Mastering Stock Price Problems: Essential Techniques for Algorithmic Success
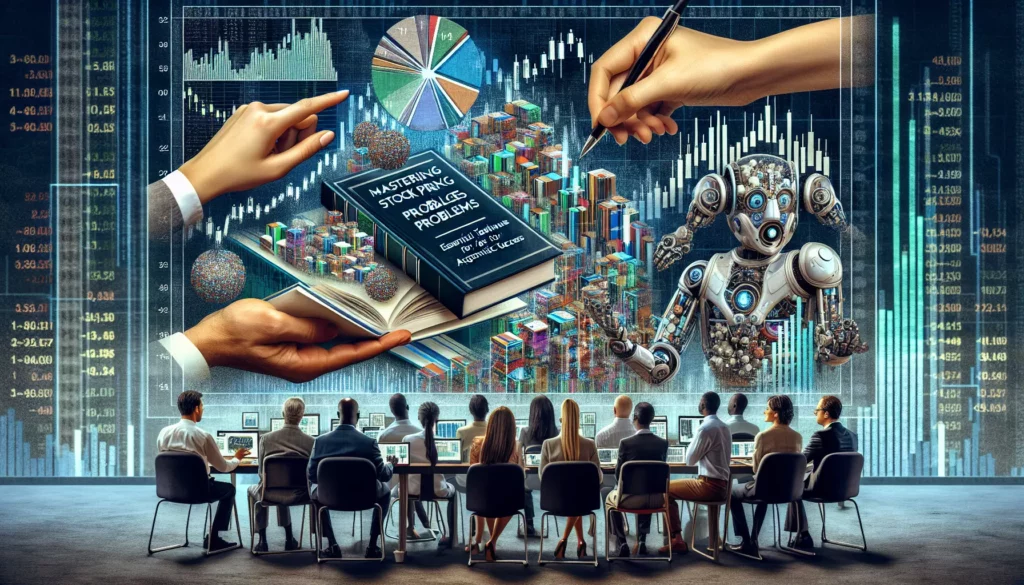
In the realm of coding interviews and algorithmic challenges, stock price problems stand out as a common and intriguing category. These problems not only test a programmer’s ability to manipulate arrays and implement efficient algorithms but also simulate real-world scenarios in financial markets. For aspiring software engineers, especially those aiming for positions at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google), mastering stock price problems is crucial.
This comprehensive guide will delve into various techniques for solving stock price problems, providing you with the tools and understanding necessary to tackle these challenges confidently. We’ll explore different problem types, analyze their complexities, and implement solutions using Python, a language favored by many for its readability and versatility in algorithmic problem-solving.
Understanding Stock Price Problems
Before diving into specific techniques, it’s essential to understand what stock price problems typically entail. These problems usually involve an array of integers representing stock prices over a period of time. The goal is often to determine the best time to buy and sell stocks to maximize profit, or to find patterns within the price data.
Common variations of stock price problems include:
- Finding the maximum profit from a single buy and sell transaction
- Determining the maximum profit with multiple buy and sell transactions
- Calculating profits with transaction fees or cooldown periods
- Identifying specific patterns in stock prices (e.g., peaks and valleys)
Let’s explore techniques to solve these problems efficiently.
Technique 1: One-Pass Approach
The one-pass approach is a fundamental technique for solving many stock price problems. It involves iterating through the array of prices once, keeping track of relevant information as we go.
Example: Best Time to Buy and Sell Stock
Problem: Given an array of stock prices, find the maximum profit you can achieve by buying on one day and selling on a different day in the future.
def max_profit(prices):
if not prices:
return 0
min_price = float('inf')
max_profit = 0
for price in prices:
if price < min_price:
min_price = price
else:
max_profit = max(max_profit, price - min_price)
return max_profit
# Example usage
prices = [7, 1, 5, 3, 6, 4]
print(max_profit(prices)) # Output: 5
In this solution, we use a single pass through the array. We keep track of the minimum price seen so far and update the maximum profit if we find a better selling opportunity. This approach has a time complexity of O(n) and space complexity of O(1), making it highly efficient.
Technique 2: Dynamic Programming
Dynamic Programming (DP) is a powerful technique for solving optimization problems. It’s particularly useful for stock price problems that involve multiple transactions or have additional constraints.
Example: Best Time to Buy and Sell Stock with Cooldown
Problem: Given an array of stock prices, find the maximum profit you can achieve. You may complete as many transactions as you like with the following restrictions: After you sell your stock, you cannot buy stock on the next day (i.e., cooldown one day).
def max_profit_with_cooldown(prices):
if not prices:
return 0
n = len(prices)
if n < 2:
return 0
# Initialize DP arrays
buy = [0] * n
sell = [0] * n
# Base cases
buy[0] = -prices[0]
buy[1] = max(-prices[0], -prices[1])
sell[1] = max(0, prices[1] - prices[0])
for i in range(2, n):
# Max profit if we buy on this day
buy[i] = max(buy[i-1], sell[i-2] - prices[i])
# Max profit if we sell on this day
sell[i] = max(sell[i-1], buy[i-1] + prices[i])
return sell[-1]
# Example usage
prices = [1, 2, 3, 0, 2]
print(max_profit_with_cooldown(prices)) # Output: 3
This DP solution uses two arrays: buy
and sell
. For each day, we calculate the maximum profit if we choose to buy or sell on that day, considering the cooldown restriction. The time and space complexity are both O(n).
