, -1), -2)
def test_add_zero(self):
self.assertEqual(add_numbers(5, 0), 5)
if __name__ == '__main__':
unittest.main()
14. Prioritize Code Security
In an increasingly digital world, code security is paramount. Develop a habit of considering security implications in your code from the beginning. This includes practices like input validation, proper error handling, and secure storage of sensitive information.
Start by learning about common security vulnerabilities in web applications, such as SQL injection and cross-site scripting (XSS). Practice writing code that prevents these vulnerabilities. As you progress, explore more advanced security concepts and stay informed about best practices in secure coding.
15. Take Breaks and Practice Self-Care
While it’s important to be dedicated to learning and improving your programming skills, it’s equally crucial to develop habits that support your overall well-being. Make it a habit to take regular breaks during coding sessions, practice good posture, and give your eyes rest from screen time.
Additionally, engage in activities outside of programming to maintain a healthy work-life balance. This could include physical exercise, hobbies, or spending time with friends and family. A well-rested and balanced mind is more productive and creative when it comes to problem-solving and coding.
Conclusion
Developing these 15 habits as a new programmer will set you on a path to success in your coding journey. Remember, forming habits takes time and consistency. Start by incorporating a few of these practices into your routine and gradually build up to include more. With patience and persistence, these habits will become second nature, enhancing your skills, productivity, and enjoyment of programming.
As you continue to grow as a programmer, platforms like AlgoCademy can be invaluable resources. With its focus on interactive coding tutorials, algorithmic thinking, and preparation for technical interviews, AlgoCademy aligns perfectly with many of the habits we’ve discussed. It provides a structured environment to practice consistent coding, solve challenges, and continually learn new concepts.
Remember, becoming a proficient programmer is a journey, not a destination. Embrace the learning process, stay curious, and most importantly, enjoy the creative and problem-solving aspects of coding. With these habits as your foundation, you’re well on your way to becoming a skilled and successful programmer.