When to Use a Running Sum Approach in Algorithmic Problem Solving
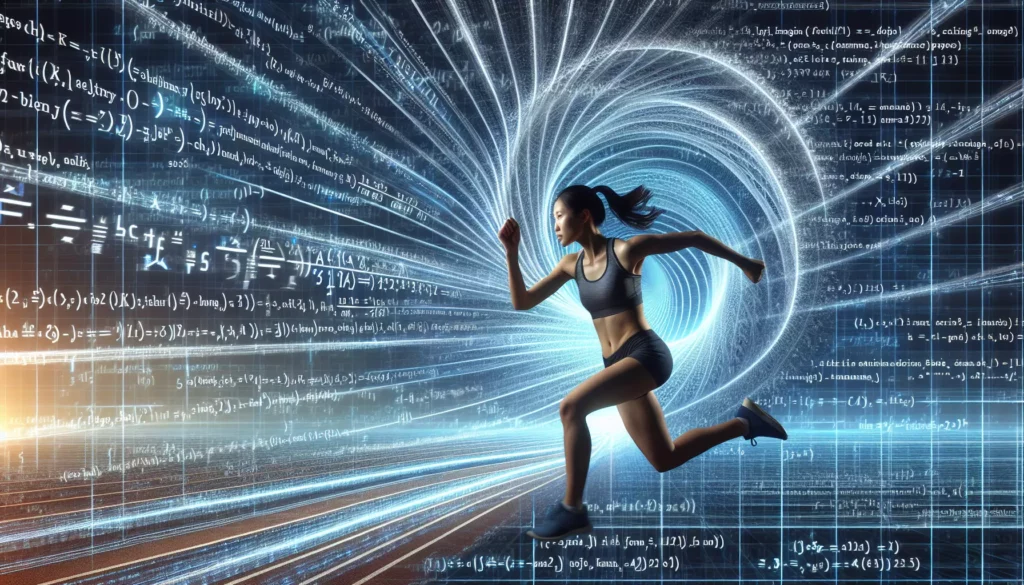
In the world of algorithmic problem-solving, efficiency and elegance often go hand in hand. Among the various techniques that programmers employ to tackle complex problems, the running sum approach stands out as a powerful and versatile tool. This method, also known as the prefix sum or cumulative sum, can significantly optimize solutions for a wide range of problems, particularly those involving array manipulations and range queries. In this comprehensive guide, we’ll explore when and how to use the running sum approach, its benefits, and practical examples to help you master this essential technique.
Understanding the Running Sum Approach
Before diving into specific use cases, let’s establish a clear understanding of what the running sum approach entails:
A running sum is a cumulative total of a sequence of numbers, where each element in the resulting array represents the sum of all previous elements up to that point. For an array A
of length n
, the running sum array R
would be defined as:
R[i] = A[0] + A[1] + A[2] + ... + A[i]
This simple concept forms the foundation for solving a variety of problems with improved time complexity.
When to Consider Using a Running Sum
The running sum approach is particularly useful in the following scenarios:
- Range Sum Queries
- Subarray Problems
- Sliding Window Calculations
- Prefix and Suffix Computations
- Optimization of Space-Time Tradeoffs
Let’s explore each of these scenarios in detail.
1. Range Sum Queries
One of the most common applications of the running sum approach is in efficiently handling range sum queries. When you need to calculate the sum of elements within a specific range of an array multiple times, using a running sum can dramatically reduce the time complexity.
Problem Example: Given an array of integers, answer multiple queries asking for the sum of elements between indices i and j (inclusive).
Naive Approach: For each query, iterate through the array from index i to j and sum the elements. This results in O(n) time complexity per query, where n is the size of the range.
Running Sum Approach: Precompute the running sum array. Then, for each query, the sum of elements between indices i and j can be calculated as:
sum(i, j) = R[j] - R[i-1] (if i > 0)
sum(i, j) = R[j] (if i == 0)
This reduces the time complexity of each query to O(1), with a one-time O(n) preprocessing step to compute the running sum array.
2. Subarray Problems
Many problems involving subarrays can be efficiently solved using the running sum approach. This is particularly useful when dealing with questions about contiguous segments of an array.
Problem Example: Find the subarray with the maximum sum (Kadane’s Algorithm).
While Kadane’s Algorithm itself doesn’t explicitly use a running sum array, the concept of maintaining a running sum is at its core. Here’s how you might implement it:
def max_subarray_sum(arr):
max_sum = float('-inf')
current_sum = 0
for num in arr:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
In this implementation, current_sum
acts as a running sum, constantly updated to keep track of the maximum sum ending at the current position.
3. Sliding Window Calculations
The running sum approach can be incredibly useful when dealing with sliding window problems, especially when the window size is fixed.
Problem Example: Given an array of integers and a window size k, find the maximum sum of any contiguous subarray of size k.
Running Sum Approach:
def max_sum_subarray(arr, k):
n = len(arr)
if n < k:
return None
# Compute sum of first window
window_sum = sum(arr[:k])
max_sum = window_sum
# Slide the window and update max_sum
for i in range(k, n):
window_sum = window_sum - arr[i-k] + arr[i]
max_sum = max(max_sum, window_sum)
return max_sum
This approach maintains a running sum of the current window, updating it in O(1) time as the window slides, resulting in an overall O(n) time complexity.
4. Prefix and Suffix Computations
The running sum concept can be extended to create prefix and suffix arrays, which are useful in problems requiring information about elements before or after a given index.
Problem Example: Given an array of integers, for each element, find the product of all other elements.
