How to Practice Coding Interviews in Different Programming Languages
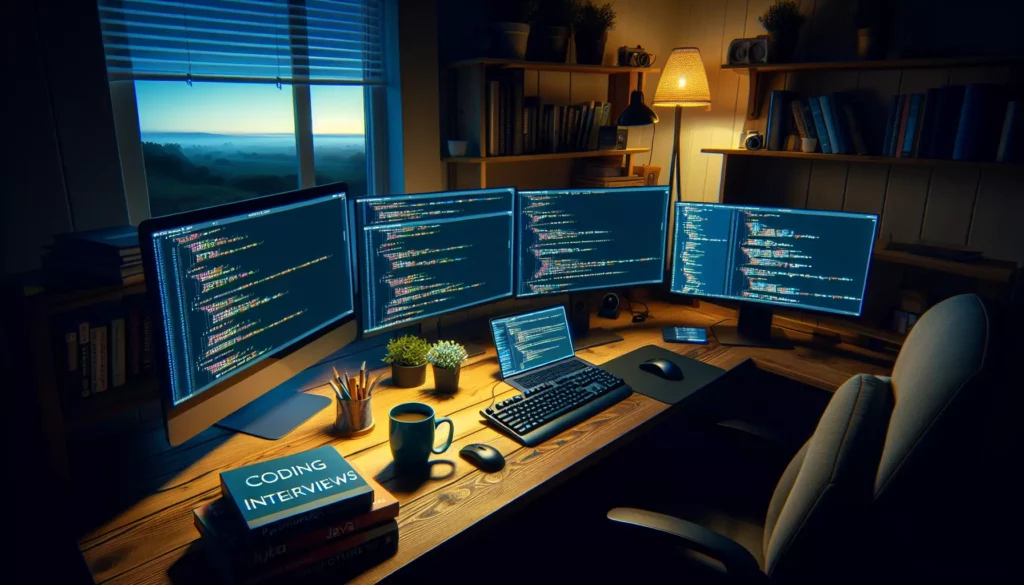
Preparing for coding interviews can be a daunting task, especially when you’re expected to showcase your skills in multiple programming languages. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech firm, mastering the art of coding interviews is crucial. In this comprehensive guide, we’ll explore effective strategies to practice coding interviews across various programming languages, helping you build confidence and improve your problem-solving skills.
1. Understanding the Importance of Multi-Language Proficiency
Before diving into specific practice techniques, it’s essential to understand why being proficient in multiple programming languages is valuable for coding interviews:
- Versatility: Different companies may prefer different languages, and being adaptable increases your chances of success.
- Deeper understanding: Learning multiple languages helps you grasp fundamental programming concepts more thoroughly.
- Problem-solving skills: Each language has its strengths, and knowing when to apply which language demonstrates advanced problem-solving abilities.
- Career opportunities: Multi-language proficiency opens doors to a wider range of job opportunities.
2. Choosing the Right Languages to Focus On
While it’s beneficial to have a broad knowledge base, it’s also important to prioritize your learning. Here are some popular languages often used in coding interviews:
- Python: Known for its simplicity and readability, Python is a favorite in many coding interviews.
- Java: A versatile language used in many enterprise-level applications.
- C++: Preferred for its performance and low-level control, especially in system programming roles.
- JavaScript: Essential for web development positions and increasingly popular in other domains.
- Go: Growing in popularity, especially for backend and distributed systems roles.
Choose 2-3 languages to focus on, based on your target companies and roles.
3. Setting Up Your Practice Environment
To effectively practice coding interviews in different languages, you need a suitable environment:
- IDE or Text Editor: Choose a versatile IDE like Visual Studio Code, which supports multiple languages, or use language-specific IDEs like PyCharm for Python or IntelliJ for Java.
- Online Platforms: Utilize platforms like LeetCode, HackerRank, or CodeSignal, which allow you to solve problems in various languages.
- Version Control: Set up Git repositories for each language to track your progress and solutions.
- Language-specific tools: Install necessary compilers, interpreters, and package managers for each language you’re practicing.
4. Developing a Structured Practice Routine
Consistency is key when preparing for coding interviews. Here’s a suggested routine to follow:
- Daily Problem Solving: Aim to solve at least one problem per day in each of your chosen languages.
- Weekly Language Rotation: Focus on a different language each week to maintain and improve your skills across all chosen languages.
- Time-Boxed Practice: Simulate interview conditions by giving yourself a time limit (usually 30-45 minutes) to solve each problem.
- Review and Refactor: After solving a problem, review your solution and try to optimize it in terms of time and space complexity.
