Strategies for Solving Palindrome Problems: A Comprehensive Guide
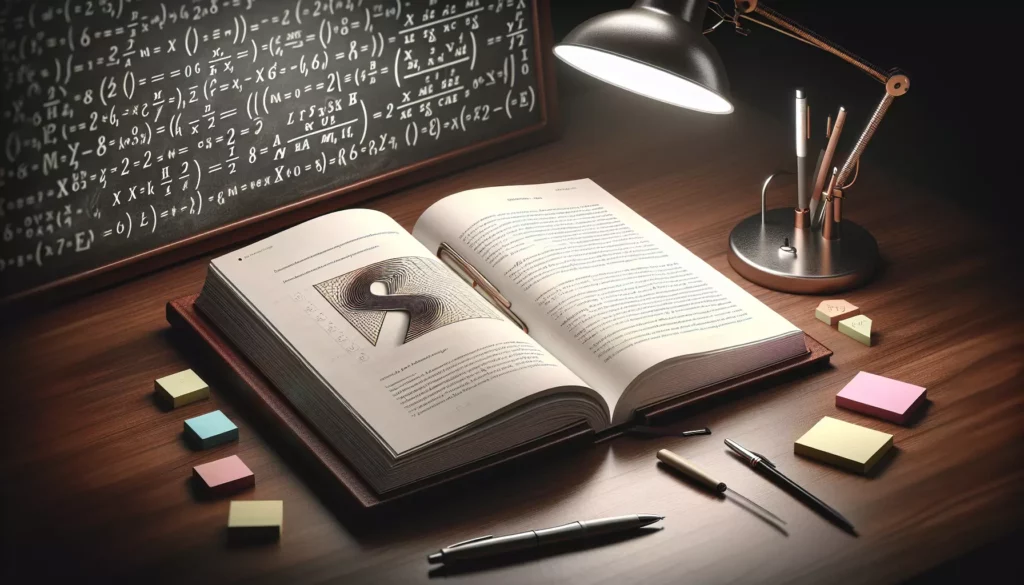
Welcome to our in-depth guide on solving palindrome problems! If you’re preparing for coding interviews or simply looking to enhance your algorithmic skills, understanding palindromes and the strategies to work with them is crucial. In this article, we’ll explore various approaches to tackle palindrome-related challenges, from basic concepts to advanced techniques. Let’s dive in!
Table of Contents
- What is a Palindrome?
- The Importance of Palindromes in Coding Interviews
- Basic Strategies for Palindrome Problems
- Advanced Techniques for Palindrome Manipulation
- Common Palindrome Problems and Solutions
- Optimizing Palindrome Solutions
- Real-world Applications of Palindrome Algorithms
- Practice Problems to Hone Your Skills
- Conclusion
1. What is a Palindrome?
Before we delve into solving palindrome problems, let’s ensure we have a solid understanding of what a palindrome is. A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward, disregarding spaces, punctuation, and capitalization.
Examples of palindromes include:
- Words: “radar”, “level”, “deified”
- Phrases: “A man, a plan, a canal: Panama”, “Never odd or even”
- Numbers: 12321, 45654, 11611
Understanding this concept is fundamental to solving palindrome-related problems efficiently.
2. The Importance of Palindromes in Coding Interviews
Palindrome problems are popular in coding interviews for several reasons:
- Algorithmic Thinking: They test a candidate’s ability to manipulate strings and arrays, a fundamental skill in programming.
- Problem-Solving Skills: Palindrome problems often require creative approaches and can be solved using various techniques, showcasing a candidate’s problem-solving abilities.
- Efficiency: Many palindrome problems can be optimized, allowing interviewers to assess a candidate’s ability to improve upon basic solutions.
- Versatility: Palindrome concepts can be applied to strings, numbers, and even linked lists, making them versatile interview questions.
Given their significance, mastering palindrome problems can significantly boost your confidence and performance in technical interviews.
