When to Use an In-Place Algorithm: A Comprehensive Guide
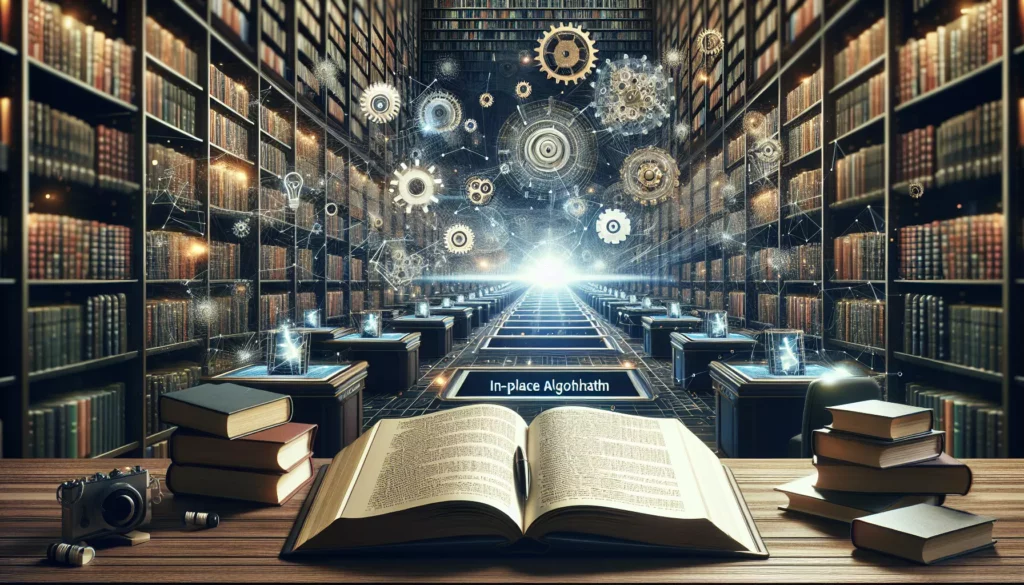
In the world of programming and algorithm design, efficiency is key. As developers, we’re constantly seeking ways to optimize our code, reduce resource consumption, and improve overall performance. One powerful technique in our toolkit is the use of in-place algorithms. But when should we reach for this particular tool? In this comprehensive guide, we’ll explore the concept of in-place algorithms, their benefits and drawbacks, and the scenarios where they shine brightest.
What is an In-Place Algorithm?
Before we dive into the when and why, let’s establish a clear understanding of what an in-place algorithm is. An in-place algorithm is a type of algorithm that transforms input using no auxiliary data structure. In other words, it modifies the input directly, using only a small amount of extra storage space that does not depend on the input size.
The key characteristics of an in-place algorithm are:
- It operates directly on the input data structure
- It uses only O(1) extra space, or a small amount of extra space that’s constant regardless of input size
- It usually modifies the original input
A classic example of an in-place algorithm is the bubble sort. Let’s take a look at a simple implementation:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1]:
arr[j], arr[j+1] = arr[j+1], arr[j]
return arr
# Example usage
numbers = [64, 34, 25, 12, 22, 11, 90]
sorted_numbers = bubble_sort(numbers)
print(sorted_numbers) # Output: [11, 12, 22, 25, 34, 64, 90]
In this example, the bubble_sort function modifies the input array directly, without using any additional arrays or data structures.
Benefits of In-Place Algorithms
Now that we understand what in-place algorithms are, let’s explore their advantages:
1. Space Efficiency
The primary benefit of in-place algorithms is their space efficiency. By operating directly on the input data structure, they require minimal additional memory. This can be crucial when dealing with large datasets or in memory-constrained environments.
2. Cache Friendliness
In-place algorithms often exhibit better cache performance. Since they work on a single data structure, they can take advantage of data locality, potentially leading to fewer cache misses and improved overall performance.
3. Simplicity
In some cases, in-place algorithms can lead to simpler implementations. Without the need to manage additional data structures, the code can be more straightforward and easier to understand.
