The Role of Algorithms in Everyday Problem Solving
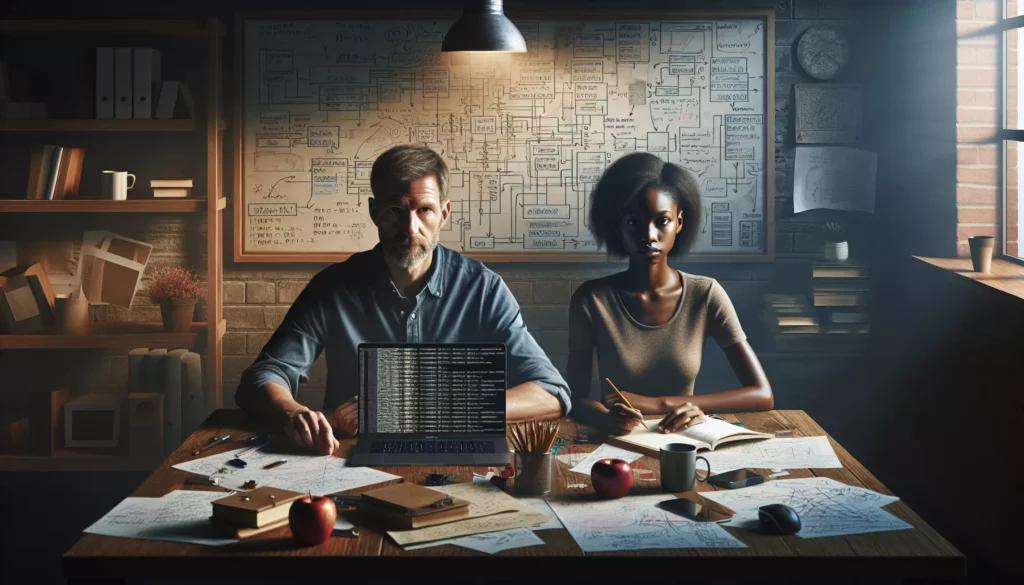
In today’s digital age, algorithms have become an integral part of our daily lives, often working behind the scenes to solve complex problems and make our experiences more efficient. While many people associate algorithms primarily with computer science and programming, their applications extend far beyond the realm of technology. In this comprehensive guide, we’ll explore the fascinating world of algorithms and how they play a crucial role in everyday problem-solving.
What Are Algorithms?
Before diving into the practical applications of algorithms, it’s essential to understand what they are. At its core, an algorithm is a step-by-step procedure or set of rules designed to solve a specific problem or accomplish a particular task. Think of it as a recipe for problem-solving, where each step is carefully defined and executed in a specific order to achieve the desired outcome.
Algorithms can be simple or complex, ranging from basic instructions for making a sandwich to intricate mathematical formulas used in advanced scientific research. The key characteristic of an algorithm is its ability to produce a consistent and predictable result when given the same input.
The Importance of Algorithmic Thinking
Algorithmic thinking is a problem-solving approach that involves breaking down complex problems into smaller, manageable steps. This skill is not only valuable in computer programming but also in various aspects of our daily lives. By developing algorithmic thinking skills, we can:
- Approach problems more systematically
- Identify patterns and relationships
- Create efficient solutions
- Improve decision-making processes
- Enhance logical reasoning abilities
Platforms like AlgoCademy recognize the importance of algorithmic thinking and provide interactive coding tutorials and resources to help individuals develop these crucial skills. Whether you’re a beginner or preparing for technical interviews at major tech companies, understanding algorithms is a fundamental aspect of programming and problem-solving.
Algorithms in Everyday Life
Now that we have a basic understanding of algorithms and their importance, let’s explore some common areas where algorithms play a significant role in our daily lives:
1. Navigation and Transportation
One of the most visible applications of algorithms in our daily lives is in navigation and transportation systems. GPS navigation apps like Google Maps and Waze use complex algorithms to:
- Calculate the shortest or fastest route between two points
- Predict traffic patterns and suggest alternative routes
- Estimate arrival times based on current conditions
- Optimize ride-sharing services and public transportation schedules
These algorithms process vast amounts of data, including real-time traffic information, historical patterns, and user-reported incidents, to provide accurate and efficient navigation solutions.
