Key Concepts in Functional Programming: A Comprehensive Guide
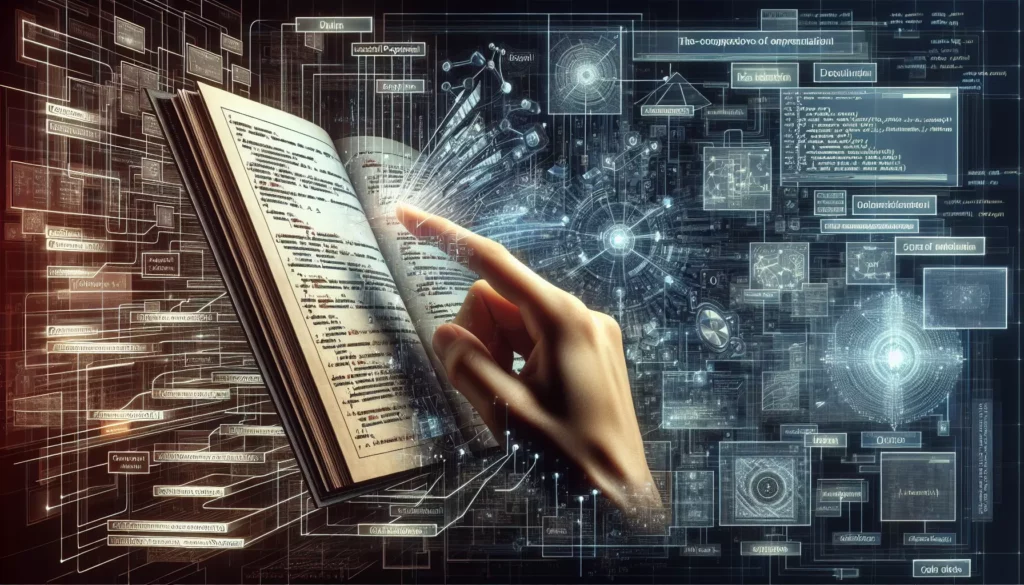
Functional programming is a paradigm that has gained significant traction in recent years, especially in the world of software development. As coding education platforms like AlgoCademy continue to evolve, understanding functional programming concepts becomes increasingly important for aspiring developers and those preparing for technical interviews at major tech companies. In this comprehensive guide, we’ll explore the key concepts of functional programming, their applications, and how they can enhance your problem-solving skills.
Table of Contents
- Introduction to Functional Programming
- Pure Functions
- Immutability
- First-Class and Higher-Order Functions
- Recursion
- Lazy Evaluation
- Referential Transparency
- Function Composition
- Currying
- Monads
- Benefits of Functional Programming
- Applications in Modern Development
- Preparing for Technical Interviews
- Conclusion
1. Introduction to Functional Programming
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. It emphasizes the application of functions to inputs to produce outputs without modifying state. This approach contrasts with imperative programming, which emphasizes changes in state.
The roots of functional programming lie in lambda calculus, a formal system developed in the 1930s to investigate function definition, function application, and recursion. Today, functional programming concepts are incorporated into many programming languages, including JavaScript, Python, Scala, Haskell, and Clojure.
2. Pure Functions
Pure functions are at the core of functional programming. A pure function is a function that:
- Always produces the same output for the same input
- Has no side effects (doesn’t modify external state)
Here’s an example of a pure function in JavaScript:
function add(a, b) {
return a + b;
}
This function always returns the same result for the same inputs and doesn’t modify any external state. Pure functions are easier to test, debug, and reason about, making them a powerful tool in a developer’s arsenal.
3. Immutability
Immutability is the practice of not changing data once it has been created. In functional programming, we create new data structures instead of modifying existing ones. This approach helps prevent unintended side effects and makes it easier to track changes in your program.
Here’s an example of working with immutable data in JavaScript:
const originalArray = [1, 2, 3];
const newArray = [...originalArray, 4]; // Creates a new array
console.log(originalArray); // [1, 2, 3]
console.log(newArray); // [1, 2, 3, 4]
In this example, instead of modifying the original array, we create a new array with the additional element.
4. First-Class and Higher-Order Functions
In functional programming, functions are treated as first-class citizens. This means that functions can be:
- Assigned to variables
- Passed as arguments to other functions
- Returned from functions
Higher-order functions are functions that can take other functions as arguments or return functions. They are a powerful concept in functional programming that allows for greater abstraction and code reuse.
Here’s an example of a higher-order function in JavaScript:
function multiplyBy(factor) {
return function(number) {
return number * factor;
}
}
const double = multiplyBy(2);
console.log(double(5)); // 10
In this example, multiplyBy
is a higher-order function that returns another function. This returned function is then assigned to the variable double
.
5. Recursion
Recursion is a technique where a function calls itself to solve a problem. It’s often used in functional programming as an alternative to imperative loops. Recursive functions typically have a base case (to stop the recursion) and a recursive case.
Here’s an example of a recursive function to calculate factorial:
function factorial(n) {
if (n === 0 || n === 1) {
return 1; // Base case
}
return n * factorial(n - 1); // Recursive case
}
console.log(factorial(5)); // 120
Recursion can lead to elegant solutions for complex problems, especially when dealing with tree-like data structures or divide-and-conquer algorithms.
