Mastering Subsequence Problems: Strategies and Solutions for Coding Interviews
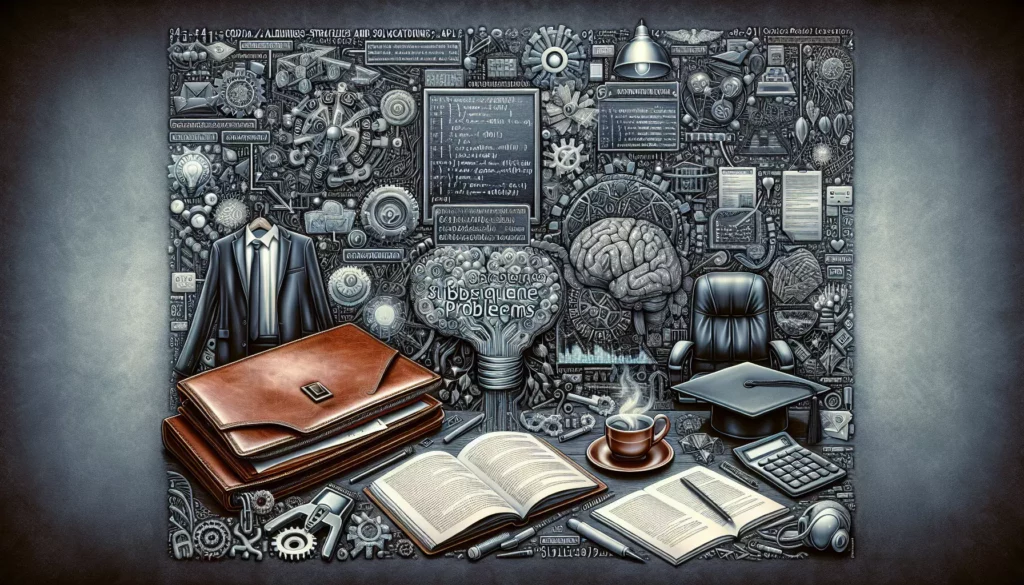
In the world of coding interviews and algorithmic problem-solving, subsequence problems are a common and important category that often appear in technical assessments, particularly for positions at major tech companies. These problems test a candidate’s ability to manipulate sequences, think dynamically, and optimize solutions. In this comprehensive guide, we’ll explore various strategies for solving subsequence problems, providing you with the tools and knowledge needed to tackle these challenges confidently.
What are Subsequence Problems?
Before diving into strategies, let’s clarify what we mean by subsequence problems. A subsequence is a sequence that can be derived from another sequence by deleting some or no elements without changing the order of the remaining elements. For example, “ace” is a subsequence of “abcde”.
Subsequence problems often involve tasks such as:
- Finding the longest common subsequence between two strings
- Determining if one string is a subsequence of another
- Finding the longest increasing subsequence in an array
- Counting the number of distinct subsequences
These problems can be challenging because they often require efficient algorithms to handle large inputs and may have multiple valid solutions.
Key Strategies for Solving Subsequence Problems
1. Dynamic Programming
Dynamic Programming (DP) is perhaps the most powerful and commonly used technique for solving subsequence problems. It works by breaking down a complex problem into simpler subproblems and storing the results for future use.
Key principles of using DP for subsequence problems:
- Identify overlapping subproblems
- Define a clear recurrence relation
- Use memoization or tabulation to store intermediate results
Example: Longest Common Subsequence (LCS)
def lcs(X, Y):
m, n = len(X), len(Y)
L = [[0] * (n + 1) for _ in range(m + 1)]
for i in range(1, m + 1):
for j in range(1, n + 1):
if X[i-1] == Y[j-1]:
L[i][j] = L[i-1][j-1] + 1
else:
L[i][j] = max(L[i-1][j], L[i][j-1])
return L[m][n]
# Example usage
X = "ABCDGH"
Y = "AEDFHR"
print(f"Length of LCS is {lcs(X, Y)}")
This DP approach has a time complexity of O(mn) and space complexity of O(mn), where m and n are the lengths of the input strings.
2. Two-Pointer Technique
The two-pointer technique is particularly useful for problems involving two sequences or when you need to compare elements at different positions.
