How to Handle Technical Debt in Long-Term Projects: A Comprehensive Guide
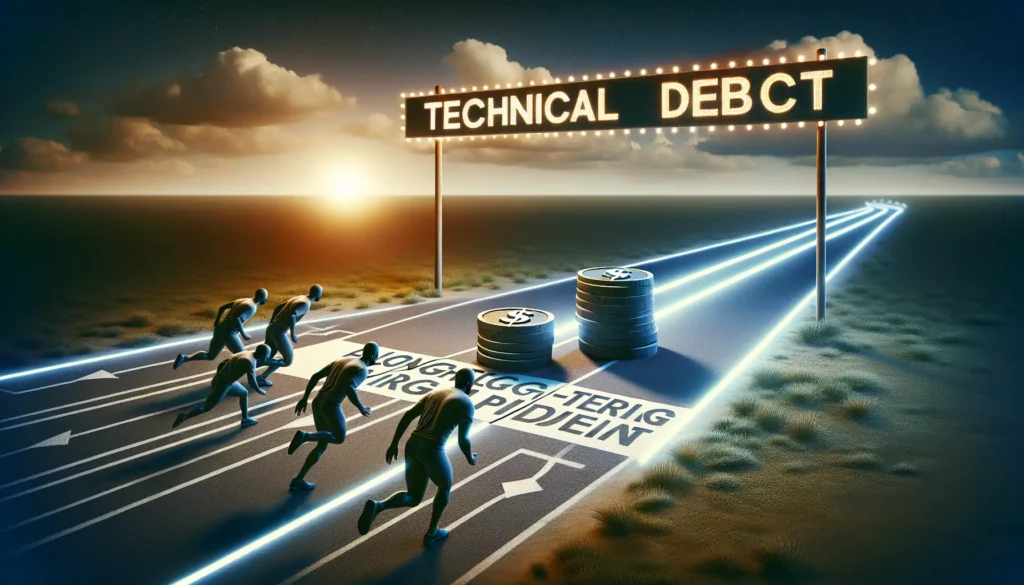
In the world of software development, technical debt is an inevitable reality that every development team faces, especially in long-term projects. As a concept introduced by Ward Cunningham in 1992, technical debt refers to the implied cost of additional rework caused by choosing an easy solution now instead of using a better approach that would take longer. While it’s often necessary to incur some technical debt to meet deadlines or ship features quickly, left unchecked, it can significantly hinder a project’s progress and scalability. In this comprehensive guide, we’ll explore strategies to effectively handle technical debt in long-term projects, ensuring your codebase remains healthy and maintainable over time.
Understanding Technical Debt
Before diving into strategies for managing technical debt, it’s crucial to understand what it is and why it occurs. Technical debt can be thought of as a loan taken out on your codebase. Like financial debt, it accrues interest over time, making it increasingly difficult and expensive to pay off the longer it remains.
Technical debt can manifest in various forms:
- Code duplication
- Poor code quality or design
- Outdated dependencies
- Lack of documentation
- Insufficient test coverage
- Architectural shortcomings
It’s important to note that not all technical debt is bad. Sometimes, taking on technical debt is a strategic decision to meet business objectives. The key is to be aware of the debt you’re incurring and have a plan to address it.
The Impact of Technical Debt on Long-Term Projects
In long-term projects, the effects of technical debt can be particularly severe:
- Decreased Productivity: As technical debt accumulates, developers spend more time working around existing issues rather than implementing new features.
- Increased Bugs: Poor code quality and architectural issues often lead to more bugs, requiring additional time for fixes.
- Difficulty in Onboarding: New team members struggle to understand and work with a codebase burdened by technical debt.
- Reduced Agility: The ability to pivot or adapt to new requirements becomes increasingly challenging.
- Higher Costs: The longer technical debt persists, the more expensive it becomes to address.
Strategies for Handling Technical Debt
Now that we understand the impact of technical debt, let’s explore strategies to effectively manage it in long-term projects:
1. Acknowledge and Quantify Technical Debt
The first step in handling technical debt is to acknowledge its existence and quantify it. This involves:
- Conducting regular code reviews and audits
- Using static code analysis tools to identify problematic areas
- Tracking metrics like code complexity, test coverage, and bug frequency
- Creating a technical debt register to document known issues
By quantifying technical debt, you can prioritize which areas to address first and track progress over time.
2. Implement the Boy Scout Rule
The Boy Scout Rule, adapted for programming by Robert C. Martin, states: “Leave the code better than you found it.” This simple principle can have a significant impact on managing technical debt. Encourage your team to make small improvements whenever they work on a piece of code. This might involve:
- Refactoring small sections of code
- Improving variable or function names
- Adding missing comments or documentation
- Fixing minor bugs or inefficiencies
Over time, these small improvements can add up to substantial reductions in technical debt.
3. Allocate Time for Debt Repayment
Make technical debt repayment a regular part of your development process. This can be achieved by:
- Dedicating a percentage of each sprint to addressing technical debt (e.g., 20% of development time)
- Planning specific “cleanup sprints” focused entirely on reducing technical debt
- Including refactoring tasks in the definition of “done” for user stories
By consistently allocating time to address technical debt, you prevent it from accumulating to unmanageable levels.
4. Prioritize and Plan Debt Repayment
Not all technical debt is created equal. Some debt has a higher impact on the project than others. Prioritize repayment based on factors such as:
- Impact on development speed
- Risk of introducing bugs
- Effect on system performance
- Difficulty of future maintenance
Create a roadmap for addressing high-priority debt items and integrate it into your project planning.
