When to Use an Adjacency List vs Matrix: A Comprehensive Guide
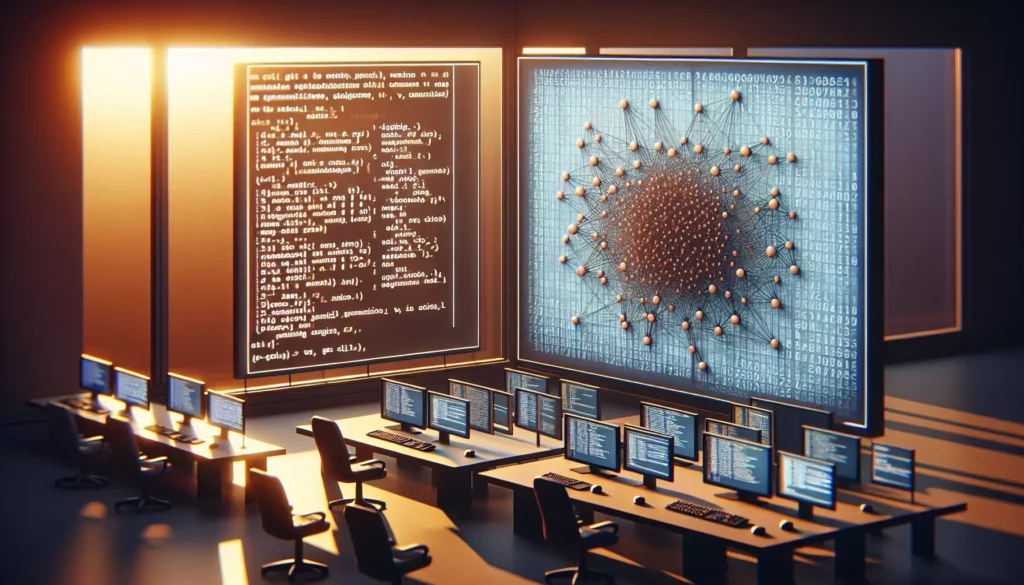
In the world of graph theory and computer science, representing relationships between entities is a fundamental concept. Two popular methods for representing graphs are adjacency lists and adjacency matrices. Each has its own strengths and weaknesses, making them suitable for different scenarios. In this comprehensive guide, we’ll explore when to use an adjacency list versus an adjacency matrix, providing you with the knowledge to make informed decisions in your coding projects and technical interviews.
Understanding Graphs
Before diving into the specifics of adjacency lists and matrices, let’s briefly review what graphs are and why they’re important in computer science.
A graph is a data structure consisting of a set of vertices (also called nodes) and a set of edges that connect these vertices. Graphs are used to represent various relationships and connections in real-world scenarios, such as:
- Social networks (users as vertices, friendships as edges)
- Road networks (intersections as vertices, roads as edges)
- Computer networks (devices as vertices, connections as edges)
- Dependency relationships in software (modules as vertices, dependencies as edges)
Graphs can be directed (edges have a specific direction) or undirected (edges have no direction). They can also be weighted (edges have associated values) or unweighted.
Adjacency List: An Overview
An adjacency list is a collection of unordered lists used to represent a graph. Each list describes the set of neighbors of a vertex in the graph. In practice, an adjacency list is often implemented as an array or hash table of linked lists.
Implementing an Adjacency List
Here’s a simple implementation of an adjacency list in Python:
class Graph:
def __init__(self, num_vertices):
self.num_vertices = num_vertices
self.adj_list = [[] for _ in range(num_vertices)]
def add_edge(self, u, v):
self.adj_list[u].append(v)
self.adj_list[v].append(u) # For undirected graph
def print_graph(self):
for vertex in range(self.num_vertices):
print(f"Vertex {vertex}: {self.adj_list[vertex]}")
# Example usage
g = Graph(4)
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 3)
g.print_graph()
Advantages of Adjacency Lists
- Space Efficiency for Sparse Graphs: Adjacency lists are memory-efficient for sparse graphs (graphs with few edges compared to the number of vertices).
- Fast Vertex Operations: Adding or removing a vertex is efficient, as it only requires adding or removing a list.
- Efficient Edge Iteration: Iterating over all edges of a vertex is fast, as you only need to traverse the corresponding list.
Disadvantages of Adjacency Lists
- Slow Edge Weight Queries: Checking if an edge exists between two vertices or finding the weight of an edge can be slower, especially for large graphs.
- Less Cache-Friendly: Due to the use of linked lists, adjacency lists may have poor cache performance.
