10 Beginner-Friendly Programming Projects to Kickstart Your Coding Journey
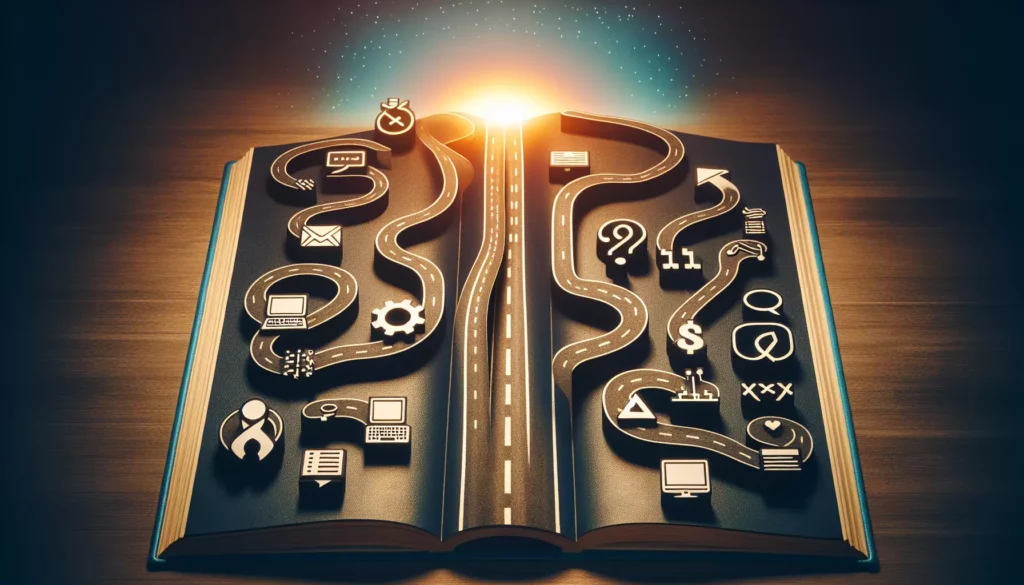
Are you new to programming and looking for some hands-on projects to hone your skills? You’re in the right place! As part of our mission at AlgoCademy to support coding education and programming skills development, we’ve compiled a list of 10 beginner-friendly programming projects that will help you apply your knowledge and build confidence in your coding abilities. These projects are designed to be accessible yet challenging, allowing you to grow as a programmer while creating something tangible.
Why Beginner Projects Matter
Before we dive into the project ideas, let’s briefly discuss why working on beginner projects is crucial for your development as a programmer:
- Practical Application: Projects allow you to apply theoretical knowledge in real-world scenarios.
- Problem-Solving Skills: You’ll encounter and overcome various challenges, improving your problem-solving abilities.
- Portfolio Building: Completed projects serve as excellent additions to your programming portfolio.
- Motivation: Seeing your ideas come to life can be incredibly motivating and encouraging.
- Learning New Concepts: Each project introduces you to new programming concepts and best practices.
Now, let’s explore 10 beginner-friendly programming projects that will help you build a strong foundation in coding.
1. To-Do List Application
A to-do list application is a classic beginner project that helps you understand the basics of user input, data storage, and simple CRUD (Create, Read, Update, Delete) operations.
Key Features:
- Add new tasks
- Mark tasks as complete
- Delete tasks
- View all tasks
Learning Opportunities:
- Working with arrays or lists
- Implementing basic user interfaces
- Handling user input
- File I/O for data persistence (optional)
Sample Code (Python):
class TodoList:
def __init__(self):
self.tasks = []
def add_task(self, task):
self.tasks.append({"task": task, "completed": False})
def mark_complete(self, index):
if 0 <= index < len(self.tasks):
self.tasks[index]["completed"] = True
def delete_task(self, index):
if 0 <= index < len(self.tasks):
del self.tasks[index]
def view_tasks(self):
for i, task in enumerate(self.tasks):
status = "✓" if task["completed"] else " "
print(f"{i}. [{status}] {task['task']}")
# Usage
todo_list = TodoList()
todo_list.add_task("Learn Python")
todo_list.add_task("Build a to-do list app")
todo_list.mark_complete(0)
todo_list.view_tasks()
2. Calculator
Building a calculator is an excellent way to practice working with user input, conditional statements, and basic arithmetic operations.
