Mastering Mock Interviews: Your Ultimate Guide to Technical Interview Preparation
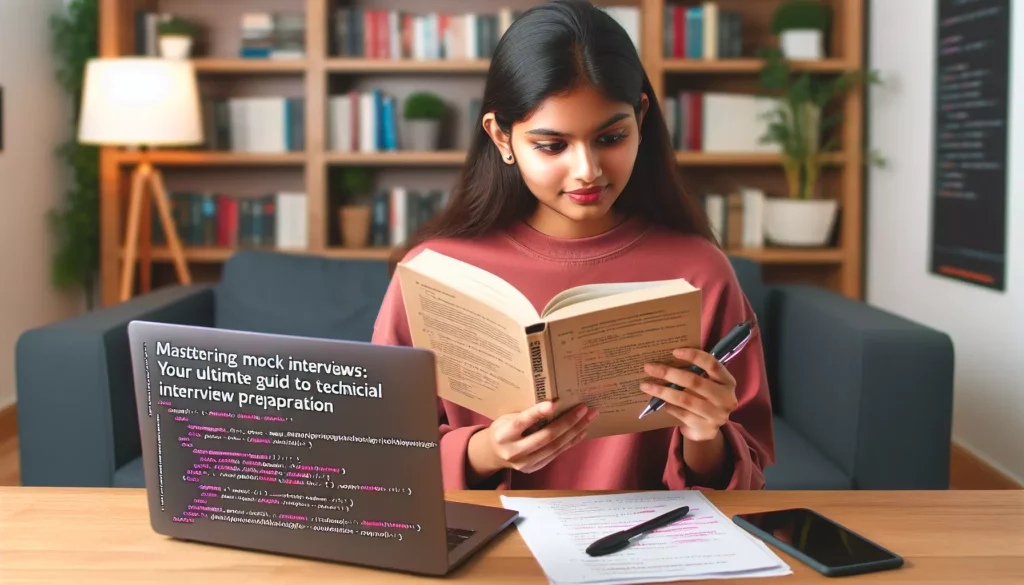
In the competitive world of software engineering, landing your dream job at a top tech company often comes down to your performance in technical interviews. Whether you’re aiming for a position at a FAANG company (Facebook, Amazon, Apple, Netflix, Google) or any other tech giant, mastering the art of the technical interview is crucial. One of the most effective ways to prepare for these high-stakes encounters is through mock interviews. In this comprehensive guide, we’ll explore how to effectively use mock interviews in your preparation, ensuring you’re ready to tackle any coding challenge that comes your way.
Why Mock Interviews Matter
Before diving into the strategies for effective mock interviews, it’s important to understand why they’re such a valuable tool in your preparation arsenal:
- Realistic Practice: Mock interviews simulate the actual interview environment, helping you get comfortable with the format and pressure.
- Skill Assessment: They provide an opportunity to identify your strengths and weaknesses in various areas of computer science and coding.
- Feedback Loop: You can receive immediate feedback on your performance, allowing you to improve rapidly.
- Confidence Building: Regular practice in mock interviews can boost your confidence for the real thing.
- Time Management: They help you develop a sense of timing, crucial for completing coding challenges within the allotted time frame.
Setting Up Effective Mock Interviews
To get the most out of your mock interview practice, consider the following steps:
1. Choose the Right Platform
There are several platforms available for conducting mock interviews:
- AlgoCademy: Offers AI-powered mock interviews tailored to your skill level and target companies.
- LeetCode: Provides a vast array of coding problems and a mock interview feature.
- Pramp: Pairs you with other candidates for peer-to-peer mock interviews.
- InterviewBit: Offers company-specific mock interviews and programming challenges.
2. Simulate Real Interview Conditions
To make your mock interviews as effective as possible:
- Use a whiteboard or a simple text editor instead of your usual IDE.
- Set a timer to mimic the time constraints of a real interview.
- Practice explaining your thought process out loud as you code.
- Dress professionally, as you would for an actual interview.
3. Cover a Wide Range of Topics
Ensure your mock interviews cover the full spectrum of topics you might encounter:
- Data Structures (Arrays, Linked Lists, Trees, Graphs, etc.)
- Algorithms (Sorting, Searching, Dynamic Programming, etc.)
- System Design
- Object-Oriented Programming
- Database Concepts
- Networking Fundamentals
Strategies for Effective Mock Interview Practice
Now that you’ve set up your mock interview environment, let’s explore strategies to maximize your learning:
1. Start with a Warm-Up
Before diving into complex problems, start each mock interview session with a simpler problem to get your mind in the right gear. This could be a basic string manipulation or array problem. For example:
def reverse_string(s):
return s[::-1]
# Test the function
print(reverse_string("hello")) # Output: olleh
2. Focus on Problem-Solving Approach
During mock interviews, pay special attention to your problem-solving approach:
- Understand the Problem: Clarify any ambiguities in the problem statement.
- Plan Your Approach: Outline your solution before coding.
- Implement the Solution: Write clean, efficient code.
- Test Your Solution: Consider edge cases and potential optimizations.
3. Practice Time Management
Allocate your time wisely during mock interviews:
- Spend 5-10 minutes understanding the problem and planning your approach.
- Allocate 20-30 minutes for implementation.
- Reserve 5-10 minutes for testing and optimization.
