Essential Tools Every Programmer Should Know: Boosting Your Coding Efficiency
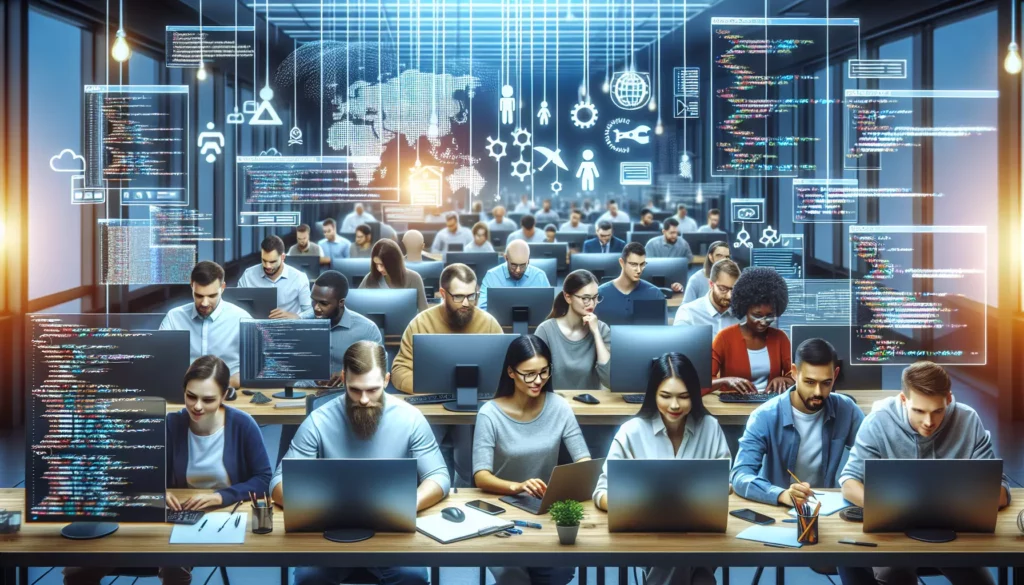
In the ever-evolving world of programming, having the right tools at your disposal can make a significant difference in your productivity and efficiency. Whether you’re a beginner just starting your coding journey or an experienced developer looking to optimize your workflow, this comprehensive guide will introduce you to the essential tools that every programmer should know. From integrated development environments (IDEs) to version control systems, we’ll explore a wide range of tools that can help you write better code, collaborate more effectively, and streamline your development process.
1. Integrated Development Environments (IDEs)
An Integrated Development Environment (IDE) is a software application that provides comprehensive facilities for software development. It typically includes a source code editor, build automation tools, and a debugger. IDEs are essential for programmers as they offer a centralized interface for writing, testing, and debugging code.
Popular IDEs:
- Visual Studio Code: A lightweight, yet powerful, open-source IDE developed by Microsoft. It supports multiple programming languages and has a vast ecosystem of extensions.
- IntelliJ IDEA: A Java-centric IDE known for its intelligent code completion and refactoring capabilities.
- PyCharm: An IDE specifically designed for Python development, offering advanced features like code analysis and debugging.
- Eclipse: A versatile IDE that supports multiple programming languages and is particularly popular for Java development.
Choosing the right IDE depends on your preferred programming language and specific needs. Many IDEs offer free versions or trial periods, so it’s worth experimenting with different options to find the one that suits you best.
2. Version Control Systems
Version control systems (VCS) are essential tools for managing and tracking changes in your codebase. They allow you to collaborate with other developers, maintain a history of your project, and easily revert to previous versions if needed.
Popular Version Control Systems:
- Git: The most widely used distributed version control system. It’s fast, flexible, and supports branching and merging.
- Subversion (SVN): A centralized version control system that’s simpler to use than Git but less flexible.
- Mercurial: Another distributed version control system that’s known for its simplicity and ease of use.
Git has become the de facto standard for version control in the software development industry. Learning Git is crucial for any programmer, as it’s used in most modern development workflows.
Git Hosting Platforms:
- GitHub: The most popular Git repository hosting service, offering features like issue tracking, pull requests, and project management tools.
- GitLab: An alternative to GitHub that provides both cloud-hosted and self-hosted options.
- Bitbucket: Another Git hosting platform that integrates well with other Atlassian tools like Jira and Confluence.
