The Ethics of Software Development: Writing Code Responsibly
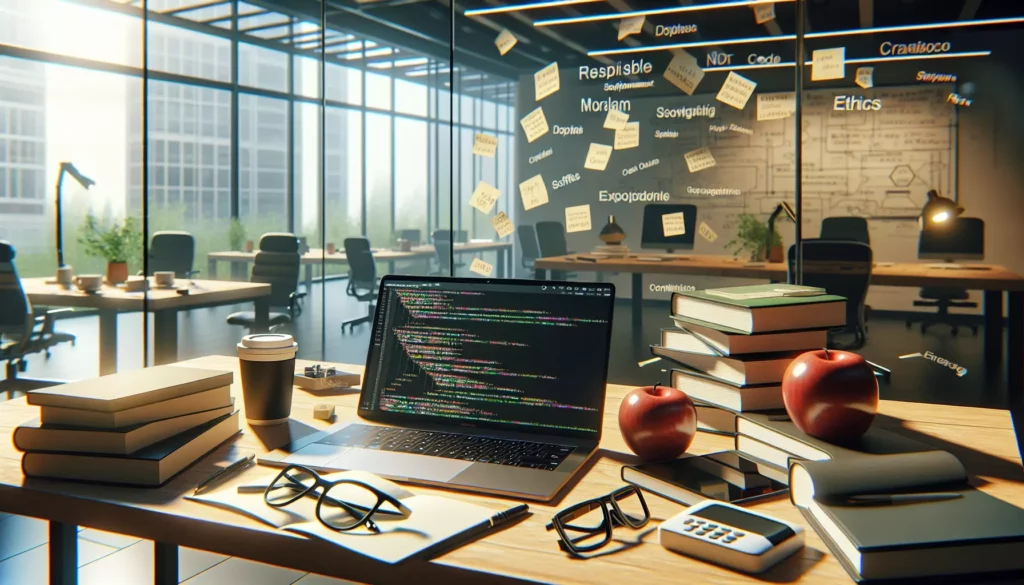
In today’s digital age, software development has become an integral part of our lives, powering everything from smartphones to complex systems that manage critical infrastructure. As the influence of technology continues to grow, so does the responsibility of software developers. The ethics of software development is a crucial topic that every programmer, from beginners to seasoned professionals, must consider. This article will explore the various ethical considerations in coding, the importance of responsible development practices, and how to navigate the complex landscape of software ethics.
Understanding the Importance of Ethics in Software Development
Ethics in software development refers to the moral principles and values that guide the creation, implementation, and maintenance of software systems. It encompasses a wide range of considerations, including privacy, security, accessibility, and social impact. As software increasingly shapes our world, developers must recognize their role in creating technologies that can significantly affect individuals and society as a whole.
The importance of ethics in software development cannot be overstated. Here are some key reasons why ethical considerations are crucial:
- Trust and Reputation: Ethical practices build trust with users and stakeholders, enhancing the reputation of both the developer and the organization.
- Legal Compliance: Many ethical considerations align with legal requirements, helping developers avoid potential legal issues.
- Social Responsibility: Developers have a duty to create software that benefits society and minimizes harm.
- Long-term Sustainability: Ethical software is more likely to be sustainable and successful in the long run.
- Personal Integrity: Adhering to ethical principles allows developers to maintain their personal and professional integrity.
Key Ethical Considerations in Software Development
To write code responsibly, developers must be aware of various ethical considerations. Let’s explore some of the most critical areas:
1. Privacy and Data Protection
In an era of big data and constant connectivity, protecting user privacy is paramount. Developers must consider:
- Data Collection: Only collect necessary data and be transparent about what is being collected.
- Data Storage: Implement robust security measures to protect stored data.
- Data Usage: Use data only for its intended purpose and obtain user consent for any additional uses.
- Data Sharing: Be cautious about sharing user data with third parties and ensure proper safeguards are in place.
