The Role of Test-Driven Development in Writing Robust Code
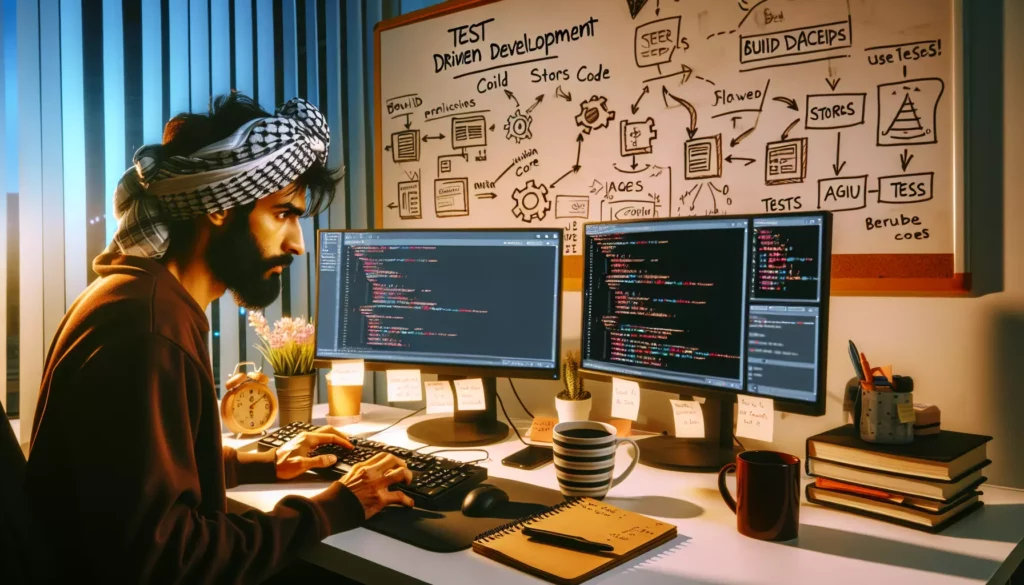
In the ever-evolving landscape of software development, writing robust and reliable code is paramount. One methodology that has gained significant traction in recent years is Test-Driven Development (TDD). This approach not only helps in creating more stable and maintainable code but also plays a crucial role in improving overall software quality. In this comprehensive guide, we’ll explore the ins and outs of Test-Driven Development, its benefits, challenges, and how it can be effectively implemented in your coding practice.
What is Test-Driven Development?
Test-Driven Development is a software development process that relies on the repetition of a very short development cycle. It begins with the developer writing a failing automated test case that defines a desired improvement or new function. This is followed by producing the minimum amount of code to pass that test, and then refactoring the new code to acceptable standards.
The TDD cycle typically follows these steps:
- Write a test
- Run the test (which should fail because the feature hasn’t been implemented)
- Write the minimum amount of code to make the test pass
- Run the test again (which should now pass)
- Refactor the code
- Repeat
This cycle is often referred to as “Red-Green-Refactor,” where Red represents the failing test, Green represents the passing test, and Refactor represents the optimization of the code.
The Benefits of Test-Driven Development
1. Improved Code Quality
One of the primary benefits of TDD is the improvement in code quality. By writing tests before the actual code, developers are forced to think about the design and functionality of their code from the outset. This leads to cleaner, more modular, and more maintainable code.
2. Better Code Coverage
TDD naturally leads to higher test coverage. Since tests are written for each feature before the implementation, it’s less likely that any functionality will be left untested. This comprehensive testing helps in catching bugs early in the development process.
3. Faster Debugging
When a test fails, it’s immediately clear which piece of code is causing the issue. This makes debugging faster and more efficient, as developers can quickly isolate and fix problems.
4. Living Documentation
Tests serve as a form of documentation for the codebase. They describe what the code should do and how it should behave, providing valuable insights for other developers who might work on the project in the future.
5. Confidence in Refactoring
With a comprehensive suite of tests in place, developers can confidently refactor and improve their code. If a change breaks existing functionality, the tests will catch it immediately.
Implementing Test-Driven Development
Now that we understand the benefits of TDD, let’s look at how to implement it in practice.
Step 1: Write a Failing Test
The first step in TDD is to write a test that fails. This test should describe a single aspect of the desired functionality. For example, if we’re building a calculator application, we might start with a test for addition:
def test_addition():
calculator = Calculator()
result = calculator.add(2, 3)
assert result == 5
Running this test will fail because we haven’t implemented the Calculator class or its add method yet.
Step 2: Write the Minimum Code to Pass the Test
Next, we write just enough code to make the test pass. In this case, we might write:
class Calculator:
def add(self, a, b):
return 5
This implementation is obviously not correct for all cases, but it passes our specific test.
Step 3: Refactor
Now that our test is passing, we can refactor our code to make it more general and efficient:
class Calculator:
def add(self, a, b):
return a + b
Our test still passes, but now our implementation is correct for all cases of addition.
Step 4: Repeat
We continue this process, writing more tests for different scenarios and edge cases, implementing the code to pass these tests, and refactoring as necessary.
TDD Best Practices
To get the most out of Test-Driven Development, consider the following best practices:
1. Keep Tests Small and Focused
Each test should focus on a single piece of functionality. This makes tests easier to write, understand, and maintain.
2. Use Descriptive Test Names
Test names should clearly describe what is being tested. For example, “test_addition_of_two_positive_numbers” is more informative than “test_add”.
