34 Proven Strategies to Boost Your Coding Speed and Efficiency
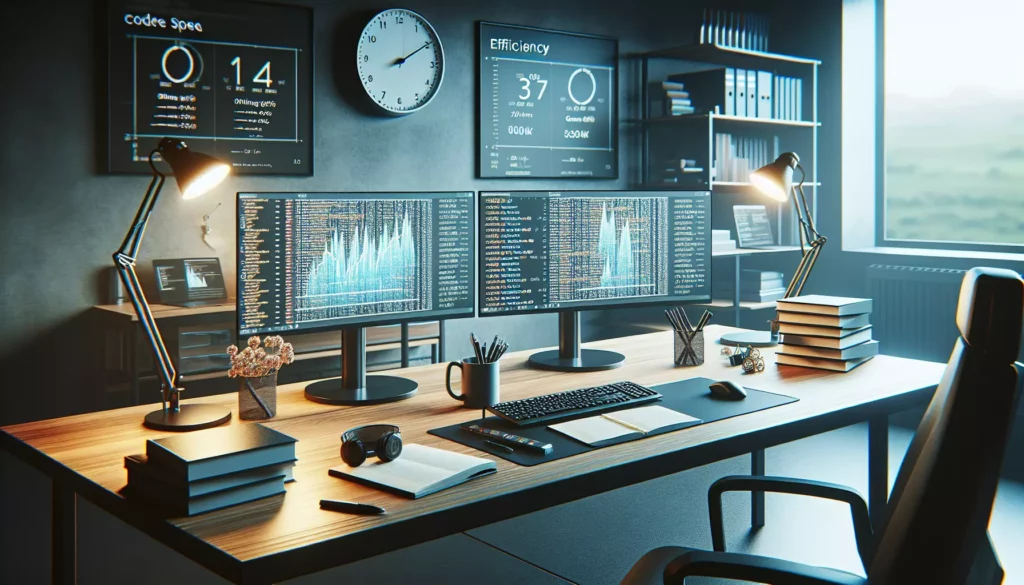
In the fast-paced world of software development, the ability to code quickly and efficiently is a highly sought-after skill. Whether you’re a beginner looking to improve your coding abilities or an experienced developer aiming to boost your productivity, this comprehensive guide will provide you with 34 practical strategies to enhance your coding speed and efficiency. By implementing these techniques, you’ll be well on your way to becoming a more proficient programmer and tackling complex coding challenges with ease.
1. Master Your Development Environment
One of the most fundamental ways to improve your coding speed is to become intimately familiar with your development environment. This includes your text editor or Integrated Development Environment (IDE), as well as any tools and plugins you use regularly.
Learn Keyboard Shortcuts
Keyboard shortcuts can significantly speed up your coding process by reducing the time spent navigating menus and performing repetitive tasks. Take the time to learn and memorize the most commonly used shortcuts for your preferred IDE or text editor. For example, in Visual Studio Code, some essential shortcuts include:
- Ctrl + C / Ctrl + V: Copy and paste
- Ctrl + F: Find
- Ctrl + H: Replace
- Ctrl + /: Toggle line comment
- Ctrl + D: Select next occurrence
Customize Your IDE
Most modern IDEs allow for extensive customization. Take advantage of this by setting up your development environment to suit your specific needs and preferences. This may include:
- Installing productivity-enhancing plugins or extensions
- Configuring code snippets for frequently used code blocks
- Setting up custom key bindings
- Choosing a color scheme and font that reduces eye strain
2. Embrace Version Control
Using a version control system like Git is essential for efficient coding, especially when working on larger projects or collaborating with others. Version control allows you to:
- Track changes to your code over time
- Experiment with new features without fear of breaking existing functionality
- Collaborate seamlessly with other developers
- Revert to previous versions if needed
Make sure to commit your changes frequently and write clear, descriptive commit messages. This practice will save you time in the long run by making it easier to understand the history of your codebase and locate specific changes when needed.
3. Utilize Code Snippets and Templates
Code snippets and templates can significantly speed up your coding process by allowing you to quickly insert commonly used code patterns. Most IDEs provide built-in snippet functionality, but you can also create your own custom snippets for frequently used code blocks.