Key aspects of the two-pointer technique:
- Use two pointers to traverse sequences simultaneously
- Move pointers based on specific conditions
- Efficiently compare elements without nested loops
Example: Is Subsequence
def isSubsequence(s: str, t: str) -> bool:
i, j = 0, 0
while i < len(s) and j < len(t):
if s[i] == t[j]:
i += 1
j += 1
return i == len(s)
# Example usage
s = "abc"
t = "ahbgdc"
print(f"Is '{s}' a subsequence of '{t}'? {isSubsequence(s, t)}")
This approach has a time complexity of O(n), where n is the length of the target string t.
3. Binary Search
Binary search can be an effective technique for certain types of subsequence problems, especially when dealing with sorted sequences or when searching for optimal values.
Key points for using binary search in subsequence problems:
- Identify a monotonic property in the problem
- Define clear boundaries for the search space
- Implement efficient checks for the mid-point of the search range
Example: Longest Increasing Subsequence (LIS) using Binary Search
import bisect
def lengthOfLIS(nums):
if not nums:
return 0
tails = [0] * len(nums)
size = 0
for num in nums:
i = bisect.bisect_left(tails, num, 0, size)
tails[i] = num
size = max(size, i + 1)
return size
# Example usage
nums = [10,9,2,5,3,7,101,18]
print(f"Length of LIS: {lengthOfLIS(nums)}")
This approach has a time complexity of O(n log n) and space complexity of O(n), where n is the length of the input array.
4. Greedy Algorithms
Greedy algorithms can be effective for certain types of subsequence problems, especially when local optimal choices lead to a global optimum.
Key aspects of using greedy algorithms:
- Identify the greedy choice at each step
- Prove that the greedy choice is safe and optimal
- Implement the solution efficiently
Example: Maximum Subarray Sum (Kadane’s Algorithm)
def maxSubArray(nums):
max_sum = current_sum = nums[0]
for num in nums[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Example usage
nums = [-2,1,-3,4,-1,2,1,-5,4]
print(f"Maximum subarray sum: {maxSubArray(nums)}")
This greedy approach has a time complexity of O(n) and space complexity of O(1), where n is the length of the input array.
Advanced Techniques for Subsequence Problems
1. Segment Trees
Segment trees are a powerful data structure for handling range queries and updates efficiently. They can be particularly useful for certain types of subsequence problems that involve range operations.
Key points for using segment trees:
- Construct the segment tree efficiently
- Implement query and update operations
- Use lazy propagation for range updates when necessary
Example: Range Minimum Query (RMQ)
class SegmentTree:
def __init__(self, arr):
self.n = len(arr)
self.tree = [0] * (4 * self.n)
self._build(arr, 0, 0, self.n - 1)
def _build(self, arr, node, start, end):
if start == end:
self.tree[node] = arr[start]
else:
mid = (start + end) // 2
self._build(arr, 2 * node + 1, start, mid)
self._build(arr, 2 * node + 2, mid + 1, end)
self.tree[node] = min(self.tree[2 * node + 1], self.tree[2 * node + 2])
def query(self, left, right):
return self._query(0, 0, self.n - 1, left, right)
def _query(self, node, start, end, left, right):
if left > end or right < start:
return float('inf')
if left <= start and end <= right:
return self.tree[node]
mid = (start + end) // 2
left_min = self._query(2 * node + 1, start, mid, left, right)
right_min = self._query(2 * node + 2, mid + 1, end, left, right)
return min(left_min, right_min)
# Example usage
arr = [1, 3, 2, 7, 9, 11]
st = SegmentTree(arr)
print(f"Minimum in range [1, 4]: {st.query(1, 4)}")
The time complexity for construction is O(n), and for each query, it’s O(log n), where n is the length of the input array.
2. Fenwick Trees (Binary Indexed Trees)
Fenwick trees, also known as Binary Indexed Trees (BIT), are another efficient data structure for handling range sum queries and point updates. They can be useful for certain subsequence problems involving cumulative sums or frequency counts.
Key aspects of using Fenwick trees:
- Understand the binary representation of indices
- Implement efficient update and query operations
- Use for problems involving range sum queries
Example: Range Sum Query
class FenwickTree:
def __init__(self, n):
self.size = n
self.tree = [0] * (n + 1)
def update(self, i, delta):
while i <= self.size:
self.tree[i] += delta
i += i & (-i)
def sum(self, i):
total = 0
while i > 0:
total += self.tree[i]
i -= i & (-i)
return total
def range_sum(self, left, right):
return self.sum(right) - self.sum(left - 1)
# Example usage
arr = [1, 3, 5, 7, 9, 11]
ft = FenwickTree(len(arr))
for i, val in enumerate(arr, 1):
ft.update(i, val)
print(f"Sum in range [2, 5]: {ft.range_sum(2, 5)}")
Both update and query operations have a time complexity of O(log n), where n is the size of the Fenwick tree.
