Top Programming Languages to Master for Coding Interviews: A Comprehensive Guide
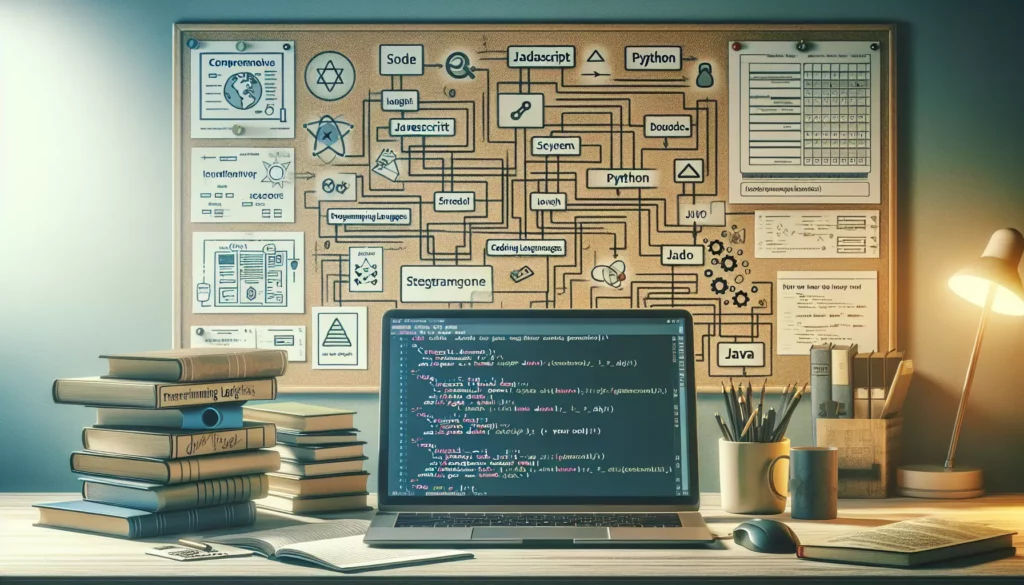
Are you gearing up for coding interviews and wondering which programming languages to focus on? You’re not alone. With the tech industry constantly evolving, it’s crucial to choose the right languages that will not only impress interviewers but also set you up for success in your future career. In this comprehensive guide, we’ll explore the most in-demand programming languages for coding interviews, their strengths, and why they’re essential for aspiring developers.
Table of Contents
- Why Your Choice of Programming Language Matters
- Top Programming Languages for Coding Interviews
- Language-Specific Tips for Coding Interviews
- Beyond Languages: What Else Matters in Coding Interviews
- Preparing for Coding Interviews with AlgoCademy
- Conclusion
Why Your Choice of Programming Language Matters
Before diving into specific languages, it’s important to understand why your choice of programming language for coding interviews is significant. While many companies allow candidates to use their preferred language, your selection can impact several factors:
- Problem-solving efficiency: Some languages have built-in features or libraries that make certain problems easier to solve.
- Code readability: Clear, concise code is crucial in interviews. Some languages naturally lend themselves to more readable solutions.
- Industry relevance: Choosing a widely-used language demonstrates your awareness of industry trends.
- Company-specific preferences: Some companies may have a preference based on their tech stack.
- Your comfort level: You’ll perform best in a language you’re most comfortable with.
With these factors in mind, let’s explore the top programming languages that can give you an edge in coding interviews.
Top Programming Languages for Coding Interviews
1. Python
Python has become increasingly popular in recent years, and for good reason. Its simplicity, readability, and versatility make it an excellent choice for coding interviews.
Why Python?
- Easy to read and write: Python’s clean syntax allows you to express concepts in fewer lines of code.
- Extensive standard library: Python comes with “batteries included,” offering many built-in functions and modules.
- Ideal for algorithmic problems: Python’s high-level data structures make it perfect for solving complex algorithmic challenges.
- Popular in data science and machine learning: If you’re targeting roles in these fields, Python is a must-know.
Example: Reversing a string in Python
def reverse_string(s):
return s[::-1]
# Usage
original = "Hello, World!"
reversed = reverse_string(original)
print(reversed) # Output: !dlroW ,olleH
This simple example showcases Python’s concise syntax and powerful slicing capabilities.
2. Java
Java remains a cornerstone in the software development world, especially for large-scale enterprise applications. Its popularity in coding interviews is unwavering.
Why Java?
- Object-oriented programming: Java’s strong OOP principles are valued in many software engineering roles.
- Platform independence: “Write once, run anywhere” philosophy is attractive to many companies.
- Robust standard library: Java’s extensive API provides tools for almost any programming task.
- Strong typing: This can help catch errors early and demonstrate your attention to detail.