3. Basic Strategies for Palindrome Problems
Let’s start with some fundamental strategies for solving palindrome problems:
3.1. Two-Pointer Technique
The two-pointer technique is a simple and effective method for checking if a string is a palindrome:
def is_palindrome(s):
left = 0
right = len(s) - 1
while left < right:
if s[left] != s[right]:
return False
left += 1
right -= 1
return True
# Example usage
print(is_palindrome("radar")) # Output: True
print(is_palindrome("hello")) # Output: False
This approach uses two pointers, one starting from the beginning and the other from the end of the string. It compares characters moving towards the center, returning false if any mismatch is found.
3.2. Reverse and Compare
Another straightforward method is to reverse the string and compare it with the original:
def is_palindrome(s):
return s == s[::-1]
# Example usage
print(is_palindrome("level")) # Output: True
print(is_palindrome("python")) # Output: False
This method is concise but may not be as efficient for very long strings due to the creation of a new reversed string.
3.3. Recursive Approach
For those who prefer recursive solutions, here’s a recursive approach to check for palindromes:
def is_palindrome(s):
if len(s) <= 1:
return True
return s[0] == s[-1] and is_palindrome(s[1:-1])
# Example usage
print(is_palindrome("kayak")) # Output: True
print(is_palindrome("algorithm")) # Output: False
This recursive method checks the outermost characters and then recursively checks the inner substring.
4. Advanced Techniques for Palindrome Manipulation
As we move beyond basic palindrome checking, let’s explore some advanced techniques for palindrome manipulation:
4.1. Manacher’s Algorithm
Manacher’s Algorithm is an efficient technique for finding the longest palindromic substring in linear time. While it’s more complex than basic approaches, it’s incredibly useful for optimizing certain palindrome problems:
def manacher(s):
t = '#'.join('^{}$'.format(s))
n = len(t)
P = [0] * n
C = R = 0
for i in range(1, n-1):
P[i] = (R > i) and min(R - i, P[2*C - i])
while t[i + 1 + P[i]] == t[i - 1 - P[i]]:
P[i] += 1
if i + P[i] > R:
C, R = i, i + P[i]
max_len, center_index = max((n, i) for i, n in enumerate(P))
return s[(center_index - max_len)//2: (center_index + max_len)//2]
# Example usage
print(manacher("babad")) # Output: "bab" or "aba"
print(manacher("cbbd")) # Output: "bb"
Manacher’s Algorithm uses dynamic programming to efficiently find the longest palindromic substring in O(n) time complexity.
4.2. Palindromic Substrings
Finding all palindromic substrings is another common problem. Here’s an efficient approach using dynamic programming:
def count_palindromic_substrings(s):
n = len(s)
dp = [[False] * n for _ in range(n)]
count = 0
# All substrings of length 1 are palindromes
for i in range(n):
dp[i][i] = True
count += 1
# Check for substrings of length 2
for i in range(n - 1):
if s[i] == s[i + 1]:
dp[i][i + 1] = True
count += 1
# Check for substrings of length 3 or more
for length in range(3, n + 1):
for i in range(n - length + 1):
j = i + length - 1
if s[i] == s[j] and dp[i + 1][j - 1]:
dp[i][j] = True
count += 1
return count
# Example usage
print(count_palindromic_substrings("aaa")) # Output: 6
print(count_palindromic_substrings("abc")) # Output: 3
This approach uses a 2D DP table to store whether substrings are palindromes, allowing for efficient counting of all palindromic substrings.
5. Common Palindrome Problems and Solutions
Let’s explore some common palindrome problems you might encounter in coding interviews, along with their solutions:
5.1. Longest Palindromic Substring
Problem: Given a string, find the longest palindromic substring.
def longest_palindrome_substring(s):
if not s:
return ""
start = 0
max_length = 1
for i in range(len(s)):
# Check for odd-length palindromes
left, right = i, i
while left >= 0 and right < len(s) and s[left] == s[right]:
if right - left + 1 > max_length:
start = left
max_length = right - left + 1
left -= 1
right += 1
# Check for even-length palindromes
left, right = i, i + 1
while left >= 0 and right < len(s) and s[left] == s[right]:
if right - left + 1 > max_length:
start = left
max_length = right - left + 1
left -= 1
right += 1
return s[start:start + max_length]
# Example usage
print(longest_palindrome_substring("babad")) # Output: "bab" or "aba"
print(longest_palindrome_substring("cbbd")) # Output: "bb"
5.2. Palindrome Partitioning
Problem: Given a string s, partition s such that every substring of the partition is a palindrome. Return all possible palindrome partitioning of s.
