How to Prepare for Coding Interviews at FAANG Companies: A Comprehensive Guide
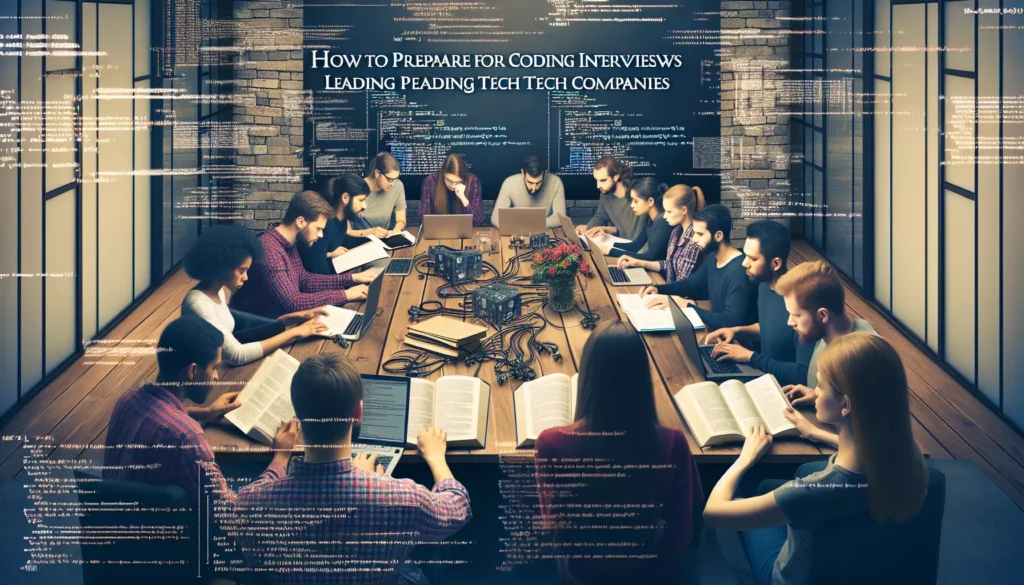
Preparing for coding interviews at FAANG (Facebook, Amazon, Apple, Netflix, Google) companies can be a daunting task. These tech giants are known for their rigorous interview processes and high standards. However, with the right approach and preparation, you can significantly increase your chances of success. In this comprehensive guide, we’ll explore the various aspects of FAANG interview preparation and provide you with actionable strategies to help you ace your coding interviews.
1. Understanding the FAANG Interview Process
Before diving into preparation strategies, it’s crucial to understand what to expect during the FAANG interview process. While each company may have slight variations, the general structure typically includes:
- Initial phone screen or online assessment
- One or more technical phone interviews
- On-site interviews (or virtual on-sites due to COVID-19)
- Behavioral interviews
- System design interviews (for more experienced candidates)
The coding interviews at FAANG companies are designed to assess your problem-solving skills, coding ability, and algorithmic thinking. They often involve solving complex problems on a whiteboard or in a shared coding environment.
2. Mastering Data Structures and Algorithms
A solid foundation in data structures and algorithms is crucial for success in FAANG coding interviews. Focus on the following key areas:
2.1 Essential Data Structures
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees, N-ary Trees)
- Graphs
- Hash Tables
- Heaps
2.2 Fundamental Algorithms
- Sorting (QuickSort, MergeSort, HeapSort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Backtracking
- Divide and Conquer
Make sure you not only understand these concepts theoretically but can also implement them efficiently in code.
3. Practice, Practice, Practice
Consistent practice is key to improving your problem-solving skills and coding speed. Here are some effective ways to practice:
3.1 Coding Platforms
Utilize online coding platforms to solve algorithmic problems regularly. Some popular options include:
- LeetCode
- HackerRank
- CodeSignal
- AlgoExpert
These platforms offer a wide range of problems, often categorized by difficulty and topic. Aim to solve at least 2-3 problems daily, focusing on medium and hard difficulty levels as you progress.
3.2 Mock Interviews
Participate in mock interviews to simulate the real interview experience. You can use platforms like:
- Pramp
- InterviewBit
- Interviewing.io
These platforms allow you to practice with peers or experienced interviewers, providing valuable feedback on your performance.
3.3 Time-Boxed Problem Solving
Set a timer when solving problems to mimic the time pressure of real interviews. Aim to solve medium difficulty problems within 20-30 minutes and hard problems within 45-60 minutes.
4. Develop a Problem-Solving Framework
Having a structured approach to problem-solving can help you tackle unfamiliar questions during interviews. Here’s a recommended framework:
- Understand the problem: Clarify any ambiguities and ask questions.
- Identify the inputs and outputs: Determine what you’re given and what you need to produce.
- Consider edge cases: Think about potential corner cases or extreme scenarios.
- Brainstorm solutions: Consider multiple approaches before settling on one.
- Explain your approach: Communicate your thought process clearly to the interviewer.
- Implement the solution: Write clean, efficient code.
- Test your solution: Walk through your code with sample inputs and edge cases.
- Analyze time and space complexity: Discuss the efficiency of your solution.
- Optimize if necessary: Consider ways to improve your initial solution.
Practice applying this framework to various problems to make it second nature during interviews.