Key Features:
- Basic arithmetic operations (addition, subtraction, multiplication, division)
- User input for numbers and operations
- Display results
Learning Opportunities:
- Handling user input and type conversion
- Implementing mathematical operations
- Using conditional statements
- Error handling (e.g., division by zero)
Sample Code (JavaScript):
function calculate(num1, operator, num2) {
num1 = parseFloat(num1);
num2 = parseFloat(num2);
switch (operator) {
case '+':
return num1 + num2;
case '-':
return num1 - num2;
case '*':
return num1 * num2;
case '/':
if (num2 === 0) {
return "Error: Division by zero";
}
return num1 / num2;
default:
return "Error: Invalid operator";
}
}
// Usage
console.log(calculate(5, '+', 3)); // Output: 8
console.log(calculate(10, '/', 2)); // Output: 5
console.log(calculate(7, '*', 4)); // Output: 28
3. Guess the Number Game
This simple game helps you practice working with random number generation, user input, and conditional statements while creating an interactive experience.
Key Features:
- Generate a random number
- Accept user guesses
- Provide feedback (too high, too low, or correct)
- Keep track of the number of attempts
Learning Opportunities:
- Random number generation
- Loops and conditional statements
- User input validation
- Basic game logic
Sample Code (Java):
import java.util.Random;
import java.util.Scanner;
public class GuessTheNumber {
public static void main(String[] args) {
Random random = new Random();
int numberToGuess = random.nextInt(100) + 1;
int attempts = 0;
boolean hasGuessedCorrectly = false;
Scanner scanner = new Scanner(System.in);
System.out.println("Welcome to Guess the Number!");
System.out.println("I'm thinking of a number between 1 and 100.");
while (!hasGuessedCorrectly) {
System.out.print("Enter your guess: ");
int guess = scanner.nextInt();
attempts++;
if (guess < numberToGuess) {
System.out.println("Too low! Try again.");
} else if (guess > numberToGuess) {
System.out.println("Too high! Try again.");
} else {
hasGuessedCorrectly = true;
System.out.println("Congratulations! You've guessed the number in " + attempts + " attempts.");
}
}
scanner.close();
}
}
4. Password Generator
A password generator is a practical project that introduces you to string manipulation, randomization, and basic security concepts.
Key Features:
- Generate random passwords of specified length
- Include various character types (uppercase, lowercase, numbers, special characters)
- Allow user to specify password requirements
Learning Opportunities:
- Working with strings and characters
- Randomization techniques
- User input and validation
- Basic security considerations
Sample Code (Python):
import random
import string
def generate_password(length, use_uppercase, use_lowercase, use_numbers, use_special):
characters = ''
if use_uppercase:
characters += string.ascii_uppercase
if use_lowercase:
characters += string.ascii_lowercase
if use_numbers:
characters += string.digits
if use_special:
characters += string.punctuation
if not characters:
return "Error: No character types selected"
password = ''.join(random.choice(characters) for _ in range(length))
return password
# Usage
password = generate_password(12, True, True, True, True)
print(f"Generated Password: {password}")
5. Tic-Tac-Toe Game
Creating a Tic-Tac-Toe game helps you practice working with 2D arrays or lists, game logic, and user interaction.
Key Features:
- Display the game board
- Allow two players to take turns
- Check for win conditions
- Declare a winner or a draw
Learning Opportunities:
- Working with 2D data structures
- Implementing game logic
- Handling user input and validation
- Basic AI for a computer player (optional)
Sample Code (JavaScript):
class TicTacToe {
constructor() {
this.board = [
['', '', ''],
['', '', ''],
['', '', '']
];
this.currentPlayer = 'X';
}
makeMove(row, col) {
if (this.board[row][col] === '') {
this.board[row][col] = this.currentPlayer;
this.currentPlayer = this.currentPlayer === 'X' ? 'O' : 'X';
return true;
}
return false;
}
checkWin() {
// Check rows, columns, and diagonals
for (let i = 0; i < 3; i++) {
if (this.board[i][0] !== '' &&
this.board[i][0] === this.board[i][1] &&
this.board[i][1] === this.board[i][2]) {
return this.board[i][0];
}
if (this.board[0][i] !== '' &&
this.board[0][i] === this.board[1][i] &&
this.board[1][i] === this.board[2][i]) {
return this.board[0][i];
}
}
if (this.board[0][0] !== '' &&
this.board[0][0] === this.board[1][1] &&
this.board[1][1] === this.board[2][2]) {
return this.board[0][0];
}
if (this.board[0][2] !== '' &&
this.board[0][2] === this.board[1][1] &&
this.board[1][1] === this.board[2][0]) {
return this.board[0][2];
}
return null;
}
isBoardFull() {
return this.board.every(row => row.every(cell => cell !== ''));
}
printBoard() {
for (let row of this.board) {
console.log(row.join(' | '));
console.log('---------');
}
}
}
// Usage
let game = new TicTacToe();
game.makeMove(0, 0);
game.makeMove(1, 1);
game.makeMove(0, 1);
game.makeMove(2, 2);
game.makeMove(0, 2);
game.printBoard();
console.log(game.checkWin());
6. Weather App
Building a weather app introduces you to working with APIs, JSON data, and displaying information to users.
