The Crucial Role of Test Cases in Coding Interviews: A Comprehensive Guide
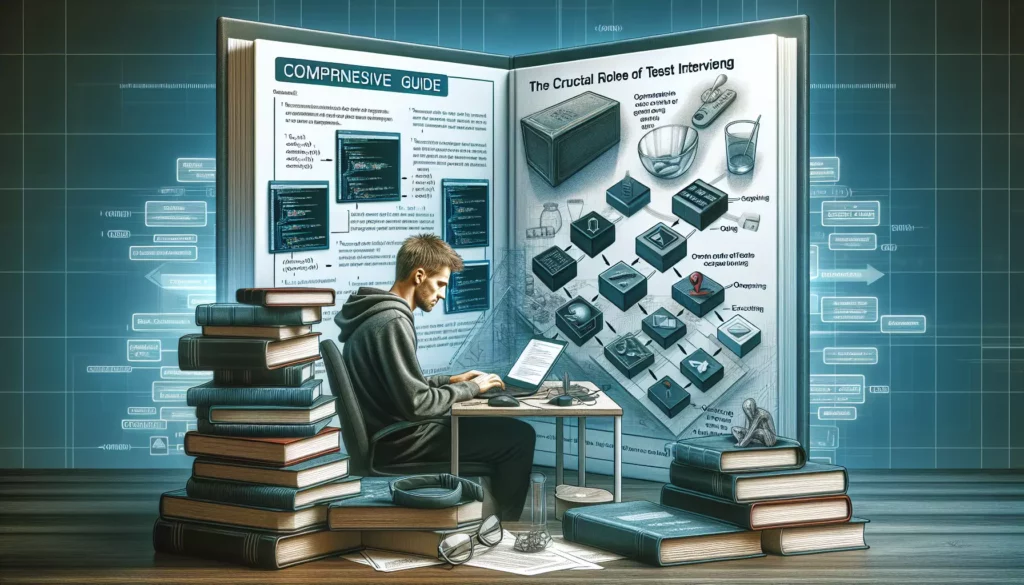
In the competitive landscape of tech industry recruitment, coding interviews have become a standard practice for evaluating candidates’ programming skills. Among the various components of these interviews, test cases play a pivotal role in assessing a candidate’s problem-solving abilities, attention to detail, and overall coding proficiency. This comprehensive guide will delve into the importance of test cases in coding interviews, exploring their significance, best practices, and how they contribute to both the interviewer’s evaluation process and the candidate’s success.
Understanding Test Cases in Coding Interviews
Before we dive into the importance of test cases, let’s first establish what they are in the context of coding interviews. Test cases are specific scenarios or inputs designed to verify the correctness and efficiency of a given solution to a programming problem. They serve as a way to validate that the code produces the expected output for various input conditions, including edge cases and potential pitfalls.
Types of Test Cases
In coding interviews, you may encounter several types of test cases:
- Basic Test Cases: These cover the standard, expected inputs and outputs for the problem.
- Edge Cases: These test the boundaries of the problem, such as minimum or maximum values, empty inputs, or unusual data types.
- Corner Cases: These are specific scenarios that might be easily overlooked but are crucial for the correctness of the solution.
- Performance Test Cases: These evaluate the efficiency of the solution, often using large inputs to test time and space complexity.
The Importance of Test Cases in Coding Interviews
Now that we’ve established what test cases are, let’s explore why they are so crucial in coding interviews:
1. Demonstrating Problem Understanding
One of the primary reasons test cases are important is that they showcase a candidate’s understanding of the problem at hand. By creating comprehensive test cases, you demonstrate that you’ve thought through various scenarios and potential issues that might arise. This critical thinking is highly valued by interviewers as it indicates a thorough approach to problem-solving.
2. Ensuring Code Correctness
Test cases serve as a verification mechanism for your code. They help you catch errors and edge cases that might not be immediately apparent. By running your solution through a variety of test cases, you can ensure that it works correctly for all possible inputs, not just the obvious ones.
3. Improving Code Quality
The process of creating and considering test cases often leads to improvements in code quality. As you think about different scenarios, you may identify areas where your code can be optimized or made more robust. This iterative process of testing and refining is a hallmark of good software development practices.
4. Showcasing Attention to Detail
Interviewers pay close attention to how candidates handle test cases. By thoroughly testing your code and considering various scenarios, you demonstrate a high level of attention to detail. This quality is highly valued in software development, where overlooking small details can lead to significant issues in production.
5. Facilitating Communication
Test cases provide a common ground for discussion between the interviewer and the candidate. They allow you to explain your thought process, discuss potential issues, and showcase your problem-solving approach. This communication is often as important as the code itself in evaluating a candidate’s fit for a role.
6. Simulating Real-World Scenarios
In actual software development, writing test cases is a crucial part of the development process. By emphasizing test cases in interviews, companies assess a candidate’s ability to think like a developer and consider the broader implications of their code beyond just solving the immediate problem.
