The Crucial Role of Math in Learning to Code: Unveiling the Connection
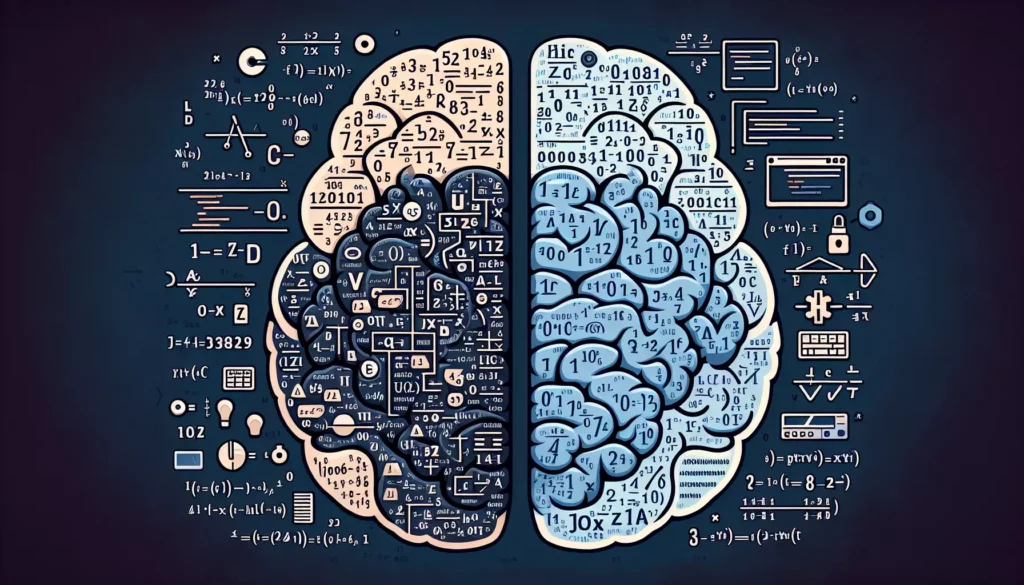
When embarking on a journey to learn coding, many aspiring programmers wonder about the importance of mathematics in their pursuit. The relationship between math and coding is both intricate and fundamental, playing a crucial role in developing strong programming skills. In this comprehensive guide, we’ll explore the significance of math in coding education, its practical applications, and how it contributes to becoming a proficient programmer.
The Fundamental Connection Between Math and Coding
At its core, coding is a form of problem-solving that relies heavily on logical thinking and abstract reasoning – skills that are deeply rooted in mathematical concepts. While not all programming tasks require advanced mathematical knowledge, a solid foundation in math can significantly enhance a coder’s ability to understand and implement complex algorithms, data structures, and computational processes.
Key Mathematical Concepts in Coding
- Algebra: Used in variable manipulation and equation solving
- Logic: Essential for creating conditional statements and boolean operations
- Set theory: Fundamental for understanding data structures like arrays and hash tables
- Graph theory: Crucial for network algorithms and data visualization
- Discrete mathematics: Important for algorithmic analysis and optimization
- Statistics and probability: Vital for data science and machine learning applications
How Math Enhances Coding Skills
Understanding mathematical concepts can significantly improve a programmer’s ability to write efficient, scalable, and optimized code. Here are some ways in which math contributes to coding proficiency:
1. Problem-Solving and Algorithmic Thinking
Mathematics trains the mind to break down complex problems into smaller, manageable parts – a skill directly applicable to algorithmic thinking in programming. When faced with a coding challenge, programmers often use mathematical reasoning to devise step-by-step solutions, much like solving a math problem.
2. Efficiency and Optimization
Mathematical knowledge helps programmers understand the time and space complexity of algorithms, enabling them to create more efficient code. Concepts like Big O notation, which describes the performance or complexity of an algorithm, are rooted in mathematical principles.
3. Data Structures and Algorithms
Many data structures and algorithms used in programming are based on mathematical concepts. For example, binary search trees leverage principles of binary systems and logarithms, while graph algorithms often rely on concepts from graph theory.
4. Machine Learning and AI
Advanced areas of computer science, such as machine learning and artificial intelligence, heavily rely on mathematical concepts. Linear algebra, calculus, and statistics form the backbone of many ML algorithms and neural network architectures.
5. Computer Graphics and Game Development
Creating visually appealing graphics and realistic game physics requires a strong understanding of geometry, trigonometry, and linear algebra. These mathematical concepts are essential for rendering 3D objects, calculating trajectories, and simulating physical interactions.