Key Features:
- Fetch weather data from an API
- Allow users to input a location
- Display current weather conditions
- Show a simple forecast (optional)
Learning Opportunities:
- Making API requests
- Parsing JSON data
- Handling asynchronous operations
- Basic error handling
Sample Code (Python with requests library):
import requests
def get_weather(city):
api_key = "YOUR_API_KEY_HERE"
base_url = "http://api.openweathermap.org/data/2.5/weather"
params = {
"q": city,
"appid": api_key,
"units": "metric"
}
response = requests.get(base_url, params=params)
if response.status_code == 200:
data = response.json()
temperature = data["main"]["temp"]
description = data["weather"][0]["description"]
return f"Weather in {city}: {temperature}°C, {description}"
else:
return "Error fetching weather data"
# Usage
city = input("Enter a city name: ")
print(get_weather(city))
7. URL Shortener
A URL shortener is a practical project that introduces you to working with databases, web frameworks, and URL manipulation.
Key Features:
- Accept a long URL as input
- Generate a short, unique code
- Store the mapping between short code and original URL
- Redirect users from short URL to original URL
Learning Opportunities:
- Basic web development concepts
- Working with databases (e.g., SQLite)
- URL handling and redirection
- Generating unique codes
Sample Code (Python with Flask and SQLite):
from flask import Flask, request, redirect
import sqlite3
import string
import random
app = Flask(__name__)
def get_db():
db = sqlite3.connect('urls.db')
db.row_factory = sqlite3.Row
return db
def init_db():
db = get_db()
db.execute('CREATE TABLE IF NOT EXISTS urls (id INTEGER PRIMARY KEY, original TEXT, short TEXT)')
db.close()
def generate_short_code():
characters = string.ascii_letters + string.digits
return ''.join(random.choice(characters) for _ in range(6))
@app.route('/', methods=['GET', 'POST'])
def index():
if request.method == 'POST':
original_url = request.form['url']
short_code = generate_short_code()
db = get_db()
db.execute('INSERT INTO urls (original, short) VALUES (?, ?)', (original_url, short_code))
db.commit()
db.close()
return f"Shortened URL: http://localhost:5000/{short_code}"
return '''
<form method="post">
URL to shorten: <input type="text" name="url">
<input type="submit" value="Shorten">
</form>
'''
@app.route('/<short_code>')
def redirect_to_url(short_code):
db = get_db()
result = db.execute('SELECT original FROM urls WHERE short = ?', (short_code,)).fetchone()
db.close()
if result:
return redirect(result['original'])
return "URL not found", 404
if __name__ == '__main__':
init_db()
app.run(debug=True)
8. Simple Blog System
Creating a basic blog system helps you understand CRUD operations, database interactions, and user authentication.
Key Features:
- User registration and login
- Create, read, update, and delete blog posts
- Display a list of blog posts
- Basic text formatting for blog content
Learning Opportunities:
- User authentication and authorization
- Database design and management
- Web form handling
- HTML templating
Sample Code (Python with Flask and SQLite):
from flask import Flask, request, render_template, redirect, session
import sqlite3
from werkzeug.security import generate_password_hash, check_password_hash
app = Flask(__name__)
app.secret_key = 'your_secret_key'
def get_db():
db = sqlite3.connect('blog.db')
db.row_factory = sqlite3.Row
return db
def init_db():
db = get_db()
db.execute('CREATE TABLE IF NOT EXISTS users (id INTEGER PRIMARY KEY, username TEXT, password TEXT)')
db.execute('CREATE TABLE IF NOT EXISTS posts (id INTEGER PRIMARY KEY, title TEXT, content TEXT, author_id INTEGER)')
db.close()
@app.route('/register', methods=['GET', 'POST'])
def register():
if request.method == 'POST':
username = request.form['username']
password = request.form['password']
db = get_db()
db.execute('INSERT INTO users (username, password) VALUES (?, ?)',
(username, generate_password_hash(password)))
db.commit()
db.close()
return redirect('/login')
return render_template('register.html')
@app.route('/login', methods=['GET', 'POST'])
def login():
if request.method == 'POST':
username = request.form['username']
password = request.form['password']
db = get_db()
user = db.execute('SELECT * FROM users WHERE username = ?', (username,)).fetchone()
db.close()
if user and check_password_hash(user['password'], password):
session['user_id'] = user['id']
return redirect('/')
return render_template('login.html')
@app.route('/')
def index():
db = get_db()
posts = db.execute('SELECT posts.*, users.username FROM posts JOIN users ON posts.author_id = users.id').fetchall()
db.close()
return render_template('index.html', posts=posts)
@app.route('/create', methods=['GET', 'POST'])
def create_post():
if 'user_id' not in session:
return redirect('/login')
if request.method == 'POST':
title = request.form['title']
content = request.form['content']
db = get_db()
db.execute('INSERT INTO posts (title, content, author_id) VALUES (?, ?, ?)',
(title, content, session['user_id']))
db.commit()
db.close()
return redirect('/')
return render_template('create_post.html')
if __name__ == '__main__':
init_db()
app.run(debug=True)
9. File Organizer
A file organizer script helps you practice working with file systems, automation, and data processing.
