Test-Driven Development: What It Is and Why You Should Learn It
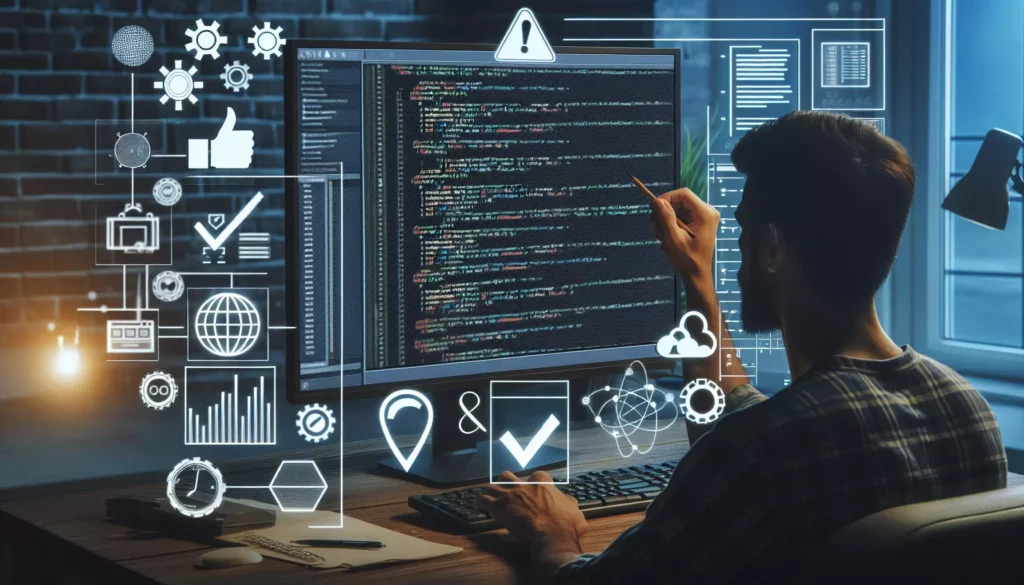
In the ever-evolving world of software development, methodologies and practices come and go. However, one approach that has stood the test of time and continues to gain traction is Test-Driven Development (TDD). If you’re a programmer looking to enhance your skills and improve the quality of your code, TDD is a methodology you should seriously consider learning. In this comprehensive guide, we’ll explore what TDD is, its benefits, potential drawbacks, and why it might be the game-changer you need in your coding journey.
What is Test-Driven Development?
Test-Driven Development is a software development process that relies on the repetition of a very short development cycle. It begins with the developer writing a failing automated test case that defines a desired improvement or new function. This is followed by producing the minimum amount of code to pass that test, and then refactoring the new code to acceptable standards.
The TDD cycle typically follows these steps:
- Write a test: The developer writes a test that defines a function or improvements of a function, which should fail initially.
- Run all tests: The developer runs all tests and sees if the new test fails.
- Write the code: The developer writes some code that causes the test to pass.
- Run tests: If all tests pass, the developer can be confident that the new code meets the test requirements.
- Refactor code: The developer refactors the code, making it cleaner and more efficient.
- Repeat: The process starts over with a new test.
This cycle is often referred to as “Red-Green-Refactor”, where “Red” represents the failing test, “Green” represents the passing test, and “Refactor” represents the optimization of the code.
The Benefits of Test-Driven Development
Test-Driven Development offers numerous benefits that can significantly improve your coding skills and the quality of your software. Let’s explore some of the key advantages:
1. Improved Code Quality
By writing tests before the actual code, you’re forced to think about the problem domain and what you want your code to do. This leads to better-designed, cleaner, and more maintainable code. The constant refactoring step in TDD also ensures that your code remains clean and efficient.
2. Better Code Coverage
Since you’re writing tests for every piece of functionality, TDD naturally leads to higher test coverage. This means more of your code is tested, reducing the likelihood of bugs and errors in production.
3. Easier Debugging
When a test fails, you know exactly where the problem is. This makes debugging much easier and faster, as you can pinpoint the issue to the most recent changes you’ve made.
4. Living Documentation
Your tests serve as documentation for your code. They describe what your code should do in various scenarios, making it easier for other developers (or yourself in the future) to understand the codebase.
5. Faster Development in the Long Run
While TDD might seem slower at first, it often leads to faster development in the long run. By catching bugs early and having a suite of tests to rely on, you spend less time debugging and more time adding new features.
6. Confidence in Code Changes
With a comprehensive test suite, you can make changes or refactor your code with confidence. If you break something, your tests will let you know immediately.
Potential Drawbacks of Test-Driven Development
While TDD offers many benefits, it’s important to be aware of some potential challenges:
1. Initial Slowdown
When first adopting TDD, you may experience a slowdown in development speed. Writing tests before code takes time, and there’s a learning curve involved.
2. Overemphasis on Unit Testing
TDD primarily focuses on unit tests, which might lead to neglecting other types of testing, such as integration or system tests.
3. Maintenance of Test Suite
As your project grows, so does your test suite. Maintaining a large number of tests can become time-consuming and complex.
4. Resistance to Change
Some developers may resist adopting TDD, especially if they’re used to traditional development methods.
Should You Learn Test-Driven Development?
The short answer is: yes, you should definitely consider learning Test-Driven Development. Here’s why:
1. Industry Demand
Many companies, especially those working on large-scale or mission-critical applications, value TDD skills. Learning TDD can make you more attractive to potential employers.