2. Social Media and Content Recommendations
Social media platforms and content streaming services rely heavily on algorithms to personalize user experiences and keep users engaged. These recommendation algorithms analyze user behavior, preferences, and interactions to:
- Curate personalized news feeds and content suggestions
- Recommend friends or connections
- Serve targeted advertisements
- Suggest movies, TV shows, or music based on viewing history
For example, Netflix uses sophisticated algorithms to analyze your viewing habits and recommend shows you’re likely to enjoy, while Facebook’s News Feed algorithm determines which posts appear at the top of your feed based on your interactions and interests.
3. Online Shopping and E-commerce
E-commerce platforms leverage algorithms to enhance the shopping experience and increase sales. These algorithms are responsible for:
- Product recommendations based on browsing and purchase history
- Dynamic pricing strategies
- Inventory management and demand forecasting
- Fraud detection and prevention
- Optimizing search results and product rankings
Amazon, for instance, uses sophisticated recommendation algorithms to suggest products you might be interested in, based on your browsing history, purchases, and the behavior of similar customers.
4. Financial Services and Banking
The financial sector relies heavily on algorithms for various operations, including:
- Credit scoring and loan approval processes
- Fraud detection and prevention in transactions
- Algorithmic trading in stock markets
- Risk assessment and insurance pricing
- Personal finance management and budgeting apps
These algorithms analyze vast amounts of financial data to make quick decisions, detect anomalies, and provide personalized financial advice to customers.
5. Healthcare and Medical Diagnosis
In the healthcare industry, algorithms are increasingly being used to improve patient care and assist medical professionals. Some applications include:
- Medical image analysis for early disease detection
- Predictive analytics for patient outcomes
- Drug discovery and development
- Personalized treatment recommendations
- Hospital resource management and scheduling
Machine learning algorithms, in particular, have shown promising results in analyzing medical data and assisting in diagnoses, sometimes even outperforming human experts in specific tasks.
Basic Types of Algorithms
To better understand how algorithms work in everyday problem-solving, let’s explore some fundamental types of algorithms:
1. Search Algorithms
Search algorithms are designed to find specific items or information within a larger dataset. Common examples include:
- Linear search: Sequentially checking each element in a list
- Binary search: Efficiently searching a sorted list by repeatedly dividing the search interval in half
- Depth-first search and Breadth-first search: Used for traversing or searching tree or graph data structures
These algorithms are crucial in various applications, from finding items in a database to navigating through file systems.
2. Sorting Algorithms
Sorting algorithms arrange data in a specific order, typically ascending or descending. Some popular sorting algorithms include:
- Bubble sort: Simple but inefficient for large datasets
- Quicksort: Efficient for large datasets, uses a divide-and-conquer approach
- Merge sort: Stable and efficient, also uses divide-and-conquer
- Insertion sort: Efficient for small datasets or partially sorted data
Sorting algorithms are essential in many applications, from organizing contact lists to optimizing database queries.
3. Greedy Algorithms
Greedy algorithms make locally optimal choices at each step with the hope of finding a global optimum. They are often used in optimization problems, such as:
- Dijkstra’s algorithm for finding the shortest path in a graph
- Huffman coding for data compression
- Kruskal’s algorithm for finding minimum spanning trees
While greedy algorithms don’t always produce the best solution, they are often efficient and provide good approximations for complex problems.
4. Dynamic Programming
Dynamic programming is a technique for solving complex problems by breaking them down into simpler subproblems. It’s particularly useful for optimization problems and is applied in various fields, including:
- Sequence alignment in bioinformatics
- Resource allocation in economics
- Shortest path problems in computer networks
- Optimal scheduling and planning
Dynamic programming often provides more efficient solutions compared to naive recursive approaches for problems with overlapping subproblems.