Technique 3: State Machine Approach
The state machine approach is particularly useful for problems with complex constraints or multiple possible actions at each step. It involves modeling the problem as a set of states and transitions between them.
Example: Best Time to Buy and Sell Stock with Transaction Fee
Problem: You are given an array prices where prices[i] is the price of a given stock on the ith day, and an integer fee representing a transaction fee. Find the maximum profit you can achieve. You may complete as many transactions as you like, but you need to pay the transaction fee for each transaction.
def max_profit_with_fee(prices, fee):
if not prices:
return 0
n = len(prices)
# Initialize states
cash = 0
hold = -prices[0]
for i in range(1, n):
# Max profit if we have cash (not holding stock)
cash = max(cash, hold + prices[i] - fee)
# Max profit if we are holding stock
hold = max(hold, cash - prices[i])
return cash
# Example usage
prices = [1, 3, 2, 8, 4, 9]
fee = 2
print(max_profit_with_fee(prices, fee)) # Output: 8
In this solution, we use two states: cash
(not holding stock) and hold
(holding stock). At each step, we decide whether to maintain our current state or transition to the other state, always choosing the option that maximizes our profit. This approach has a time complexity of O(n) and space complexity of O(1).
Technique 4: Kadane’s Algorithm
Kadane’s Algorithm is primarily used for solving the maximum subarray problem, but it can be adapted for certain stock price problems, especially those involving cumulative gains or losses.
Example: Maximum Difference Between Increasing Elements
Problem: Given an array of integers, find the maximum difference between two elements such that the larger element appears after the smaller one.
def max_profit_kadane(prices):
if not prices or len(prices) < 2:
return 0
max_diff = 0
min_so_far = prices[0]
for price in prices[1:]:
if price > min_so_far:
max_diff = max(max_diff, price - min_so_far)
else:
min_so_far = price
return max_diff
# Example usage
prices = [7, 1, 5, 3, 6, 4]
print(max_profit_kadane(prices)) # Output: 5
This adaptation of Kadane’s algorithm maintains the minimum price seen so far and updates the maximum difference when a larger price is encountered. The time complexity is O(n) and space complexity is O(1).
Technique 5: Stack-based Approach
Stack-based approaches are particularly useful for problems that involve finding patterns in stock prices, such as identifying peaks and valleys or solving problems related to stock spans.
Example: Stock Span Problem
Problem: The stock span problem is a financial problem where we have a series of n daily price quotes for a stock and we need to calculate the span of the stock’s price for all n days. The span of the stock’s price on a given day i is defined as the maximum number of consecutive days just before the given day, for which the price of the stock on the current day is less than or equal to its price on the given day.
def calculate_span(prices):
n = len(prices)
spans = [1] * n
stack = []
for i in range(n):
while stack and prices[stack[-1]] <= prices[i]:
stack.pop()
if stack:
spans[i] = i - stack[-1]
else:
spans[i] = i + 1
stack.append(i)
return spans
# Example usage
prices = [100, 80, 60, 70, 60, 75, 85]
print(calculate_span(prices)) # Output: [1, 1, 1, 2, 1, 4, 6]
This solution uses a stack to keep track of the indices of prices. It efficiently calculates the span for each day by maintaining a stack of indices of prices in decreasing order. The time and space complexity are both O(n).
Advanced Techniques and Optimizations
As you become more comfortable with the basic techniques, you can explore more advanced approaches and optimizations:
1. Space Optimization in Dynamic Programming
Many DP solutions can be optimized to use O(1) space instead of O(n) by observing that we often only need the results from the previous few states.
2. Segment Trees
For problems involving range queries on stock prices, segment trees can provide efficient solutions with O(log n) query time.
3. Sliding Window Technique
This technique is useful for problems that involve finding optimal subarray within a specific window size.
4. Binary Search Variations
Some stock price problems can be solved efficiently using binary search, especially when looking for a specific value or optimizing a parameter.
Common Pitfalls and How to Avoid Them
When solving stock price problems, be aware of these common pitfalls:
- Overlooking Edge Cases: Always consider scenarios with empty arrays, single-element arrays, or arrays with all identical elements.
- Incorrect Profit Calculation: Ensure you’re calculating profits correctly, especially in problems with multiple transactions.
- Ignoring Problem Constraints: Pay close attention to constraints like cooldown periods or transaction fees.
- Inefficient Solutions: Avoid nested loops when possible, as they often lead to O(n^2) time complexity, which is typically too slow for large datasets.
Practice Problems
To reinforce your understanding and skills, try solving these stock price problems:
- Best Time to Buy and Sell Stock III (at most two transactions allowed)
- Best Time to Buy and Sell Stock IV (at most k transactions allowed)
- Stock Price Fluctuation (design a data structure to track stock prices)
- Online Stock Span (calculate stock spans in real-time)
- Minimize Maximum of Products Distributed to Any Store (a variation involving distribution)
Conclusion
Mastering stock price problems is a valuable skill for any programmer, especially those preparing for technical interviews at top tech companies. The techniques we’ve explored – one-pass approach, dynamic programming, state machine modeling, Kadane’s algorithm, and stack-based solutions – form a solid foundation for tackling a wide range of stock price challenges.
Remember, the key to success lies not just in memorizing solutions, but in understanding the underlying principles and being able to adapt them to new problems. As you practice, focus on identifying patterns, optimizing your solutions, and explaining your thought process clearly.
Keep honing your skills, and you’ll be well-prepared to tackle stock price problems and a wide array of other algorithmic challenges in your coding journey. Happy coding!