How to Handle Graph Component Problems: A Comprehensive Guide
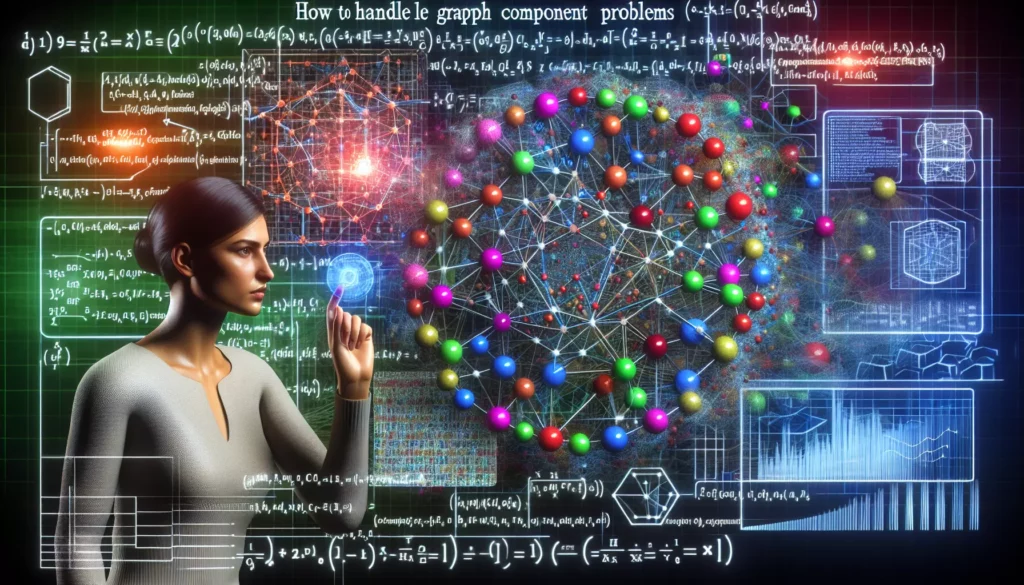
In the world of computer science and algorithmic problem-solving, graph problems are a crucial area that often appears in technical interviews, especially for positions at major tech companies. Understanding how to handle graph component problems is essential for any programmer looking to excel in their career. This comprehensive guide will walk you through the concept of graph components, common problems involving them, and effective strategies to solve these challenges.
What are Graph Components?
Before diving into specific problems and solutions, it’s important to understand what graph components are. In graph theory, a component (or connected component) of an undirected graph is a subgraph in which any two vertices are connected to each other by paths, and which is connected to no additional vertices in the supergraph.
In simpler terms, a component is a part of a graph where all nodes are connected to each other, either directly or indirectly, but are not connected to nodes in other components. Understanding components is crucial for solving many graph-related problems, as they often involve analyzing or manipulating these distinct parts of a graph.
Common Graph Component Problems
Let’s explore some common types of graph component problems you might encounter in coding interviews or real-world applications:
1. Counting Connected Components
One of the most fundamental graph component problems is simply counting the number of connected components in a graph. This problem tests your ability to traverse a graph and identify distinct groups of connected nodes.
2. Finding the Largest Component
This problem involves not just identifying components, but also determining which one contains the most nodes. It’s a step up from basic component counting and requires keeping track of component sizes.
3. Component Coloring
In this type of problem, you need to assign a unique “color” or identifier to each component in the graph. This can be useful in various applications, such as image segmentation or network analysis.
4. Connectivity Queries
These problems involve determining whether two nodes are in the same component. They often require efficient data structures to handle multiple queries quickly.
5. Adding Edges to Connect Components
This is a more advanced problem where you need to find the minimum number of edges to add to connect all components in a graph. It often involves concepts like minimum spanning trees.
Strategies for Solving Graph Component Problems
Now that we’ve identified common types of problems, let’s discuss strategies and algorithms you can use to solve them efficiently:
1. Depth-First Search (DFS)
DFS is a fundamental algorithm for traversing or searching graph structures. It’s particularly useful for identifying connected components.
Here’s a basic implementation of DFS in Python for finding connected components:
def dfs(graph, node, visited, component):
visited[node] = True
component.append(node)
for neighbor in graph[node]:
if not visited[neighbor]:
dfs(graph, neighbor, visited, component)
def find_components(graph):
visited = {node: False for node in graph}
components = []
for node in graph:
if not visited[node]:
component = []
dfs(graph, node, visited, component)
components.append(component)
return components
2. Breadth-First Search (BFS)
BFS is another graph traversal algorithm that can be used to find connected components. It’s particularly useful when you need to find the shortest path between nodes.
Here’s a BFS implementation for finding components:
from collections import deque
def bfs(graph, start, visited, component):
queue = deque([start])
visited[start] = True
component.append(start)
while queue:
node = queue.popleft()
for neighbor in graph[node]:
if not visited[neighbor]:
visited[neighbor] = True
component.append(neighbor)
queue.append(neighbor)
def find_components(graph):
visited = {node: False for node in graph}
components = []
for node in graph:
if not visited[node]:
component = []
bfs(graph, node, visited, component)
components.append(component)
return components
3. Union-Find (Disjoint Set) Data Structure
The Union-Find data structure is particularly useful for problems involving dynamic connectivity. It can efficiently handle operations like connecting two components and checking if two nodes are in the same component.
