When to Consider Using a Counter Variable in Programming
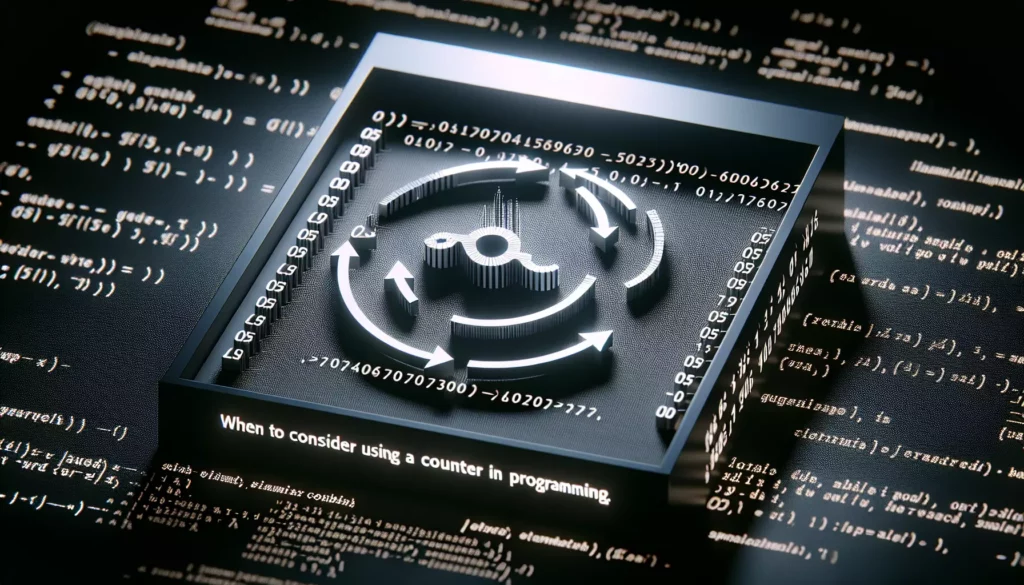
In the world of programming, counter variables are fundamental tools that play a crucial role in various algorithms and problem-solving scenarios. As you progress in your coding journey, understanding when and how to use counter variables effectively can significantly enhance your ability to write efficient and elegant code. This article will explore the concept of counter variables, their applications, and provide practical examples to illustrate their importance in programming.
What is a Counter Variable?
A counter variable, also known as a loop counter or iterator, is a variable used to keep track of the number of iterations or occurrences of a particular event in a program. It is typically initialized with a starting value and then incremented or decremented as the program executes. Counter variables are commonly used in loops, algorithms, and various programming constructs to control flow, track progress, or accumulate results.
When Should You Use a Counter Variable?
Counter variables are versatile tools that can be employed in numerous programming scenarios. Here are some common situations where using a counter variable is beneficial:
1. Controlling Loop Iterations
One of the most common uses of counter variables is to control the number of iterations in a loop. This is particularly useful when you need to perform a specific action a predetermined number of times.
for (int i = 0; i < 5; i++) {
System.out.println("Iteration " + i);
}
In this example, the variable ‘i’ serves as a counter, ensuring the loop executes exactly five times.
2. Tracking Array or List Indices
When working with arrays or lists, counter variables are often used to keep track of indices, allowing you to access or modify specific elements.
int[] numbers = {1, 2, 3, 4, 5};
int sum = 0;
for (int i = 0; i < numbers.length; i++) {
sum += numbers[i];
}
System.out.println("Sum: " + sum);
Here, the counter ‘i’ is used to iterate through each element of the array and calculate the sum.
3. Counting Occurrences
Counter variables are excellent for keeping track of how many times a specific condition or event occurs within a program.
String text = "Hello, World!";
int vowelCount = 0;
for (char c : text.toCharArray()) {
if ("aeiouAEIOU".indexOf(c) != -1) {
vowelCount++;
}
}
System.out.println("Number of vowels: " + vowelCount);
In this example, ‘vowelCount’ acts as a counter to tally the number of vowels in the given string.
4. Implementing Algorithms
Many algorithms rely on counter variables to keep track of progress or to control the flow of execution. For instance, in sorting algorithms like bubble sort, a counter is often used to track the number of passes through the array.
public static void bubbleSort(int[] arr) {
int n = arr.length;
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < n - i - 1; j++) {
if (arr[j] > arr[j + 1]) {
// Swap arr[j] and arr[j+1]
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
In this bubble sort implementation, both ‘i’ and ‘j’ serve as counter variables to control the nested loops and ensure proper sorting.
5. Generating Sequences
Counter variables are useful for generating sequences of numbers or patterns. This can be particularly helpful in mathematical computations or when creating test data.
public static void generateFibonacci(int n) {
int a = 0, b = 1;
System.out.print(a + " " + b + " ");
for (int i = 2; i < n; i++) {
int c = a + b;
System.out.print(c + " ");
a = b;
b = c;
}
}
In this Fibonacci sequence generator, ‘i’ acts as a counter to determine how many numbers in the sequence to generate.
