92 Tips for Improving Your Coding Speed and Efficiency
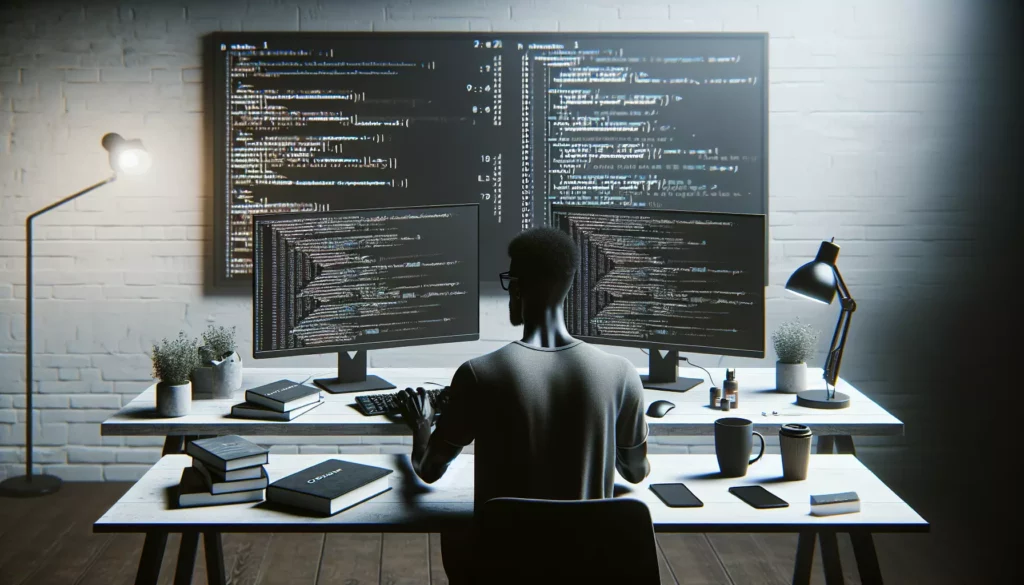
In the fast-paced world of software development, the ability to code quickly and efficiently is a valuable skill that can set you apart from your peers. Whether you’re a beginner looking to enhance your coding abilities or an experienced programmer aiming to boost your productivity, this comprehensive guide will provide you with 92 actionable tips to improve your coding speed and efficiency. Let’s dive in!
1. Master Your IDE or Text Editor
Your Integrated Development Environment (IDE) or text editor is your primary tool for writing code. Mastering it can significantly improve your coding speed and efficiency.
1. Learn Keyboard Shortcuts
Memorize and use keyboard shortcuts for common actions like copy, paste, find, and replace. This can save you countless mouse clicks and valuable time.
2. Customize Your Environment
Set up your IDE or text editor to suit your preferences. Customize themes, fonts, and layouts to create a comfortable coding environment.
3. Use Code Snippets
Create and use code snippets for frequently used code blocks. Most modern IDEs allow you to define custom snippets that can be quickly inserted with a few keystrokes.
4. Enable Auto-Completion
Take advantage of auto-completion features to reduce typing and minimize errors. This can significantly speed up your coding process.
5. Utilize Multi-Cursor Editing
Learn to use multi-cursor editing to make simultaneous changes across multiple lines of code. This can be a huge time-saver for repetitive tasks.
2. Optimize Your Workflow
Streamlining your workflow can lead to significant improvements in coding speed and efficiency.
6. Plan Before You Code
Spend time planning your approach before diving into coding. A clear plan can help you avoid unnecessary rewrites and save time in the long run.
7. Use Version Control
Implement a version control system like Git to track changes, collaborate with others, and easily revert to previous versions if needed.
8. Automate Repetitive Tasks
Use scripts or tools to automate repetitive tasks like file organization, code formatting, or deployment processes.
9. Implement Continuous Integration
Set up continuous integration to automatically build and test your code with each commit. This can help catch errors early and save debugging time later.
10. Practice Time Management
Use techniques like the Pomodoro Technique to manage your time effectively and maintain focus during coding sessions.
3. Enhance Your Coding Skills
Continuously improving your coding skills is crucial for increasing your speed and efficiency.
11. Learn Touch Typing
Improve your typing speed and accuracy through touch typing practice. This can significantly reduce the time spent on inputting code.
12. Master Your Programming Language
Deepen your understanding of your primary programming language. Knowing language-specific features and best practices can lead to more efficient code writing.
13. Study Algorithms and Data Structures
A solid grasp of algorithms and data structures can help you choose the most efficient solutions for coding problems.
14. Practice Regularly
Engage in coding challenges or personal projects to keep your skills sharp and discover new techniques.
15. Read Other People’s Code
Analyze well-written code from open-source projects or experienced developers to learn new patterns and techniques.
4. Write Clean and Maintainable Code
Writing clean, maintainable code not only improves readability but also saves time in the long run.
16. Follow Coding Standards
Adhere to established coding standards and style guides for your language and project. This improves code consistency and readability.
17. Use Meaningful Names
Choose descriptive and meaningful names for variables, functions, and classes. This reduces the need for comments and makes your code self-explanatory.