Here’s a basic implementation of Union-Find:
class UnionFind:
def __init__(self, n):
self.parent = list(range(n))
self.rank = [0] * n
def find(self, x):
if self.parent[x] != x:
self.parent[x] = self.find(self.parent[x])
return self.parent[x]
def union(self, x, y):
px, py = self.find(x), self.find(y)
if px == py:
return False
if self.rank[px] < self.rank[py]:
self.parent[px] = py
elif self.rank[px] > self.rank[py]:
self.parent[py] = px
else:
self.parent[py] = px
self.rank[px] += 1
return True
4. Tarjan’s Algorithm
Tarjan’s algorithm is an efficient graph algorithm for finding strongly connected components in a directed graph. While our focus is mainly on undirected graphs, it’s worth mentioning this algorithm for more advanced problems.
Solving Specific Graph Component Problems
Now, let’s apply these strategies to solve some of the common problems we identified earlier:
1. Counting Connected Components
To count connected components, we can use either DFS or BFS. The number of times we need to start a new traversal is equal to the number of components.
def count_components(graph):
visited = set()
count = 0
for node in graph:
if node not in visited:
dfs(graph, node, visited)
count += 1
return count
def dfs(graph, node, visited):
visited.add(node)
for neighbor in graph[node]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
2. Finding the Largest Component
To find the largest component, we can modify our DFS or BFS to keep track of component sizes:
def largest_component(graph):
visited = set()
max_size = 0
for node in graph:
if node not in visited:
size = dfs_size(graph, node, visited)
max_size = max(max_size, size)
return max_size
def dfs_size(graph, node, visited):
visited.add(node)
size = 1
for neighbor in graph[node]:
if neighbor not in visited:
size += dfs_size(graph, neighbor, visited)
return size
3. Component Coloring
We can use DFS or BFS to color each component with a unique identifier:
def color_components(graph):
colors = {}
color = 0
for node in graph:
if node not in colors:
dfs_color(graph, node, colors, color)
color += 1
return colors
def dfs_color(graph, node, colors, color):
colors[node] = color
for neighbor in graph[node]:
if neighbor not in colors:
dfs_color(graph, neighbor, colors, color)
4. Connectivity Queries
For efficient connectivity queries, Union-Find is an excellent choice:
def process_queries(graph, queries):
n = len(graph)
uf = UnionFind(n)
# Connect all edges in the graph
for node in graph:
for neighbor in graph[node]:
uf.union(node, neighbor)
# Process queries
results = []
for a, b in queries:
results.append(uf.find(a) == uf.find(b))
return results
5. Adding Edges to Connect Components
This problem is related to the minimum spanning tree concept. We can use a greedy approach with Union-Find:
def min_edges_to_connect(n, edges):
uf = UnionFind(n)
added_edges = 0
for a, b in edges:
if uf.union(a, b):
added_edges += 1
return n - 1 - added_edges # Minimum number of additional edges needed
Advanced Topics in Graph Component Problems
As you become more comfortable with basic graph component problems, you may encounter more advanced topics. Here are a few to be aware of:
1. Biconnected Components
Biconnected components are maximal biconnected subgraphs of a given graph. A graph is biconnected if it remains connected after removing any single vertex. Identifying biconnected components is crucial in network reliability analysis.
2. Strongly Connected Components
In directed graphs, strongly connected components are subgraphs where every vertex is reachable from every other vertex. Tarjan’s algorithm and Kosaraju’s algorithm are commonly used to find strongly connected components.
3. Dynamic Connectivity
Dynamic connectivity problems involve maintaining connected components in a graph that’s changing over time (with edges being added or removed). Advanced data structures like Euler Tour Trees can be used for these problems.
4. Graph Decomposition
Graph decomposition involves breaking down a graph into smaller, more manageable parts. Techniques like tree decomposition and modular decomposition fall under this category and are used in solving complex graph problems.
Real-World Applications of Graph Component Problems
Understanding graph components isn’t just an academic exercise. These concepts have numerous real-world applications:
1. Social Network Analysis
Identifying components in social networks can reveal distinct communities or groups of users. This is valuable for targeted marketing, recommendation systems, and understanding social dynamics.
2. Image Segmentation
In computer vision, graph component algorithms are used to segment images into distinct regions, which is crucial for object recognition and image understanding.
3. Network Reliability
In telecommunications and computer networks, component analysis helps identify critical points of failure and improve overall network reliability.
4. Biological Networks
In bioinformatics, graph components can represent functional modules in protein-protein interaction networks or metabolic pathways.
5. Transportation Systems
Component analysis in transportation networks can help optimize routes, identify isolated areas, and improve overall system connectivity.
Tips for Tackling Graph Component Problems in Interviews
When faced with a graph component problem in a coding interview, keep these tips in mind:
- Clarify the Problem: Make sure you understand whether the graph is directed or undirected, weighted or unweighted, and what specific operations are required.
- Choose the Right Algorithm: Based on the problem requirements, decide whether DFS, BFS, Union-Find, or another algorithm is most appropriate.
- Consider Edge Cases: Think about empty graphs, graphs with a single node, or fully connected graphs.
- Optimize for Space and Time: Be prepared to discuss the time and space complexity of your solution and potential optimizations.
- Practice Implementation: Be comfortable implementing graph representations (adjacency list or matrix) and traversal algorithms from scratch.
- Explain Your Approach: Clearly communicate your thought process, including why you chose a particular approach and any trade-offs involved.
Conclusion
Graph component problems are a fundamental part of computer science and appear frequently in technical interviews and real-world applications. By understanding the core concepts, mastering key algorithms like DFS, BFS, and Union-Find, and practicing a variety of problems, you’ll be well-equipped to handle graph component challenges in your coding career.
Remember, the key to mastering these problems is consistent practice and a deep understanding of the underlying principles. As you continue to work on graph problems, you’ll develop intuition for choosing the right approach and implementing efficient solutions.
Keep exploring, practicing, and pushing your boundaries in graph theory and algorithms. With dedication and the right approach, you’ll be well-prepared to tackle even the most challenging graph component problems in your coding interviews and beyond.