For example, in Visual Studio Code, you can create a custom snippet for a basic HTML5 structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>${1:Document}</title>
</head>
<body>
${2}
</body>
</html>
By using snippets, you can quickly generate boilerplate code and focus on implementing the unique aspects of your project.
4. Practice Touch Typing
Improving your typing speed and accuracy can have a significant impact on your overall coding efficiency. Touch typing allows you to focus on the code itself rather than searching for keys on the keyboard. There are numerous online resources and tools available to help you improve your typing skills, such as:
- Typing.com
- 10FastFingers
- TypeRacer
Aim to practice regularly and gradually increase your typing speed and accuracy over time.
5. Learn and Use Keyboard-Centric Navigation
Reducing your reliance on the mouse can significantly speed up your coding process. Many IDEs and text editors offer powerful keyboard-based navigation features. For example:
- Use Ctrl + Arrow keys to move between words
- Use Home and End keys to jump to the beginning or end of a line
- Use Ctrl + Home and Ctrl + End to jump to the beginning or end of a file
- Use Ctrl + F to search within a file
By mastering these navigation techniques, you can move around your codebase more efficiently and reduce the time spent switching between keyboard and mouse.
6. Implement Code Formatting Tools
Consistent code formatting not only improves readability but also saves time in the long run. Instead of manually formatting your code, use automated tools to handle this task. Many IDEs have built-in formatting capabilities, or you can use standalone tools like:
- Prettier for JavaScript, CSS, and HTML
- Black for Python
- gofmt for Go
By automating code formatting, you can focus on writing code rather than worrying about indentation and style consistency.
7. Master Regular Expressions
Regular expressions (regex) are powerful tools for pattern matching and text manipulation. Learning to use regex effectively can significantly speed up tasks like searching, replacing, and validating text. For example, to match an email address, you might use the following regex pattern:
^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$
Invest time in learning regex syntax and practice using it in your projects. Many programming languages and text editors support regex, making it a versatile skill to have in your toolkit.
8. Leverage Code Generation Tools
Code generation tools can automate the creation of repetitive code structures, saving you time and reducing the likelihood of errors. Some examples include:
- ORM tools for generating database models and queries
- Swagger for generating API documentation and client libraries
- Protocol Buffers for generating serialization code
By using these tools, you can focus on implementing business logic rather than writing boilerplate code.
9. Implement Continuous Integration and Continuous Deployment (CI/CD)
CI/CD pipelines automate the process of testing, building, and deploying your code. By implementing CI/CD, you can:
- Catch errors early in the development process
- Ensure consistent code quality across your team
- Streamline the deployment process
- Reduce the time spent on manual testing and deployment tasks
Popular CI/CD tools include Jenkins, GitLab CI, and GitHub Actions.
10. Use Static Code Analysis Tools
Static code analysis tools can help you identify potential issues in your code before runtime. These tools can catch common programming errors, enforce coding standards, and suggest improvements. Some popular static analysis tools include:
- ESLint for JavaScript
- Pylint for Python
- SonarQube for multiple languages
By integrating these tools into your development workflow, you can catch and fix issues early, saving time in the debugging process.
11. Learn and Use Design Patterns
Design patterns are reusable solutions to common programming problems. By familiarizing yourself with established design patterns, you can:
- Solve complex problems more quickly
- Write more maintainable and scalable code
- Communicate more effectively with other developers
Some essential design patterns to learn include:
- Singleton
- Factory
- Observer
- Strategy
- Decorator
12. Implement Test-Driven Development (TDD)
Test-Driven Development is a software development approach where you write tests before implementing the actual code. While it may seem counterintuitive at first, TDD can lead to:
- Cleaner, more modular code
- Faster identification of bugs and issues
- Improved overall code quality
- Reduced time spent on debugging and maintenance
To implement TDD, follow these steps:
- Write a failing test for the desired functionality
- Implement the minimum code necessary to pass the test
- Refactor the code while ensuring all tests still pass
13. Master Your Programming Language
Becoming proficient in your primary programming language is crucial for coding efficiency. This includes understanding:
- Language-specific idioms and best practices
- Built-in functions and libraries
- Performance considerations and optimization techniques
Invest time in reading language documentation, studying well-written code, and practicing advanced language features.
