How to Learn Data Structures and Algorithms Effectively: A Comprehensive Guide
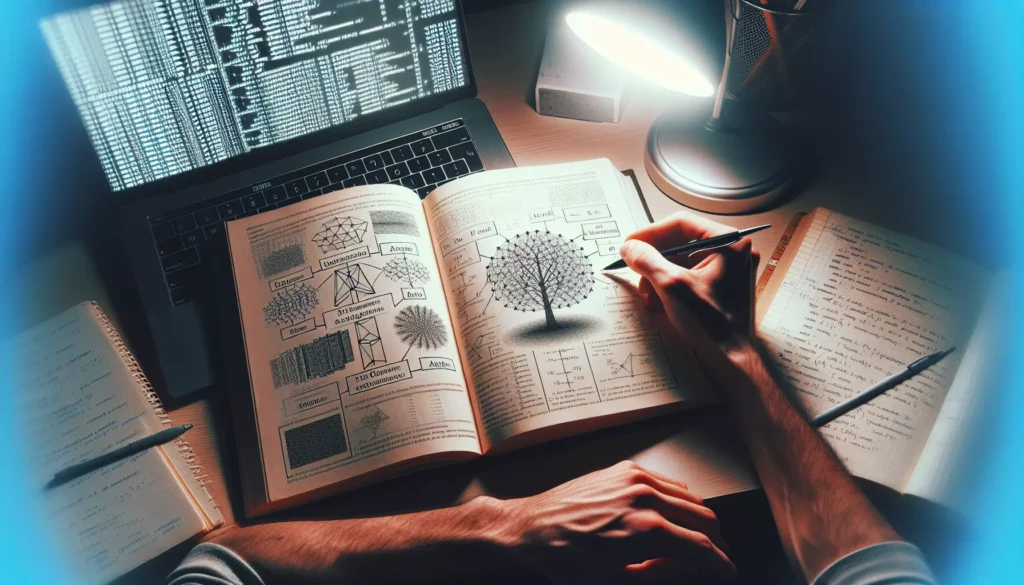
In the ever-evolving world of computer science and software development, mastering data structures and algorithms is crucial for becoming a proficient programmer. Whether you’re a beginner looking to build a strong foundation or an experienced developer aiming to ace technical interviews at top tech companies, this guide will provide you with effective strategies to learn data structures and algorithms.
Table of Contents
- Understanding the Importance of Data Structures and Algorithms
- Building a Strong Foundation
- Choosing the Right Learning Resources
- Hands-on Practice and Problem Solving
- Utilizing Visualization Techniques
- Understanding Time and Space Complexity
- Creating a Structured Learning Path
- Participating in Coding Challenges and Competitions
- Engaging in Peer Learning and Discussion
- Exploring Real-world Applications
- Preparing for Technical Interviews
- Embracing Continuous Learning
- Conclusion
1. Understanding the Importance of Data Structures and Algorithms
Before diving into the learning process, it’s essential to grasp why data structures and algorithms are so crucial in the field of computer science and software development:
- Problem-solving skills: Learning data structures and algorithms sharpens your ability to break down complex problems into manageable components.
- Efficiency: Proper use of data structures and algorithms leads to more efficient and optimized code.
- Scalability: Understanding these concepts allows you to build systems that can handle large amounts of data and users.
- Interview preparation: Many tech companies, especially FAANG (Facebook, Amazon, Apple, Netflix, Google), heavily emphasize these topics in their interview processes.
- Foundation for advanced topics: A solid grasp of data structures and algorithms is essential for understanding more advanced computer science concepts.
2. Building a Strong Foundation
To effectively learn data structures and algorithms, you need to have a strong foundation in programming basics:
- Choose a programming language: Pick a language you’re comfortable with or one that’s commonly used in interviews (e.g., Python, Java, C++, JavaScript).
- Master the basics: Ensure you have a good understanding of variables, data types, control structures, functions, and object-oriented programming concepts.
- Learn about memory management: Understand how memory allocation works in your chosen language, as this knowledge is crucial for many data structures.
- Study basic math concepts: Refresh your knowledge of algebra, logarithms, and basic probability, as these often come up in algorithm analysis.
3. Choosing the Right Learning Resources
With countless resources available, it’s important to select those that best suit your learning style and goals:
- Online courses: Platforms like Coursera, edX, and Udacity offer comprehensive courses on data structures and algorithms.
- Books: Classic texts like “Introduction to Algorithms” by Cormen et al. and “Cracking the Coding Interview” by Gayle Laakmann McDowell are invaluable resources.
- Interactive platforms: Websites like AlgoCademy, LeetCode, and HackerRank provide hands-on coding exercises and tutorials.
- Video tutorials: YouTube channels and online video courses can offer visual explanations of complex concepts.
- University lecture notes: Many top universities publish their computer science course materials online for free.
4. Hands-on Practice and Problem Solving
Theory alone is not enough; practical application is key to mastering data structures and algorithms:
- Implement from scratch: Try coding basic data structures (like linked lists, stacks, queues) and algorithms (sorting, searching) from scratch to truly understand their inner workings.
- Solve coding problems: Regularly practice solving algorithmic problems on platforms like AlgoCademy, LeetCode, or HackerRank.