6. Lazy Evaluation
Lazy evaluation is a strategy where the evaluation of an expression is delayed until its value is needed. This can lead to performance improvements and the ability to work with infinite data structures.
While JavaScript doesn’t natively support lazy evaluation, we can simulate it using generator functions:
function* infiniteSequence() {
let i = 0;
while (true) {
yield i++;
}
}
const generator = infiniteSequence();
console.log(generator.next().value); // 0
console.log(generator.next().value); // 1
console.log(generator.next().value); // 2
In this example, the infiniteSequence
generator function can potentially generate an infinite sequence of numbers, but values are only calculated when requested.
7. Referential Transparency
Referential transparency is a property of functions where an expression can be replaced by its value without changing the program’s behavior. This is closely related to the concept of pure functions.
For example, consider this function:
function greet(name) {
return `Hello, ${name}!`;
}
This function is referentially transparent because we can replace any call to greet("Alice")
with its result "Hello, Alice!"
without changing the program’s behavior.
8. Function Composition
Function composition is the process of combining two or more functions to produce a new function. It’s a powerful technique for building complex operations out of simpler ones.
Here’s an example of function composition in JavaScript:
const double = x => x * 2;
const increment = x => x + 1;
const doubleAndIncrement = x => increment(double(x));
console.log(doubleAndIncrement(3)); // 7
In this example, we compose the double
and increment
functions to create a new function doubleAndIncrement
.
9. Currying
Currying is the technique of translating a function with multiple arguments into a sequence of functions, each with a single argument. This can make functions more flexible and easier to compose.
Here’s an example of currying in JavaScript:
function curry(f) {
return function(a) {
return function(b) {
return f(a, b);
};
};
}
function sum(a, b) {
return a + b;
}
let curriedSum = curry(sum);
console.log(curriedSum(1)(2)); // 3
In this example, we’ve curried the sum
function, allowing us to partially apply it with one argument at a time.
10. Monads
Monads are a more advanced concept in functional programming. They are structures that represent computations rather than data. Monads are used to handle side effects, manage state, and deal with complex operations in a pure functional way.
While monads are a complex topic, a simple example of a monad-like structure in JavaScript is the Promise:
const fetchUser = id =>
new Promise((resolve, reject) => {
// Simulating an API call
setTimeout(() => {
if (id === 1) {
resolve({ id: 1, name: 'John Doe' });
} else {
reject('User not found');
}
}, 1000);
});
fetchUser(1)
.then(user => console.log(user))
.catch(error => console.error(error));
In this example, the Promise acts like a monad, encapsulating a value that may not be available immediately and providing methods to chain operations.
11. Benefits of Functional Programming
Functional programming offers several benefits:
- Predictability: Pure functions and immutability make code behavior more predictable.
- Testability: Pure functions are easier to test as they don’t depend on or modify external state.
- Concurrency: The absence of side effects makes it easier to write concurrent programs.
- Modularity: Function composition and higher-order functions promote code reuse and modularity.
- Debugging: Immutability and pure functions make it easier to trace program execution and find bugs.
12. Applications in Modern Development
Functional programming concepts are widely used in modern development:
- React: React’s concept of pure components is inspired by functional programming principles.
- Redux: The Redux state management library is built on functional programming concepts like immutability and pure functions.
- Serverless Computing: The stateless nature of serverless functions aligns well with functional programming principles.
- Data Processing: Functional programming is well-suited for data processing tasks, especially when working with large datasets.
- Machine Learning: Some machine learning algorithms and data preprocessing tasks can be elegantly expressed using functional programming concepts.
13. Preparing for Technical Interviews
Understanding functional programming concepts can be beneficial for technical interviews, especially at major tech companies. Here are some tips:
- Practice implementing common algorithms using functional programming techniques.
- Get comfortable with higher-order functions like
map
,filter
, andreduce
. - Learn to solve problems using recursion instead of loops.
- Understand the benefits and trade-offs of functional programming compared to other paradigms.
- Be prepared to discuss how functional programming concepts can improve code quality and maintainability.
Platforms like AlgoCademy can be invaluable in preparing for these interviews, offering interactive coding tutorials and problem-solving exercises that incorporate functional programming concepts.
14. Conclusion
Functional programming is a powerful paradigm that offers a different way of thinking about and structuring code. By emphasizing pure functions, immutability, and declarative programming, it provides tools for writing more predictable, testable, and maintainable code.
While it may require a shift in mindset for developers accustomed to imperative programming, the concepts of functional programming are increasingly relevant in modern software development. Whether you’re building web applications, processing data, or preparing for technical interviews at top tech companies, a solid understanding of functional programming principles can be a valuable asset.
As you continue your journey in programming, consider exploring functional programming languages like Haskell or Clojure, or applying functional concepts in languages you already know. Platforms like AlgoCademy can provide structured learning paths and practical exercises to help you master these concepts and apply them to real-world problems.
Remember, the goal is not to use functional programming exclusively, but to add it to your toolkit of programming paradigms. By understanding when and how to apply functional concepts, you can write cleaner, more efficient code and tackle a wider range of programming challenges.