2. Code Quality Improvement
TDD can significantly improve the quality of your code, making you a better developer overall. It encourages you to think about edge cases and potential issues before you even start coding.
3. Confidence in Your Code
With TDD, you’ll have more confidence in your code. You’ll know that your code works as intended because you have tests to prove it.
4. Faster Debugging and Refactoring
TDD can save you time in the long run by making debugging easier and allowing you to refactor with confidence.
5. Better Understanding of Requirements
Writing tests first forces you to clearly understand the requirements before you start coding. This can lead to better-designed solutions and fewer misunderstandings.
How to Get Started with Test-Driven Development
If you’re convinced that TDD is worth learning, here are some steps to get started:
1. Learn the Basics of Unit Testing
Before diving into TDD, make sure you understand the basics of unit testing in your preferred programming language. Familiarize yourself with testing frameworks like JUnit for Java, pytest for Python, or Jest for JavaScript.
2. Start Small
Begin with simple problems and gradually work your way up to more complex scenarios. A good starting point might be implementing basic mathematical functions or string manipulations using TDD.
3. Practice the Red-Green-Refactor Cycle
Get comfortable with the TDD cycle. Write a failing test, make it pass with the simplest code possible, then refactor. Repeat this process until it becomes second nature.
4. Use TDD Katas
TDD katas are coding exercises specifically designed to practice TDD. Some popular ones include the FizzBuzz kata, the Roman Numerals kata, and the Bowling Game kata.
5. Apply TDD to Your Projects
Once you’re comfortable with the basics, start applying TDD to your personal or work projects. Start with new features or modules to get a feel for how TDD works in a real-world context.
6. Learn from the Community
Join TDD communities, attend workshops, or participate in coding dojos. Learning from experienced practitioners can provide valuable insights and help you avoid common pitfalls.
TDD in Practice: A Simple Example
Let’s walk through a simple example of TDD in practice. We’ll implement a function that checks if a number is prime using Python and the pytest framework.
Step 1: Write a Failing Test
First, we’ll write a test for our yet-to-be-implemented is_prime
function:
# test_prime.py
def test_is_prime():
assert is_prime(2) == True
assert is_prime(3) == True
assert is_prime(4) == False
assert is_prime(5) == True
assert is_prime(9) == False
assert is_prime(11) == True
Running this test will fail because we haven’t implemented the is_prime
function yet.
Step 2: Write the Minimum Code to Pass the Test
Now, let’s implement the is_prime
function with the minimum code to pass our tests:
# prime.py
def is_prime(n):
if n < 2:
return False
for i in range(2, int(n**0.5) + 1):
if n % i == 0:
return False
return True
Step 3: Run the Tests
Run the tests again. They should all pass now.
Step 4: Refactor (if necessary)
In this case, our implementation is already quite efficient, so we don’t need to refactor. However, in real-world scenarios, you might want to improve the code’s readability or performance at this stage.
Step 5: Add More Tests
Let’s add more tests to cover edge cases:
# test_prime.py
def test_is_prime_edge_cases():
assert is_prime(1) == False
assert is_prime(0) == False
assert is_prime(-1) == False
assert is_prime(97) == True
assert is_prime(100) == False
Run the tests again. If they all pass, great! If not, go back to step 2 and modify your code to handle these edge cases.
TDD and AlgoCademy: A Perfect Match
Test-Driven Development aligns perfectly with AlgoCademy’s focus on developing strong programming skills and preparing for technical interviews. Here’s how TDD can enhance your learning experience on AlgoCademy:
1. Problem-Solving Skills
TDD encourages you to think about the problem and its edge cases before diving into the implementation. This approach is crucial when solving algorithmic problems, which is a key focus of AlgoCademy.
2. Code Quality
AlgoCademy emphasizes writing clean, efficient code. TDD naturally leads to better-designed, more modular code, which is essential when tackling complex algorithms and data structures.
3. Debugging Practice
When preparing for technical interviews, being able to quickly identify and fix bugs is crucial. TDD provides ample practice in debugging, as you’re constantly writing tests and making them pass.
4. Confidence in Your Solutions
Having a comprehensive test suite gives you confidence in your solutions. This confidence is invaluable when facing challenging problems in AlgoCademy’s curriculum or during actual technical interviews.
5. Interview Preparation
Many tech companies value TDD skills. By learning and practicing TDD, you’re adding another valuable skill to your repertoire, potentially giving you an edge in interviews.
Conclusion: Embracing Test-Driven Development
Test-Driven Development is more than just a coding practice; it’s a mindset that can significantly improve your skills as a developer. While it may require an initial investment of time and effort to learn, the long-term benefits in terms of code quality, confidence, and efficiency make it well worth the effort.
As you progress through AlgoCademy’s curriculum and prepare for technical interviews, consider incorporating TDD into your learning process. Start small, be patient with yourself as you learn, and gradually apply TDD principles to more complex problems. You’ll likely find that it not only improves your code but also enhances your problem-solving skills and makes you a more confident programmer.
Remember, becoming proficient in TDD is a journey. It takes time and practice to fully internalize the TDD workflow and reap its benefits. But with persistence and the right mindset, you’ll find that TDD can be a powerful tool in your development toolkit, helping you write better code and tackle even the most challenging programming problems with confidence.
So, should you learn Test-Driven Development? Absolutely. Your future self – and your code – will thank you for it.