Adjacency Matrix: An Overview
An adjacency matrix is a 2D array of size V x V, where V is the number of vertices in the graph. The entry matrix[i][j] is 1 (or the edge weight) if there is an edge from vertex i to vertex j, and 0 otherwise.
Implementing an Adjacency Matrix
Here’s a simple implementation of an adjacency matrix in Python:
class Graph:
def __init__(self, num_vertices):
self.num_vertices = num_vertices
self.adj_matrix = [[0 for _ in range(num_vertices)] for _ in range(num_vertices)]
def add_edge(self, u, v, weight=1):
self.adj_matrix[u][v] = weight
self.adj_matrix[v][u] = weight # For undirected graph
def print_graph(self):
for row in self.adj_matrix:
print(row)
# Example usage
g = Graph(4)
g.add_edge(0, 1)
g.add_edge(0, 2)
g.add_edge(1, 2)
g.add_edge(2, 3)
g.print_graph()
Advantages of Adjacency Matrices
- Fast Edge Weight Queries: Checking if an edge exists or finding its weight is an O(1) operation.
- Simple Implementation: Adjacency matrices are straightforward to implement and use.
- Efficient for Dense Graphs: They perform well for dense graphs (graphs with many edges).
Disadvantages of Adjacency Matrices
- Space Inefficiency for Sparse Graphs: They use O(V^2) space regardless of the number of edges, which is inefficient for sparse graphs.
- Slow Vertex Operations: Adding or removing a vertex requires resizing the entire matrix, which can be costly.
- Inefficient Edge Iteration: To find all edges of a vertex, you need to scan an entire row or column, which is O(V) even if the vertex has few edges.
When to Use an Adjacency List
Adjacency lists are generally preferred in the following scenarios:
- Sparse Graphs: When the number of edges is much less than V^2, adjacency lists are more space-efficient.
- Dynamic Graphs: If you frequently need to add or remove vertices, adjacency lists handle these operations more efficiently.
- Memory-Constrained Environments: In situations where memory is a concern, especially for large graphs, adjacency lists are often the better choice.
- Algorithms Requiring Edge Iteration: For algorithms that frequently need to iterate over all edges of a vertex (e.g., depth-first search, breadth-first search), adjacency lists provide faster access.
Example: Social Network Analysis
Consider a social network application where users (vertices) can be friends with other users (edges). In most social networks, the number of connections (friends) for each user is relatively small compared to the total number of users. This makes it a sparse graph, ideal for an adjacency list representation.
class SocialNetwork:
def __init__(self):
self.users = {}
def add_user(self, user_id):
if user_id not in self.users:
self.users[user_id] = set()
def add_friendship(self, user1, user2):
self.add_user(user1)
self.add_user(user2)
self.users[user1].add(user2)
self.users[user2].add(user1)
def get_friends(self, user_id):
return self.users.get(user_id, set())
# Example usage
network = SocialNetwork()
network.add_friendship("Alice", "Bob")
network.add_friendship("Alice", "Charlie")
network.add_friendship("Bob", "David")
print(f"Alice's friends: {network.get_friends('Alice')}")
print(f"Bob's friends: {network.get_friends('Bob')}")
In this example, using an adjacency list (implemented as a dictionary of sets) allows for efficient addition of new users and friendships, as well as quick retrieval of a user’s friends.
When to Use an Adjacency Matrix
Adjacency matrices are typically preferred in the following situations:
- Dense Graphs: When the number of edges is close to V^2, adjacency matrices don’t waste much space and provide constant-time edge queries.
- Small Graphs: For graphs with a small number of vertices, the simplicity and constant-time edge queries of matrices can outweigh their space inefficiency.
- Weighted Graphs: When you need to frequently update or query edge weights, matrices provide O(1) access.
- Algorithms Requiring Quick Edge Existence Checks: If your algorithm frequently needs to check if an edge exists between two vertices, matrices offer constant-time lookups.