5. Mastering Core Data Structures and Algorithms
Regardless of the programming language, certain fundamental concepts are crucial for coding interviews:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Sorting and Searching Algorithms
- Dynamic Programming
- Recursion
Practice implementing these data structures and algorithms in each of your chosen languages. Pay attention to the nuances and built-in functionalities each language offers.
6. Language-Specific Practice Techniques
6.1 Python
When practicing coding interviews in Python:
- Master list comprehensions and generator expressions for concise code.
- Utilize built-in functions like
map()
,filter()
, andreduce()
. - Familiarize yourself with the
collections
module for advanced data structures.
Example of a list comprehension in Python:
squares = [x**2 for x in range(10)]
print(squares) # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
6.2 Java
For Java practice:
- Focus on object-oriented programming principles.
- Practice using Java Collections Framework (List, Set, Map interfaces).
- Implement common design patterns.
Example of using Java’s ArrayList:
import java.util.ArrayList;
import java.util.List;
public class JavaExample {
public static void main(String[] args) {
List<Integer> numbers = new ArrayList<>();
numbers.add(1);
numbers.add(2);
numbers.add(3);
System.out.println(numbers); // Output: [1, 2, 3]
}
}
6.3 C++
When practicing C++:
- Focus on memory management and pointers.
- Utilize the Standard Template Library (STL) for efficient data structures and algorithms.
- Practice implementing custom data structures.
Example of using C++ vectors:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
// Output: 1 2 3 4 5
return 0;
}
6.4 JavaScript
For JavaScript practice:
- Master asynchronous programming with Promises and async/await.
- Practice functional programming concepts.
- Familiarize yourself with ES6+ features.
Example of using async/await in JavaScript:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('Error:', error);
}
}
fetchData();
7. Utilizing Online Resources and Platforms
Take advantage of various online resources to enhance your multi-language coding interview practice:
- LeetCode: Offers a vast collection of coding problems with solutions in multiple languages.
- HackerRank: Provides language-specific tracks and coding challenges.
- CodeSignal: Offers interview practice problems and assessments in various languages.
- GeeksforGeeks: Provides tutorials and practice problems for multiple programming languages.
- GitHub: Explore open-source projects in different languages to learn best practices and coding styles.
8. Mock Interviews and Pair Programming
To simulate real interview conditions:
- Participate in mock interviews with peers or mentors in different languages.
- Join coding communities or study groups focused on interview preparation.
- Practice pair programming to improve your ability to communicate your thought process.
9. Analyzing and Learning from Solutions
After solving problems:
- Compare your solutions with others in the same language.
- Analyze solutions in different languages to understand language-specific optimizations.
- Implement the same solution in multiple languages to reinforce your skills.
10. Focusing on Time and Space Complexity
Regardless of the language:
- Practice analyzing the time and space complexity of your solutions.
- Learn to optimize your code for better performance.
- Understand the trade-offs between time and space in different languages.
11. Staying Updated with Language Features
Programming languages evolve, so:
- Keep track of new features and updates in your chosen languages.
- Experiment with new language features in your practice problems.
- Read language-specific blogs and documentation regularly.
12. Building Projects in Different Languages
To apply your skills in real-world scenarios:
- Develop small projects in each of your chosen languages.
- Try implementing the same project in multiple languages to compare approaches.
- Contribute to open-source projects in different languages.
13. Leveraging Language-Specific Communities
Engage with language-specific communities:
- Join forums like Stack Overflow or Reddit communities for each language.
- Participate in language-specific meetups or conferences.
- Follow influential developers and thought leaders in each language on social media.
14. Practicing System Design in Multiple Languages
For senior roles, system design is crucial:
- Practice designing scalable systems using different languages and frameworks.
- Understand how language choice affects system architecture.
- Implement key components of a system design in multiple languages.
15. Developing Language-Agnostic Problem-Solving Skills
While language proficiency is important, focus on developing language-agnostic skills:
- Practice breaking down complex problems into smaller, manageable parts.
- Develop a systematic approach to problem-solving that can be applied across languages.
- Improve your ability to communicate your thought process clearly, regardless of the language used.
Conclusion
Practicing coding interviews in different programming languages requires dedication, consistency, and a structured approach. By following the strategies outlined in this guide, you can develop the versatility and problem-solving skills needed to excel in technical interviews across various languages and platforms.
Remember, the goal is not just to memorize solutions in different languages, but to develop a deep understanding of programming concepts that can be applied regardless of the language. With persistent practice and a focus on fundamental principles, you’ll be well-prepared to tackle coding interviews in any language, opening doors to exciting opportunities in the tech industry.
Keep coding, stay curious, and embrace the challenge of mastering multiple languages. Your efforts will not only prepare you for interviews but also make you a more well-rounded and adaptable software engineer.