5. Machine Learning Algorithms
Machine learning algorithms are a subset of artificial intelligence that enable systems to learn and improve from experience without being explicitly programmed. Common types include:
- Supervised learning: Classification and regression algorithms
- Unsupervised learning: Clustering and dimensionality reduction algorithms
- Reinforcement learning: Algorithms that learn through interaction with an environment
These algorithms are at the forefront of many technological advancements, from voice recognition systems to autonomous vehicles.
Implementing Algorithms in Programming
For those interested in coding and software development, understanding how to implement algorithms is crucial. Here’s a simple example of a binary search algorithm implemented in Python:
def binary_search(arr, target):
left = 0
right = len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
# Example usage
sorted_array = [1, 3, 5, 7, 9, 11, 13, 15, 17]
target = 7
result = binary_search(sorted_array, target)
if result != -1:
print(f"Element {target} found at index {result}")
else:
print(f"Element {target} not found in the array")
This implementation demonstrates how a binary search algorithm can efficiently find a target value in a sorted array. Understanding and implementing such algorithms is a key focus of coding education platforms like AlgoCademy, which provide interactive tutorials and resources for learners to practice and improve their algorithmic skills.
The Future of Algorithms in Problem-Solving
As technology continues to advance, the role of algorithms in everyday problem-solving is only expected to grow. Some emerging trends and future applications include:
1. Artificial Intelligence and Advanced Machine Learning
AI and machine learning algorithms are becoming increasingly sophisticated, enabling more complex problem-solving capabilities. Future applications may include:
- More accurate and personalized healthcare diagnostics
- Advanced natural language processing for human-computer interaction
- Improved autonomous systems for transportation and robotics
- Enhanced predictive analytics for business decision-making
2. Quantum Algorithms
As quantum computing technology develops, new algorithms designed to harness the power of quantum systems are emerging. These algorithms have the potential to solve certain problems exponentially faster than classical algorithms, with applications in:
- Cryptography and cybersecurity
- Drug discovery and materials science
- Financial modeling and optimization
- Climate modeling and weather prediction
3. Edge Computing and IoT Algorithms
With the growth of the Internet of Things (IoT) and edge computing, algorithms are being developed to process data closer to its source, enabling:
- Real-time decision-making in smart cities and industrial settings
- Improved energy efficiency in smart homes and buildings
- Enhanced privacy and security for personal devices
- More efficient data processing for autonomous vehicles
4. Ethical and Explainable Algorithms
As algorithms play an increasingly significant role in decision-making processes, there’s a growing focus on developing ethical and explainable algorithms. This includes:
- Algorithms designed to be transparent and interpretable
- Fairness-aware machine learning to reduce bias in automated decisions
- Privacy-preserving algorithms for sensitive data processing
- Robust and reliable algorithms for critical systems
Conclusion
Algorithms have become an indispensable part of our daily lives, silently working behind the scenes to solve complex problems and enhance our experiences across various domains. From the moment we wake up and check our personalized news feed to navigating through traffic and making online purchases, algorithms are constantly at work, processing vast amounts of data and making decisions in fractions of a second.
Understanding the role of algorithms in everyday problem-solving not only helps us appreciate the technology around us but also empowers us to think more critically and systematically about the challenges we face. By developing algorithmic thinking skills, we can approach problems more efficiently and create innovative solutions in both our personal and professional lives.
As we look to the future, the importance of algorithms in problem-solving will only continue to grow. Emerging technologies like artificial intelligence, quantum computing, and edge computing promise to unlock new possibilities and tackle even more complex challenges. At the same time, the development of ethical and explainable algorithms will be crucial in ensuring that these powerful tools are used responsibly and for the benefit of society as a whole.
For those interested in delving deeper into the world of algorithms and coding, platforms like AlgoCademy offer valuable resources and interactive tutorials to help you develop your skills. Whether you’re a beginner looking to start your coding journey or an experienced programmer preparing for technical interviews at top tech companies, understanding algorithms is a fundamental step towards becoming a proficient problem-solver in the digital age.
As we continue to rely on algorithms to navigate the complexities of modern life, it’s clear that their role in everyday problem-solving will only become more significant. By embracing algorithmic thinking and staying informed about the latest developments in this field, we can better prepare ourselves for the challenges and opportunities that lie ahead in our increasingly algorithmic world.