Running Sum (Product) Approach:
def product_except_self(nums):
n = len(nums)
prefix = [1] * n
suffix = [1] * n
result = [1] * n
# Compute prefix products
for i in range(1, n):
prefix[i] = prefix[i-1] * nums[i-1]
# Compute suffix products
for i in range(n-2, -1, -1):
suffix[i] = suffix[i+1] * nums[i+1]
# Combine prefix and suffix
for i in range(n):
result[i] = prefix[i] * suffix[i]
return result
This solution uses the concept of running product (analogous to running sum) to efficiently compute the required result in O(n) time complexity.
5. Optimization of Space-Time Tradeoffs
In some cases, using a running sum can help optimize the space-time tradeoff in algorithms. By precomputing and storing certain values, we can often achieve faster query times at the cost of some additional space.
Problem Example: Given a matrix, efficiently answer queries about the sum of elements in a rectangular region.
Running Sum Approach: Use a 2D prefix sum matrix.
def precompute_2d_prefix_sum(matrix):
if not matrix or not matrix[0]:
return []
m, n = len(matrix), len(matrix[0])
prefix_sum = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(1, m + 1):
for j in range(1, n + 1):
prefix_sum[i][j] = (
prefix_sum[i-1][j] +
prefix_sum[i][j-1] -
prefix_sum[i-1][j-1] +
matrix[i-1][j-1]
)
return prefix_sum
def query_sum(prefix_sum, r1, c1, r2, c2):
return (
prefix_sum[r2+1][c2+1] -
prefix_sum[r1][c2+1] -
prefix_sum[r2+1][c1] +
prefix_sum[r1][c1]
)
This approach allows for O(1) time complexity for each query, at the cost of O(m*n) additional space and preprocessing time.
Benefits of the Running Sum Approach
Now that we’ve explored various scenarios where the running sum approach is applicable, let’s summarize its key benefits:
- Time Efficiency: By precomputing sums, we can often reduce time complexity for subsequent operations from O(n) to O(1).
- Versatility: The concept can be applied to a wide range of problems, from simple array manipulations to complex matrix operations.
- Space-Time Tradeoff: It offers a way to balance between time efficiency and space usage, often allowing for significant speedups with manageable space overhead.
- Simplification of Complex Queries: It can turn potentially complex range-based queries into simple arithmetic operations.
- Foundation for Advanced Techniques: Understanding running sums paves the way for more advanced data structures like Fenwick Trees and Segment Trees.
Potential Drawbacks and Considerations
While the running sum approach is powerful, it’s important to be aware of its limitations:
- Space Complexity: The need to store precomputed sums can increase space usage, which might be a concern for very large datasets.
- Preprocessing Overhead: The initial computation of the running sum array adds a one-time cost, which might not be worthwhile for small datasets or when few queries are expected.
- Immutable Data: This approach is most effective when the underlying data doesn’t change frequently. For mutable data structures, maintaining the running sum can add complexity.
- Floating Point Precision: When dealing with floating-point numbers, accumulating a running sum can lead to precision errors due to the limitations of floating-point arithmetic.
Advanced Applications and Extensions
As you become more comfortable with the basic running sum concept, consider exploring these advanced applications and extensions:
1. 2D and Higher Dimensional Running Sums
The running sum concept can be extended to two or more dimensions. This is particularly useful for problems involving matrices or higher-dimensional data structures.
Example: Computing the sum of elements in a rectangular region of a 2D matrix in O(1) time after O(m*n) preprocessing.
2. Difference Arrays
The difference array is the inverse operation of the running sum. It’s useful for efficiently applying range updates to an array.
Example: Given an array and multiple update operations of the form “add value v to all elements in range [l, r]”, efficiently perform these updates and retrieve the final array.
3. Fenwick Trees (Binary Indexed Trees)
Fenwick Trees are a more advanced data structure that builds upon the running sum concept. They allow for efficient updates and range sum queries in O(log n) time.
Use Case: When you need to perform both range sum queries and point updates efficiently on a mutable array.
4. Segment Trees
Segment Trees are another advanced data structure that can be seen as an extension of the running sum idea. They allow for even more flexible range queries and updates.
Use Case: When you need to perform various types of range queries (sum, min, max, etc.) and range updates efficiently.
5. Running Sum with Modular Arithmetic
In some problems, especially in competitive programming, you might need to compute running sums under modular arithmetic.
Example: Computing the sum of a very large range of numbers modulo a prime number, useful in certain number theory problems.
Practice Problems
To solidify your understanding of the running sum approach, try solving these problems:
- Range Sum Query – Immutable (LeetCode)
- Subarray Sum Equals K (LeetCode)
- Continuous Subarray Sum (LeetCode)
- Matrix Block Sum (LeetCode)
- Product of Array Except Self (LeetCode)
Conclusion
The running sum approach is a fundamental technique in algorithmic problem-solving that can significantly optimize solutions for a wide range of problems. By precomputing cumulative sums, we can transform potentially expensive operations into constant-time queries, leading to more efficient algorithms.
As you continue to develop your programming skills, keep the running sum approach in your toolkit. It’s not just about knowing when to use it, but also about recognizing problem patterns where it might be applicable. With practice, you’ll develop an intuition for identifying situations where this technique can provide elegant and efficient solutions.
Remember, while the running sum approach is powerful, it’s just one of many algorithmic techniques. The key to becoming a proficient programmer is to understand a variety of approaches and know when to apply each one. Keep practicing, exploring new problems, and expanding your algorithmic repertoire. Happy coding!