3. Suffix Arrays and LCP Arrays
Suffix arrays and Longest Common Prefix (LCP) arrays are powerful tools for string processing and can be particularly useful for certain types of subsequence problems involving strings.
Key points for using suffix arrays and LCP arrays:
- Construct the suffix array efficiently
- Build the LCP array using the suffix array
- Use these structures for efficient string matching and analysis
Example: Finding the Longest Repeated Substring
from typing import List
def build_suffix_array(s: str) -> List[int]:
n = len(s)
sa = list(range(n))
sa.sort(key=lambda i: s[i:])
return sa
def build_lcp_array(s: str, sa: List[int]) -> List[int]:
n = len(s)
rank = [0] * n
for i in range(n):
rank[sa[i]] = i
k = 0
lcp = [0] * (n - 1)
for i in range(n):
if rank[i] == n - 1:
k = 0
continue
j = sa[rank[i] + 1]
while i + k < n and j + k < n and s[i + k] == s[j + k]:
k += 1
lcp[rank[i]] = k
if k > 0:
k -= 1
return lcp
def longest_repeated_substring(s: str) -> str:
sa = build_suffix_array(s)
lcp = build_lcp_array(s, sa)
max_len = max(lcp)
if max_len == 0:
return ""
index = lcp.index(max_len)
return s[sa[index]:sa[index] + max_len]
# Example usage
s = "banana"
print(f"Longest repeated substring in '{s}': {longest_repeated_substring(s)}")
The time complexity for building the suffix array and LCP array is O(n log n), where n is the length of the input string.
Common Pitfalls and How to Avoid Them
When solving subsequence problems, there are several common pitfalls that you should be aware of and try to avoid:
- Inefficient Brute Force Approaches: While brute force solutions can be a good starting point, they often lead to time limit exceeded errors in coding interviews. Always consider more efficient algorithms, especially for large inputs.
- Overlooking Edge Cases: Make sure to consider empty sequences, sequences with a single element, and other edge cases that might cause your algorithm to fail.
- Incorrect Base Cases in Recursive Solutions: When using recursion, ensure that your base cases are correctly defined and handle all possible scenarios.
- Mismanaging Array Indices: Off-by-one errors are common in subsequence problems. Double-check your array indexing, especially when dealing with multiple sequences.
- Ignoring Space Complexity: While optimizing for time, don’t forget about space complexity. Sometimes, a solution with slightly higher time complexity but significantly lower space complexity might be preferred.
- Overcomplicating the Solution: Sometimes, a simple two-pointer approach or a well-implemented dynamic programming solution can be more efficient and easier to understand than a complex algorithm.
Practice Problems
To solidify your understanding and skills in solving subsequence problems, here are some practice problems you can try:
- Longest Palindromic Subsequence
- Shortest Common Supersequence
- Edit Distance
- Distinct Subsequences
- Longest Bitonic Subsequence
- Box Stacking Problem
- Maximum Sum Increasing Subsequence
- Longest Repeating Subsequence
- Minimum number of deletions to make a sorted sequence
- Longest Arithmetic Subsequence
These problems cover a range of difficulties and techniques, allowing you to practice and improve your skills in tackling subsequence challenges.
Conclusion
Mastering subsequence problems is crucial for success in coding interviews, especially for positions at major tech companies. By understanding and applying the strategies discussed in this guide – from dynamic programming and two-pointer techniques to advanced data structures like segment trees and suffix arrays – you’ll be well-equipped to tackle a wide range of subsequence challenges.
Remember that practice is key. As you work through different problems, you’ll develop an intuition for which techniques to apply in various scenarios. Don’t be discouraged if you struggle at first; subsequence problems can be tricky, but with persistence and practice, you’ll improve your problem-solving skills and become more confident in your abilities.
Keep exploring new problems, analyzing different approaches, and refining your coding skills. With dedication and the right strategies, you’ll be well-prepared to excel in your coding interviews and tackle complex subsequence problems with confidence.