Example of responsible data handling in code:
// Encrypt sensitive user data before storing
function encryptUserData(userData) {
const encryptionKey = process.env.ENCRYPTION_KEY;
const cipher = crypto.createCipher('aes-256-cbc', encryptionKey);
let encryptedData = cipher.update(JSON.stringify(userData), 'utf8', 'hex');
encryptedData += cipher.final('hex');
return encryptedData;
}
// Only store necessary user information
const userDataToStore = {
id: user.id,
email: user.email,
// Avoid storing sensitive information like passwords or full addresses
};
const encryptedUserData = encryptUserData(userDataToStore);
database.storeUser(encryptedUserData);
2. Security and System Integrity
Ensuring the security of software systems is a fundamental ethical responsibility. This includes:
- Vulnerability Management: Regularly assess and address security vulnerabilities.
- Authentication and Authorization: Implement strong authentication mechanisms and proper access controls.
- Secure Coding Practices: Follow best practices to prevent common security issues like SQL injection or cross-site scripting (XSS).
- Incident Response: Have a plan in place to respond to security breaches quickly and effectively.
Example of implementing secure authentication:
const bcrypt = require('bcrypt');
async function hashPassword(password) {
const saltRounds = 10;
return await bcrypt.hash(password, saltRounds);
}
async function verifyPassword(password, hashedPassword) {
return await bcrypt.compare(password, hashedPassword);
}
// Usage
async function registerUser(username, password) {
const hashedPassword = await hashPassword(password);
// Store username and hashedPassword in the database
}
async function loginUser(username, password) {
const user = await database.getUser(username);
if (user && await verifyPassword(password, user.hashedPassword)) {
// Login successful
} else {
// Login failed
}
}
3. Accessibility and Inclusivity
Ethical software development means creating products that are accessible to all users, including those with disabilities. Considerations include:
- Compliance with Accessibility Standards: Follow guidelines like WCAG (Web Content Accessibility Guidelines).
- Inclusive Design: Design interfaces that cater to diverse user needs and abilities.
- Assistive Technology Compatibility: Ensure compatibility with screen readers and other assistive technologies.
- Language and Cultural Sensitivity: Consider internationalization and localization to make software inclusive for users from different backgrounds.
Example of improving accessibility in web development:
<!-- Use semantic HTML elements -->
<nav aria-label="Main Navigation">
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
<!-- Provide alternative text for images -->
<img src="logo.png" alt="Company Logo">
<!-- Ensure proper color contrast -->
<style>
body {
color: #333;
background-color: #fff;
}
</style>
<!-- Use ARIA attributes when necessary -->
<button aria-label="Close dialog" onclick="closeDialog()">X</button>
4. Algorithmic Fairness and Bias
As artificial intelligence and machine learning become more prevalent, ensuring algorithmic fairness is crucial. Developers must consider:
- Bias in Training Data: Recognize and mitigate biases in the data used to train AI models.
- Fairness in Decision-Making: Ensure that algorithms do not discriminate against protected groups.
- Transparency: Strive for explainable AI and provide insights into how decisions are made.
- Regular Audits: Continuously monitor and evaluate algorithmic outcomes for potential biases.
Example of addressing bias in a machine learning model:
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.linear_model import LogisticRegression
from aif360.datasets import BinaryLabelDataset
from aif360.metrics import BinaryLabelDatasetMetric
from aif360.algorithms.preprocessing import Reweighing
# Load and preprocess the data
data = pd.read_csv('employment_data.csv')
X = data.drop(['employed', 'gender'], axis=1)
y = data['employed']
protected_attribute = data['gender']
# Create a BinaryLabelDataset
bld = BinaryLabelDataset(df=data, label_names=['employed'], protected_attribute_names=['gender'])
# Check for bias
metric = BinaryLabelDatasetMetric(bld, unprivileged_groups=[{'gender': 0}], privileged_groups=[{'gender': 1}])
print(f"Disparate impact before mitigation: {metric.disparate_impact()}")
# Apply bias mitigation technique (Reweighing)
rw = Reweighing(unprivileged_groups=[{'gender': 0}], privileged_groups=[{'gender': 1}])
bld_transformed = rw.fit_transform(bld)
# Check for bias after mitigation
metric_transformed = BinaryLabelDatasetMetric(bld_transformed, unprivileged_groups=[{'gender': 0}], privileged_groups=[{'gender': 1}])
print(f"Disparate impact after mitigation: {metric_transformed.disparate_impact()}")
# Proceed with model training using the transformed dataset
5. Environmental Impact
The environmental footprint of software is often overlooked, but it’s an important ethical consideration. Developers should focus on:
- Energy Efficiency: Optimize code to reduce energy consumption and carbon footprint.
