How to Handle Concurrent Modifications in Software Development
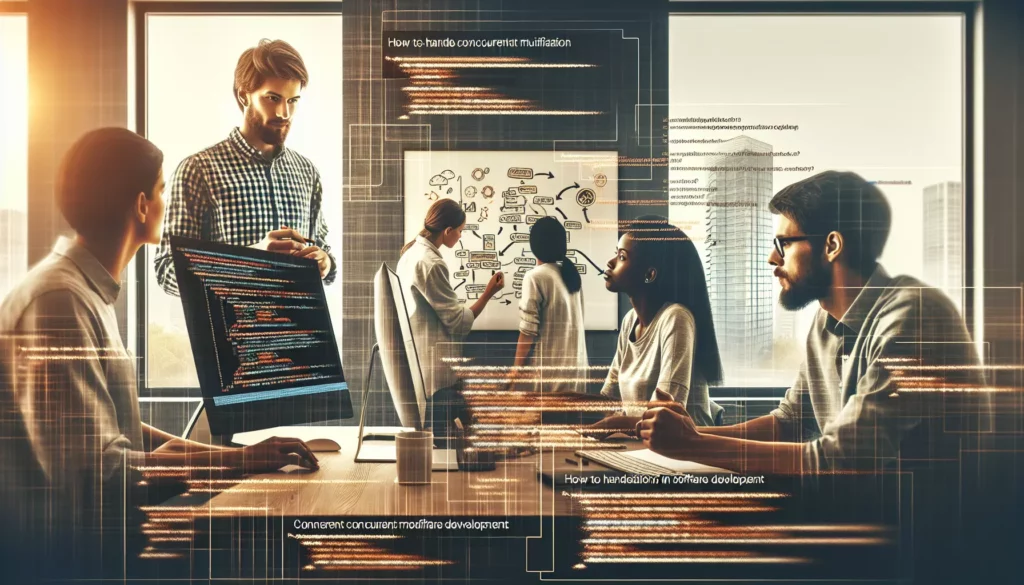
In the world of software development, managing concurrent modifications is a crucial skill that every programmer must master. As systems become more complex and distributed, the likelihood of multiple users or processes attempting to modify the same data simultaneously increases. This can lead to data inconsistencies, race conditions, and other synchronization issues that can compromise the integrity and reliability of your software. In this comprehensive guide, we’ll explore various strategies and techniques to effectively handle concurrent modifications in your applications.
Understanding Concurrent Modifications
Before diving into solutions, it’s essential to understand what concurrent modifications are and why they pose a challenge in software development. Concurrent modifications occur when multiple threads, processes, or users attempt to access and modify shared resources simultaneously. This can happen in various scenarios, such as:
- Multiple users editing the same document in a collaborative editing tool
- Several transactions updating the same database record
- Multiple threads accessing and modifying shared variables in a multithreaded application
The primary challenge with concurrent modifications is maintaining data consistency and preventing conflicts that can lead to unexpected behavior or data corruption. Let’s explore some common approaches to handle these situations effectively.
1. Locking Mechanisms
One of the most straightforward approaches to handling concurrent modifications is through the use of locking mechanisms. Locks ensure that only one thread or process can access a shared resource at a time, preventing simultaneous modifications.
Types of Locks
- Exclusive Locks (Write Locks): Allow only one thread to access the resource for reading or writing.
- Shared Locks (Read Locks): Allow multiple threads to read the resource simultaneously but prevent writing.
- Optimistic Locks: Allow concurrent access but verify that no changes have occurred before committing modifications.
- Pessimistic Locks: Prevent concurrent access by locking the resource before any operation.
Implementing Locks in Java
Here’s an example of how to implement a simple lock using Java’s synchronized
keyword:
public class SharedResource {
private int value;
public synchronized void updateValue(int newValue) {
this.value = newValue;
}
public synchronized int getValue() {
return this.value;
}
}
In this example, the synchronized
keyword ensures that only one thread can execute the updateValue
or getValue
method at a time, preventing concurrent modifications.
Advantages and Disadvantages of Locking
Advantages:
- Simple to implement and understand
- Effective for preventing concurrent access
Disadvantages:
- Can lead to performance bottlenecks, especially with high contention
- Risk of deadlocks if not implemented carefully
- May be too restrictive for scenarios where concurrent read access is acceptable
2. Optimistic Concurrency Control
Optimistic concurrency control (OCC) is an approach that allows multiple transactions to proceed without locking the data. Instead, it checks for conflicts at the time of commit and rolls back transactions if conflicts are detected.
How OCC Works
- When a transaction starts, it takes a snapshot of the data.
- The transaction proceeds with its operations without locking the data.