Example: Implementing a basic LinkedList in Java
public class LinkedList<T> {
private class Node {
T data;
Node next;
Node(T data) {
this.data = data;
this.next = null;
}
}
private Node head;
public void add(T data) {
Node newNode = new Node(data);
if (head == null) {
head = newNode;
} else {
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
}
public void printList() {
Node current = head;
while (current != null) {
System.out.print(current.data + " -> ");
current = current.next;
}
System.out.println("null");
}
}
// Usage
LinkedList<Integer> list = new LinkedList<>();
list.add(1);
list.add(2);
list.add(3);
list.printList(); // Output: 1 -> 2 -> 3 -> null
This example demonstrates Java’s object-oriented nature and how it handles data structures, which are common topics in coding interviews.
3. C++
C++ is known for its performance and is widely used in systems programming, game development, and other performance-critical applications. It’s a favorite among competitive programmers and is often used in coding interviews for roles that require low-level system knowledge.
Why C++?
- High performance: C++ allows for fine-grained control over system resources.
- STL (Standard Template Library): Provides efficient implementations of common data structures and algorithms.
- Object-oriented and generic programming: Supports multiple programming paradigms.
- Memory management: Understanding pointers and memory allocation can impress interviewers.
Example: Implementing a simple stack using STL in C++
#include <iostream>
#include <stack>
int main() {
std::stack<int> s;
s.push(1);
s.push(2);
s.push(3);
std::cout << "Top element: " << s.top() << std::endl;
s.pop();
std::cout << "Size after pop: " << s.size() << std::endl;
while (!s.empty()) {
std::cout << s.top() << " ";
s.pop();
}
return 0;
}
// Output:
// Top element: 3
// Size after pop: 2
// 2 1
This example shows how C++ can efficiently implement data structures using the STL, which is a valuable skill in coding interviews.
4. JavaScript
As the language of the web, JavaScript is essential for front-end development and has gained significant traction in back-end development with Node.js. Its versatility makes it a popular choice in coding interviews, especially for web-focused roles.
Why JavaScript?
- Ubiquity in web development: Essential for both client-side and server-side programming.
- Asynchronous programming: Understanding callbacks, promises, and async/await is highly valued.
- Functional programming features: JavaScript’s support for functional programming concepts is increasingly important.
- Dynamic typing: Allows for flexible and rapid development, which can be advantageous in interviews.
Example: Implementing a debounce function in JavaScript
function debounce(func, delay) {
let timeoutId;
return function (...args) {
clearTimeout(timeoutId);
timeoutId = setTimeout(() => {
func.apply(this, args);
}, delay);
};
}
// Usage
const expensiveOperation = () => {
console.log("Expensive operation executed!");
};
const debouncedOperation = debounce(expensiveOperation, 300);
// This will only log once after 300ms
debouncedOperation();
debouncedOperation();
debouncedOperation();
This example demonstrates JavaScript’s capability to handle higher-order functions and closures, which are often topics of discussion in interviews.
5. Go (Golang)
Go, developed by Google, has gained popularity for its simplicity, efficiency, and strong support for concurrent programming. While it may not be as common in coding interviews as the languages mentioned above, its growing adoption in the industry makes it worth considering.
Why Go?
- Simplicity and readability: Go’s minimalist syntax makes it easy to write and understand code quickly.
- Built-in concurrency: Goroutines and channels make concurrent programming more accessible.
- Fast compilation and execution: Go’s performance can be impressive in coding challenges.
- Growing popularity in cloud and networking: Particularly relevant for backend and systems programming roles.
Example: Implementing a concurrent web crawler in Go
package main
import (
"fmt"
"net/http"
"sync"
)
func crawl(url string, wg *sync.WaitGroup) {
defer wg.Done()
resp, err := http.Get(url)
if err != nil {
fmt.Printf("Error crawling %s: %v\n", url, err)
return
}
defer resp.Body.Close()
fmt.Printf("Crawled %s - Status: %s\n", url, resp.Status)
}
func main() {
urls := []string{
"https://golang.org",
"https://google.com",
"https://github.com",
}
var wg sync.WaitGroup
for _, url := range urls {
wg.Add(1)
go crawl(url, &wg)
}
wg.Wait()
fmt.Println("All URLs crawled")
}
This example showcases Go’s concurrency features, which can be a strong point in interviews, especially for roles involving distributed systems or high-performance applications.
Language-Specific Tips for Coding Interviews
While knowing the syntax and features of a language is crucial, here are some language-specific tips to help you excel in coding interviews:
Python
- Master list comprehensions for concise data manipulation.
- Understand the differences between lists, tuples, and dictionaries.
- Familiarize yourself with built-in functions like map(), filter(), and reduce().
- Learn to use generators for memory-efficient iterations.
Java
- Be comfortable with Java Collections Framework (List, Set, Map interfaces).
- Understand the principles of inheritance and polymorphism.
