How to Make the Most of Pair Programming Sessions: A Comprehensive Guide
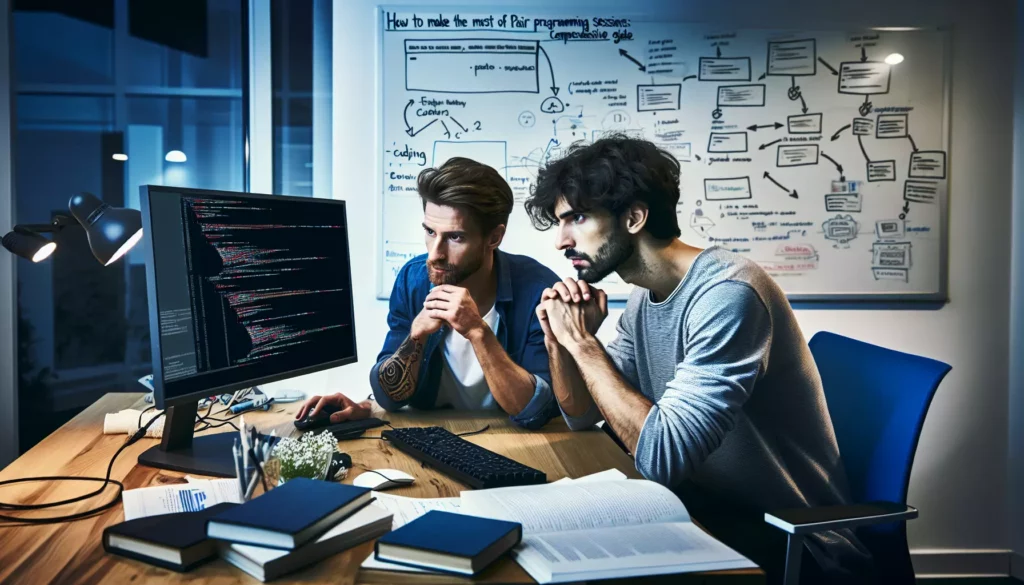
Pair programming is a collaborative approach to software development where two programmers work together at a single workstation. This technique has gained popularity in recent years due to its potential to improve code quality, knowledge sharing, and team dynamics. In this comprehensive guide, we’ll explore how to make the most of pair programming sessions, covering everything from preparation to execution and follow-up.
Table of Contents
- Understanding Pair Programming
- Benefits of Pair Programming
- Preparing for Pair Programming
- Roles in Pair Programming
- Effective Communication During Sessions
- Best Practices for Successful Pair Programming
- Common Challenges and How to Overcome Them
- Tools for Remote Pair Programming
- Measuring the Success of Pair Programming
- Pair Programming in Technical Interviews
- Conclusion
Understanding Pair Programming
Pair programming is a software development technique where two programmers work together at one workstation. Typically, one programmer, known as the “driver,” writes the code, while the other, known as the “navigator,” reviews each line of code as it’s typed. The two programmers switch roles frequently, usually every 30 minutes to an hour.
This approach is not just about two people working on the same piece of code; it’s about collaboration, constant communication, and shared responsibility for the quality of the work produced.
Benefits of Pair Programming
Pair programming offers numerous benefits that can significantly improve the software development process:
- Improved Code Quality: With two sets of eyes on the code, errors are caught earlier, leading to fewer bugs and higher-quality code.
- Knowledge Sharing: Developers can learn from each other’s techniques, tricks, and problem-solving approaches.
- Enhanced Focus: Working with a partner can help maintain concentration and reduce distractions.
- Faster Problem Solving: Two minds working together can often solve complex problems more quickly than one working alone.
- Better Team Dynamics: Pair programming fosters communication and collaboration within the team.
- Onboarding New Team Members: It’s an excellent way to get new team members up to speed on the codebase and team practices.
Preparing for Pair Programming
Proper preparation is key to a successful pair programming session. Here are some steps to ensure you’re ready:
- Set Clear Objectives: Define what you want to achieve during the session. Is it to complete a specific feature, debug a problem, or review code?
- Choose the Right Partner: If possible, pair with someone who complements your skills. A mix of experience levels can be beneficial.
- Prepare Your Environment: Ensure your development environment is set up correctly. This includes having the necessary tools, access to repositories, and a comfortable workspace.
- Review Relevant Materials: If you’re working on an existing project, review any relevant documentation or previous code to familiarize yourself with the context.
- Agree on Coding Standards: Discuss and agree on coding standards and practices before you begin.
- Schedule Breaks: Plan for regular breaks to maintain focus and prevent burnout.
Roles in Pair Programming
In pair programming, there are typically two roles: the driver and the navigator. Understanding these roles is crucial for an effective session.
The Driver
The driver is responsible for:
- Typing the actual code
- Focusing on the immediate task of implementing the current piece of functionality
- Explaining their thought process as they code
The Navigator
The navigator’s responsibilities include:
- Reviewing the code as it’s written
- Thinking about the bigger picture and how the current code fits into the overall architecture
- Spotting potential issues or bugs
- Suggesting improvements or alternative approaches
- Researching solutions to problems that arise
It’s important to switch roles regularly to maintain engagement and share the cognitive load.
Effective Communication During Sessions
Communication is the cornerstone of successful pair programming. Here are some tips for effective communication:
- Be Clear and Concise: Express your ideas clearly and avoid unnecessary jargon.
- Ask Questions: Don’t hesitate to ask for clarification if you don’t understand something.