5. Improve Code Quality Standards
Preventing new technical debt is just as important as addressing existing debt. Implement and enforce high code quality standards through:
- Establishing coding guidelines and best practices
- Using linters and code formatters
- Implementing continuous integration (CI) with automated code quality checks
- Conducting regular code reviews
By maintaining high standards, you reduce the introduction of new technical debt.
6. Enhance Test Coverage
Comprehensive test coverage is crucial for managing technical debt. It allows developers to refactor code with confidence, knowing that existing functionality is preserved. Improve your test coverage by:
- Writing unit tests for new code
- Adding integration and end-to-end tests
- Implementing test-driven development (TDD) practices
- Using code coverage tools to identify areas lacking tests
Aim for a balance of different types of tests to ensure comprehensive coverage.
7. Refactor Strategically
When addressing technical debt through refactoring, it’s important to approach it strategically:
- Focus on high-impact areas first
- Break large refactoring tasks into smaller, manageable chunks
- Use the strangler fig pattern for large-scale architectural changes
- Ensure thorough testing before and after refactoring
Remember, the goal of refactoring is to improve the internal structure of the code without changing its external behavior.
8. Keep Dependencies Updated
Outdated dependencies can be a significant source of technical debt. Regularly update your project’s dependencies to:
- Benefit from performance improvements and bug fixes
- Ensure compatibility with newer systems and tools
- Address security vulnerabilities
Implement a process for regularly reviewing and updating dependencies, and consider using tools that automate dependency updates.
9. Improve Documentation
Good documentation is crucial for managing technical debt, especially in long-term projects where team members may change over time. Improve your documentation by:
- Writing clear and concise code comments
- Maintaining up-to-date API documentation
- Creating architectural diagrams and system overviews
- Documenting design decisions and trade-offs
Well-documented code is easier to understand, maintain, and refactor, reducing the accumulation of technical debt.
10. Foster a Culture of Quality
Managing technical debt is not just about processes and tools; it’s also about culture. Foster a team culture that values code quality and continuous improvement:
- Educate team members about the importance of managing technical debt
- Celebrate efforts to improve code quality and reduce debt
- Encourage open discussions about technical challenges and solutions
- Lead by example in following best practices and addressing technical debt
A team that understands and values code quality is more likely to proactively manage technical debt.
Implementing Technical Debt Management in Your Project
Now that we’ve covered strategies for handling technical debt, let’s look at how to implement these in your long-term project:
1. Assess Your Current State
Start by assessing your project’s current technical debt. Use code analysis tools, review your backlog, and gather feedback from team members. Create a comprehensive list of technical debt items.
2. Categorize and Prioritize
Categorize your technical debt items (e.g., code quality, architecture, testing, documentation) and prioritize them based on their impact and urgency.
3. Create a Debt Repayment Plan
Develop a plan for addressing high-priority debt items. This might involve:
// Example: Technical Debt Repayment Plan
1. Refactor authentication module (2 weeks)
2. Increase unit test coverage to 80% (ongoing, 10% of sprint time)
3. Update outdated dependencies (1 week)
4. Improve API documentation (1 week)
5. Refactor data access layer (3 weeks)
4. Integrate with Development Process
Incorporate technical debt management into your regular development process. This could involve:
- Adding technical debt items to your backlog
- Including debt repayment tasks in sprint planning
- Tracking technical debt metrics in your project dashboards
5. Monitor and Adjust
Regularly review your technical debt levels and the effectiveness of your management strategies. Be prepared to adjust your approach based on the results and changing project needs.
Tools for Managing Technical Debt
Several tools can assist in managing technical debt:
- SonarQube: Provides continuous inspection of code quality and security
- JArchitect/NDepend: Offers code analysis and architecture management
- CodeClimate: Automates code review and monitors test coverage
- Coveralls: Tracks code coverage over time
- ESLint/TSLint: Identifies and fixes problems in JavaScript/TypeScript code
- Prettier: Ensures consistent code formatting
Case Study: Reducing Technical Debt in a Legacy System
Let’s consider a hypothetical case study to illustrate the process of handling technical debt in a long-term project:
Background: A company has been maintaining a large e-commerce platform for over a decade. The system has accumulated significant technical debt, causing frequent bugs, slow development cycles, and difficulty in adding new features.
Approach:
- Assessment: The team conducted a thorough code review and used static analysis tools to identify major areas of technical debt.
- Prioritization: They prioritized addressing the outdated authentication system, improving test coverage, and refactoring the product catalog module.
- Plan: A six-month plan was created, allocating 30% of development time to technical debt repayment.
- Implementation: The team:
- Replaced the authentication system with a modern, secure solution
- Increased unit test coverage from 40% to 75%
- Refactored the product catalog module, improving its performance by 40%
- Updated all major dependencies to their latest versions
- Continuous Improvement: They implemented the Boy Scout Rule and regular “cleanup sprints” to prevent future debt accumulation.
Results: After six months, the team reported a 50% reduction in bug reports, a 30% increase in development speed for new features, and significantly improved system performance.
Conclusion
Managing technical debt is a crucial aspect of maintaining healthy, long-term software projects. By acknowledging its existence, implementing strategies to address it, and fostering a culture of code quality, development teams can keep technical debt under control and ensure their projects remain scalable and maintainable over time.
Remember, the goal is not to eliminate technical debt entirely—some level of technical debt is often necessary and even beneficial. The key is to manage it actively, making informed decisions about when to take on debt and when to pay it off.
By following the strategies outlined in this guide and adapting them to your specific project needs, you can effectively handle technical debt in your long-term projects, leading to more robust, efficient, and successful software development efforts.