Best Practices for Handling Test Cases in Coding Interviews
Now that we understand the importance of test cases, let’s look at some best practices for dealing with them effectively in coding interviews:
1. Start with Basic Test Cases
Begin by considering the most straightforward, expected inputs and outputs for the problem. These basic test cases help you verify that your solution works for the most common scenarios.
// Example: Function to add two numbers
function add(a, b) {
return a + b;
}
// Basic test cases
console.log(add(2, 3) === 5); // true
console.log(add(0, 0) === 0); // true
console.log(add(-1, 1) === 0); // true
2. Consider Edge Cases
Think about the boundaries of the problem. What happens with extremely large or small inputs? What about empty inputs or unusual data types? Edge cases often reveal hidden bugs in your code.
// Edge cases for the add function
console.log(add(Number.MAX_SAFE_INTEGER, 1) === Number.MAX_SAFE_INTEGER + 1); // true
console.log(add(Number.MIN_SAFE_INTEGER, -1) === Number.MIN_SAFE_INTEGER - 1); // true
console.log(add(0, -0) === 0); // true
3. Don’t Forget Corner Cases
Corner cases are specific scenarios that might be easily overlooked. For example, in a string manipulation problem, what happens with Unicode characters or strings with only whitespace?
// Corner case: Adding non-number types
console.log(add("2", 3) === 5); // false (returns "23" due to string concatenation)
console.log(add([], {}) === 0); // false (returns "[object Object]")
4. Test for Performance
For problems that involve large datasets or complex algorithms, consider how your solution performs with very large inputs. This demonstrates your understanding of time and space complexity.
// Performance test case
function generateLargeArray(size) {
return Array(size).fill().map(() => Math.floor(Math.random() * 1000));
}
const largeArray = generateLargeArray(1000000);
console.time("Large array sum");
const sum = largeArray.reduce((a, b) => add(a, b), 0);
console.timeEnd("Large array sum");
5. Write Test Cases Before Coding
A good practice is to write out some test cases before you start coding. This helps you clarify the problem requirements and gives you a clear target for what your code needs to accomplish.
6. Use Test-Driven Development (TDD) Approach
Consider using a TDD approach where you write tests first, then write code to pass those tests. This methodology can lead to more robust and well-thought-out solutions.
// TDD approach example
function reverseString(str) {
// Implementation to be written
}
// Write tests first
console.log(reverseString("hello") === "olleh"); // should be true
console.log(reverseString("") === ""); // should be true
console.log(reverseString("a") === "a"); // should be true
// Now implement the function to pass these tests
function reverseString(str) {
return str.split('').reverse().join('');
}
7. Verbalize Your Thought Process
As you consider and write test cases, explain your thinking to the interviewer. This gives them insight into your problem-solving approach and can lead to valuable discussions.
Common Pitfalls to Avoid
While working with test cases in coding interviews, be aware of these common pitfalls:
1. Overlooking Edge Cases
Many candidates focus solely on the happy path and forget to consider edge cases. Always think about extreme inputs, empty sets, and boundary conditions.
2. Ignoring Performance Considerations
Don’t just focus on correctness; also consider how your solution scales with larger inputs. A solution that works for small inputs might be impractical for large datasets.
3. Not Testing Incrementally
Test your code incrementally as you write it, rather than waiting until the end to run all tests. This makes debugging easier and helps you catch issues early.
4. Assuming Perfect Inputs
In real-world scenarios, inputs are often imperfect. Consider how your code handles unexpected inputs, such as null values or incorrect data types.
// Example: Handling unexpected inputs
function safeAdd(a, b) {
if (typeof a !== 'number' || typeof b !== 'number') {
throw new Error("Inputs must be numbers");
}
return a + b;
}
try {
console.log(safeAdd(2, "3")); // Throws error
} catch (e) {
console.log(e.message); // "Inputs must be numbers"
}
5. Not Considering Time Constraints
Remember that in an interview setting, you have limited time. While thorough testing is important, make sure you balance it with actually implementing the solution.
