How to Prepare for Coding Interviews at Startups: A Comprehensive Guide
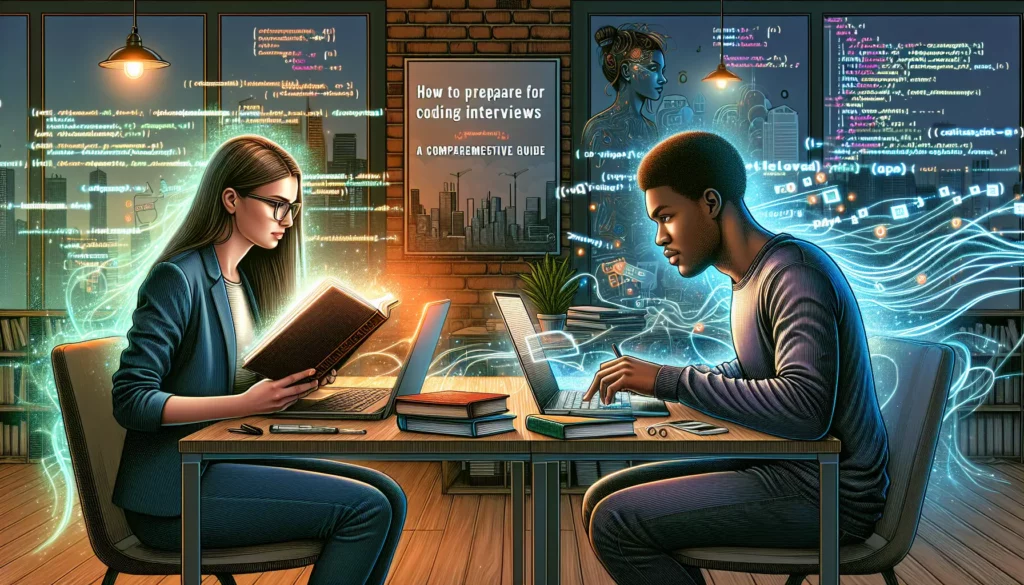
Preparing for coding interviews at startups can be an exciting yet challenging process. Unlike larger tech companies, startups often have unique expectations and interview processes. This comprehensive guide will walk you through the essential steps to ace your coding interviews at startups, helping you stand out from the competition and land your dream job.
1. Understand the Startup Environment
Before diving into technical preparation, it’s crucial to understand the startup ecosystem and how it differs from larger corporations:
- Fast-paced environment: Startups often move quickly and require adaptable employees.
- Wear multiple hats: You may be expected to take on various roles and responsibilities.
- Limited resources: Startups typically have smaller teams and tighter budgets.
- Innovation-focused: Many startups prioritize creativity and novel solutions.
- Cultural fit: Team dynamics and personality fit are often as important as technical skills.
2. Master the Fundamentals
While startups may have unique interview processes, a solid foundation in computer science fundamentals is still essential:
2.1. Data Structures
Ensure you have a strong grasp of common data structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
2.2. Algorithms
Familiarize yourself with key algorithmic concepts:
- Sorting and Searching
- Recursion
- Dynamic Programming
- Greedy Algorithms
- Graph Algorithms
- Divide and Conquer
2.3. Time and Space Complexity
Understanding Big O notation and being able to analyze the efficiency of your solutions is crucial. Practice evaluating the time and space complexity of different algorithms and data structures.
3. Focus on Practical Coding Skills
Startups often emphasize practical skills over theoretical knowledge. Here’s how to sharpen your coding abilities:
3.1. Choose a Programming Language
Select a language you’re comfortable with and that aligns with the startup’s tech stack. Popular choices include:
- Python
- JavaScript
- Java
- C++
- Ruby
3.2. Practice Coding Problems
Regularly solve coding problems on platforms like:
- LeetCode
- HackerRank
- CodeSignal
- Project Euler
Aim to solve at least one problem daily, focusing on a variety of difficulty levels and problem types.
3.3. Implement Data Structures from Scratch
Build your own implementations of common data structures to deepen your understanding. For example, try coding a binary search tree:
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinarySearchTree:
def __init__(self):
self.root = None
def insert(self, value):
if not self.root:
self.root = Node(value)
else:
self._insert_recursive(self.root, value)
def _insert_recursive(self, node, value):
if value < node.value:
if node.left is None:
node.left = Node(value)
else:
self._insert_recursive(node.left, value)
else:
if node.right is None:
node.right = Node(value)
else:
self._insert_recursive(node.right, value)
3.4. Work on Personal Projects
Develop small projects that showcase your skills and creativity. This demonstrates initiative and practical application of your knowledge.
4. Understand Startup-Specific Technologies
Research the technologies commonly used in startups and familiarize yourself with them:
4.1. Web Development
- Frontend: React, Vue.js, Angular
- Backend: Node.js, Django, Ruby on Rails
4.2. Mobile Development
- iOS: Swift
- Android: Kotlin
- Cross-platform: React Native, Flutter
4.3. DevOps and Cloud Services
- Docker
- Kubernetes
- AWS, Google Cloud, or Azure
4.4. Databases
- SQL: PostgreSQL, MySQL
- NoSQL: MongoDB, Cassandra
5. Enhance Your Problem-Solving Skills
Startups value candidates who can think creatively and solve complex problems efficiently. Here’s how to improve your problem-solving abilities:
5.1. Practice the STAR Method
When discussing your experiences or solving problems, use the STAR method:
- Situation: Describe the context.