18. Keep Functions Small and Focused
Write small, single-purpose functions that are easy to understand and maintain. This also promotes code reusability.
19. Comment Wisely
Use comments to explain why something is done, not what is done. Let your code speak for itself as much as possible.
20. Refactor Regularly
Continuously refactor your code to improve its structure and eliminate redundancies. This can make future modifications easier and faster.
5. Leverage Tools and Libraries
Using the right tools and libraries can significantly boost your coding efficiency.
21. Use Package Managers
Utilize package managers like npm, pip, or Maven to easily install and manage dependencies in your projects.
22. Leverage Existing Libraries
Don’t reinvent the wheel. Use well-established libraries for common functionalities to save time and reduce potential bugs.
23. Implement Linters
Use linting tools to automatically catch coding style issues and potential errors before they become problems.
24. Utilize Code Generators
For repetitive boilerplate code, consider using code generators to create initial structures quickly.
25. Employ Static Analysis Tools
Use static analysis tools to identify potential bugs, security vulnerabilities, and code smells automatically.
6. Optimize Your Development Environment
A well-optimized development environment can significantly improve your coding speed and efficiency.
26. Use Multiple Monitors
If possible, use multiple monitors to have your code, documentation, and testing environment visible simultaneously.
27. Invest in a Good Keyboard
A high-quality keyboard can improve typing comfort and speed, especially for long coding sessions.
28. Organize Your Workspace
Keep your physical and digital workspaces organized to minimize distractions and time spent searching for resources.
29. Use Virtual Desktops
Utilize virtual desktops to separate different aspects of your work, like coding, debugging, and documentation.
30. Optimize Your Computer’s Performance
Ensure your computer is running optimally by managing startup programs, updating drivers, and maintaining sufficient free disk space.
7. Enhance Your Problem-Solving Skills
Improving your problem-solving skills can lead to faster and more efficient coding.
31. Break Problems into Smaller Parts
Divide complex problems into smaller, manageable sub-problems. This makes the overall task less daunting and easier to solve.
32. Use Pseudocode
Before writing actual code, sketch out your solution in pseudocode. This can help clarify your thoughts and identify potential issues early.
33. Learn Multiple Approaches
Study different problem-solving techniques and algorithms. Having a diverse toolkit allows you to choose the most efficient solution for each problem.
34. Practice Debugging
Enhance your debugging skills to quickly identify and fix issues in your code. This can save significant time during development.
35. Collaborate and Seek Feedback
Discuss problems with colleagues or online communities. Fresh perspectives can often lead to more efficient solutions.
8. Improve Your Testing Strategies
Effective testing can catch bugs early and save time in the long run.
36. Write Unit Tests
Develop a habit of writing unit tests for your code. This can help catch bugs early and make refactoring easier.
37. Implement Test-Driven Development (TDD)
Consider adopting TDD, where you write tests before implementing features. This can lead to more robust and well-designed code.
38. Use Automated Testing Tools
Leverage automated testing tools to run your test suite quickly and consistently.
39. Practice Code Reviews
Regularly review your own code and participate in peer code reviews. This can help catch issues early and improve overall code quality.
40. Learn to Write Testable Code
Design your code with testability in mind. This often leads to more modular and maintainable code structures.
9. Enhance Your Typing Skills
Improving your typing speed and accuracy can directly impact your coding efficiency.
41. Practice Typing Code
Use typing practice websites that focus on code snippets rather than just plain text.
42. Learn Common Coding Symbols
Practice typing common coding symbols like brackets, parentheses, and operators without looking at the keyboard.
43. Use Typing Games
Engage in typing games designed for programmers to make the learning process more enjoyable.
44. Set Typing Speed Goals
Set incremental goals for your typing speed and track your progress over time.
45. Consider Alternative Keyboard Layouts
Explore alternative keyboard layouts like Dvorak or Colemak, which some programmers find more efficient for coding.
10. Master Debugging Techniques
Efficient debugging can save countless hours during development.
46. Use Debugging Tools
Familiarize yourself with your IDE’s debugging tools, including breakpoints, watch windows, and step-through functionality.
47. Learn to Read Error Messages
Develop the skill to quickly interpret error messages and stack traces to pinpoint issues faster.
48. Implement Logging
Use logging in your code to track program flow and variable states, making it easier to identify issues.
49. Practice Rubber Duck Debugging
Explain your code and the problem you’re facing to an inanimate object (like a rubber duck). This process often helps clarify the issue.
50. Use Version Control for Debugging
Leverage version control systems to compare different versions of your code and identify when and where issues were introduced.
11. Optimize Your Code
Writing optimized code from the start can save time on performance improvements later.
51. Learn Big O Notation
Understand Big O notation to analyze and improve the time and space complexity of your algorithms.
52. Use Appropriate Data Structures
Choose the right data structures for your tasks to optimize performance and memory usage.
53. Avoid Premature Optimization
Focus on writing clear, correct code first. Optimize only when necessary and after profiling.
54. Learn Memory Management
Understand how memory is managed in your programming language to write more efficient code.
55. Use Caching Techniques
Implement caching where appropriate to avoid redundant computations and improve performance.