- Start with easy problems: Begin with simpler problems to build confidence, then gradually increase the difficulty.
- Focus on understanding: Don’t just memorize solutions; strive to understand the underlying principles and patterns.
- Implement multiple solutions: For each problem, try to come up with different approaches and compare their efficiency.
5. Utilizing Visualization Techniques
Visual aids can greatly enhance your understanding of complex data structures and algorithms:
- Use online visualizers: Tools like VisuAlgo and Algorithm Visualizer can help you see how algorithms work step-by-step.
- Draw diagrams: Sketch out data structures and algorithm flow on paper or using digital drawing tools.
- Animate your code: Use tools like Python Tutor to visualize code execution and memory allocation.
- Create mental models: Develop mental representations of how different data structures and algorithms function.
6. Understanding Time and Space Complexity
Analyzing the efficiency of algorithms is a crucial skill:
- Learn Big O notation: Understand how to express the time and space complexity of algorithms using Big O notation.
- Analyze common algorithms: Study the time and space complexity of fundamental algorithms like sorting and searching.
- Practice complexity analysis: For each problem you solve, try to determine its time and space complexity.
- Compare algorithms: Understand the trade-offs between different algorithms for the same problem.
7. Creating a Structured Learning Path
Organize your learning journey to ensure you cover all essential topics:
- Basic data structures: Arrays, linked lists, stacks, queues
- Advanced data structures: Trees, graphs, heaps, hash tables
- Sorting algorithms: Bubble sort, insertion sort, merge sort, quicksort
- Searching algorithms: Linear search, binary search
- Graph algorithms: BFS, DFS, Dijkstra’s algorithm, minimum spanning trees
- Dynamic programming
- Greedy algorithms
- String manipulation algorithms
- Bit manipulation
8. Participating in Coding Challenges and Competitions
Engage in competitive programming to sharpen your skills:
- Join coding contests: Participate in platforms like Codeforces, TopCoder, or Google Code Jam.
- Time-bound practice: Solve problems under time constraints to improve your speed and efficiency.
- Learn from others: After contests, study the solutions of top performers to learn new techniques.
- Collaborate: Form or join a competitive programming group to practice and learn together.
9. Engaging in Peer Learning and Discussion
Collaborative learning can significantly enhance your understanding:
- Join online communities: Participate in forums like Stack Overflow, Reddit’s r/algorithms, or Discord servers dedicated to programming.
- Form study groups: Connect with peers who are also learning data structures and algorithms.
- Attend meetups or workshops: Look for local or online events focused on algorithms and problem-solving.
- Explain concepts to others: Teaching or explaining concepts to peers can reinforce your own understanding.
10. Exploring Real-world Applications
Understanding how data structures and algorithms are used in practice can boost motivation and provide context:
- Study case studies: Look into how major tech companies use specific algorithms to solve real-world problems.
- Contribute to open-source projects: Find projects that implement interesting algorithms and contribute to them.
- Build your own projects: Create applications that utilize the data structures and algorithms you’ve learned.
- Analyze existing systems: Study the algorithms behind technologies you use daily, like search engines or recommendation systems.
11. Preparing for Technical Interviews
If your goal is to excel in technical interviews, especially at FAANG companies, consider these additional steps:
- Mock interviews: Practice with peers or use platforms like Pramp for simulated interview experiences.
- System design: Learn about designing large-scale distributed systems, as this is often part of senior-level interviews.
- Behavioral questions: Prepare for non-technical aspects of interviews by practicing common behavioral questions.
- Company-specific preparation: Research the interview process and common questions asked by your target companies.
- Whiteboard practice: Get comfortable explaining your thought process and coding on a whiteboard or shared document.
12. Embracing Continuous Learning
The field of computer science is constantly evolving, so it’s important to cultivate a mindset of continuous learning:
- Stay updated: Follow computer science journals, blogs, and news to keep up with the latest developments in algorithms and data structures.
- Revisit concepts: Regularly review and deepen your understanding of previously learned topics.
- Explore advanced topics: Once you’re comfortable with the basics, delve into more complex areas like parallel algorithms or machine learning algorithms.
- Attend conferences: Participate in computer science conferences or watch recorded talks to learn about cutting-edge research and applications.
Conclusion
Learning data structures and algorithms effectively is a journey that requires dedication, practice, and a structured approach. By following the strategies outlined in this guide, you can build a strong foundation, develop problem-solving skills, and prepare yourself for success in technical interviews and your career as a software developer.
Remember that mastery comes with time and consistent effort. Don’t be discouraged by initial difficulties; each problem you solve and concept you grasp brings you one step closer to becoming a proficient programmer. Embrace the challenge, stay curious, and enjoy the process of expanding your knowledge and skills in this fundamental area of computer science.
With platforms like AlgoCademy offering interactive tutorials, AI-powered assistance, and a wealth of resources, you have more tools than ever at your disposal to support your learning journey. Take advantage of these resources, practice regularly, and soon you’ll find yourself confidently tackling complex algorithmic problems and excelling in technical interviews at top tech companies.
Happy coding, and may your algorithms always be efficient and your data structures well-organized!