- Task: Explain the challenge or goal.
- Action: Detail the steps you took.
- Result: Share the outcome and lessons learned.
5.2. Develop a Problem-Solving Framework
Create a systematic approach to tackling coding problems:
- Understand the problem thoroughly
- Identify the inputs and expected outputs
- Break down the problem into smaller steps
- Consider multiple approaches
- Choose the most efficient solution
- Implement the solution
- Test and refine your code
5.3. Practice Thinking Aloud
Many startups use pair programming or collaborative coding interviews. Practice explaining your thought process while coding to improve your communication skills.
6. Prepare for Startup-Specific Interview Formats
Startups often employ unique interview techniques. Familiarize yourself with these common formats:
6.1. Take-Home Assignments
Many startups give candidates a project to complete at home. Tips for success:
- Read instructions carefully
- Manage your time effectively
- Write clean, well-documented code
- Include tests and error handling
- Prepare to explain your design decisions
6.2. Pair Programming Sessions
Practice coding with a partner to simulate pair programming interviews:
- Communicate clearly
- Be open to feedback
- Ask questions when needed
- Show your problem-solving process
6.3. System Design Interviews
While less common in startups, some may include system design questions. Prepare by:
- Studying scalable architectures
- Understanding microservices
- Practicing drawing system diagrams
- Considering trade-offs in design decisions
7. Develop Soft Skills
In a startup environment, soft skills are crucial. Focus on developing:
7.1. Communication
- Practice explaining technical concepts to non-technical people
- Work on active listening skills
- Learn to give and receive constructive feedback
7.2. Adaptability
- Be open to learning new technologies
- Show flexibility in your approach to problem-solving
- Demonstrate willingness to take on diverse responsibilities
7.3. Teamwork
- Participate in open-source projects
- Engage in coding communities or hackathons
- Practice giving credit to others and sharing success
8. Research the Startup
Before your interview, thoroughly research the startup:
- Understand their product or service
- Read about their company culture
- Research their funding and growth stage
- Look into their tech stack and development practices
- Prepare thoughtful questions about the company and role
9. Mock Interviews and Code Reviews
Practice makes perfect. Engage in mock interviews and code reviews:
9.1. Mock Interviews
- Use platforms like Pramp or InterviewBit for peer mock interviews
- Ask friends or mentors to conduct mock interviews
- Record yourself solving problems to review later
9.2. Code Reviews
- Participate in open-source projects to get your code reviewed
- Review others’ code to improve your own skills
- Use platforms like CodeReview Stack Exchange
10. Prepare for Behavioral Questions
Startups often place a high value on cultural fit. Be ready to answer questions about:
- Your motivation for joining a startup
- How you handle ambiguity and fast-paced environments
- Your approach to learning new technologies
- Times you’ve shown initiative or leadership
- How you handle conflicts or disagreements
11. Stay Updated with Industry Trends
Demonstrate your passion and industry awareness by staying informed about:
- Latest programming languages and frameworks
- Emerging technologies (e.g., AI, blockchain)
- Best practices in software development
- Startup ecosystem news and trends
12. Build Your Online Presence
Many startups will look at your online presence. Consider:
- Maintaining an active GitHub profile with sample projects
- Creating a personal website or tech blog
- Participating in coding forums or Stack Overflow
- Networking on LinkedIn and Twitter
13. Practice Coding Under Time Pressure
Improve your ability to code efficiently under time constraints:
- Participate in timed coding contests
- Set time limits for practice problems
- Learn to prioritize requirements and deliver MVP solutions
14. Prepare for Technical Discussions
Be ready to engage in in-depth technical discussions about:
- Your previous projects and the technologies used
- Trade-offs between different technologies or approaches
- Your understanding of software design principles (SOLID, DRY, etc.)
- Your experience with Agile methodologies
15. Follow Up and Learn from Each Interview
After each interview:
- Send a thank-you note to your interviewers
- Reflect on what went well and areas for improvement
- Ask for feedback if you don’t receive an offer
- Continue practicing and refining your skills
Conclusion
Preparing for coding interviews at startups requires a multifaceted approach. By mastering the fundamentals, honing your practical skills, understanding the startup environment, and developing your soft skills, you’ll be well-equipped to tackle any interview challenge. Remember, the key is consistent practice and a genuine passion for problem-solving and innovation.
As you embark on your journey to land a role at an exciting startup, keep in mind that the process itself is a valuable learning experience. Each interview, whether successful or not, provides an opportunity to grow and refine your skills. Stay persistent, remain curious, and approach each opportunity with enthusiasm. With dedication and the right preparation, you’ll be well on your way to securing your dream job in the dynamic world of startups.