- Resource Management: Design systems that use computational resources efficiently.
- Sustainable Practices: Consider the lifecycle of hardware and promote sustainable IT practices.
- Green Hosting: Choose environmentally friendly hosting solutions when deploying applications.
Example of optimizing code for energy efficiency:
// Inefficient code
function calculateSum(numbers) {
return numbers.reduce((sum, num) => sum + num, 0);
}
// More efficient code
function calculateSumEfficient(numbers) {
let sum = 0;
for (let i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
return sum;
}
// Usage
const largeArray = Array.from({ length: 1000000 }, () => Math.random());
console.time('Inefficient');
calculateSum(largeArray);
console.timeEnd('Inefficient');
console.time('Efficient');
calculateSumEfficient(largeArray);
console.timeEnd('Efficient');
Ethical Frameworks and Guidelines for Software Development
To help navigate the complex ethical landscape of software development, several frameworks and guidelines have been established. Some of the most prominent include:
1. ACM Code of Ethics and Professional Conduct
The Association for Computing Machinery (ACM) provides a comprehensive code of ethics for computing professionals. It covers principles such as:
- Contribute to society and human well-being
- Avoid harm
- Be honest and trustworthy
- Respect privacy
- Honor confidentiality
2. IEEE Code of Ethics
The Institute of Electrical and Electronics Engineers (IEEE) offers a code of ethics that emphasizes:
- Holding paramount the safety, health, and welfare of the public
- Disclosing promptly factors that might endanger the public or the environment
- Avoiding real or perceived conflicts of interest
- Rejecting bribery in all its forms
3. The Software Engineering Code of Ethics and Professional Practice
Jointly developed by the ACM and IEEE Computer Society, this code provides specific guidance for software engineers, including:
- Work in the public interest
- Act in a manner that is in the best interests of their client and employer
- Ensure that their products meet the highest professional standards possible
- Maintain integrity and independence in their professional judgment
4. Ethical OS Toolkit
Developed by the Institute for the Future and Omidyar Network, the Ethical OS Toolkit provides a framework for anticipating the long-term impacts of technology products. It includes:
- Risk zones to consider (e.g., truth, disinformation, and propaganda)
- Future scenarios to contemplate
- Strategies for mitigating ethical risks
Implementing Ethical Practices in Software Development
Knowing about ethical considerations is just the first step. Implementing them in day-to-day development practices is crucial. Here are some strategies for writing code responsibly:
1. Incorporate Ethics into the Software Development Lifecycle
Ethical considerations should be part of every stage of development:
- Requirements Gathering: Include ethical requirements alongside functional ones.
- Design: Consider the ethical implications of design decisions.
- Implementation: Follow secure coding practices and ethical guidelines.
- Testing: Include tests for ethical compliance, such as privacy and accessibility checks.
- Deployment: Ensure ethical considerations are maintained in the production environment.
- Maintenance: Regularly review and update systems to address evolving ethical concerns.
2. Conduct Regular Ethical Audits
Periodically assess your software and development practices for ethical compliance:
- Use automated tools to check for accessibility issues and security vulnerabilities.
- Perform manual reviews to identify potential ethical concerns.
- Seek feedback from diverse user groups to uncover unforeseen ethical issues.
3. Foster a Culture of Ethical Awareness
Create an environment where ethical considerations are valued and discussed:
- Provide ethics training for all team members.
- Encourage open discussions about ethical dilemmas.
- Recognize and reward ethical decision-making.
4. Collaborate with Ethicists and Domain Experts
For complex ethical issues, consider partnering with experts:
- Engage ethicists to help navigate challenging moral questions.
- Consult domain experts to understand the specific ethical concerns in different fields (e.g., healthcare, finance).
5. Implement Ethical Review Processes
Establish formal procedures for ethical review:
- Create an ethics review board for significant projects or features.
- Include ethical considerations in code reviews and project approvals.
- Develop checklists for common ethical issues to ensure they’re consistently addressed.
Case Studies: Ethical Challenges in Software Development
To better understand the practical implications of ethical software development, let’s examine a few real-world case studies:
Case Study 1: Facial Recognition Technology
A software company develops facial recognition technology for law enforcement agencies. While the technology could help solve crimes, it raises significant ethical concerns:
- Privacy: The system collects and processes biometric data of individuals without their explicit consent.