Key Features:
- Scan a directory for files
- Categorize files based on their extensions
- Move files to appropriate folders
- Generate a report of organized files
Learning Opportunities:
- File and directory manipulation
- Working with file extensions and types
- Error handling for file operations
- Generating reports or logs
Sample Code (Python):
import os
import shutil
from collections import defaultdict
def organize_files(source_dir):
file_types = defaultdict(list)
for filename in os.listdir(source_dir):
if os.path.isfile(os.path.join(source_dir, filename)):
file_ext = os.path.splitext(filename)[1].lower()
file_types[file_ext].append(filename)
for file_ext, files in file_types.items():
ext_dir = os.path.join(source_dir, file_ext[1:])
os.makedirs(ext_dir, exist_ok=True)
for file in files:
source_path = os.path.join(source_dir, file)
dest_path = os.path.join(ext_dir, file)
shutil.move(source_path, dest_path)
return dict(file_types)
def generate_report(organized_files):
report = "File Organization Report\n"
report += "========================\n\n"
for file_ext, files in organized_files.items():
report += f"{file_ext} files:\n"
for file in files:
report += f" - {file}\n"
report += "\n"
return report
# Usage
source_directory = "/path/to/your/directory"
organized = organize_files(source_directory)
report = generate_report(organized)
print(report)
with open("organization_report.txt", "w") as f:
f.write(report)
10. Memory Game
Creating a memory game helps you practice working with arrays, randomization, and game logic while building an interactive user interface.
Key Features:
- Generate a grid of cards with hidden symbols
- Allow users to flip cards
- Check for matches
- Keep track of score and attempts
Learning Opportunities:
- Working with 2D arrays
- Implementing game logic
- Randomization techniques
- Basic UI design and interaction
Sample Code (JavaScript):
class MemoryGame {
constructor(size) {
this.size = size;
this.board = [];
this.symbols = ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H'];
this.flippedCards = [];
this.matchedPairs = 0;
this.attempts = 0;
}
initializeBoard() {
let symbols = this.symbols.slice(0, this.size * this.size / 2);
symbols = symbols.concat(symbols);
symbols.sort(() => Math.random() - 0.5);
for (let i = 0; i < this.size; i++) {
this.board[i] = [];
for (let j = 0; j < this.size; j++) {
this.board[i][j] = {
symbol: symbols[i * this.size + j],
flipped: false
};
}
}
}
flipCard(row, col) {
if (this.flippedCards.length === 2) {
return false;
}
if (!this.board[row][col].flipped) {
this.board[row][col].flipped = true;
this.flippedCards.push({row, col});
if (this.flippedCards.length === 2) {
this.attempts++;
this.checkMatch();
}
return true;
}
return false;
}
checkMatch() {
const [card1, card2] = this.flippedCards;
if (this.board[card1.row][card1.col].symbol === this.board[card2.row][card2.col].symbol) {
this.matchedPairs++;
} else {
setTimeout(() => {
this.board[card1.row][card1.col].flipped = false;
this.board[card2.row][card2.col].flipped = false;
}, 1000);
}
this.flippedCards = [];
}
isGameOver() {
return this.matchedPairs === this.size * this.size / 2;
}
printBoard() {
for (let row of this.board) {
console.log(row.map(card => card.flipped ? card.symbol : '*').join(' '));
}
}
}
// Usage
let game = new MemoryGame(4);
game.initializeBoard();
game.printBoard();
// Simulate game play
game.flipCard(0, 0);
game.flipCard(1, 1);
game.printBoard();
console.log(`Attempts: ${game.attempts}`);
console.log(`Game Over: ${game.isGameOver()}`);
Conclusion
These 10 beginner-friendly programming projects offer a great starting point for new programmers to apply their knowledge and build practical skills. Each project focuses on different aspects of programming, from basic data structures and algorithms to user interfaces and game logic.
As you work through these projects, remember that the learning process is just as important as the final product. Don’t be afraid to experiment, make mistakes, and seek help when needed. The AlgoCademy platform provides additional resources, tutorials, and AI-powered assistance to support your learning journey.
Once you’ve completed these projects, you’ll have a solid foundation in programming concepts and be better prepared to tackle more complex challenges. Consider expanding on these projects by adding new features, improving the user interface, or optimizing the code for better performance.
Remember, consistent practice and hands-on experience are key to becoming a proficient programmer. Keep coding, stay curious, and enjoy the process of building your skills!