Top 10 Most Common Coding Interview Questions: Ace Your Technical Interview
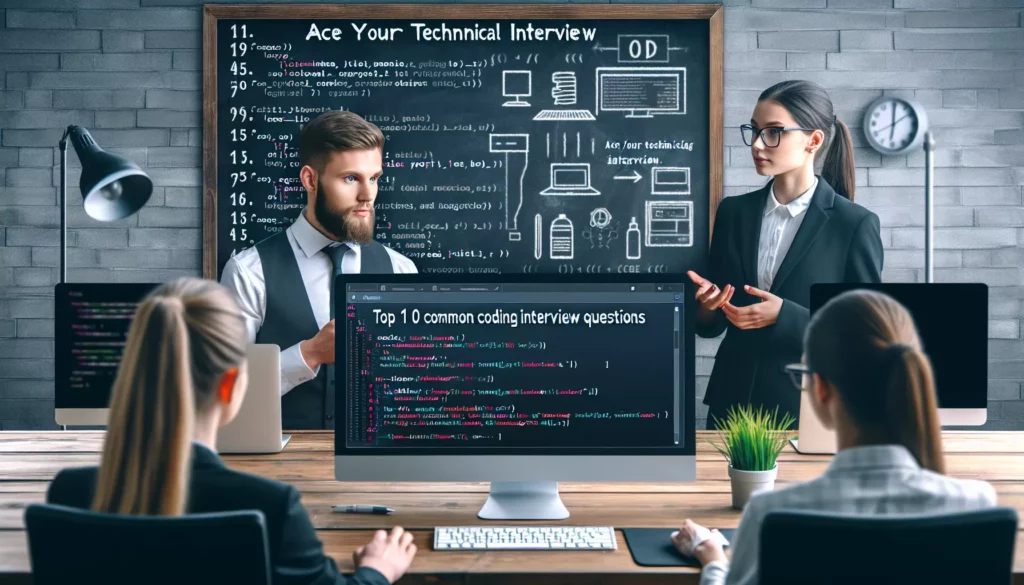
Are you preparing for a coding interview with a top tech company? Whether you’re aiming for a position at a FAANG (Facebook, Amazon, Apple, Netflix, Google) company or any other tech giant, it’s crucial to be well-prepared for the technical interview process. In this comprehensive guide, we’ll explore the most common coding interview questions that you’re likely to encounter, along with strategies to tackle them effectively.
Why Coding Interviews Matter
Before we dive into the specific questions, it’s important to understand why coding interviews are such a critical part of the hiring process for tech companies. These interviews serve several purposes:
- Assess your problem-solving skills
- Evaluate your coding proficiency
- Gauge your ability to think algorithmically
- Determine how well you can communicate technical concepts
- Test your ability to work under pressure
By mastering these common coding interview questions, you’ll not only increase your chances of landing your dream job but also improve your overall programming skills.
The Top 10 Most Common Coding Interview Questions
Let’s explore the ten most frequently asked coding interview questions, along with explanations and sample solutions. Remember, it’s not just about memorizing the answers – understanding the underlying concepts and problem-solving approaches is key.
1. Two Sum
Problem: Given an array of integers and a target sum, return the indices of two numbers in the array that add up to the target sum.
Example:
Input: nums = [2, 7, 11, 15], target = 9
Output: [0, 1]
Explanation: nums[0] + nums[1] = 2 + 7 = 9
Solution: This problem can be solved efficiently using a hash table.
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
Time Complexity: O(n)
Space Complexity: O(n)
2. Reverse a Linked List
Problem: Given the head of a singly linked list, reverse the list and return the new head.
Example:
Input: 1 -> 2 -> 3 -> 4 -> 5
Output: 5 -> 4 -> 3 -> 2 -> 1
Solution: This can be solved using an iterative approach with three pointers.
