Object-Oriented Programming: The Backbone of Modern Software Development
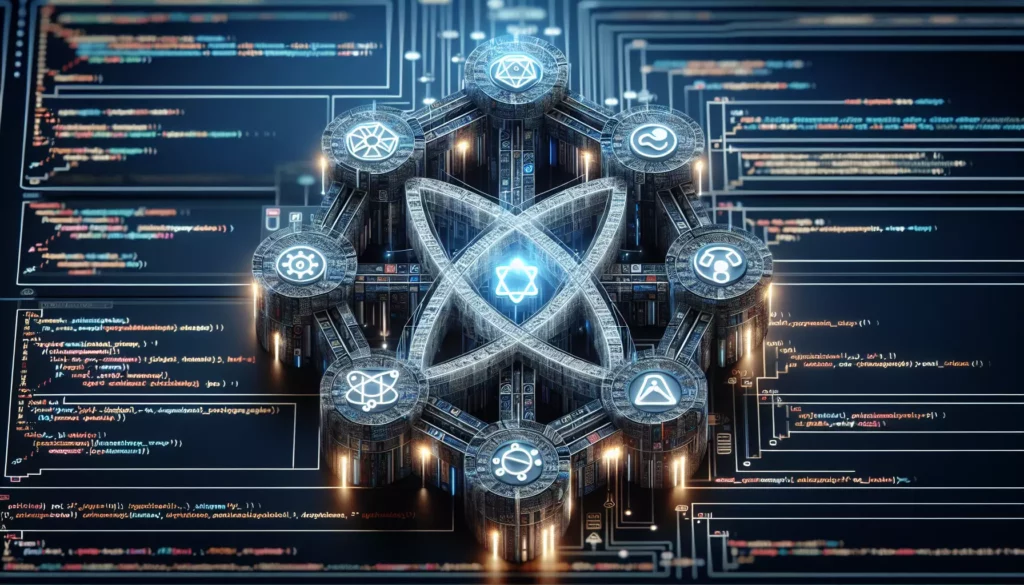
In the ever-evolving world of software development, object-oriented programming (OOP) stands as a cornerstone methodology that has revolutionized how we design and build complex systems. Whether you’re a beginner just starting your coding journey or an experienced developer looking to refine your skills for technical interviews at top tech companies, understanding OOP is crucial. In this comprehensive guide, we’ll dive deep into the concept of object-oriented programming, explore its importance, and see how it applies to real-world scenarios, particularly in the context of preparing for coding interviews at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google).
What is Object-Oriented Programming?
Object-Oriented Programming is a programming paradigm that organizes software design around data, or objects, rather than functions and logic. An object is a data field that has unique attributes and behavior. OOP focuses on the objects that developers want to manipulate rather than the logic required to manipulate them.
This approach to programming is well-suited for programs that are large, complex, and actively updated or maintained. By creating objects, OOP allows for code reuse, scalability, and efficiency in a way that’s difficult to achieve with other programming paradigms.
The Four Pillars of OOP
To truly understand object-oriented programming, it’s essential to grasp its four fundamental principles:
- Encapsulation: This principle involves bundling the data and the methods that operate on that data within one unit, like a class in Java or Python. Encapsulation also means restricting direct access to some of an object’s components, which is a means of preventing accidental interference and misuse of the methods and data.
- Abstraction: Abstraction means hiding complex implementation details and showing only the necessary features of an object. It helps in reducing programming complexity and effort.
- Inheritance: This allows new classes to be based on existing classes, inheriting their attributes and methods. Inheritance supports the concept of hierarchical classification and promotes code reusability.
- Polymorphism: Literally meaning “many forms,” polymorphism allows objects of different classes to be treated as objects of a common super class. It provides a way to perform a single action in different forms and the ability to redefine methods for derived classes.
Why is Object-Oriented Programming Important?
OOP has become the predominant programming paradigm in modern software development for several compelling reasons:
1. Modularity for Easier Troubleshooting
Object-oriented programming allows you to break down your software into bite-sized problems that you can solve one at a time. This reduces the complexity of your overall program and makes it easier to understand and maintain. When troubleshooting, you can zero in on a specific module without having to understand the entire system.
2. Reuse of Code Through Inheritance
In OOP, new classes can be created based on existing classes. This means you can use the existing code without having to rewrite it, saving time and reducing redundancy. This concept is particularly useful when preparing for coding interviews, as it demonstrates your ability to write efficient and scalable code.
