How to Handle Coding Interview Questions You Don’t Know the Answer To
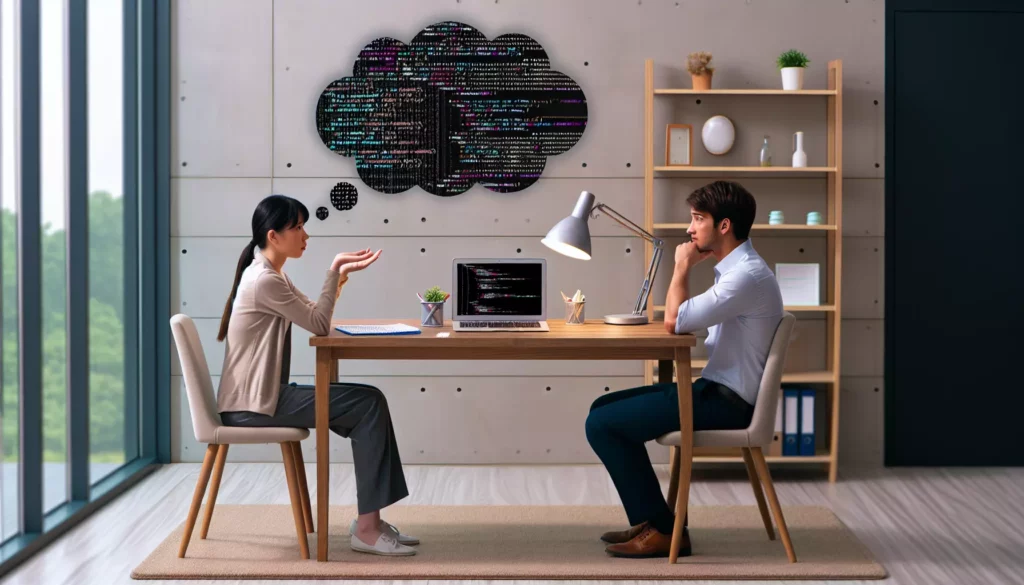
Coding interviews can be intimidating, especially when you encounter a question you’re not sure how to answer. However, how you handle these challenging moments can be just as important as knowing the solution. In this comprehensive guide, we’ll explore effective strategies for tackling unfamiliar coding interview questions, helping you navigate these situations with confidence and professionalism.
1. Stay Calm and Maintain a Positive Attitude
The first and most crucial step when faced with a difficult coding question is to remain calm. Remember, interviewers are not just evaluating your technical skills but also your ability to handle pressure and unfamiliar situations.
- Take a deep breath and remind yourself that it’s okay not to know everything.
- Maintain a positive attitude and view the challenge as an opportunity to learn and showcase your problem-solving skills.
- Avoid negative self-talk or outward expressions of frustration, as these can hinder your performance and leave a poor impression.
2. Clarify the Question
Before diving into a solution, make sure you fully understand the problem at hand. Don’t hesitate to ask for clarification – this shows that you’re thoughtful and detail-oriented.
- Repeat the question back to the interviewer to confirm your understanding.
- Ask about any constraints or specific requirements that might not be immediately obvious.
- Inquire about input formats, expected outputs, and any edge cases to consider.
For example, you might say:
“Just to make sure I understand correctly, we’re looking to implement a function that finds the kth largest element in an unsorted array. Are there any constraints on the array size or the types of elements it can contain?”
3. Think Aloud and Communicate Your Thought Process
One of the most valuable things you can do during a coding interview is to think aloud. This gives the interviewer insight into your problem-solving approach and allows them to provide guidance if needed.
- Verbalize your initial thoughts on potential approaches to the problem.
- Discuss any similar problems you’ve encountered and how they might relate.
- Explain your reasoning as you consider different algorithms or data structures.
For instance:
“This problem reminds me of sorting algorithms. We could potentially use a quicksort approach to partition the array and find the kth largest element. Alternatively, we might consider using a heap data structure for more efficient selection.”
4. Break Down the Problem
When faced with a complex problem, it’s often helpful to break it down into smaller, more manageable components. This approach can make the problem less overwhelming and help you identify parts you do know how to solve.
- Identify the main steps or subproblems within the larger problem.
- Focus on solving one component at a time.
- Discuss how these individual parts might fit together to form a complete solution.
For example, if asked to implement a basic web crawler, you might break it down like this:
- Function to fetch and parse HTML content from a given URL
- Function to extract links from parsed HTML
- Data structure to store visited URLs and manage the crawl frontier
- Main crawling loop to process URLs and respect crawling policies
5. Start with a Brute Force Approach
If you’re unsure of an optimal solution, begin with a brute force approach. This demonstrates your ability to devise a working solution, even if it’s not the most efficient.
- Explain that you’re starting with a simple solution to establish a baseline.
- Discuss the time and space complexity of your brute force approach.
- Mention that you’ll look for optimizations after implementing the initial solution.
Here’s an example of how you might communicate this:
“To start, I’ll implement a simple brute force solution that checks all possible combinations. This will have a time complexity of O(n^2), which isn’t optimal, but it will give us a working solution. Once we have this in place, we can look at ways to optimize it.”
6. Use Pseudocode to Outline Your Approach
Before diving into actual code, it can be helpful to outline your approach using pseudocode. This allows you to focus on the logic and structure of your solution without getting bogged down in syntax details.
- Write high-level pseudocode to sketch out your algorithm.
- Discuss the main steps and logic flow of your solution.
- Use this as an opportunity to catch any logical errors before implementation.