14. Learn Multiple Programming Languages
While mastering one language is important, learning multiple languages can broaden your perspective and improve your problem-solving skills. Different languages often have unique approaches to solving problems, which can inspire more efficient solutions in your primary language.
Consider learning languages from different paradigms, such as:
- Object-oriented: Java, C++
- Functional: Haskell, Scala
- Dynamic: Python, Ruby
- Systems: Rust, Go
15. Use Code Refactoring Techniques
Refactoring is the process of restructuring existing code without changing its external behavior. Regular refactoring can lead to more maintainable and efficient code. Some common refactoring techniques include:
- Extracting methods or functions
- Renaming variables and functions for clarity
- Simplifying complex conditional statements
- Removing duplicate code
Many IDEs offer automated refactoring tools that can speed up this process.
16. Implement Code Reviews
Code reviews are an excellent way to improve code quality and share knowledge within a team. By reviewing others’ code and having your code reviewed, you can:
- Identify potential bugs or inefficiencies
- Learn new techniques and approaches
- Ensure consistency across the codebase
- Improve overall code quality
Implement a consistent code review process in your team or seek out code review opportunities in open-source projects.
17. Use Debugging Tools Effectively
Efficient debugging is crucial for maintaining coding speed. Familiarize yourself with your IDE’s debugging tools and features, such as:
- Breakpoints
- Step-through execution
- Watch variables
- Call stack analysis
Additionally, learn to use language-specific debugging tools and techniques, such as Python’s pdb or JavaScript’s console.log() statements.
18. Implement Error Handling and Logging
Proper error handling and logging can save significant time when debugging issues in production. Implement robust error handling mechanisms and use logging to capture relevant information about the application’s state and behavior.
For example, in Python, you might use a try-except block with logging:
import logging
logging.basicConfig(level=logging.INFO)
try:
# Some potentially risky operation
result = perform_operation()
except Exception as e:
logging.error(f"An error occurred: {str(e)}")
# Handle the error appropriately
else:
logging.info(f"Operation completed successfully: {result}")
19. Use Code Profiling Tools
Code profiling tools help identify performance bottlenecks in your code. By using profilers, you can:
- Identify slow functions or methods
- Analyze memory usage
- Optimize resource-intensive operations
Some popular profiling tools include:
- cProfile for Python
- Chrome DevTools for JavaScript
- VisualVM for Java
20. Implement Pair Programming
Pair programming involves two developers working together on the same code. This practice can lead to:
- Faster problem-solving
- Reduced errors and bugs
- Knowledge sharing and skill improvement
- Better code quality
Consider implementing pair programming sessions in your team or finding a coding partner to work with regularly.
21. Use Code Documentation Tools
Well-documented code is easier to understand and maintain. Use documentation tools to generate clear and concise documentation for your code. Some popular documentation tools include:
- Javadoc for Java
- Sphinx for Python
- JSDoc for JavaScript
Consistently documenting your code can save time when revisiting old projects or onboarding new team members.
22. Implement Code Metrics
Code metrics provide quantitative measures of your code’s complexity, maintainability, and quality. By tracking these metrics, you can identify areas for improvement and monitor your progress over time. Some useful code metrics include:
- Cyclomatic complexity
- Lines of code
- Code coverage
- Maintainability index
Tools like SonarQube and CodeClimate can help you track these metrics automatically.
23. Use Code Completion and Intelligent Code Suggestions
Modern IDEs offer powerful code completion and suggestion features. These can significantly speed up your coding process by:
- Automatically completing variable and function names
- Suggesting appropriate method calls and parameters
- Providing quick documentation and type information
Take the time to learn and configure these features in your IDE to maximize their benefits.
24. Implement Continuous Learning
The field of software development is constantly evolving. To maintain and improve your coding efficiency, it’s crucial to engage in continuous learning. Some ways to stay updated include:
- Reading technical blogs and articles
- Attending conferences and meetups
- Participating in online courses and tutorials
- Contributing to open-source projects
25. Use Code Visualization Tools
Code visualization tools can help you understand complex codebases more quickly. These tools generate visual representations of your code structure, dependencies, and relationships. Some popular code visualization tools include:
- Sourcetrail
- CodeSee
- Understand
By using these tools, you can gain a better understanding of large projects and identify areas for improvement more efficiently.