3. Flexibility Through Polymorphism
Polymorphism allows you to use a single interface to represent different types of actions. This flexibility can lead to more intuitive design of complex systems and can make your code more adaptable to change.
4. Effective Problem Modeling
OOP allows you to model real-world problems by representing them with objects and classes. This intuitive approach to problem-solving can be especially beneficial when tackling complex coding challenges during technical interviews.
5. Security Through Encapsulation
By bundling data and methods together and restricting access to internal details, OOP provides a level of security that’s harder to achieve with other programming paradigms. This is crucial when working on large-scale projects or when data integrity is paramount.
OOP in Action: A Practical Example
To better understand how OOP works in practice, let’s consider a simple example that might come up in a coding interview. We’ll create a basic structure for a library system using Python.
class Book:
def __init__(self, title, author, isbn):
self.title = title
self.author = author
self.isbn = isbn
self.is_checked_out = False
def check_out(self):
if not self.is_checked_out:
self.is_checked_out = True
return True
return False
def return_book(self):
if self.is_checked_out:
self.is_checked_out = False
return True
return False
class Library:
def __init__(self):
self.books = []
def add_book(self, book):
self.books.append(book)
def check_out_book(self, isbn):
for book in self.books:
if book.isbn == isbn:
return book.check_out()
return False
def return_book(self, isbn):
for book in self.books:
if book.isbn == isbn:
return book.return_book()
return False
# Usage
library = Library()
book1 = Book("The Great Gatsby", "F. Scott Fitzgerald", "9780743273565")
book2 = Book("To Kill a Mockingbird", "Harper Lee", "9780446310789")
library.add_book(book1)
library.add_book(book2)
print(library.check_out_book("9780743273565")) # True
print(library.check_out_book("9780743273565")) # False (already checked out)
print(library.return_book("9780743273565")) # True
print(library.return_book("9780743273565")) # False (already returned)
In this example, we’ve created two classes: Book
and Library
. The Book
class encapsulates the properties of a book (title, author, ISBN) and methods to check out and return the book. The Library
class manages a collection of books and provides methods to add books, check them out, and return them.
This structure demonstrates several OOP principles:
- Encapsulation: The
Book
class encapsulates the data (title, author, ISBN) and the methods that operate on that data (check_out, return_book). - Abstraction: The
Library
class provides a high-level interface for managing books, hiding the complexities of how books are stored and manipulated. - Potential for Inheritance: We could easily create subclasses of
Book
, such asTextbook
orNovel
, that inherit from the baseBook
class and add specific attributes or methods. - Polymorphism: If we had different types of books (e.g., E-books, Audiobooks) that all implemented a
check_out
method, we could treat them polymorphically in theLibrary
class.
OOP and Technical Interviews
When preparing for technical interviews, especially for positions at major tech companies like FAANG, understanding and applying OOP principles is crucial. Here’s why:
1. Design Patterns
Many common design patterns in software development are based on OOP principles. Familiarity with these patterns and the ability to implement them using OOP can set you apart in an interview. Some popular OOP-based design patterns include:
- Singleton Pattern
- Factory Pattern
- Observer Pattern
- Strategy Pattern
2. System Design Questions
System design questions are common in interviews at companies like Google, Facebook, or Amazon. OOP principles are fundamental to designing scalable and maintainable systems. Being able to model complex systems using classes and objects, and explain your design decisions using OOP terminology, can greatly impress your interviewers.
3. Code Organization and Readability
Interviewers often evaluate your ability to write clean, organized, and readable code. OOP, when used correctly, promotes these qualities. By encapsulating related functionality within classes and using meaningful names for classes and methods, you demonstrate your ability to write professional-grade code.
4. Problem-Solving Approach
OOP encourages a particular approach to problem-solving: breaking down complex problems into smaller, manageable objects. This approach aligns well with how many tech companies approach large-scale software development.
Common OOP Interview Questions
To help you prepare for OOP-related questions in technical interviews, here are some common questions you might encounter:
- Explain the difference between abstraction and encapsulation.
- What is method overloading and method overriding? How do they differ?
- Explain the concept of multiple inheritance and its potential pitfalls.
- What is the difference between an interface and an abstract class?
- How does polymorphism work in practice? Can you provide an example?