Math in Different Programming Domains
The importance of math varies across different programming domains. Let’s explore how math applies to various areas of software development:
Web Development
While basic web development may not require advanced math skills, certain aspects benefit from mathematical knowledge:
- Responsive design calculations
- CSS animations and transformations
- JavaScript-based data visualizations
- Performance optimization and load time calculations
Mobile App Development
Mobile app developers can leverage math in several ways:
- UI layout and scaling calculations
- Implementing custom animations and transitions
- Optimizing app performance and resource usage
- Location-based services and GPS calculations
Data Science and Analytics
This field heavily relies on mathematical concepts:
- Statistical analysis and hypothesis testing
- Predictive modeling and regression analysis
- Data visualization and interpretation
- Machine learning algorithms and neural networks
Game Development
Game developers frequently use math in various aspects of game creation:
- Physics simulations and collision detection
- 3D graphics rendering and shading
- Procedural content generation
- AI behavior and pathfinding algorithms
Essential Mathematical Skills for Programmers
While the level of math required may vary depending on the specific programming field, there are some fundamental mathematical skills that benefit all programmers:
1. Algebra
Algebraic thinking is crucial for understanding variables, functions, and equations in programming. It helps in:
- Manipulating and solving equations
- Understanding function composition and decomposition
- Working with abstract data types
2. Logic and Boolean Algebra
These concepts are fundamental to programming logic and control flow:
- Creating and evaluating conditional statements
- Understanding truth tables and logical operators
- Implementing efficient branching and decision-making in code
3. Set Theory
Set theory provides a foundation for understanding data structures and database operations:
- Working with arrays, lists, and other collections
- Understanding database operations like unions and intersections
- Implementing efficient search and sorting algorithms
4. Graph Theory
Graph theory concepts are valuable in various programming applications:
- Network analysis and optimization
- Social network algorithms
- Pathfinding and navigation systems
5. Discrete Mathematics
Discrete math provides tools for analyzing algorithms and data structures:
- Understanding algorithmic complexity and Big O notation
- Analyzing recursive algorithms
- Working with combinatorics and probability in programming
Practical Examples of Math in Coding
To illustrate the practical application of math in coding, let’s look at some common programming tasks that involve mathematical concepts:
1. Calculating Fibonacci Numbers
The Fibonacci sequence is a classic example that combines recursive thinking with mathematical series:
function fibonacci(n) {
if (n <= 1) return n;
return fibonacci(n - 1) + fibonacci(n - 2);
}
2. Implementing a Binary Search
Binary search uses logarithmic time complexity, a concept rooted in mathematics:
function binarySearch(arr, target) {
let left = 0;
let right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
3. Calculating Prime Numbers
Determining prime numbers involves understanding number theory and efficient algorithms:
function isPrime(n) {
if (n <= 1) return false;
for (let i = 2; i <= Math.sqrt(n); i++) {
if (n % i === 0) return false;
}
return true;
}
4. Implementing a Simple Neural Network
Neural networks rely heavily on linear algebra and calculus:
class SimpleNeuralNetwork {
constructor(inputNodes, hiddenNodes, outputNodes) {
this.inputNodes = inputNodes;
this.hiddenNodes = hiddenNodes;
this.outputNodes = outputNodes;
this.weights_ih = new Matrix(this.hiddenNodes, this.inputNodes);
this.weights_ho = new Matrix(this.outputNodes, this.hiddenNodes);
this.weights_ih.randomize();
this.weights_ho.randomize();
this.bias_h = new Matrix(this.hiddenNodes, 1);
this.bias_o = new Matrix(this.outputNodes, 1);
this.bias_h.randomize();
this.bias_o.randomize();
}
// ... (additional methods for forward propagation, training, etc.)
}
Overcoming Math Anxiety in Coding
For those who feel intimidated by the mathematical aspects of coding, it’s important to remember that many programming tasks don’t require advanced math skills. Here are some strategies to overcome math anxiety and build confidence in your coding journey:
1. Focus on Practical Applications
Start by learning the math concepts that directly apply to your programming interests. For example, if you’re into web development, focus on the math needed for responsive layouts and animations.
2. Learn Math Through Coding
Use coding projects to reinforce mathematical concepts. Implement algorithms that solve mathematical problems to see the direct connection between math and programming.
3. Utilize Online Resources
Take advantage of online courses, tutorials, and interactive platforms that teach math concepts in the context of programming. Websites like Khan Academy, Coursera, and Codecademy offer courses that blend math and coding instruction.
4. Practice Regularly
Consistent practice is key to improving both math and coding skills. Set aside time for coding challenges that incorporate mathematical thinking, such as those found on platforms like LeetCode or HackerRank.
5. Collaborate and Seek Help
Join coding communities or study groups where you can discuss mathematical concepts with peers. Don’t hesitate to ask for help or explanations when you encounter challenging math-related coding problems.
The Future of Math in Coding
As technology continues to advance, the intersection of mathematics and coding is likely to become even more pronounced. Emerging fields such as quantum computing, advanced AI, and complex data analysis will require programmers with strong mathematical foundations. By developing a solid understanding of mathematical concepts alongside coding skills, programmers can position themselves for success in these cutting-edge areas.
Conclusion
While it’s possible to learn coding without an extensive mathematical background, having a strong foundation in math can significantly enhance your programming skills and open up more opportunities in the field. Math provides the logical thinking and problem-solving skills that are at the heart of effective coding.
Remember that learning to code is a journey, and improving your math skills can be part of that journey. As you progress in your coding education, you’ll naturally encounter mathematical concepts that are relevant to your work. Embrace these opportunities to deepen your understanding of both math and coding, and you’ll find yourself becoming a more versatile and capable programmer.
Whether you’re just starting out or looking to advance your coding skills, platforms like AlgoCademy can provide valuable resources and guidance. With interactive tutorials, AI-powered assistance, and a focus on algorithmic thinking, AlgoCademy can help you develop the mathematical and coding skills needed to tackle complex programming challenges and prepare for technical interviews at top tech companies.
Ultimately, the importance of math in coding lies not just in its direct applications, but in the way it shapes your approach to problem-solving and logical thinking. By cultivating these skills, you’ll be well-equipped to face the diverse challenges of the ever-evolving world of programming.