26. Implement Code Reuse and Modularization
Writing modular, reusable code can significantly improve your coding efficiency. By breaking your code into smaller, self-contained modules, you can:
- Reduce code duplication
- Improve maintainability
- Enhance testability
- Facilitate code reuse across projects
Focus on writing functions and classes that have a single responsibility and can be easily reused in different contexts.
27. Use Code Generation Libraries
Many programming languages have libraries that can generate code for common tasks. These libraries can save time and reduce errors when working with repetitive code structures. Some examples include:
- Lombok for Java: Reduces boilerplate code for getters, setters, and constructors
- AutoPep8 for Python: Automatically formats code to conform to PEP 8 style guide
- Yeoman for JavaScript: Scaffolds new projects and generates boilerplate code
28. Implement Time Management Techniques
Effective time management is crucial for improving coding efficiency. Consider implementing techniques such as:
- Pomodoro Technique: Work in focused 25-minute intervals with short breaks in between
- Time blocking: Allocate specific time slots for different tasks or projects
- Eisenhower Matrix: Prioritize tasks based on their importance and urgency
Experiment with different time management strategies to find what works best for you.
29. Use Code Complexity Analysis Tools
Code complexity analysis tools can help you identify overly complex parts of your codebase that may be difficult to maintain or prone to bugs. By addressing these areas, you can improve the overall quality and efficiency of your code. Some popular complexity analysis tools include:
- Radon for Python
- ESLint complexity rules for JavaScript
- SonarQube for multiple languages
30. Implement Code Reviews and Pull Requests
Regular code reviews and pull requests can help catch issues early and improve overall code quality. Implement a process where team members review each other’s code before merging changes. This practice can:
- Identify bugs and potential issues
- Ensure code consistency and adherence to best practices
- Facilitate knowledge sharing among team members
- Improve overall code quality
31. Use Code Search Tools
Efficient code search can save significant time when working on large codebases. Familiarize yourself with advanced search techniques in your IDE and consider using specialized code search tools like:
- Sourcegraph
- OpenGrok
- Ripgrep
These tools can help you quickly locate specific code snippets, functions, or usage examples across your entire codebase.
32. Implement Code Review Checklists
Develop and use code review checklists to ensure consistent and thorough reviews. A well-designed checklist can help reviewers focus on important aspects of the code, such as:
- Code style and formatting
- Error handling and edge cases
- Performance considerations
- Security best practices
- Documentation and comments
By using checklists, you can streamline the review process and improve the overall quality of your code.
33. Use Code Collaboration Tools
Effective collaboration is essential for efficient coding, especially when working in teams. Utilize collaboration tools to streamline communication and code sharing. Some popular options include:
- GitHub or GitLab for version control and code reviews
- Slack or Microsoft Teams for team communication
- Jira or Trello for project management and task tracking
- Confluence or Notion for documentation and knowledge sharing
34. Implement Continuous Performance Monitoring
Continuously monitoring your application’s performance can help you identify and address issues quickly, leading to more efficient coding and problem-solving. Implement tools and practices for ongoing performance monitoring, such as:
- Application Performance Management (APM) tools like New Relic or Datadog
- Real User Monitoring (RUM) for web applications
- Log analysis and alerting systems
- Regular performance testing and benchmarking
Conclusion
Improving your coding speed and efficiency is an ongoing process that requires dedication, practice, and the right tools and techniques. By implementing the strategies outlined in this guide, you can significantly enhance your productivity as a developer and tackle complex coding challenges with greater ease.
Remember that becoming a more efficient coder is not just about typing faster or using more shortcuts. It’s about developing a holistic approach to software development that encompasses everything from mastering your tools and languages to implementing best practices in code organization, testing, and collaboration.
As you work on improving your coding efficiency, keep in mind that consistency is key. Start by implementing a few of these strategies and gradually incorporate more as you become comfortable with them. Regularly assess your progress and be open to adjusting your approach based on what works best for you and your team.
By continuously refining your skills and embracing efficient coding practices, you’ll not only become a more productive developer but also contribute to creating higher-quality, more maintainable software. Happy coding!