Here’s an example of pseudocode for finding the maximum subarray sum:
function maxSubarraySum(array):
maxSum = -infinity
currentSum = 0
for each element in array:
currentSum = max(element, currentSum + element)
maxSum = max(maxSum, currentSum)
return maxSum
7. Draw Diagrams or Visualizations
Visual representations can be incredibly helpful when explaining your thought process or understanding complex problems. Don’t hesitate to use the whiteboard or a piece of paper to draw diagrams.
- Sketch out data structures like trees, graphs, or arrays.
- Use flowcharts to illustrate the logic flow of your algorithm.
- Demonstrate how your solution works with a small example input.
For instance, when explaining a binary search tree insertion, you might draw the tree structure and show how it changes as new elements are inserted.
8. Leverage Your Existing Knowledge
Even if you don’t know the exact solution to a problem, you can often apply principles or techniques from similar problems you’ve encountered before.
- Identify patterns or similarities with known algorithms or data structures.
- Discuss how you might adapt solutions from related problems.
- Apply general problem-solving strategies like divide-and-conquer or dynamic programming.
For example:
“This problem seems similar to the longest common subsequence problem we covered in dynamic programming. We might be able to adapt that approach by modifying the state definition and transition function.”
9. Ask for Hints or Guidance
If you’re truly stuck, it’s okay to ask for a hint or some guidance. This shows that you’re proactive and willing to learn.
- Be specific about where you’re struggling or what aspect you need help with.
- Ask if there are any specific data structures or algorithms that might be particularly useful for this problem.
- Inquire about any assumptions you can make or constraints you should consider.
You might phrase your request like this:
“I’m considering using a hash table to optimize the lookup process, but I’m not sure if that’s the best approach here. Do you have any suggestions on what data structure might be most appropriate for this problem?”
10. Practice Implementation in Small Steps
Once you have a general idea of your approach, start implementing your solution in small, manageable steps. This incremental approach allows you to make progress and potentially uncover insights as you go.
- Begin with the core functionality and add complexity gradually.
- Test each component as you implement it to catch errors early.
- Refactor and optimize your code as you progress.
For example, if implementing a sorting algorithm:
- Start with a function that can swap two elements in an array.
- Implement the partitioning logic for quicksort.
- Write the main quicksort function using recursion.
- Add optimizations like choosing a good pivot or handling small subarrays differently.
11. Analyze Time and Space Complexity
Even if your solution isn’t optimal, demonstrating that you understand its efficiency is crucial. Analyze and discuss the time and space complexity of your approach.
- Identify the dominant operations in your algorithm.
- Consider best-case, average-case, and worst-case scenarios.
- Discuss any trade-offs between time and space complexity in your solution.
For instance:
“The current solution has a time complexity of O(n log n) due to the sorting step, and a space complexity of O(1) since we’re sorting in-place. We could potentially improve the time complexity to O(n) on average by using a different approach, such as the quickselect algorithm, but this would come at the cost of worse worst-case performance.”
12. Propose Optimizations and Alternatives
After implementing a basic solution, discuss potential optimizations or alternative approaches. This demonstrates your ability to critically evaluate and improve upon your work.
- Consider ways to reduce time or space complexity.
- Discuss trade-offs between different approaches.
- Mention any advanced data structures or algorithms that could be applied.
You might say something like:
“Now that we have a working solution using a hash map, we could potentially optimize this further by using a trie data structure. This would reduce our space complexity in cases where we have many strings with common prefixes, although it might slightly increase the implementation complexity.”
13. Test Your Solution
Once you have a working solution, it’s important to test it thoroughly. This shows attention to detail and a commitment to producing reliable code.
- Start with simple test cases to verify basic functionality.
- Consider edge cases and boundary conditions.
- Discuss how you would approach more comprehensive testing in a real-world scenario.
For example:
// Test cases for a function that finds the maximum subarray sum
assert(maxSubarraySum([1, -3, 2, 1, -1]) == 3);
assert(maxSubarraySum([-2, -3, 4, -1, -2, 1, 5, -3]) == 7);
assert(maxSubarraySum([-1, -2, -3]) == -1);
assert(maxSubarraySum([]) == 0); // Assuming 0 for empty array
14. Reflect on the Problem and Your Approach
After completing the problem (or making significant progress), take a moment to reflect on the experience. This demonstrates your ability to learn and improve.
- Discuss what you learned from the problem.
- Identify areas where you could improve or study further.
- Ask the interviewer for feedback or insights on alternative approaches.
You might conclude with something like:
“This problem really highlighted the importance of considering space-time trade-offs. I realize I could benefit from more practice with dynamic programming techniques. Are there any particular resources or problem sets you’d recommend for improving in this area?”
15. Stay Engaged and Professional Throughout
Remember that the interview process is not just about finding the correct answer, but also about assessing your problem-solving skills, communication abilities, and how you handle challenges.
- Maintain a positive and professional demeanor, even if you’re struggling.
- Show enthusiasm for learning and tackling difficult problems.
- Be receptive to feedback and willing to iterate on your solutions.
Conclusion
Encountering unfamiliar questions in a coding interview is a common experience, even for seasoned developers. By following these strategies, you can navigate these challenges effectively, demonstrating your problem-solving skills, adaptability, and professionalism.
Remember, interviewers are often more interested in your approach and thought process than in your ability to immediately produce a perfect solution. By staying calm, communicating clearly, and systematically working through the problem, you can turn a challenging question into an opportunity to showcase your strengths as a developer.
Continuous practice and exposure to a wide range of programming problems will help build your confidence and expand your problem-solving toolkit. Platforms like AlgoCademy offer a wealth of resources, including interactive coding tutorials, AI-powered assistance, and a vast array of practice problems to help you prepare for technical interviews and improve your coding skills.
With dedication, practice, and the right mindset, you can approach even the most challenging coding interview questions with confidence and skill. Remember, every difficult question is an opportunity to learn and grow as a programmer.