12. Enhance Your Command Line Skills
Proficiency with the command line can significantly speed up various development tasks.
56. Learn Basic Shell Commands
Master essential shell commands for file manipulation, process management, and system navigation.
57. Use Command Line Tools
Familiarize yourself with command line tools specific to your development stack, such as git, npm, or docker.
58. Create Aliases and Scripts
Set up aliases and shell scripts for frequently used command sequences to save time.
59. Use Command Line Text Editors
Learn to use command line text editors like Vim or Emacs for quick file edits without leaving the terminal.
60. Master Pipe and Redirection
Understand and use pipe (|) and redirection (>, <) operators to chain commands and manipulate input/output efficiently.
13. Improve Your Code Organization
Well-organized code is easier to navigate, understand, and modify, leading to improved coding efficiency.
61. Follow a Consistent File Structure
Adopt a logical and consistent file structure for your projects to make navigation easier.
62. Use Design Patterns
Learn and apply appropriate design patterns to solve common programming problems efficiently.
63. Implement Modular Programming
Break your code into modular, reusable components to improve maintainability and reduce redundancy.
64. Use Clear Naming Conventions
Adopt clear and consistent naming conventions for files, classes, functions, and variables.
65. Organize Imports and Dependencies
Keep your import statements and dependencies organized and remove unused ones regularly.
14. Enhance Your Git Skills
Proficiency with Git can streamline your version control workflow and collaboration.
66. Master Basic Git Commands
Ensure you’re comfortable with basic Git commands like clone, commit, push, pull, and merge.
67. Use Branching Strategies
Adopt a branching strategy like Git Flow or GitHub Flow to manage feature development and releases effectively.
68. Leverage Git Hooks
Use Git hooks to automate tasks like code linting or running tests before commits or pushes.
69. Learn Interactive Rebasing
Master interactive rebasing to clean up your commit history before merging or pushing.
70. Use Git Aliases
Set up Git aliases for frequently used commands or command sequences to save time.
15. Improve Your Documentation Skills
Good documentation can save time for you and your team in the long run.
71. Write Clear README Files
Create comprehensive README files for your projects, including setup instructions, usage examples, and contribution guidelines.
72. Use Inline Documentation
Write clear and concise inline documentation for complex code sections to aid understanding and maintenance.
73. Generate API Documentation
Use tools like Swagger or Javadoc to automatically generate API documentation from your code comments.
74. Maintain a Personal Knowledge Base
Keep a personal wiki or knowledge base to document solutions to problems you’ve encountered.
75. Document Your Workflow
Document your development workflow and processes to help onboard new team members and maintain consistency.
16. Enhance Your Coding Mindset
Developing the right mindset can significantly impact your coding speed and efficiency.
76. Embrace Continuous Learning
Stay curious and continuously learn new technologies, techniques, and best practices in your field.
77. Practice Mindfulness
Use mindfulness techniques to improve focus and reduce stress during coding sessions.
78. Develop a Growth Mindset
View challenges as opportunities for growth rather than obstacles.
79. Learn from Mistakes
Analyze your mistakes and use them as learning opportunities to improve your skills.
80. Set Realistic Goals
Set achievable, incremental goals for your coding projects and personal development.
17. Optimize Your Physical Environment
Your physical environment can have a significant impact on your coding efficiency.
81. Ensure Proper Ergonomics
Set up your workspace with ergonomic considerations to prevent discomfort and potential health issues.
82. Optimize Lighting
Ensure your workspace has adequate lighting to reduce eye strain and maintain focus.
83. Minimize Distractions
Create a quiet, distraction-free environment for focused coding sessions.
84. Take Regular Breaks
Implement regular break intervals to rest your eyes and mind, improving overall productivity.
85. Stay Hydrated and Nourished
Keep water and healthy snacks nearby to maintain energy levels during coding sessions.
18. Leverage AI and Machine Learning Tools
Embrace AI-powered tools to enhance your coding efficiency.
86. Use AI-Powered Code Completion
Leverage AI-driven code completion tools like GitHub Copilot or Tabnine to speed up coding.
87. Implement Chatbots for Quick Answers
Use AI chatbots or virtual assistants to quickly find answers to coding questions.
88. Utilize AI for Code Review
Explore AI-powered code review tools to catch potential issues before human review.
89. Leverage Machine Learning for Bug Prediction
Use machine learning models to predict potential bugs in your codebase.
90. Explore AI-Assisted Refactoring
Investigate tools that use AI to suggest and assist with code refactoring.
19. Collaborate Effectively
Efficient collaboration can significantly improve overall coding speed and productivity.
91. Use Collaboration Platforms
Leverage platforms like Slack or Microsoft Teams for quick communication and file sharing with team members.
92. Participate in Pair Programming
Engage in pair programming sessions to share knowledge, catch errors quickly, and improve overall code quality.
Conclusion
Improving your coding speed and efficiency is a continuous journey that involves mastering your tools, optimizing your workflow, enhancing your skills, and adopting the right mindset. By implementing these 92 tips, you can significantly boost your productivity and become a more proficient programmer. Remember, the key is to consistently apply these practices and continuously seek ways to improve. Happy coding!