3. Text Editors
While IDEs offer comprehensive features, sometimes you need a lightweight tool for quick edits or working with individual files. Text editors are perfect for this purpose and often come with syntax highlighting and other helpful features for coding.
Popular Text Editors:
- Sublime Text: A fast and feature-rich text editor with a large ecosystem of plugins.
- Atom: An open-source, customizable text editor developed by GitHub.
- Notepad++: A lightweight, Windows-specific text editor with support for multiple programming languages.
- Vim: A highly configurable text editor that’s known for its efficiency once mastered.
Many programmers use a combination of IDEs and text editors depending on the task at hand. It’s valuable to be proficient with at least one text editor, as they can be useful in various scenarios, such as editing configuration files or making quick changes to scripts.
4. Package Managers
Package managers are tools that automate the process of installing, updating, configuring, and removing software packages or libraries. They are essential for managing dependencies in your projects and keeping your development environment organized.
Popular Package Managers:
- npm (Node Package Manager): The default package manager for JavaScript and Node.js.
- pip: The package installer for Python.
- Maven: A build automation and project management tool primarily used for Java projects.
- Composer: A dependency manager for PHP.
Learning to use package managers effectively can save you a lot of time and effort in managing project dependencies and keeping your development environment consistent across different machines.
5. Debugging Tools
Debugging is an essential part of the development process, and having the right tools can make it much easier to identify and fix issues in your code.
Popular Debugging Tools:
- Browser Developer Tools: Built-in debugging tools in web browsers like Chrome DevTools or Firefox Developer Tools.
- GDB (GNU Debugger): A powerful debugger for C, C++, and other languages.
- pdb: The built-in Python debugger.
- Visual Studio Debugger: An integrated debugger in Visual Studio for .NET and C++ development.
Many IDEs come with built-in debugging tools, but it’s also valuable to be familiar with standalone debuggers for more complex scenarios or when working in environments where an IDE is not available.
6. Containerization Tools
Containerization has become increasingly important in modern software development, allowing developers to package applications with their dependencies and run them consistently across different environments.
Popular Containerization Tools:
- Docker: The most widely used containerization platform, allowing you to build, ship, and run applications in containers.
- Kubernetes: An open-source container orchestration system for automating application deployment, scaling, and management.
- Podman: An alternative to Docker that doesn’t require a daemon and can run containers as a non-root user.
Learning containerization tools like Docker can greatly simplify the process of deploying and scaling applications, especially in cloud environments.
7. Continuous Integration/Continuous Deployment (CI/CD) Tools
CI/CD tools automate the process of building, testing, and deploying code changes. They are essential for maintaining code quality and enabling rapid, reliable software delivery.
Popular CI/CD Tools:
- Jenkins: An open-source automation server that supports building, deploying, and automating any project.
- GitLab CI/CD: An integrated CI/CD solution that’s part of the GitLab platform.
- Travis CI: A cloud-based CI service that integrates well with GitHub repositories.
- CircleCI: Another cloud-based CI/CD platform known for its speed and flexibility.
Implementing CI/CD practices with these tools can help improve code quality, reduce bugs, and accelerate the development process.
8. Code Analysis Tools
Code analysis tools help identify potential issues, enforce coding standards, and improve overall code quality. They can catch bugs early in the development process and help maintain consistent coding practices across a team.
Popular Code Analysis Tools:
- ESLint: A static code analysis tool for identifying problematic patterns in JavaScript code.
- SonarQube: An open-source platform for continuous inspection of code quality.
- Pylint: A static code analysis tool for Python that checks for errors and enforces a coding standard.
- ReSharper: A popular productivity tool for .NET developers that includes code analysis features.
Integrating code analysis tools into your development workflow can help catch potential issues early and improve the overall quality of your codebase.
9. API Development and Testing Tools
For developers working with APIs, having the right tools for development, testing, and documentation is crucial.
Popular API Tools:
- Postman: A popular tool for API development and testing, allowing you to send HTTP requests and analyze responses.
- Swagger: An open-source framework for designing, building, and documenting RESTful APIs.
- cURL: A command-line tool for transferring data using various protocols, often used for testing APIs.
- Insomnia: A cross-platform HTTP client that simplifies the process of interacting with REST APIs.
These tools can significantly streamline the process of developing and testing APIs, making it easier to create robust and well-documented interfaces.