def palindrome_partitioning(s):
def is_palindrome(start, end):
while start < end:
if s[start] != s[end]:
return False
start += 1
end -= 1
return True
def backtrack(start, path):
if start == len(s):
result.append(path[:])
return
for end in range(start, len(s)):
if is_palindrome(start, end):
path.append(s[start:end+1])
backtrack(end + 1, path)
path.pop()
result = []
backtrack(0, [])
return result
# Example usage
print(palindrome_partitioning("aab")) # Output: [["a","a","b"], ["aa","b"]]
5.3. Palindrome Number
Problem: Determine whether an integer is a palindrome. An integer is a palindrome when it reads the same backward as forward.
def is_palindrome_number(x):
if x < 0:
return False
original = x
reversed_num = 0
while x != 0:
digit = x % 10
reversed_num = reversed_num * 10 + digit
x //= 10
return original == reversed_num
# Example usage
print(is_palindrome_number(121)) # Output: True
print(is_palindrome_number(-121)) # Output: False
print(is_palindrome_number(10)) # Output: False
6. Optimizing Palindrome Solutions
Optimizing palindrome solutions often involves reducing time complexity or space usage. Here are some tips for optimization:
6.1. Use In-place Operations
Whenever possible, try to perform operations in-place to save space. For example, when reversing a string to check for palindromes, consider using two pointers instead of creating a new reversed string.
6.2. Avoid Unnecessary Computations
In palindrome checking, you only need to compare up to the middle of the string. Implement checks to stop early when a non-palindrome is detected.
6.3. Utilize Dynamic Programming
For problems involving multiple palindrome checks (like finding all palindromic substrings), consider using dynamic programming to store intermediate results and avoid redundant computations.
6.4. Consider Bit Manipulation
For certain palindrome problems involving integers, bit manipulation techniques can lead to more efficient solutions.
7. Real-world Applications of Palindrome Algorithms
Palindrome algorithms have various practical applications beyond coding interviews:
- Bioinformatics: Palindromic sequences in DNA are important in molecular biology for processes like DNA replication and transcription.
- Natural Language Processing: Palindrome detection can be used in text analysis and generation tasks.
- Data Compression: Some compression algorithms utilize palindromic patterns to achieve better compression ratios.
- Cryptography: Palindromes can be used in certain encryption and decryption schemes.
- Database Optimization: Palindrome-based algorithms can be used to optimize certain database operations, especially in text-heavy databases.
8. Practice Problems to Hone Your Skills
To further improve your palindrome problem-solving skills, try these practice problems:
- Longest Palindromic Subsequence
- Count All Palindromic Subsequences
- Shortest Palindrome (Add characters in front of a string to make it a palindrome)
- Palindrome Pairs (Given a list of words, find all pairs of unique indices such that the concatenation of the two words is a palindrome)
- Palindrome Permutation (Determine if a given string can be rearranged to form a palindrome)
Remember to start with a brute-force approach, then work on optimizing your solution. Practice implementing these problems in your preferred programming language to build muscle memory and improve your coding skills.
9. Conclusion
Mastering palindrome problems is an essential skill for any programmer preparing for technical interviews or looking to enhance their algorithmic thinking. From basic string manipulation to advanced dynamic programming techniques, palindrome problems offer a wide range of challenges that can significantly improve your problem-solving abilities.
Remember that the key to excelling at palindrome problems (and coding interviews in general) is consistent practice. Start with the basic strategies we’ve discussed, then gradually move on to more complex problems and optimization techniques. Don’t be discouraged if you find some problems challenging at first – with persistence and practice, you’ll develop the skills and intuition needed to tackle even the most complex palindrome problems.
As you continue your journey in programming and interview preparation, keep exploring new problems and techniques. Palindromes are just one of many fascinating areas in computer science and algorithm design. Stay curious, keep coding, and best of luck in your future interviews and programming endeavors!