- Know how to use Java 8+ features like lambdas and streams.
- Be aware of the differences between primitive types and their wrapper classes.
C++
- Master the use of STL containers and algorithms.
- Understand memory management and the rule of three/five.
- Know how to use templates for generic programming.
- Be familiar with C++11/14/17 features like auto, lambda expressions, and smart pointers.
JavaScript
- Understand closures and how they work.
- Master asynchronous programming with promises and async/await.
- Know the differences between var, let, and const.
- Be comfortable with array methods like map(), filter(), and reduce().
Go
- Understand how to use goroutines and channels effectively.
- Know the differences between slices and arrays.
- Be familiar with Go’s approach to error handling.
- Understand interfaces and how they enable polymorphism in Go.
Beyond Languages: What Else Matters in Coding Interviews
While choosing the right programming language is important, it’s not the only factor that determines success in coding interviews. Here are other crucial aspects to focus on:
1. Data Structures and Algorithms
Regardless of the language you choose, a solid understanding of fundamental data structures (arrays, linked lists, trees, graphs, etc.) and algorithms (sorting, searching, dynamic programming, etc.) is essential. Practice implementing these from scratch and solving problems using them.
2. Problem-Solving Skills
Interviewers are often more interested in your approach to problem-solving than in the final code. Practice breaking down complex problems, considering edge cases, and explaining your thought process clearly.
3. Time and Space Complexity Analysis
Be prepared to analyze the efficiency of your solutions in terms of both time and space complexity. Understanding Big O notation is crucial for discussing the performance characteristics of your code.
4. System Design (for more senior roles)
For senior positions, you may be asked about designing large-scale systems. Familiarize yourself with concepts like load balancing, caching, database sharding, and microservices architecture.
5. Coding Best Practices
Write clean, readable, and well-commented code. Show that you understand principles of good software design, such as modularity, DRY (Don’t Repeat Yourself), and SOLID principles.
6. Version Control
While not always directly tested in coding interviews, familiarity with version control systems (particularly Git) is often expected and can be a bonus point.
7. Soft Skills
Don’t underestimate the importance of communication skills, ability to work in a team, and passion for technology. These soft skills can sometimes be the deciding factor in an interview.
Preparing for Coding Interviews with AlgoCademy
Now that we’ve covered the essential programming languages and skills for coding interviews, let’s explore how AlgoCademy can help you prepare effectively:
1. Comprehensive Curriculum
AlgoCademy offers a structured learning path that covers all the major topics you’ll encounter in coding interviews. From basic data structures to advanced algorithms, our curriculum is designed to build your skills progressively.
2. Interactive Coding Challenges
Practice makes perfect. AlgoCademy provides a wide range of coding challenges that simulate real interview questions. These interactive exercises allow you to write, run, and test your code in real-time, helping you gain hands-on experience.
3. Multiple Language Support
Whether you prefer Python, Java, C++, JavaScript, or Go, AlgoCademy supports multiple programming languages. This allows you to practice in your language of choice or even explore new languages to broaden your skill set.
4. AI-Powered Assistance
Stuck on a problem? AlgoCademy’s AI-powered assistant can provide hints, explain concepts, and even help debug your code. This feature ensures that you’re never left frustrated and can always make progress in your learning journey.
5. Performance Analytics
Track your progress with detailed analytics. AlgoCademy provides insights into your strengths and weaknesses, helping you focus your efforts where they’re most needed.
6. Mock Interviews
Practice makes perfect. AlgoCademy offers mock interview sessions that simulate the pressure and time constraints of real coding interviews. These sessions help you get comfortable with the interview process and improve your performance under pressure.
7. Community Support
Join a community of like-minded learners. Discuss problems, share solutions, and learn from peers. The collaborative environment at AlgoCademy enhances your learning experience and keeps you motivated.
8. Up-to-date Content
The tech industry evolves rapidly, and so do interview questions. AlgoCademy regularly updates its content to reflect current trends and common interview questions at top tech companies.
Conclusion
Choosing the right programming language for coding interviews is an important decision, but it’s just one piece of the puzzle. Whether you opt for the simplicity of Python, the robustness of Java, the performance of C++, the versatility of JavaScript, or the efficiency of Go, what matters most is your problem-solving ability and deep understanding of computer science fundamentals.
Remember, the best language for you is often the one you’re most comfortable with and can think in naturally under pressure. However, being versatile and having knowledge of multiple languages can be a significant advantage, especially when targeting specific companies or roles.
As you prepare for your coding interviews, leverage platforms like AlgoCademy to hone your skills, practice consistently, and gain confidence. With dedication, the right resources, and a solid grasp of the languages and concepts we’ve discussed, you’ll be well-equipped to tackle any coding interview that comes your way.
Happy coding, and best of luck with your interviews!