How to Prepare for Coding Interviews in a Short Time Frame: A Comprehensive Guide
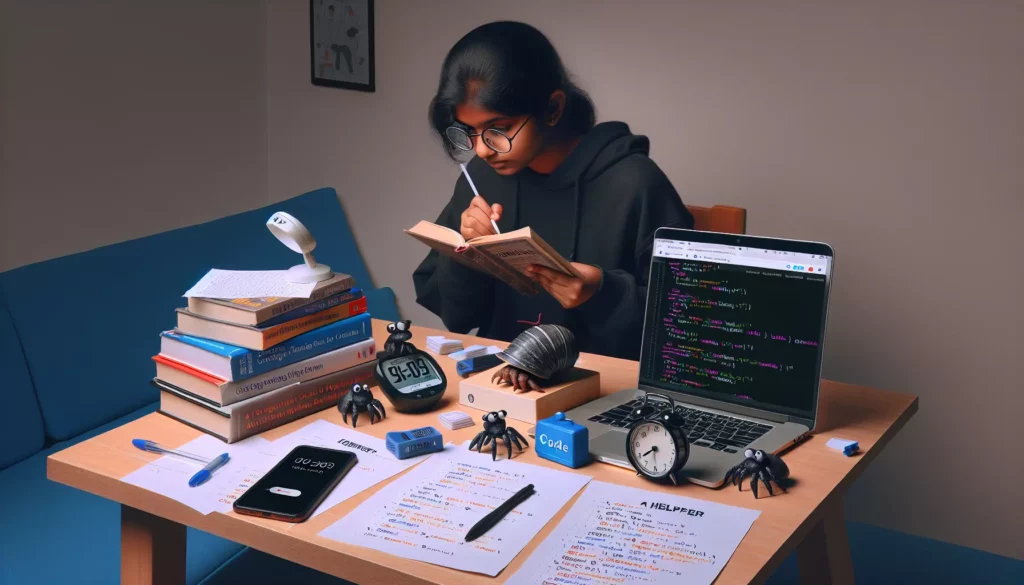
Preparing for coding interviews can be a daunting task, especially when you’re working with a limited timeframe. Whether you’re a recent graduate looking to land your first tech job or an experienced developer aiming to make a career switch, mastering the art of coding interviews is crucial. In this comprehensive guide, we’ll explore effective strategies and resources to help you prepare for coding interviews efficiently, even when time is not on your side.
Understanding the Importance of Coding Interviews
Before diving into preparation strategies, it’s essential to understand why coding interviews are so important in the tech industry. Companies, especially major tech giants often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), use coding interviews to assess a candidate’s problem-solving skills, algorithmic thinking, and ability to write clean, efficient code under pressure.
These interviews typically involve solving algorithmic problems, discussing system design, and sometimes even pair programming. The goal is not just to find someone who can code, but to identify individuals who can think critically, communicate effectively, and adapt to new challenges – all crucial skills in the fast-paced world of technology.
Setting Realistic Goals
When preparing for coding interviews in a short time frame, it’s crucial to set realistic goals. You can’t expect to master every algorithm and data structure in existence within a few weeks. Instead, focus on the following key areas:
- Fundamental data structures (arrays, linked lists, trees, graphs, hash tables)
- Basic algorithms (sorting, searching, traversal)
- Problem-solving techniques (recursion, dynamic programming, greedy algorithms)
- Time and space complexity analysis
- Coding best practices and clean code principles
By concentrating on these core areas, you’ll build a solid foundation that can be applied to a wide range of interview questions.
Creating a Study Plan
With limited time, a structured study plan is essential. Here’s a sample 4-week plan you can adapt based on your current skill level and available time:
Week 1: Fundamentals and Review
- Day 1-2: Review basic data structures (arrays, linked lists, stacks, queues)
- Day 3-4: Study trees and graphs
- Day 5-6: Focus on hash tables and their applications
- Day 7: Practice problems involving these data structures
Week 2: Algorithms and Problem-Solving Techniques
- Day 1-2: Study and implement sorting algorithms (quicksort, mergesort, etc.)
- Day 3-4: Learn about searching algorithms and binary search variations
- Day 5-6: Dive into recursion and dynamic programming
- Day 7: Practice problems focusing on these algorithms
Week 3: Advanced Topics and System Design
- Day 1-2: Study greedy algorithms and their applications
- Day 3-4: Learn about system design principles and practice designing simple systems
- Day 5-6: Focus on time and space complexity analysis
- Day 7: Mixed practice problems and mock interviews
Week 4: Practice and Mock Interviews
- Day 1-3: Solve medium to hard level problems on platforms like LeetCode or HackerRank
- Day 4-6: Conduct mock interviews with friends or use online platforms
- Day 7: Review weak areas and do final preparations
Leveraging Online Resources
In today’s digital age, there’s no shortage of online resources to help you prepare for coding interviews. Here are some top recommendations:
1. Coding Platforms
- LeetCode: Offers a vast collection of coding problems, many of which are frequently asked in real interviews.
- HackerRank: Provides a mix of algorithmic challenges and skill-based certifications.
- CodeSignal: Features a “Interview Practice” section with problems categorized by companies and roles.
2. Online Courses
- AlgoCademy: Offers interactive coding tutorials and AI-powered assistance for algorithmic problem-solving.
- Coursera’s “Algorithms Specialization”: A comprehensive course covering fundamental algorithms and data structures.
- MIT OpenCourseWare’s “Introduction to Algorithms”: Free access to lecture notes and problem sets from MIT’s renowned algorithms course.
3. Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell: A classic resource with 189 programming questions and solutions.
- “Elements of Programming Interviews” by Adnan Aziz, Tsung-Hsien Lee, and Amit Prakash: Covers core elements of programming interviews with a focus on problem-solving.
4. YouTube Channels
- Back To Back SWE: Offers in-depth explanations of common interview problems.
- Tech Dose: Provides tutorials on data structures, algorithms, and system design.
Mastering the Art of Problem-Solving
Coding interviews are not just about knowing algorithms; they’re about applying that knowledge to solve problems. Here’s a step-by-step approach to tackle coding interview questions:
- Understand the problem: Read the question carefully and ask clarifying questions if needed.
- Identify the inputs and outputs: Clearly define what you’re given and what you need to produce.
- Consider edge cases: Think about potential special cases or extreme inputs.
- Brainstorm solutions: Consider multiple approaches before settling on one.
- Plan your approach: Outline your solution in pseudocode or with a diagram.
- Implement the solution: Write clean, readable code.
- Test your code: Run through examples, including edge cases.
- Optimize: Consider time and space complexity, and look for ways to improve efficiency.
Practice this approach repeatedly with various problems to make it second nature.
Improving Your Coding Skills
While solving problems is crucial, don’t neglect the importance of writing clean, efficient code. Here are some tips to improve your coding skills:
- Practice consistent coding style: Follow industry-standard conventions for the language you’re using.
- Write readable code: Use meaningful variable names and add comments where necessary.
- Break down complex problems: Use helper functions to make your code more modular and easier to understand.
- Learn to use built-in functions and libraries: Familiarize yourself with the standard library of your chosen language.
- Optimize as you go: Consider time and space complexity while writing your initial solution.
Here’s an example of how you might solve a common coding interview problem using these principles:
def is_palindrome(s: str) -> bool:
"""
Check if a given string is a palindrome.
A palindrome reads the same backwards as forwards.
Args:
s (str): The input string to check
Returns:
bool: True if the string is a palindrome, False otherwise
Time complexity: O(n), where n is the length of the string
Space complexity: O(1), as we're using constant extra space
"""
# Remove non-alphanumeric characters and convert to lowercase
cleaned_s = ''.join(char.lower() for char in s if char.isalnum())
# Use two pointers to check if the string is a palindrome
left, right = 0, len(cleaned_s) - 1
while left < right:
if cleaned_s[left] != cleaned_s[right]:
return False
left += 1
right -= 1
return True
# Test the function
print(is_palindrome("A man, a plan, a canal: Panama")) # Should print True
print(is_palindrome("race a car")) # Should print False
This solution demonstrates clean code practices, efficient algorithm usage, and consideration for edge cases.
Mastering the Art of Communication
A often overlooked aspect of coding interviews is the ability to communicate effectively. Here are some tips to improve your communication during interviews:
- Think out loud: Explain your thought process as you work through the problem.
- Ask clarifying questions: Don’t hesitate to seek clarification on problem details or constraints.
- Explain your approach: Before you start coding, outline your planned solution to the interviewer.
- Discuss trade-offs: If there are multiple ways to solve the problem, explain why you chose your approach.
- Be open to feedback: If the interviewer provides hints or suggestions, incorporate them gracefully.
Preparing for System Design Questions
For more senior positions, system design questions are often a crucial part of the interview process. While mastering system design in a short time frame is challenging, you can still make significant progress:
- Understand basic components: Learn about databases, caching, load balancing, and microservices.
- Study scalability concepts: Familiarize yourself with horizontal vs. vertical scaling, sharding, and replication.
- Practice with real-world systems: Try to design simplified versions of popular systems like Twitter, Netflix, or Uber.
- Learn to estimate: Practice back-of-the-envelope calculations for system requirements.
Resources like “System Design Interview” by Alex Xu and the “System Design Primer” on GitHub can be invaluable for this preparation.
Managing Interview Anxiety
Coding interviews can be stressful, especially when you’re under time pressure. Here are some strategies to manage anxiety:
- Practice under timed conditions: Simulate interview conditions to get comfortable with time pressure.
- Use relaxation techniques: Deep breathing or meditation can help calm nerves before an interview.
- Prepare your environment: If it’s a virtual interview, ensure your setup is comfortable and distraction-free.
- Remember it’s a conversation: View the interview as a chance to showcase your skills, not just a test.
- Learn from each experience: Every interview, successful or not, is an opportunity to improve.
The Day Before the Interview
As your interview approaches, here’s what you should do the day before:
- Review your strongest areas: Go over problems you’re confident with to boost your morale.
- Lightly review weak areas: Briefly go over topics you find challenging, but don’t stress over mastering them at the last minute.
- Prepare your setup: If it’s a virtual interview, test your camera, microphone, and internet connection.
- Get a good night’s sleep: Proper rest is crucial for mental acuity during the interview.
- Plan your day: Know exactly when and where your interview is, and plan to arrive (or log in) early.
Conclusion
Preparing for coding interviews in a short time frame is challenging but not impossible. By focusing on fundamental concepts, practicing problem-solving techniques, and leveraging online resources, you can significantly improve your chances of success. Remember, the goal is not just to pass the interview, but to become a better problem solver and coder in the process.
Platforms like AlgoCademy can be invaluable in this journey, offering structured learning paths, interactive coding challenges, and AI-powered assistance to help you tackle complex algorithmic problems. With dedication, smart preparation, and the right resources, you can walk into your coding interview with confidence, ready to showcase your skills and land that dream job in tech.
Good luck with your preparation, and remember – every problem you solve brings you one step closer to acing that interview!