4. Verbalize Your Thought Process
Get comfortable explaining your thinking out loud. This is crucial for real interviews where interviewers want to understand your problem-solving approach. For example:
"To solve this problem, I'm thinking of using a hash map to store the frequency of each character. Then, I'll iterate through the string again to find the first character with a frequency of 1. Here's how I'd implement it:"
def first_unique_char(s):
char_count = {}
for char in s:
char_count[char] = char_count.get(char, 0) + 1
for i, char in enumerate(s):
if char_count[char] == 1:
return i
return -1
# Test the function
print(first_unique_char("leetcode")) # Output: 0
5. Embrace the Iterative Process
Don’t aim for perfection on the first try. Instead, focus on:
- Getting a working solution first, even if it’s not optimal.
- Iteratively improving your solution.
- Discussing potential optimizations, even if you don’t implement them all.
6. Practice Different Types of Questions
Vary your practice to cover different types of questions:
- Algorithmic Problems: Focus on efficiency and optimal solutions.
- Data Structure Implementation: Practice implementing and using various data structures.
- System Design Questions: Learn to discuss high-level architecture and trade-offs.
- Behavioral Questions: Prepare stories about your past projects and experiences.
Advanced Mock Interview Techniques
As you become more comfortable with basic mock interviews, consider these advanced techniques:
1. Peer Mock Interviews
Partner with a friend or colleague for mock interviews. This adds a layer of realism and helps you practice with different interviewing styles.
2. Record Your Sessions
Record your mock interviews (video if possible) to review later. This can help you identify areas for improvement in your communication and problem-solving approach.
3. Use AI-Powered Tools
Leverage AI tools like those offered by AlgoCademy to get personalized feedback and suggestions for improvement. These tools can analyze your code, problem-solving approach, and even your verbal explanations.
4. Practice Under Pressure
Simulate high-pressure situations by setting stricter time limits or adding distractions to your environment. This can help you stay calm and focused during real interviews.
5. Mock System Design Interviews
For more senior positions, practice system design interviews. These often involve open-ended questions about designing large-scale systems. For example:
"Design a URL shortening service like bit.ly"
Possible high-level approach:
1. Frontend: Simple web interface for users to input long URLs
2. Backend API:
- Receive long URL
- Generate unique short code
- Store mapping in database
3. Database:
- Key-Value store (e.g., Redis) for fast lookups
- Relational database for analytics and user data
4. URL Redirection Service:
- Receive short URL
- Look up original URL
- Redirect user
5. Analytics Service:
- Track clicks, user agents, etc.
6. Load Balancer:
- Distribute traffic across multiple servers
Key considerations:
- Scalability
- Reliability
- Security (prevent abuse)
- Performance (fast redirects)
Common Pitfalls to Avoid in Mock Interviews
As you practice with mock interviews, be aware of these common mistakes:
1. Neglecting Communication
Don’t fall into the trap of silently coding. Practice explaining your thought process clearly and concisely.
2. Ignoring Time Management
Always keep an eye on the clock. It’s better to have a working sub-optimal solution than an incomplete optimal one.
3. Overlooking Edge Cases
Always consider edge cases in your solutions. For example:
def divide(a, b):
if b == 0:
raise ValueError("Cannot divide by zero")
return a / b
# Test the function
print(divide(10, 2)) # Output: 5.0
try:
print(divide(10, 0))
except ValueError as e:
print(f"Error: {e}") # Output: Error: Cannot divide by zero
4. Forgetting to Test
Always test your code with sample inputs, including edge cases. This shows attention to detail and thoroughness.
5. Neglecting to Optimize
Even if your initial solution works, always consider ways to optimize it. Discuss time and space complexity.
Leveraging Mock Interview Feedback
The feedback you receive from mock interviews is gold. Here’s how to make the most of it:
1. Create a Feedback Log
Keep a detailed log of feedback from each mock interview. Include:
- The problem you were asked
- Your approach and solution
- Feedback received
- Areas for improvement
2. Identify Patterns
Look for recurring themes in your feedback. Are you consistently struggling with a particular type of problem or concept?
3. Targeted Practice
Use your feedback log to guide your further practice. Focus on areas where you need the most improvement.
4. Reflect and Adapt
Regularly review your progress. Are you seeing improvement in areas you’ve been focusing on? Adjust your study plan accordingly.
Integrating Mock Interviews into Your Overall Preparation Strategy
While mock interviews are incredibly valuable, they should be part of a comprehensive preparation strategy:
1. Balance Theory and Practice
Combine mock interviews with theoretical study. Understand the underlying principles of algorithms and data structures.
2. Use Varied Resources
Supplement mock interviews with:
- Coding challenges on platforms like LeetCode and HackerRank
- Textbooks and online courses for in-depth understanding
- Coding projects to build practical skills
3. Stay Updated
Keep abreast of current trends in software development and the specific technologies used by your target companies.
4. Practice Soft Skills
Don’t neglect behavioral interview preparation. Practice discussing your past projects, challenges you’ve overcome, and your motivations.
Conclusion: The Path to Interview Success
Effectively using mock interviews in your preparation can significantly boost your chances of success in technical interviews. By simulating real interview conditions, focusing on a problem-solving approach, managing your time effectively, and leveraging feedback, you can transform from a nervous candidate to a confident problem-solver.
Remember, the goal of mock interviews isn’t just to solve problems—it’s to develop the skills and mindset needed to tackle any challenge that comes your way. With consistent practice and a strategic approach to improvement, you’ll be well-prepared to shine in your next technical interview, whether it’s at a FAANG company or any other tech organization.
As you continue your journey in coding education and programming skills development, platforms like AlgoCademy can be invaluable resources. With their focus on interactive coding tutorials, AI-powered assistance, and step-by-step guidance, they can complement your mock interview practice and help you build the strong foundation in algorithmic thinking and problem-solving that top tech companies seek.
So, embrace the challenge, stay persistent, and remember that each mock interview is a step closer to your dream job in the tech industry. Happy coding, and best of luck in your interviews!