45 Essential Tips for Writing Effective Documentation for Your Code
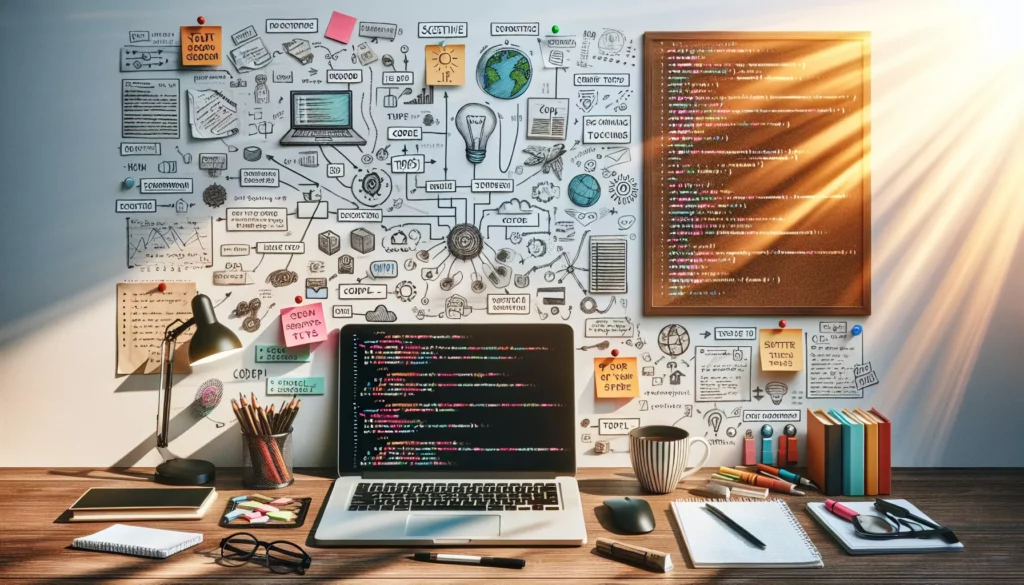
In the world of software development, writing clean, efficient code is only half the battle. The other crucial aspect is creating clear, comprehensive documentation that allows other developers (and your future self) to understand, maintain, and build upon your work. At AlgoCademy, we emphasize the importance of documentation as a key component of coding education and skill development. In this article, we’ll explore 45 essential tips for writing effective documentation for your code, helping you elevate your programming skills and become a more valuable contributor to any development team.
1. Start with the “Why”
Before diving into the details of your code, explain the purpose and motivation behind your project or module. This provides crucial context for anyone reading your documentation.
2. Use Clear and Concise Language
Avoid jargon and overly complex explanations. Write in a way that both beginners and experienced developers can understand.
3. Structure Your Documentation
Organize your documentation with clear headings, subheadings, and a logical flow of information. This makes it easier for readers to find what they’re looking for.
4. Include Installation Instructions
Provide step-by-step instructions on how to install and set up your project, including any dependencies or prerequisites.
5. Document API Endpoints
If your project includes an API, clearly document each endpoint, including its purpose, required parameters, and expected responses.
6. Use Code Examples
Illustrate key concepts and usage with relevant code snippets. For example:
// Example of how to use the calculateArea function
const radius = 5;
const area = calculateArea(radius);
console.log(`The area of a circle with radius ${radius} is ${area}`);
7. Explain Complex Algorithms
If your code includes complex algorithms, provide a high-level explanation of how they work and why certain decisions were made.
8. Document Function Parameters and Return Values
For each function, clearly state what parameters it accepts and what it returns. Include type information where relevant.
9. Use Consistent Formatting
Maintain a consistent style throughout your documentation. This includes consistent use of headings, code formatting, and overall layout.
10. Include a Table of Contents
For longer documentation, provide a table of contents to help readers navigate the document easily.
11. Version Your Documentation
Keep your documentation in sync with your code versions. Clearly indicate which version of the software the documentation applies to.
12. Use Diagrams and Flowcharts
Visual aids can greatly enhance understanding, especially for complex systems or processes. Include diagrams or flowcharts where appropriate.
13. Provide Usage Examples
Show how your code or library should be used in real-world scenarios. This helps developers understand how to integrate your work into their projects.
14. Document Error Handling
Explain how your code handles errors and exceptions, and provide guidance on how users should handle these situations.