- Before committing, the system checks if the data has been modified since the snapshot was taken.
- If no modifications have occurred, the transaction commits successfully.
- If modifications are detected, the transaction is rolled back, and the process may be retried.
Implementing OCC in a Database Context
Here’s an example of how you might implement OCC in a database context using SQL:
-- Start transaction
BEGIN TRANSACTION;
-- Read the current version of the record
SELECT version FROM users WHERE id = 1;
-- Perform updates
UPDATE users SET name = 'John Doe', version = version + 1
WHERE id = 1 AND version = @original_version;
-- Check if the update was successful
IF @@ROWCOUNT = 0
-- Conflict detected, rollback the transaction
ROLLBACK TRANSACTION;
ELSE
-- No conflict, commit the transaction
COMMIT TRANSACTION;
END IF;
In this example, we use a version column to detect conflicts. If the version hasn’t changed since we read it, the update succeeds. Otherwise, it fails, indicating a concurrent modification.
Advantages and Disadvantages of OCC
Advantages:
- High concurrency in read-heavy scenarios
- No need for locking, reducing the risk of deadlocks
- Works well in distributed systems
Disadvantages:
- Can lead to wasted work if conflicts are frequent
- May require complex conflict resolution strategies
- Not suitable for high-contention scenarios
3. Conflict-free Replicated Data Types (CRDTs)
Conflict-free Replicated Data Types (CRDTs) are a class of data structures designed to support concurrent updates without coordination between replicas. CRDTs ensure that all replicas converge to the same state, regardless of the order in which updates are applied.
Types of CRDTs
- State-based CRDTs (CvRDTs): Propagate the entire state between replicas.
- Operation-based CRDTs (CmRDTs): Propagate only the operations between replicas.
Example: Implementing a Counter CRDT
Here’s a simple example of a state-based counter CRDT implemented in Python:
class GCounter:
def __init__(self):
self.counts = {}
def increment(self, replica_id):
if replica_id not in self.counts:
self.counts[replica_id] = 0
self.counts[replica_id] += 1
def merge(self, other):
for replica_id, count in other.counts.items():
if replica_id not in self.counts or self.counts[replica_id] < count:
self.counts[replica_id] = count
def value(self):
return sum(self.counts.values())
# Usage
counter1 = GCounter()
counter2 = GCounter()
counter1.increment("replica1")
counter1.increment("replica1")
counter2.increment("replica2")
counter1.merge(counter2)
counter2.merge(counter1)
print(counter1.value()) # Output: 3
print(counter2.value()) # Output: 3
In this example, each replica maintains its own count, and the merge operation takes the maximum count for each replica. This ensures that the counters converge to the same value, regardless of the order of operations.
Advantages and Disadvantages of CRDTs
Advantages:
- Excellent for distributed systems with eventual consistency
- No need for coordination between replicas
- Automatically resolve conflicts
Disadvantages:
- Can be complex to implement for certain data types
- May require more storage and bandwidth due to metadata
- Not suitable for all types of data or operations
4. Event Sourcing
Event Sourcing is an architectural pattern where the state of an application is determined by a sequence of events. Instead of storing the current state, Event Sourcing stores the full history of actions taken on the data.
Key Concepts of Event Sourcing
- Events: Immutable records of something that happened in the system.
- Event Store: A database that stores the sequence of events.
- Projections: Views of the data built by applying events in sequence.
Implementing Event Sourcing
Here’s a simplified example of how Event Sourcing might be implemented in C#:
public class BankAccount
{
public Guid Id { get; private set; }
public decimal Balance { get; private set; }
private List<object> _changes = new List<object>();
public BankAccount(Guid id)
{
Id = id;
Balance = 0;
}
public void Deposit(decimal amount)
{
Apply(new DepositedEvent(Id, amount));
}
public void Withdraw(decimal amount)
{
if (Balance < amount)
throw new InvalidOperationException("Insufficient funds");
Apply(new WithdrawnEvent(Id, amount));
}
private void Apply(object @event)
{
When(@event);
_changes.Add(@event);
}
private void When(object @event)
{
switch (@event)
{
case DepositedEvent e:
Balance += e.Amount;
break;
case WithdrawnEvent e:
Balance -= e.Amount;
break;
}
}
public IEnumerable<object> GetUncommittedChanges()
{
return _changes.AsEnumerable();
}
public void ClearUncommittedChanges()
{
_changes.Clear();
}
}
public class DepositedEvent
{
public Guid AccountId { get; }
public decimal Amount { get; }
public DepositedEvent(Guid accountId, decimal amount)
{
AccountId = accountId;
Amount = amount;
}
}
public class WithdrawnEvent
{
public Guid AccountId { get; }
public decimal Amount { get; }
public WithdrawnEvent(Guid accountId, decimal amount)
{
AccountId = accountId;
Amount = amount;
}
}
In this example, all changes to the bank account are recorded as events. The current state (balance) is derived by applying these events in sequence.