6. Implementing Timers or Delays
Counter variables can be used to implement simple timers or delays in your program, especially when working with game development or real-time systems.
public static void countdownTimer(int seconds) {
for (int i = seconds; i > 0; i--) {
System.out.println(i + " seconds remaining");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
System.out.println("Time's up!");
}
This countdown timer uses a counter variable ‘i’ to keep track of the remaining seconds and display them to the user.
Best Practices for Using Counter Variables
While counter variables are incredibly useful, it’s important to use them effectively and maintain clean, readable code. Here are some best practices to keep in mind:
1. Choose Meaningful Variable Names
Instead of using single-letter variable names like ‘i’, ‘j’, or ‘k’, consider using more descriptive names that convey the purpose of the counter. For example:
for (int studentCount = 0; studentCount < totalStudents; studentCount++) {
// Process student data
}
2. Initialize Counters Properly
Always initialize your counter variables with an appropriate starting value. This is typically 0 for counting occurrences, or the first index of an array (which is also usually 0 in most programming languages).
3. Use the Appropriate Data Type
Choose the right data type for your counter variable based on the expected range of values. For small counts, an int might suffice, but for larger values, you might need a long or even a BigInteger in some cases.
4. Be Mindful of Overflow
When working with large numbers or in loops that iterate many times, be aware of the possibility of integer overflow. Consider using a larger data type or implementing checks to prevent overflow situations.
5. Avoid Magic Numbers
Instead of hardcoding numbers in your loops or conditions, use constants or variables with meaningful names to make your code more readable and maintainable.
final int MAX_ATTEMPTS = 5;
for (int attempt = 1; attempt <= MAX_ATTEMPTS; attempt++) {
// Attempt some operation
}
6. Consider Using Enhanced For Loops
In some cases, especially when iterating over collections or arrays, you can use enhanced for loops (for-each loops) to avoid explicit counter variables:
int[] numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
System.out.println(number);
}
Advanced Uses of Counter Variables
As you progress in your programming journey, you’ll encounter more complex scenarios where counter variables play a crucial role. Let’s explore some advanced uses:
1. Multi-dimensional Arrays
When working with multi-dimensional arrays, you often need multiple counter variables to traverse all dimensions:
int[][] matrix = {
{1, 2, 3},
{4, 5, 6},
{7, 8, 9}
};
for (int i = 0; i < matrix.length; i++) {
for (int j = 0; j < matrix[i].length; j++) {
System.out.print(matrix[i][j] + " ");
}
System.out.println();
}
2. Nested Loops with Different Increments
Sometimes, you may need to use counter variables with different increment patterns in nested loops:
for (int i = 1; i <= 5; i++) {
for (int j = i; j <= 5; j++) {
System.out.print("* ");
}
System.out.println();
}
This code produces a pattern of asterisks in a right-angled triangle shape.
3. Implementing Sliding Window Algorithms
Sliding window algorithms often use counter variables to keep track of the window’s start and end positions:
public static int maxSumSubarray(int[] arr, int k) {
int n = arr.length;
int maxSum = Integer.MIN_VALUE;
int windowSum = 0;
for (int i = 0; i < n; i++) {
windowSum += arr[i];
if (i >= k - 1) {
maxSum = Math.max(maxSum, windowSum);
windowSum -= arr[i - (k - 1)];
}
}
return maxSum;
}
This function finds the maximum sum of a subarray of size ‘k’ in the given array.
4. Implementing Recursion with Memoization
When optimizing recursive algorithms using memoization, counter variables can be used to track the number of function calls and cache hits:
public class FibonacciMemoization {
private static Map<Integer, Long> memo = new HashMap<>();
private static int functionCalls = 0;
private static int cacheHits = 0;
public static long fibonacci(int n) {
functionCalls++;
if (n <= 1) {
return n;
}
if (memo.containsKey(n)) {
cacheHits++;
return memo.get(n);
}
long result = fibonacci(n - 1) + fibonacci(n - 2);
memo.put(n, result);
return result;
}
public static void main(String[] args) {
int n = 40;
long result = fibonacci(n);
System.out.println("Fibonacci(" + n + ") = " + result);
System.out.println("Function calls: " + functionCalls);
System.out.println("Cache hits: " + cacheHits);
}
}
In this example, ‘functionCalls’ and ‘cacheHits’ serve as counter variables to analyze the efficiency of the memoization technique.
5. Implementing Custom Iterators
When creating custom iterators in object-oriented programming, counter variables are often used to keep track of the current position:
public class CustomArrayList<T> implements Iterable<T> {
private T[] elements;
private int size;
// Constructor and other methods...