- What is the ‘this’ keyword in Java (or ‘self’ in Python)? How is it used?
- Explain the concept of composition over inheritance.
- What are access modifiers and why are they important in OOP?
- How does garbage collection work in OOP languages like Java?
- What is the difference between shallow copy and deep copy?
Practical OOP Exercise: Implementing a Shape Hierarchy
Let’s work through a practical OOP exercise that you might encounter in a coding interview. We’ll implement a simple shape hierarchy in Python, demonstrating inheritance, polymorphism, and encapsulation.
import math
class Shape:
def __init__(self):
pass
def area(self):
pass
def perimeter(self):
pass
class Circle(Shape):
def __init__(self, radius):
super().__init__()
self.__radius = radius # Private attribute
def area(self):
return math.pi * self.__radius ** 2
def perimeter(self):
return 2 * math.pi * self.__radius
class Rectangle(Shape):
def __init__(self, length, width):
super().__init__()
self.__length = length # Private attribute
self.__width = width # Private attribute
def area(self):
return self.__length * self.__width
def perimeter(self):
return 2 * (self.__length + self.__width)
class Triangle(Shape):
def __init__(self, a, b, c):
super().__init__()
self.__a = a # Private attribute
self.__b = b # Private attribute
self.__c = c # Private attribute
def area(self):
# Heron's formula
s = (self.__a + self.__b + self.__c) / 2
return math.sqrt(s * (s - self.__a) * (s - self.__b) * (s - self.__c))
def perimeter(self):
return self.__a + self.__b + self.__c
# Usage
shapes = [Circle(5), Rectangle(4, 6), Triangle(3, 4, 5)]
for shape in shapes:
print(f"Area of {shape.__class__.__name__}: {shape.area():.2f}")
print(f"Perimeter of {shape.__class__.__name__}: {shape.perimeter():.2f}")
print()
This example demonstrates several key OOP concepts:
- Inheritance:
Circle
,Rectangle
, andTriangle
all inherit from the baseShape
class. - Polymorphism: We can call
area()
andperimeter()
on any shape object, and the correct method for that shape will be called. - Encapsulation: The attributes of each shape are private (denoted by double underscores), encapsulating the internal state of each object.
- Abstraction: The
Shape
class provides an abstract interface that all shapes must implement.
Best Practices for OOP in Interviews
When using OOP in coding interviews, keep these best practices in mind:
- Start with a clear class structure: Before diving into implementation, sketch out your class hierarchy and relationships between classes.
- Use meaningful names: Choose clear, descriptive names for your classes, methods, and attributes.
- Encapsulate data: Use private attributes and provide public methods to access and modify them when necessary.
- Follow the Single Responsibility Principle: Each class should have a single, well-defined responsibility.
- Leverage inheritance and interfaces: Use inheritance to reuse code and interfaces to define common behavior.
- Apply polymorphism: Design your classes to take advantage of polymorphic behavior where appropriate.
- Explain your design decisions: Be prepared to justify why you chose a particular class structure or design pattern.
Conclusion: Mastering OOP for Interview Success
Object-Oriented Programming is more than just a programming paradigm; it’s a powerful tool for designing and implementing complex software systems. As you prepare for coding interviews, especially for positions at top tech companies, mastering OOP principles and practices is essential.
By understanding encapsulation, abstraction, inheritance, and polymorphism, you’ll be well-equipped to tackle a wide range of programming challenges. More importantly, you’ll be able to design scalable, maintainable solutions that showcase your skills as a software developer.
Remember, the key to success in technical interviews isn’t just about knowing the syntax or memorizing algorithms. It’s about demonstrating your ability to think through problems, design efficient solutions, and write clean, organized code. OOP provides a framework for doing just that.
As you continue your journey in software development and interview preparation, make sure to practice implementing OOP concepts in your code regularly. Work on projects that allow you to design class hierarchies, use inheritance and polymorphism, and apply design patterns. The more you work with OOP, the more natural it will become, and the better prepared you’ll be to showcase your skills in your next technical interview.
Whether you’re aiming for a position at a FAANG company or any other tech firm, a strong foundation in OOP will serve you well throughout your career. So keep practicing, keep learning, and approach each coding challenge as an opportunity to refine your OOP skills. With dedication and practice, you’ll be well on your way to acing your technical interviews and building impressive, scalable software systems.