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def reverse_linked_list(head):
prev = None
current = head
while current:
next_node = current.next
current.next = prev
prev = current
current = next_node
return prev
Time Complexity: O(n)
Space Complexity: O(1)
3. Valid Parentheses
Problem: Given a string containing just the characters ‘(‘, ‘)’, ‘{‘, ‘}’, ‘[‘ and ‘]’, determine if the input string is valid. An input string is valid if:
- Open brackets must be closed by the same type of brackets.
- Open brackets must be closed in the correct order.
Example:
Input: "()[]{}"
Output: true
Input: "([)]"
Output: false
Solution: This problem can be solved using a stack.
def is_valid_parentheses(s):
stack = []
mapping = {")": "(", "}": "{", "]": "["}
for char in s:
if char in mapping:
if not stack or stack[-1] != mapping[char]:
return False
stack.pop()
else:
stack.append(char)
return len(stack) == 0
Time Complexity: O(n)
Space Complexity: O(n)
4. Merge Two Sorted Lists
Problem: Merge two sorted linked lists and return it as a new sorted list. The new list should be made by splicing together the nodes of the first two lists.
Example:
Input: l1 = 1->2->4, l2 = 1->3->4
Output: 1->1->2->3->4->4
Solution: This can be solved using a dummy node and iterating through both lists.
class ListNode:
def __init__(self, val=0, next=None):
self.val = val
self.next = next
def merge_two_lists(l1, l2):
dummy = ListNode(0)
current = dummy
while l1 and l2:
if l1.val < l2.val:
current.next = l1
l1 = l1.next
else:
current.next = l2
l2 = l2.next
current = current.next
current.next = l1 if l1 else l2
return dummy.next
Time Complexity: O(n + m), where n and m are the lengths of the input lists
Space Complexity: O(1)
5. Maximum Subarray
Problem: Given an integer array nums, find the contiguous subarray (containing at least one number) which has the largest sum and return its sum.
Example:
Input: nums = [-2,1,-3,4,-1,2,1,-5,4]
Output: 6
Explanation: [4,-1,2,1] has the largest sum = 6.
Solution: This problem can be solved using Kadane’s algorithm.
def max_subarray(nums):
max_sum = current_sum = nums[0]
for num in nums[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
Time Complexity: O(n)
Space Complexity: O(1)
6. Best Time to Buy and Sell Stock
Problem: You are given an array prices where prices[i] is the price of a given stock on the ith day. You want to maximize your profit by choosing a single day to buy one stock and choosing a different day in the future to sell that stock. Return the maximum profit you can achieve from this transaction. If you cannot achieve any profit, return 0.
Example:
Input: prices = [7,1,5,3,6,4]
Output: 5
Explanation: Buy on day 2 (price = 1) and sell on day 5 (price = 6), profit = 6-1 = 5.
Solution: This can be solved by keeping track of the minimum price and maximum profit.
def max_profit(prices):
if not prices:
return 0
min_price = float('inf')
max_profit = 0
for price in prices:
min_price = min(min_price, price)
current_profit = price - min_price
max_profit = max(max_profit, current_profit)
return max_profit
Time Complexity: O(n)
Space Complexity: O(1)
7. Climbing Stairs
Problem: You are climbing a staircase. It takes n steps to reach the top. Each time you can either climb 1 or 2 steps. In how many distinct ways can you climb to the top?
Example:
Input: n = 3
Output: 3
Explanation: There are three ways to climb to the top.
1. 1 step + 1 step + 1 step
2. 1 step + 2 steps
3. 2 steps + 1 step
Solution: This problem can be solved using dynamic programming.
def climb_stairs(n):
if n <= 2:
return n
dp = [0] * (n + 1)
dp[1] = 1
dp[2] = 2
for i in range(3, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
Time Complexity: O(n)
Space Complexity: O(n)
8. Invert Binary Tree
Problem: Invert a binary tree.
Example:
Input:
4
/ \
2 7
/ \ / \
1 3 6 9
Output:
4
/ \
7 2
/ \ / \
9 6 3 1
Solution: This can be solved recursively.