Example: Game Board Connectivity
Consider a game board where each cell is connected to its adjacent cells. This forms a grid-like graph structure, which is typically dense (each cell is connected to up to 4 neighbors). An adjacency matrix can be an efficient representation for this scenario.
class GameBoard:
def __init__(self, size):
self.size = size
self.board = [[0 for _ in range(size)] for _ in range(size)]
def connect_cells(self, x1, y1, x2, y2):
if self.is_valid_cell(x1, y1) and self.is_valid_cell(x2, y2):
cell1 = x1 * self.size + y1
cell2 = x2 * self.size + y2
self.board[cell1][cell2] = 1
self.board[cell2][cell1] = 1
def is_valid_cell(self, x, y):
return 0 <= x < self.size and 0 <= y < self.size
def are_connected(self, x1, y1, x2, y2):
if self.is_valid_cell(x1, y1) and self.is_valid_cell(x2, y2):
cell1 = x1 * self.size + y1
cell2 = x2 * self.size + y2
return self.board[cell1][cell2] == 1
return False
# Example usage
game = GameBoard(3)
game.connect_cells(0, 0, 0, 1)
game.connect_cells(0, 1, 1, 1)
game.connect_cells(1, 1, 2, 1)
print(f"(0,0) connected to (0,1): {game.are_connected(0, 0, 0, 1)}")
print(f"(0,0) connected to (1,1): {game.are_connected(0, 0, 1, 1)}")
In this example, the adjacency matrix allows for constant-time checks of cell connectivity, which is crucial for efficient game logic processing.
Performance Comparison
To better understand when to choose between adjacency lists and matrices, let’s compare their performance for common operations:
Operation | Adjacency List | Adjacency Matrix |
---|---|---|
Add Vertex | O(1) | O(V^2) |
Remove Vertex | O(V + E) | O(V^2) |
Add Edge | O(1) | O(1) |
Remove Edge | O(V) | O(1) |
Query: Is there an edge between u and v? | O(V) | O(1) |
Space Complexity | O(V + E) | O(V^2) |
As you can see, adjacency lists excel in space efficiency and vertex operations, while adjacency matrices shine in edge queries and modifications.
Hybrid Approaches
In some cases, a hybrid approach combining elements of both adjacency lists and matrices can be beneficial. For example:
- Adjacency List with Hash Tables: Instead of using linked lists, you can use hash tables to store adjacent vertices. This improves the edge query time to O(1) on average, while maintaining the space efficiency of adjacency lists for sparse graphs.
- Compressed Sparse Row (CSR): This format combines the space efficiency of adjacency lists with the cache-friendly nature of arrays, making it suitable for large, sparse graphs in high-performance computing scenarios.
Example: Adjacency List with Hash Tables
from collections import defaultdict
class Graph:
def __init__(self):
self.graph = defaultdict(dict)
def add_edge(self, u, v, weight=1):
self.graph[u][v] = weight
self.graph[v][u] = weight # For undirected graph
def has_edge(self, u, v):
return v in self.graph[u]
def get_weight(self, u, v):
return self.graph[u].get(v, None)
# Example usage
g = Graph()
g.add_edge(0, 1, 4)
g.add_edge(0, 2, 3)
g.add_edge(1, 2, 2)
g.add_edge(2, 3, 1)
print(f"Edge between 0 and 1: {g.has_edge(0, 1)}")
print(f"Weight of edge (0, 2): {g.get_weight(0, 2)}")
This implementation combines the space efficiency of adjacency lists with the fast edge queries of adjacency matrices, making it a versatile choice for many graph applications.
Considerations for Technical Interviews
When preparing for technical interviews, especially for major tech companies, it’s crucial to understand both adjacency lists and matrices. Here are some tips:
- Analyze the Problem: Before choosing a representation, analyze the given problem. Consider the graph’s density, the required operations, and any space or time constraints mentioned in the problem statement.
- Justify Your Choice: Be prepared to explain why you chose a particular representation. This demonstrates your understanding of the trade-offs involved.
- Know How to Implement Both: Practice implementing both adjacency lists and matrices. You should be comfortable coding either representation quickly and correctly.
- Understand Common Algorithms: Be familiar with how common graph algorithms (e.g., DFS, BFS, Dijkstra’s) work with both representations.
- Consider Space Complexity: In interviews, space complexity can be as important as time complexity. Be ready to discuss the space requirements of your chosen representation.
Conclusion
Choosing between an adjacency list and an adjacency matrix is a fundamental decision in graph representation that can significantly impact the performance and efficiency of your algorithms. By understanding the strengths and weaknesses of each approach, you can make informed decisions that optimize your code for specific scenarios.
Remember that the best choice often depends on the specific requirements of your problem:
- Use adjacency lists for sparse graphs, when memory is a concern, or when you need to frequently add or remove vertices.
- Opt for adjacency matrices for dense graphs, small graphs, or when you need constant-time edge weight queries.
- Consider hybrid approaches for more specialized scenarios that require balancing the advantages of both representations.
As you continue to develop your programming skills and prepare for technical interviews, practice working with both representations. This will give you the flexibility to choose the most appropriate structure for any graph problem you encounter, setting you up for success in your coding projects and future career in tech.