4. Reduced Memory Allocation Overhead
By avoiding the creation of new data structures, in-place algorithms eliminate the overhead associated with memory allocation and deallocation. This can be particularly beneficial in scenarios where frequent allocations might lead to fragmentation or performance issues.
Drawbacks of In-Place Algorithms
While in-place algorithms offer significant advantages, they’re not without their drawbacks:
1. Data Destruction
One of the main drawbacks is that in-place algorithms often modify the original input. This can be problematic if you need to preserve the original data for future use or if multiple parts of your program rely on the input remaining unchanged.
2. Potential for Bugs
In-place modifications can sometimes lead to subtle bugs, especially in multi-threaded environments or when dealing with complex data structures. Care must be taken to ensure that all parts of the program are aware of and can handle the modifications.
3. Complexity in Implementation
While in-place algorithms can sometimes lead to simpler code, in other cases, they can significantly increase the complexity of the implementation. This is especially true for more complex algorithms where maintaining the correct state without auxiliary data structures can be challenging.
4. Reduced Flexibility
In-place algorithms may limit your options for future modifications or extensions to the algorithm. The tight coupling between the algorithm and the data structure can make it harder to adapt the code to new requirements.
When to Use In-Place Algorithms
Now that we’ve explored the pros and cons, let’s dive into the scenarios where in-place algorithms are particularly useful:
1. Memory-Constrained Environments
In situations where memory is at a premium, such as embedded systems or when dealing with extremely large datasets that push the limits of available RAM, in-place algorithms can be lifesavers. They allow you to process data that might otherwise be impossible to handle due to memory constraints.
For example, consider a scenario where you need to reverse a very large array on a system with limited memory:
def reverse_array_in_place(arr):
left, right = 0, len(arr) - 1
while left < right:
arr[left], arr[right] = arr[right], arr[left]
left += 1
right -= 1
return arr
# Example usage
large_array = list(range(1000000)) # A large array of numbers
reversed_array = reverse_array_in_place(large_array)
print(reversed_array[:10]) # Print first 10 elements to verify
This in-place reversal algorithm allows us to handle very large arrays without requiring additional memory proportional to the input size.
2. Performance-Critical Applications
In high-performance computing or real-time systems where every microsecond counts, the reduced memory allocation and improved cache performance of in-place algorithms can provide a crucial edge.
A classic example is the Fast Fourier Transform (FFT), which is widely used in signal processing. Many FFT implementations use in-place algorithms to maximize performance:
import numpy as np
def fft_in_place(x):
N = len(x)
if N <= 1:
return x
even = fft_in_place(x[0::2])
odd = fft_in_place(x[1::2])
T = [np.exp(-2j * np.pi * k / N) * odd[k] for k in range(N//2)]
return [even[k] + T[k] for k in range(N//2)] + [even[k] - T[k] for k in range(N//2)]
# Example usage
signal = np.random.random(1024)
fft_result = fft_in_place(signal)
print(fft_result[:5]) # Print first 5 elements of the result
While this is a simplified example, many production FFT libraries use more complex in-place algorithms for maximum efficiency.
3. Large-Scale Data Processing
When working with big data or processing large files, in-place algorithms can be invaluable. They allow you to handle datasets that are too large to fit entirely in memory by processing them in chunks or streams.
For instance, consider a scenario where you need to remove duplicates from a large file:
def remove_duplicates_in_place(filename):
with open(filename, 'r+') as file:
seen = set()
current_pos = 0
file.seek(0)
for line in file:
if line not in seen:
seen.add(line)
file.seek(current_pos)
file.write(line)
current_pos = file.tell()
file.truncate(current_pos)
# Example usage
remove_duplicates_in_place('large_file.txt')
This function reads the file line by line, keeping track of unique lines, and overwrites the file in-place with only the unique lines. This approach allows processing of files much larger than available RAM.
4. Algorithms with Natural In-Place Implementations
Some algorithms lend themselves naturally to in-place implementations. In these cases, using an in-place approach can lead to cleaner, more intuitive code. Examples include many sorting algorithms (like quicksort and heapsort), matrix transposition, and certain graph algorithms.