15. Include Performance Considerations
If there are any performance implications or best practices for using your code efficiently, make sure to document these.
16. Link to External Resources
If your project relies on or integrates with other libraries or services, provide links to their documentation.
17. Use Comments Wisely
While inline comments are useful, don’t rely on them exclusively. Good documentation should stand on its own outside of the codebase.
18. Explain Configuration Options
If your project has configurable options, document what they are, their default values, and how to change them.
19. Include a Changelog
Maintain a changelog that documents the changes, additions, and fixes in each version of your software.
20. Document Known Limitations
Be upfront about any known limitations or edge cases in your code. This helps users avoid surprises and plan accordingly.
21. Provide Troubleshooting Guidance
Include a section on common issues and how to resolve them. This can save users (and you) a lot of time in the long run.
22. Use Markdown for Formatting
Markdown is a lightweight and easy-to-use syntax for styling text. It’s widely supported and makes your documentation more readable both in plain text and when rendered.
23. Include License Information
Clearly state the license under which your code is released. This is crucial for other developers to understand how they can use your work.
24. Document Testing Procedures
Explain how to run tests for your code and what the expected outcomes should be. This helps maintain code quality as the project evolves.
25. Use Consistent Terminology
Define key terms and use them consistently throughout your documentation to avoid confusion.
26. Provide a Quick Start Guide
Include a section that allows users to get up and running quickly with the most basic functionality of your project.
27. Document Security Considerations
If there are any security implications or best practices related to your code, make sure to document these clearly.
28. Use Tools for Documentation Generation
Consider using tools like JSDoc, Sphinx, or Doxygen to generate documentation from your code comments automatically.
29. Include Contribution Guidelines
If your project is open-source, provide clear guidelines on how others can contribute to the project.
30. Document Dependencies
List all external dependencies required by your project, including version numbers and installation instructions.
31. Use Code Highlighting
When including code snippets in your documentation, use syntax highlighting to improve readability. For example:
function calculateArea(radius) {
return Math.PI * radius * radius;
}
32. Provide Examples of Input and Output
For functions or methods, provide examples of expected input and corresponding output to clarify usage.
33. Document Design Decisions
Explain the rationale behind significant design decisions in your code. This helps others understand your thought process and the trade-offs made.
34. Use Consistent Naming Conventions
Use consistent naming conventions throughout your code and documentation. This makes it easier for readers to follow along.
35. Include a Glossary
For projects with domain-specific terminology, include a glossary to define key terms and concepts.
36. Document Environment Setup
Provide instructions on setting up the development environment, including any necessary tools or configurations.
37. Use Descriptive Commit Messages
While not strictly part of documentation, clear and descriptive commit messages can serve as a form of documentation for the project’s history.
38. Include Performance Benchmarks
If performance is a key feature of your code, include benchmarks and performance metrics in your documentation.
39. Document Coding Standards
If your project follows specific coding standards or style guides, document these for contributors to follow.
40. Use Task Lists
For ongoing development, include a task list or roadmap in your documentation to show planned features or improvements.
41. Provide Migration Guides
If there are breaking changes between versions, provide migration guides to help users update their implementations.
42. Use Badges
Include badges in your documentation to show the current status of your project, such as build status, test coverage, or version number.
43. Document Accessibility Features
If your project includes user interfaces, document any accessibility features and how to use them.
44. Include a FAQ Section
Anticipate common questions and include a Frequently Asked Questions (FAQ) section in your documentation.
45. Keep It Updated
Perhaps the most important tip: regularly review and update your documentation to ensure it remains accurate and relevant as your code evolves.
Conclusion
Writing effective documentation is a crucial skill for any developer, and it’s an integral part of the coding education we provide at AlgoCademy. By following these 45 tips, you’ll be well on your way to creating clear, comprehensive, and useful documentation for your code. Remember, good documentation not only helps others understand and use your work but also demonstrates your professionalism and attention to detail – qualities that are highly valued in the tech industry, especially when preparing for interviews with major tech companies.
As you continue to develop your coding skills, make documentation an essential part of your workflow. It will not only improve the quality of your projects but also enhance your ability to communicate complex technical concepts – a valuable skill for any aspiring software developer. Keep practicing, keep documenting, and watch your programming skills soar to new heights!