@Override
public Iterator<T> iterator() {
return new Iterator<T>() {
private int currentIndex = 0;
@Override
public boolean hasNext() {
return currentIndex < size;
}
@Override
public T next() {
if (!hasNext()) {
throw new NoSuchElementException();
}
return elements[currentIndex++];
}
};
}
}
In this custom ArrayList implementation, ‘currentIndex’ acts as a counter variable in the iterator to keep track of the current position.
Common Pitfalls and How to Avoid Them
While counter variables are incredibly useful, there are some common mistakes programmers make when using them. Let’s explore these pitfalls and how to avoid them:
1. Off-by-One Errors
Off-by-one errors occur when a loop iterates one time too many or too few. This often happens when using the wrong comparison operator or initializing the counter incorrectly.
Incorrect:
int[] arr = {1, 2, 3, 4, 5};
for (int i = 0; i <= arr.length; i++) {
System.out.println(arr[i]); // ArrayIndexOutOfBoundsException on last iteration
}
Correct:
int[] arr = {1, 2, 3, 4, 5};
for (int i = 0; i < arr.length; i++) {
System.out.println(arr[i]);
}
2. Forgetting to Update the Counter
In while loops or do-while loops, it’s easy to forget to update the counter, leading to infinite loops.
Incorrect:
int i = 0;
while (i < 5) {
System.out.println(i);
// Forgot to increment i
}
Correct:
int i = 0;
while (i < 5) {
System.out.println(i);
i++;
}
3. Using the Wrong Increment/Decrement Operator
Be careful when using pre-increment (++i) vs. post-increment (i++) operators, as they can lead to unexpected results in certain situations.
int i = 0;
System.out.println(i++); // Prints 0, then increments i to 1
System.out.println(++i); // Increments i to 2, then prints 2
4. Not Considering Integer Overflow
When working with large numbers, be aware of the possibility of integer overflow. Consider using a larger data type or implementing checks to prevent overflow situations.
public static void countToMaxValue() {
for (int i = 0; i <= Integer.MAX_VALUE; i++) {
if (i < 0) {
System.out.println("Overflow occurred at: " + (i - 1));
break;
}
}
}
5. Modifying the Counter Variable Within the Loop
Avoid modifying the counter variable within the loop body, as it can lead to unexpected behavior and make the code harder to understand.
Incorrect:
for (int i = 0; i < 10; i++) {
System.out.println(i);
if (i == 5) {
i = 8; // Skips iterations 6 and 7
}
}
Instead, consider using control flow statements like continue or break to alter the loop’s behavior if needed.
Alternatives to Counter Variables
While counter variables are widely used and often the best choice for many situations, there are alternative approaches that can sometimes lead to more readable or efficient code. Let’s explore some of these alternatives:
1. Enhanced For Loops (For-Each Loops)
When iterating over collections or arrays, enhanced for loops can often replace traditional counter-based loops:
int[] numbers = {1, 2, 3, 4, 5};
for (int number : numbers) {
System.out.println(number);
}
2. Stream API (Java 8+)
For more complex operations on collections, the Stream API can often replace loops with counter variables:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int sum = numbers.stream()
.filter(n -> n % 2 == 0)
.mapToInt(Integer::intValue)
.sum();
System.out.println("Sum of even numbers: " + sum);
3. Recursive Approaches
Some problems that typically use counter variables can be solved recursively, eliminating the need for an explicit counter:
public static int factorial(int n) {
if (n <= 1) {
return 1;
}
return n * factorial(n - 1);
}
4. Iterator Pattern
When working with custom data structures, implementing the Iterator pattern can provide a clean way to traverse elements without explicit counter variables:
public class CustomList<T> implements Iterable<T> {
private List<T> items = new ArrayList<>();
public void add(T item) {
items.add(item);
}
@Override
public Iterator<T> iterator() {
return items.iterator();
}
}
CustomList<String> list = new CustomList<>();
list.add("A");
list.add("B");
list.add("C");
for (String item : list) {
System.out.println(item);
}
5. Functional Programming Techniques
Functional programming paradigms often provide alternatives to imperative loops with counters. For example, using reduce operations:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
int product = numbers.stream().reduce(1, (a, b) -> a * b);
System.out.println("Product: " + product);
Conclusion
Counter variables are a fundamental concept in programming that play a crucial role in various algorithms and problem-solving scenarios. Understanding when and how to use them effectively can significantly enhance your ability to write efficient and elegant code. From controlling loop iterations to implementing complex algorithms, counter variables offer a simple yet powerful tool for managing program flow and tracking progress.
As you continue to develop your programming skills, remember that while counter variables are often the best choice for many situations, it’s also important to be aware of alternative approaches. The key is to choose the most appropriate tool for each specific problem, balancing factors such as readability, efficiency, and maintainability.
By mastering the use of counter variables and understanding their alternatives, you’ll be well-equipped to tackle a wide range of programming challenges and write more effective code. Keep practicing, experimenting with different approaches, and always strive to improve your coding skills. Happy coding!