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def invert_tree(root):
if not root:
return None
root.left, root.right = invert_tree(root.right), invert_tree(root.left)
return root
Time Complexity: O(n)
Space Complexity: O(h), where h is the height of the tree
9. Longest Palindromic Substring
Problem: Given a string s, return the longest palindromic substring in s.
Example:
Input: s = "babad"
Output: "bab"
Note: "aba" is also a valid answer.
Solution: This can be solved using dynamic programming or the expand around center approach.
def longest_palindrome(s):
if not s:
return ""
start = 0
max_length = 1
def expand_around_center(left, right):
while left >= 0 and right < len(s) and s[left] == s[right]:
left -= 1
right += 1
return right - left - 1
for i in range(len(s)):
len1 = expand_around_center(i, i)
len2 = expand_around_center(i, i + 1)
length = max(len1, len2)
if length > max_length:
start = i - (length - 1) // 2
max_length = length
return s[start:start + max_length]
Time Complexity: O(n^2)
Space Complexity: O(1)
10. Implement a Trie (Prefix Tree)
Problem: Implement a trie with insert, search, and startsWith methods.
Example:
Trie trie = new Trie();
trie.insert("apple");
trie.search("apple"); // returns true
trie.search("app"); // returns false
trie.startsWith("app"); // returns true
trie.insert("app");
trie.search("app"); // returns true
Solution: This can be implemented using a TrieNode class.
class TrieNode:
def __init__(self):
self.children = {}
self.is_end_of_word = False
class Trie:
def __init__(self):
self.root = TrieNode()
def insert(self, word):
node = self.root
for char in word:
if char not in node.children:
node.children[char] = TrieNode()
node = node.children[char]
node.is_end_of_word = True
def search(self, word):
node = self.root
for char in word:
if char not in node.children:
return False
node = node.children[char]
return node.is_end_of_word
def startsWith(self, prefix):
node = self.root
for char in prefix:
if char not in node.children:
return False
node = node.children[char]
return True
Time Complexity: O(m) for all operations, where m is the length of the key
Space Complexity: O(m) for insert, O(1) for search and startsWith
Tips for Mastering Coding Interviews
Now that we’ve covered the most common coding interview questions, here are some additional tips to help you excel in your technical interviews:
- Practice regularly: Consistent practice is key to improving your problem-solving skills. Aim to solve at least one coding problem every day.
- Understand the problem: Before diving into coding, make sure you fully understand the problem statement. Ask clarifying questions if needed.
- Think out loud: Communicate your thought process as you work through the problem. This gives the interviewer insight into your problem-solving approach.
- Consider edge cases: Always think about potential edge cases and how your solution handles them.
- Optimize your solution: After implementing a working solution, consider ways to optimize it for better time or space complexity.
- Learn from your mistakes: Review your solutions and learn from any mistakes you make. Understanding why a particular approach works or doesn’t work is crucial for improvement.
- Study data structures and algorithms: Having a solid foundation in data structures and algorithms will help you tackle a wide range of problems more effectively.
- Mock interviews: Practice with mock interviews to simulate the pressure of a real interview situation.
- Review your code: After writing your solution, take a moment to review and refactor your code for clarity and efficiency.
- Stay calm: Remember that interviewers are often more interested in your problem-solving process than in whether you get the perfect solution immediately.
Conclusion
Mastering these common coding interview questions is an excellent way to prepare for technical interviews at top tech companies. However, remember that these are just a starting point. The key to success in coding interviews lies in developing strong problem-solving skills, a deep understanding of data structures and algorithms, and the ability to communicate your thoughts effectively.
As you prepare for your interviews, consider using platforms like AlgoCademy that offer interactive coding tutorials, AI-powered assistance, and step-by-step guidance. These resources can help you progress from beginner-level coding to confidently tackling technical interviews at major tech companies.
Keep practicing, stay curious, and approach each problem as an opportunity to learn and grow. With dedication and the right preparation, you’ll be well-equipped to ace your coding interviews and land your dream job in the tech industry. Good luck!