5. Master Common Coding Patterns
Familiarize yourself with common coding patterns that frequently appear in FAANG interviews. Some essential patterns include:
- Two Pointers
- Sliding Window
- Fast and Slow Pointers
- Merge Intervals
- Cyclic Sort
- In-place Reversal of a Linked List
- Tree Breadth-First Search
- Tree Depth-First Search
- Two Heaps
- Subsets
- Modified Binary Search
- Top K Elements
- K-way Merge
- Topological Sort
Understanding these patterns can help you quickly identify the type of problem you’re dealing with and apply the appropriate solution strategy.
6. Improve Your Coding Skills
While problem-solving is crucial, your coding skills are equally important. Here are some tips to enhance your coding abilities:
6.1 Write Clean and Readable Code
Practice writing code that is easy to read and understand. Use meaningful variable names, proper indentation, and follow coding best practices. FAANG interviewers often evaluate code quality in addition to correctness.
6.2 Learn Multiple Programming Languages
While you should have a primary language of choice, being familiar with multiple languages can be beneficial. Python, Java, and C++ are commonly used in FAANG interviews. Here’s a simple example of reversing a string in Python:
def reverse_string(s):
return s[::-1]
# Example usage
input_string = "Hello, World!"
reversed_string = reverse_string(input_string)
print(reversed_string) # Output: !dlroW ,olleH
6.3 Implement Data Structures from Scratch
Practice implementing common data structures like linked lists, stacks, and binary trees from scratch. This deepens your understanding and prepares you for potential low-level design questions.
7. System Design Preparation
For more experienced candidates, system design interviews are often part of the FAANG interview process. To prepare for these:
- Study distributed systems concepts
- Learn about scalability, load balancing, and caching
- Understand database design and SQL vs. NoSQL trade-offs
- Practice designing popular systems (e.g., URL shortener, social media feed)
- Read about real-world system architectures of large-scale applications
Resources like “Designing Data-Intensive Applications” by Martin Kleppmann and “System Design Interview” by Alex Xu can be invaluable for this preparation.
8. Behavioral Interview Preparation
Don’t neglect the behavioral aspect of FAANG interviews. Prepare for questions about your past experiences, challenges you’ve faced, and how you’ve demonstrated leadership or teamwork. Use the STAR (Situation, Task, Action, Result) method to structure your responses.
9. Stay Updated with Company-Specific Information
Research the specific FAANG company you’re interviewing with. Understand their products, recent news, and company culture. This knowledge can help you tailor your responses and ask informed questions during the interview.
10. Develop a Study Schedule
Create a structured study plan to cover all aspects of interview preparation. Here’s a sample weekly schedule:
- Monday: Data Structures review and practice
- Tuesday: Algorithms review and practice
- Wednesday: Solve medium-level coding problems
- Thursday: System design study (if applicable)
- Friday: Solve hard-level coding problems
- Saturday: Mock interview or timed problem-solving session
- Sunday: Review and reflect on the week’s progress
Adjust this schedule based on your strengths, weaknesses, and available time.
11. Leverage Online Resources
Take advantage of the wealth of online resources available for FAANG interview preparation:
- YouTube channels like “Back To Back SWE” and “Tech Dose” for algorithm explanations
- Courses on platforms like Coursera, edX, or Udacity focusing on algorithms and data structures
- GitHub repositories with curated lists of interview preparation materials
- Coding interview books like “Cracking the Coding Interview” by Gayle Laakmann McDowell
12. Take Care of Your Mental and Physical Health
Preparing for FAANG interviews can be stressful. Remember to:
- Get enough sleep
- Exercise regularly
- Take breaks and practice mindfulness
- Maintain a balanced diet
- Stay connected with friends and family for support
A healthy mind and body will enhance your learning and performance during interviews.
13. Develop Your Soft Skills
While technical skills are crucial, soft skills play a significant role in FAANG interviews. Focus on improving:
- Communication: Practice explaining your thought process clearly
- Collaboration: Show your ability to work well in a team
- Adaptability: Demonstrate how you handle new challenges
- Problem-solving: Showcase your analytical thinking beyond just coding
14. Learn from Your Mistakes
After each practice session or mock interview, review your performance:
- Analyze the problems you couldn’t solve
- Understand the optimal solutions for problems you solved sub-optimally
- Keep a log of your mistakes and areas for improvement
- Regularly revisit problems you struggled with to reinforce your learning
15. Final Preparation Tips
As your interview date approaches:
- Review your strongest areas to boost confidence
- Prepare questions to ask your interviewers about the company and role
- Practice coding on a whiteboard or in a simple text editor
- Familiarize yourself with the interview platform if it’s a virtual interview
- Get a good night’s sleep before the interview day
Conclusion
Preparing for coding interviews at FAANG companies requires dedication, consistent practice, and a structured approach. By focusing on strengthening your fundamentals in data structures and algorithms, practicing regularly, and developing a solid problem-solving framework, you can significantly improve your chances of success.
Remember that the journey to landing a job at a FAANG company is as much about personal growth as it is about securing a position. The skills and knowledge you gain during this preparation process will benefit you throughout your career, regardless of the outcome of any single interview.
Stay persistent, embrace the learning process, and approach each problem as an opportunity to improve. With the right mindset and preparation, you can confidently tackle FAANG coding interviews and take a significant step towards your dream tech career.