Advantages and Disadvantages of Event Sourcing
Advantages:
- Provides a complete audit trail of all changes
- Enables easy debugging and historical analysis
- Facilitates building multiple views of the same data
Disadvantages:
- Can be complex to implement and maintain
- May require significant storage for event history
- Querying current state can be challenging and may require separate read models
5. Distributed Consensus Algorithms
In distributed systems, handling concurrent modifications often requires achieving consensus among multiple nodes. Distributed consensus algorithms help ensure that all nodes in a distributed system agree on the same data or state, even in the presence of failures or network partitions.
Popular Consensus Algorithms
- Paxos: A family of protocols for solving consensus in a network of unreliable processors.
- Raft: A consensus algorithm designed to be more understandable than Paxos, often used in distributed systems.
- Zab (ZooKeeper Atomic Broadcast): Used in Apache ZooKeeper for maintaining consistency in distributed systems.
Example: Implementing a Simple Consensus Protocol
Here’s a simplified example of a basic consensus protocol implemented in Python:
import random
class Node:
def __init__(self, id):
self.id = id
self.value = None
self.round = 0
self.accepted = False
def propose(self, value):
self.value = value
self.round += 1
return (self.round, self.id, self.value)
def receive(self, proposal):
proposal_round, proposer_id, proposal_value = proposal
if proposal_round > self.round:
self.round = proposal_round
self.value = proposal_value
self.accepted = True
return True
return False
def run_consensus(nodes, rounds):
for _ in range(rounds):
proposer = random.choice(nodes)
proposal = proposer.propose(proposer.value)
accepted_count = 0
for node in nodes:
if node.receive(proposal):
accepted_count += 1
if accepted_count > len(nodes) // 2:
return proposer.value
return None
# Usage
nodes = [Node(i) for i in range(5)]
for node in nodes:
node.value = random.randint(1, 100)
consensus_value = run_consensus(nodes, 10)
print(f"Consensus reached: {consensus_value}")
This example implements a basic consensus protocol where nodes propose values and accept proposals with higher round numbers. Consensus is reached when a majority of nodes accept a proposal.
Advantages and Disadvantages of Distributed Consensus
Advantages:
- Ensures consistency across distributed systems
- Provides fault tolerance and high availability
- Supports strong consistency models
Disadvantages:
- Can be complex to implement correctly
- May introduce latency due to communication overhead
- Can be challenging to scale to very large systems
Best Practices for Handling Concurrent Modifications
Regardless of the specific technique you choose, there are some general best practices to follow when dealing with concurrent modifications:
- Identify Shared Resources: Clearly identify which resources in your system are subject to concurrent access and modification.
- Choose the Right Technique: Select the appropriate concurrency control mechanism based on your specific use case, considering factors like read/write ratios, distribution requirements, and consistency needs.
- Use Atomic Operations: Whenever possible, use atomic operations provided by your programming language or database to simplify concurrency management.
- Minimize the Duration of Locks: If using locks, keep the locked sections as short as possible to reduce contention.
- Implement Proper Error Handling: Design your system to gracefully handle concurrency-related errors, such as deadlocks or optimistic locking failures.
- Test Thoroughly: Concurrency issues can be difficult to reproduce and debug. Implement comprehensive testing, including stress tests and race condition scenarios.
- Monitor and Profile: Use monitoring and profiling tools to identify concurrency bottlenecks and optimize your implementation.
- Consider Eventual Consistency: In some cases, eventual consistency may be sufficient and can simplify your system design.
- Document Your Approach: Clearly document your concurrency control strategy to help other developers understand and maintain the system.
Conclusion
Handling concurrent modifications is a critical aspect of modern software development, especially as systems become more distributed and complex. By understanding the various techniques available – from simple locking mechanisms to advanced concepts like CRDTs and distributed consensus algorithms – you can choose the most appropriate solution for your specific use case.
Remember that there’s no one-size-fits-all solution to concurrency challenges. The best approach depends on your system’s requirements, architecture, and constraints. By following best practices and carefully considering the trade-offs of each technique, you can build robust, scalable systems that effectively manage concurrent modifications.
As you continue to develop your skills in this area, practice implementing these concepts in your projects, and stay updated with the latest research and tools in concurrent programming. With experience and careful design, you’ll be well-equipped to tackle even the most challenging concurrency scenarios in your software development career.