- Bias: Initial tests show the system is less accurate for certain racial groups, potentially leading to false identifications.
- Surveillance: There are concerns about the technology being used for mass surveillance, infringing on civil liberties.
Ethical Considerations:
- How can the developers ensure the technology is used responsibly and not for unethical purposes?
- What steps should be taken to address and mitigate bias in the system?
- Should there be limits on data collection and retention to protect privacy?
Case Study 2: Social Media Algorithm
A social media platform’s recommendation algorithm is designed to maximize user engagement. However, it leads to some unintended consequences:
- Echo Chambers: Users are increasingly shown content that aligns with their existing views, potentially reinforcing biases.
- Misinformation: The algorithm sometimes promotes sensational but inaccurate content due to high engagement rates.
- Addiction: Some users report addictive behavior patterns, spending excessive time on the platform.
Ethical Considerations:
- How can the algorithm be adjusted to balance engagement with exposure to diverse viewpoints?
- What responsibility do the developers have in combating misinformation?
- Should features be implemented to help users manage their screen time and digital well-being?
Case Study 3: Autonomous Vehicle Decision-Making
A team is developing the decision-making algorithms for autonomous vehicles. They encounter challenging ethical scenarios:
- Trolley Problem: In unavoidable accident scenarios, how should the vehicle prioritize different lives (passengers vs. pedestrians, young vs. old, etc.)?
- Data Privacy: The vehicle collects vast amounts of data about passengers and their movements.
- Transparency: Users want to understand how the vehicle makes decisions, but the algorithms are complex and not easily explainable.
Ethical Considerations:
- How should ethical principles be encoded into the decision-making algorithms?
- What level of transparency should be provided to users about the vehicle’s decision-making process?
- How can user privacy be protected while still collecting necessary data for safe operation?
The Future of Ethical Software Development
As technology continues to advance, the ethical challenges in software development will evolve and new ones will emerge. Some areas that are likely to require increased ethical consideration in the future include:
1. Artificial Intelligence and Machine Learning
As AI systems become more sophisticated and autonomous, ethical considerations will become even more critical:
- Ensuring AI decision-making is fair, transparent, and accountable
- Addressing the potential impact of AI on employment and social structures
- Developing AI systems that align with human values and ethics
2. Internet of Things (IoT) and Ubiquitous Computing
As computing becomes more pervasive in our daily lives, new ethical challenges will arise:
- Balancing convenience with privacy in smart home and city environments
- Ensuring the security of interconnected devices to prevent widespread vulnerabilities
- Addressing the environmental impact of proliferating electronic devices
3. Quantum Computing
The advent of quantum computing will bring new ethical considerations:
- Ensuring equitable access to quantum computing resources
- Addressing the potential for quantum computers to break current encryption methods
- Considering the ethical implications of quantum simulations in fields like drug discovery and climate modeling
4. Brain-Computer Interfaces
As technology begins to interface directly with the human brain, new ethical frontiers will open:
- Protecting the privacy of thoughts and neural data
- Ensuring equitable access to cognitive enhancement technologies
- Addressing the potential for manipulation or control through brain interfaces
Conclusion
The ethics of software development is a complex and evolving field that requires ongoing attention and commitment from developers, organizations, and society as a whole. By understanding the key ethical considerations, following established guidelines, and implementing responsible development practices, we can create software that not only functions well but also contributes positively to society.
As software continues to shape our world, the responsibility of developers grows. It’s crucial for every programmer, from those just starting their journey to seasoned professionals, to consider the ethical implications of their work. By doing so, we can ensure that technology serves as a force for good, enhancing human capabilities while respecting fundamental rights and values.
Remember, ethical software development is not just about following rules or avoiding negative consequences. It’s about actively striving to create technology that improves lives, promotes fairness, and contributes to a better world. As you write code, always ask yourself: “Am I developing this responsibly? What impact will this have on users and society?” By keeping these questions at the forefront of your mind, you’ll be well on your way to becoming not just a skilled programmer, but an ethical one as well.
The future of technology is in our hands. Let’s build it responsibly, ethically, and with a commitment to the greater good.