3. Follow the AAA Pattern
Structure your tests using the Arrange-Act-Assert pattern:
- Arrange: Set up the test data and conditions
- Act: Perform the action being tested
- Assert: Check the result
4. Don’t Test Private Methods Directly
Focus on testing the public interface of your classes. Private methods should be tested indirectly through the public methods that use them.
5. Refactor Regularly
Don’t skip the refactoring step. Regularly improving your code’s structure and readability is a key part of TDD.
Challenges of Test-Driven Development
While TDD offers many benefits, it’s not without its challenges:
1. Learning Curve
TDD requires a shift in mindset and can be challenging for developers who are used to writing code first and tests later (if at all).
2. Time Investment
Writing tests before code can feel like it slows down development initially. However, this investment often pays off in the long run with fewer bugs and easier maintenance.
3. Maintaining Test Suites
As projects grow, test suites can become large and potentially slow to run. It’s important to keep tests efficient and well-organized.
4. Overemphasis on Unit Tests
While unit tests are crucial, it’s important not to neglect integration and system tests. A balance of different test types is necessary for robust software.
TDD and Different Programming Paradigms
Test-Driven Development can be applied to various programming paradigms, but the approach may need to be adapted slightly for each:
TDD in Object-Oriented Programming
In OOP, TDD often focuses on testing the behavior of objects and their interactions. Tests might check if methods produce the expected output, if objects maintain the correct state, or if they collaborate correctly with other objects.
TDD in Functional Programming
In functional programming, TDD typically involves testing pure functions. Tests check if functions produce the expected output for given inputs, without relying on or modifying external state.
TDD in Procedural Programming
In procedural programming, TDD might focus on testing individual procedures or functions, as well as the overall flow of the program.
Tools for Test-Driven Development
There are numerous tools available to support Test-Driven Development across different programming languages:
Python
- unittest: Python’s built-in testing framework
- pytest: A more feature-rich testing framework for Python
- nose: Extends unittest to make testing easier
JavaScript
- Jest: A comprehensive testing framework for JavaScript
- Mocha: A flexible JavaScript test framework
- Jasmine: A behavior-driven development framework for testing JavaScript code
Java
- JUnit: The most widely used testing framework for Java
- TestNG: A testing framework inspired by JUnit and NUnit
C#
- NUnit: A unit-testing framework for all .NET languages
- xUnit.net: A free, open-source, community-focused unit testing tool for the .NET Framework
TDD and Continuous Integration
Test-Driven Development pairs extremely well with Continuous Integration (CI) practices. In a CI environment, automated tests are run every time code is pushed to the repository. This ensures that new changes don’t break existing functionality and helps maintain code quality over time.
Some popular CI tools that support TDD include:
- Jenkins
- Travis CI
- CircleCI
- GitLab CI
- GitHub Actions
These tools can be configured to run your test suite automatically, providing quick feedback on the health of your codebase.
TDD and Code Coverage
Code coverage is a metric that measures the percentage of your code that is executed by your tests. While 100% code coverage doesn’t guarantee bug-free code, it can be a useful indicator of the thoroughness of your tests.
Many testing frameworks and CI tools provide code coverage reports. These reports can help identify areas of your code that aren’t being tested, guiding you in writing additional tests.
However, it’s important to remember that code coverage is just one metric. The quality and relevance of your tests are just as important as their quantity.
TDD and Legacy Code
Applying TDD to legacy code can be challenging, but it’s not impossible. Here are some strategies for introducing TDD to an existing codebase:
1. Start with New Features
When adding new features to legacy code, use TDD for these new additions. This allows you to gradually introduce TDD practices without the need to immediately overhaul the entire codebase.
2. Write Characterization Tests
Before modifying legacy code, write tests that describe its current behavior. These tests serve as a safety net, ensuring that your changes don’t inadvertently alter existing functionality.
3. Refactor Gradually
As you work with different parts of the legacy code, gradually refactor them to make them more testable. This might involve breaking down large functions, reducing dependencies, or introducing interfaces.
4. Use Integration Tests
For parts of the legacy code that are difficult to unit test, consider writing integration tests. These can provide some level of confidence while you work on making the code more unit-testable.
TDD and Performance
While TDD primarily focuses on correctness and design, it can also be applied to performance optimization:
1. Performance Tests
Write tests that assert performance characteristics. For example, you might write a test that ensures a particular operation completes within a specified time limit.
2. Profiling
Use profiling tools in conjunction with TDD to identify performance bottlenecks. Once identified, you can write targeted tests for these areas and then optimize the code.
3. Benchmarking
Incorporate benchmarking into your test suite. This allows you to track performance over time and catch any regressions.
Conclusion
Test-Driven Development is a powerful methodology that can significantly improve the quality and reliability of your code. By writing tests before implementation, you’re forced to think critically about your code’s design and functionality from the outset. This leads to cleaner, more modular, and more maintainable code.
While TDD does come with its challenges, particularly in terms of the initial learning curve and time investment, the long-term benefits often outweigh these costs. Reduced bug counts, easier maintenance, and improved code design are just a few of the advantages that TDD can bring to your development process.
Remember, TDD is not a silver bullet. It’s a tool in your development toolkit, and like any tool, it should be used appropriately. Not every situation may call for strict TDD, but understanding and applying its principles can make you a more effective and confident developer.
As you continue your journey in software development, consider incorporating TDD into your workflow. Start small, perhaps with a single feature or module, and gradually expand your use of TDD as you become more comfortable with the process. With practice, you’ll likely find that TDD not only improves your code but also enhances your overall approach to problem-solving in software development.