- Provide Constructive Feedback: When suggesting improvements, be specific and focus on the code, not the person.
- Listen Actively: Pay attention to your partner’s ideas and concerns.
- Think Aloud: Verbalize your thought process to keep your partner engaged and aligned.
- Be Patient: Remember that everyone has different skill levels and ways of thinking.
Best Practices for Successful Pair Programming
To get the most out of your pair programming sessions, consider the following best practices:
- Rotate Pairs Regularly: This helps spread knowledge across the team and prevents the formation of knowledge silos.
- Take Regular Breaks: Short breaks every 45-90 minutes can help maintain focus and prevent fatigue.
- Use a Timer for Role Switching: This ensures both partners get equal time in each role.
- Practice Active Collaboration: Engage in discussions, brainstorming, and problem-solving together.
- Embrace Different Perspectives: Be open to your partner’s ideas and approaches, even if they differ from yours.
- Document Decisions and Learnings: Keep notes on important decisions and new things you learn during the session.
- Review and Refactor: Regularly step back to review your work and refactor when necessary.
- Celebrate Successes: Acknowledge when you’ve solved a difficult problem or completed a challenging task.
Common Challenges and How to Overcome Them
While pair programming can be highly effective, it’s not without its challenges. Here are some common issues and strategies to address them:
1. Skill Disparity
Challenge: One programmer may be significantly more experienced than the other.
Solution: View this as a learning opportunity. The more experienced programmer can mentor the less experienced one, while the latter can bring fresh perspectives and ask important questions.
2. Personality Conflicts
Challenge: Different personalities or working styles may clash.
Solution: Establish ground rules at the beginning of the session. Focus on mutual respect and open communication. If conflicts persist, consider changing pairs.
3. Distractions
Challenge: External interruptions or off-topic conversations can derail the session.
Solution: Create a dedicated space for pair programming. Use “Do Not Disturb” signs or status indicators. Agree to stay focused on the task at hand.
4. Unequal Participation
Challenge: One person dominates the session, either as the driver or navigator.
Solution: Use a timer to ensure regular role switching. Encourage the quieter partner to voice their thoughts and ideas.
5. Fatigue
Challenge: Extended pair programming sessions can be mentally exhausting.
Solution: Take regular breaks. Limit pair programming to 4-6 hours per day. Mix it with solo work or other activities.
Tools for Remote Pair Programming
With the rise of remote work, many teams are adopting remote pair programming. Here are some tools that can facilitate this:
- Visual Studio Code Live Share: Allows real-time collaborative editing and debugging.
- GitDuck: Combines code sharing with video chat for a more immersive experience.
- Tuple: A macOS app designed specifically for remote pair programming.
- Zoom or Google Meet: For video conferencing and screen sharing.
- Discord: Offers voice chat and screen sharing, popular among developers.
When using these tools, ensure you have a stable internet connection and test the setup before your session to avoid technical difficulties.
Measuring the Success of Pair Programming
To determine if pair programming is beneficial for your team, consider measuring the following metrics:
- Code Quality: Track the number of bugs found in code produced through pair programming versus solo programming.
- Development Speed: Compare the time taken to complete tasks with and without pair programming.
- Knowledge Sharing: Survey team members to assess how much they’ve learned from pair programming sessions.
- Team Satisfaction: Gauge team members’ satisfaction and engagement levels when participating in pair programming.
- Code Review Efficiency: Measure if pair-programmed code requires less time in code reviews.
Remember that the benefits of pair programming may not be immediately apparent. Give it time and consistently gather feedback to make informed decisions about its effectiveness for your team.
Pair Programming in Technical Interviews
Many companies, especially in the tech industry, use pair programming as part of their interview process. If you’re preparing for technical interviews, especially for FAANG (Facebook, Amazon, Apple, Netflix, Google) companies, it’s crucial to be comfortable with pair programming. Here are some tips:
- Practice Regularly: Use platforms like LeetCode or HackerRank to practice coding problems with a partner.
- Communicate Clearly: Explain your thought process out loud as you work through problems.
- Be Open to Feedback: Listen to your interviewer’s suggestions and be willing to change your approach if needed.
- Ask Questions: Don’t hesitate to seek clarification on the problem or requirements.
- Stay Calm: Remember that the interviewer is assessing your problem-solving skills and ability to collaborate, not just your coding speed.
Here’s a simple example of how a pair programming interview question might look:
Interviewer: "Let's write a function that finds the first non-repeating character in a string. How would you approach this?"
You: "Okay, let's think about this step by step. We need to count the occurrences of each character and then find the first one that occurs only once. We could use a hash map to store the character counts. Does that sound like a good approach?"
Interviewer: "That sounds reasonable. Why don't you start implementing it, and we'll discuss as we go along?"
You: "Sure, I'll start by creating a hash map to store the character counts."
// You would then start writing the code, explaining your thought process as you go.
Conclusion
Pair programming is a powerful technique that can significantly enhance code quality, knowledge sharing, and team collaboration when done correctly. By understanding the roles, communicating effectively, following best practices, and addressing common challenges, you can make the most of your pair programming sessions.
Remember that like any skill, effective pair programming takes practice. Be patient with yourself and your partners as you develop this skill. Over time, you’ll likely find that pair programming not only improves your code but also makes you a better communicator and collaborator.
Whether you’re preparing for technical interviews or looking to improve your day-to-day development practices, mastering pair programming can give you a significant advantage in your programming career. So, grab a partner, set up your environment, and start coding together!