10. Collaboration and Project Management Tools
While not strictly programming tools, collaboration and project management tools are essential for working effectively in teams and managing complex projects.
Popular Collaboration and Project Management Tools:
- Slack: A popular team communication platform that integrates with many development tools.
- Jira: An issue tracking and project management tool widely used in software development.
- Trello: A flexible, card-based project management tool suitable for various methodologies.
- Confluence: A team workspace for creating, organizing, and collaborating on work.
Effective use of these tools can greatly improve team communication, project organization, and overall productivity.
11. Performance Profiling Tools
Performance profiling tools help identify bottlenecks and optimize the performance of your applications. They are crucial for ensuring that your code runs efficiently and scales well.
Popular Performance Profiling Tools:
- JProfiler: A Java profiler that helps you find performance bottlenecks, memory leaks, and resolve threading issues.
- Valgrind: An instrumentation framework for building dynamic analysis tools, particularly useful for C and C++ programs.
- Chrome DevTools Performance tab: Built-in performance profiling tools for web applications in Chrome.
- dotTrace: A performance profiler for .NET applications.
Learning to use performance profiling tools effectively can help you optimize your code and create more efficient applications.
12. Database Management Tools
For developers working with databases, having tools to manage, query, and analyze data is essential.
Popular Database Management Tools:
- MySQL Workbench: A visual database design and management tool for MySQL databases.
- pgAdmin: An open-source administration and management tool for PostgreSQL databases.
- MongoDB Compass: A GUI for MongoDB that allows you to explore and manipulate your data.
- DBeaver: A universal database tool supporting multiple database systems.
These tools can greatly simplify database management tasks and help you work more efficiently with your data.
13. Cloud Development Tools
As cloud computing becomes increasingly prevalent, familiarity with cloud development tools is becoming essential for many programmers.
Popular Cloud Development Tools:
- AWS CLI: Command-line interface for interacting with Amazon Web Services.
- Azure CLI: Command-line tool for managing Microsoft Azure resources.
- Google Cloud SDK: Set of tools for managing resources and applications hosted on Google Cloud Platform.
- Terraform: An infrastructure-as-code tool for building, changing, and versioning infrastructure safely and efficiently.
Familiarity with these tools can help you leverage cloud services effectively and manage cloud infrastructure more efficiently.
14. Documentation Tools
Good documentation is crucial for maintaining and sharing code. Documentation tools can help you create clear, organized documentation for your projects.
Popular Documentation Tools:
- Doxygen: A documentation generator for various programming languages.
- Sphinx: A tool that makes it easy to create intelligent and beautiful documentation, originally created for Python documentation.
- JSDoc: An API documentation generator for JavaScript.
- Javadoc: A documentation generator for Java.
Using these tools can help you create comprehensive and maintainable documentation for your projects, making it easier for others (and your future self) to understand and work with your code.
15. Machine Learning and Data Science Tools
For developers working in machine learning and data science, there are several essential tools and libraries to be familiar with.
Popular Machine Learning and Data Science Tools:
- TensorFlow: An open-source machine learning framework developed by Google.
- PyTorch: An open-source machine learning library developed by Facebook’s AI Research lab.
- scikit-learn: A machine learning library for Python.
- Jupyter Notebook: An open-source web application that allows you to create and share documents containing live code, equations, visualizations, and narrative text.
These tools are essential for anyone working in the fields of machine learning and data science, providing powerful capabilities for data analysis, model development, and visualization.
Conclusion
The tools mentioned in this guide represent just a fraction of the vast ecosystem available to programmers. As you progress in your coding journey, you’ll likely discover additional tools that suit your specific needs and preferences. The key is to continually explore and learn about new tools that can enhance your productivity and help you write better code.
Remember, while tools are important, they are just that – tools. The most crucial aspects of programming are understanding fundamental concepts, developing problem-solving skills, and writing clean, efficient code. Tools should complement your skills and knowledge, not replace them.
As you explore these tools, consider how they fit into your workflow and how they can help you become a more effective programmer. Don’t feel pressured to master every tool out there; instead, focus on becoming proficient with the tools that are most relevant to your work and interests.
Lastly, keep in mind that the field of programming is constantly evolving, and new tools are always emerging. Stay curious, keep learning, and don’t be afraid to experiment with new tools and technologies. Happy coding!