Here’s an example of an in-place matrix transposition:
def transpose_matrix_in_place(matrix):
for i in range(len(matrix)):
for j in range(i, len(matrix)):
matrix[i][j], matrix[j][i] = matrix[j][i], matrix[i][j]
return matrix
# Example usage
matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
]
transposed = transpose_matrix_in_place(matrix)
for row in transposed:
print(row)
This function transposes the matrix by swapping elements across the main diagonal, all without using any additional matrix.
5. When Input Preservation is Not Required
If you don’t need to preserve the original input data, and all parts of your program are aware that the input may be modified, using an in-place algorithm can be a great choice. This is often the case in single-use transformations or when working with temporary data.
For example, consider a function that capitalizes all strings in a list:
def capitalize_strings_in_place(strings):
for i in range(len(strings)):
strings[i] = strings[i].upper()
return strings
# Example usage
words = ["hello", "world", "python", "programming"]
capitalized = capitalize_strings_in_place(words)
print(capitalized) # Output: ['HELLO', 'WORLD', 'PYTHON', 'PROGRAMMING']
In this case, if we don’t need to keep the original lowercase strings, the in-place modification is perfectly acceptable and efficient.
When Not to Use In-Place Algorithms
While in-place algorithms have their place, there are scenarios where they might not be the best choice:
1. When Data Integrity is Crucial
If preserving the original input is important, either for auditing purposes, to allow for undo functionality, or because multiple parts of your program rely on the input remaining unchanged, you should avoid in-place algorithms.
2. In Functional Programming Paradigms
Functional programming emphasizes immutability and pure functions. In-place algorithms, which modify their inputs, go against these principles and should be avoided in codebases adhering strictly to functional programming paradigms.
3. When Code Clarity is More Important than Performance
In some cases, a non-in-place algorithm might be significantly easier to understand and maintain. If the performance gain from an in-place approach is minimal, it might be better to prioritize code readability.
4. When Working with Shared Resources
In multi-threaded or distributed systems, in-place modifications to shared data structures can lead to race conditions and other synchronization issues. In these cases, it’s often safer to create new data structures rather than modifying existing ones in-place.
Best Practices for Using In-Place Algorithms
If you decide that an in-place algorithm is the right choice for your situation, here are some best practices to keep in mind:
1. Document Clearly
Make sure to clearly document that your function modifies its input. This helps prevent confusion and potential bugs when others use your code.
2. Consider Providing Non-In-Place Alternatives
If possible, provide both in-place and non-in-place versions of your algorithm. This gives users the flexibility to choose based on their specific needs.
3. Use Assertions and Error Checking
Include assertions or error checks to ensure that the input can be safely modified in-place. This can help catch potential issues early.
4. Be Mindful of Edge Cases
In-place algorithms can sometimes behave unexpectedly with edge cases like empty inputs or inputs with specific patterns. Make sure to thoroughly test these scenarios.
5. Consider Copy-on-Write Semantics
In some languages or frameworks, you can implement copy-on-write semantics, where the data is only copied if it’s modified. This can provide a good balance between the efficiency of in-place algorithms and the safety of creating new data structures.
Conclusion
In-place algorithms are a powerful tool in a programmer’s arsenal, offering significant benefits in terms of space efficiency and performance. They shine brightest in memory-constrained environments, performance-critical applications, and when dealing with large-scale data processing. However, they’re not without their drawbacks, and their use should be carefully considered in light of factors such as data preservation needs, code clarity, and the overall architecture of your system.
As with many aspects of programming, the decision to use an in-place algorithm often comes down to a balance of trade-offs. By understanding the strengths and weaknesses of in-place algorithms, and considering the specific requirements and constraints of your project, you can make informed decisions about when and how to leverage this powerful technique.
Remember, the goal is always to write code that is not just efficient, but also clear, maintainable, and correct. In-place algorithms, when used judiciously, can help you achieve that goal, pushing the boundaries of what’s possible with limited resources and optimizing performance in critical scenarios. As you continue to develop your skills as a programmer, mastering the art of knowing when and how to use in-place algorithms will undoubtedly serve you well in your journey to write better, more efficient code.