The Role of Test Cases in Different Types of Coding Problems
The importance and nature of test cases can vary depending on the type of coding problem you’re tackling. Let’s explore how test cases apply to different categories of problems commonly encountered in coding interviews:
1. Array and String Manipulation
For problems involving array or string manipulation, test cases should cover:
- Empty arrays/strings
- Single-element arrays/single-character strings
- Arrays/strings with repeated elements
- Very large arrays/strings (for performance testing)
// Example: Function to find the longest substring without repeating characters
function longestUniqueSubstring(s) {
// Implementation here
}
// Test cases
console.log(longestUniqueSubstring("") === ""); // Empty string
console.log(longestUniqueSubstring("a") === "a"); // Single character
console.log(longestUniqueSubstring("abcabcbb") === "abc"); // Normal case
console.log(longestUniqueSubstring("bbbbb") === "b"); // All repeating characters
console.log(longestUniqueSubstring("pwwkew") === "wke"); // Multiple valid substrings
2. Tree and Graph Problems
For tree and graph problems, consider test cases that include:
- Empty trees/graphs
- Single-node trees/graphs
- Balanced vs unbalanced trees
- Disconnected graphs
- Cyclic vs acyclic graphs
// Example: Function to find the depth of a binary tree
function maxDepth(root) {
if (!root) return 0;
return 1 + Math.max(maxDepth(root.left), maxDepth(root.right));
}
// Test cases
console.log(maxDepth(null) === 0); // Empty tree
console.log(maxDepth({val: 1, left: null, right: null}) === 1); // Single node
console.log(maxDepth({
val: 1,
left: {val: 2, left: null, right: null},
right: {val: 3, left: null, right: null}
}) === 2); // Balanced tree
console.log(maxDepth({
val: 1,
left: null,
right: {
val: 2,
right: {val: 3, right: null, left: null},
left: null
}
}) === 3); // Unbalanced tree
3. Dynamic Programming
For dynamic programming problems, test cases should focus on:
- Base cases (smallest possible inputs)
- Typical cases that require the full algorithm
- Large inputs to test memoization/tabulation efficiency
// Example: Fibonacci sequence with memoization
function fib(n, memo = {}) {
if (n in memo) return memo[n];
if (n <= 1) return n;
memo[n] = fib(n - 1, memo) + fib(n - 2, memo);
return memo[n];
}
// Test cases
console.log(fib(0) === 0); // Base case
console.log(fib(1) === 1); // Base case
console.log(fib(5) === 5); // Small case
console.log(fib(10) === 55); // Medium case
console.time("Large Fibonacci");
console.log(fib(100)); // Large case to test efficiency
console.timeEnd("Large Fibonacci");
4. Sorting and Searching Algorithms
For sorting and searching problems, consider:
- Already sorted arrays
- Reverse sorted arrays
- Arrays with all elements the same
- Very large arrays (for performance testing)
// Example: Binary search implementation
function binarySearch(arr, target) {
let left = 0, right = arr.length - 1;
while (left <= right) {
let mid = Math.floor((left + right) / 2);
if (arr[mid] === target) return mid;
if (arr[mid] < target) left = mid + 1;
else right = mid - 1;
}
return -1;
}
// Test cases
console.log(binarySearch([1, 2, 3, 4, 5], 3) === 2); // Normal case
console.log(binarySearch([1, 2, 3, 4, 5], 6) === -1); // Target not in array
console.log(binarySearch([1], 1) === 0); // Single element array
console.log(binarySearch([], 1) === -1); // Empty array
// Performance test
const largeArray = Array(1000000).fill().map((_, i) => i);
console.time("Large array search");
console.log(binarySearch(largeArray, 999999));
console.timeEnd("Large array search");
Leveraging Test Cases for Interview Success
Understanding the importance of test cases and how to effectively use them can significantly improve your performance in coding interviews. Here are some strategies to leverage test cases for interview success:
1. Use Test Cases to Clarify the Problem
At the beginning of the interview, use test cases to ensure you fully understand the problem. Ask the interviewer about specific scenarios to clarify any ambiguities.
2. Demonstrate Proactive Problem-Solving
By coming up with diverse test cases on your own, you show the interviewer that you’re proactive in considering different scenarios and potential issues.
3. Showcase Your Debugging Skills
If your initial solution fails certain test cases, use this as an opportunity to showcase your debugging skills. Walk the interviewer through your process of identifying and fixing the issue.
4. Highlight Your Knowledge of Edge Cases
Impress the interviewer by bringing up edge cases they might not have mentioned. This demonstrates your experience and foresight in software development.
5. Use Test Cases to Optimize Your Solution
As you work through different test cases, you may identify areas where your solution can be optimized. Use this to showcase your ability to iterate and improve your code.
6. Demonstrate Your Understanding of Code Efficiency
By considering performance test cases, you show that you’re thinking about the efficiency and scalability of your solutions, not just their correctness.
Conclusion
Test cases are an integral part of the coding interview process, serving multiple crucial functions. They help ensure the correctness and efficiency of your code, demonstrate your problem-solving skills and attention to detail, and provide a framework for discussing your solution with the interviewer.
By understanding the importance of test cases and following best practices in creating and using them, you can significantly enhance your performance in coding interviews. Remember to consider various types of test cases, including basic cases, edge cases, corner cases, and performance tests. Use them not just to verify your code, but also as a tool to clarify problems, showcase your thinking process, and demonstrate your understanding of real-world software development practices.
As you prepare for coding interviews, make working with test cases a key part of your practice routine. This will not only improve your problem-solving skills but also make you a more thorough and thoughtful programmer – qualities that are highly valued in the tech industry.
Ultimately, mastering the art of working with test cases will not only help you succeed in interviews but will also make you a better software developer in your professional career. So embrace the challenge, practice diligently, and let your expertise with test cases shine in your next coding interview!