When to Consider Using a Greedy Approach in Algorithm Design
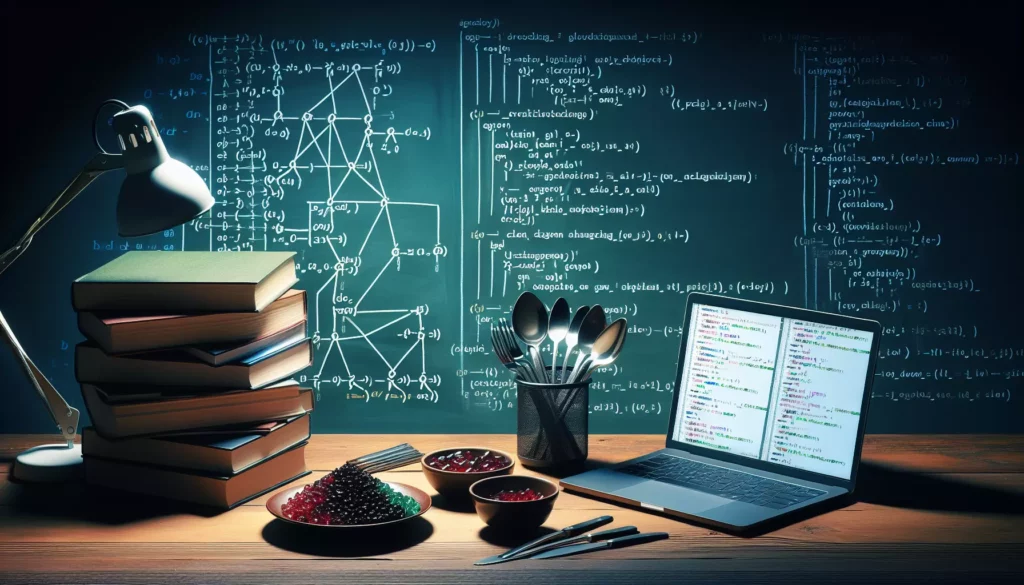
In the world of algorithm design and problem-solving, the greedy approach stands out as a powerful yet sometimes misunderstood strategy. As aspiring programmers and seasoned developers alike navigate the complexities of coding challenges, understanding when and how to apply greedy algorithms can be a game-changer. This comprehensive guide will delve into the intricacies of greedy algorithms, exploring their strengths, limitations, and the scenarios where they shine brightest.
What is a Greedy Algorithm?
Before we dive into the when and why of using greedy algorithms, let’s establish a clear understanding of what they are. A greedy algorithm is a problem-solving heuristic that makes the locally optimal choice at each step, with the hope of finding a global optimum. In simpler terms, it’s an algorithmic paradigm that follows the problem-solving heuristic of making the best possible decision at the moment without worrying about future consequences.
The key characteristics of a greedy algorithm include:
- Making locally optimal choices at each step
- Never reconsidering these choices
- Hoping that these local optima will lead to a global optimum
While this approach can lead to efficient and straightforward solutions for many problems, it’s not always guaranteed to find the best overall solution. This is why understanding when to use greedy algorithms is crucial for any programmer or algorithm designer.
The Advantages of Greedy Algorithms
Greedy algorithms come with several advantages that make them attractive in certain scenarios:
- Simplicity: Greedy algorithms are often easier to understand, implement, and debug compared to more complex approaches like dynamic programming.
- Efficiency: In many cases, greedy algorithms can provide solutions quickly, making them suitable for real-time applications or large-scale problems.
- Space Efficiency: Greedy algorithms typically require less memory as they make decisions on-the-go without storing multiple states or solutions.
- Approximation: Even when they don’t provide the optimal solution, greedy algorithms can often find a reasonably good approximation in a fraction of the time required for an exact solution.
When to Consider Using a Greedy Approach
Now that we understand the basics and benefits of greedy algorithms, let’s explore the scenarios where they are most effective:
1. Optimization Problems with Local Choice Property
Greedy algorithms excel in problems where making the locally optimal choice at each step leads to a globally optimal solution. This is known as the “greedy choice property.” If a problem exhibits this property, a greedy approach is often the best way to go.
Example: Coin Change Problem
Consider a scenario where you need to make change for a given amount using the minimum number of coins. If your coin denominations are “greedy friendly” (e.g., {1, 5, 10, 25}), a greedy approach works perfectly.
def coin_change(amount, coins):
coins.sort(reverse=True)
result = []
for coin in coins:
while amount >= coin:
amount -= coin
result.append(coin)
return result
print(coin_change(63, [1, 5, 10, 25])) # Output: [25, 25, 10, 1, 1, 1]
In this case, always choosing the largest possible coin leads to the optimal solution.
2. Problems with the Matroid Property
A matroid is a mathematical structure that generalizes the notion of linear independence in vector spaces. Many optimization problems that possess the matroid property can be solved optimally using a greedy approach.
Example: Kruskal’s Algorithm for Minimum Spanning Tree
Finding the minimum spanning tree of a graph is a classic problem that exhibits the matroid property. Kruskal’s algorithm, which is greedy in nature, always produces the optimal solution.
class UnionFind:
def __init__(self, vertices):
self.parent = {v: v for v in vertices}
self.rank = {v: 0 for v in vertices}
def find(self, item):
if self.parent[item] != item:
self.parent[item] = self.find(self.parent[item])
return self.parent[item]
def union(self, x, y):
xroot = self.find(x)
yroot = self.find(y)
if self.rank[xroot] < self.rank[yroot]:
self.parent[xroot] = yroot
elif self.rank[xroot] > self.rank[yroot]:
self.parent[yroot] = xroot
else:
self.parent[yroot] = xroot
self.rank[xroot] += 1
def kruskal_mst(graph):
edges = [(w, u, v) for u in graph for v, w in graph[u].items()]
edges.sort()
vertices = list(graph.keys())
uf = UnionFind(vertices)
mst = []
for w, u, v in edges:
if uf.find(u) != uf.find(v):
uf.union(u, v)
mst.append((u, v, w))
return mst
graph = {
'A': {'B': 4, 'C': 2},
'B': {'A': 4, 'C': 1, 'D': 5},
'C': {'A': 2, 'B': 1, 'D': 8, 'E': 10},
'D': {'B': 5, 'C': 8, 'E': 2, 'F': 6},
'E': {'C': 10, 'D': 2, 'F': 3},
'F': {'D': 6, 'E': 3}
}
print(kruskal_mst(graph))
# Output: [('B', 'C', 1), ('A', 'C', 2), ('D', 'E', 2), ('E', 'F', 3), ('A', 'B', 4)]
3. Interval Scheduling Problems
Many scheduling problems, particularly those involving non-overlapping intervals, can be efficiently solved using greedy algorithms.
Example: Activity Selection Problem
Given a set of activities with start and finish times, select the maximum number of non-overlapping activities.
def activity_selection(activities):
# Sort activities by finish time
activities.sort(key=lambda x: x[1])
selected = [activities[0]]
last_finish = activities[0][1]
for activity in activities[1:]:
if activity[0] >= last_finish:
selected.append(activity)
last_finish = activity[1]
return selected
activities = [(1, 4), (3, 5), (0, 6), (5, 7), (3, 8), (5, 9), (6, 10), (8, 11), (8, 12), (2, 13), (12, 14)]
print(activity_selection(activities))
# Output: [(1, 4), (5, 7), (8, 11), (12, 14)]
4. Data Compression Scenarios
Some data compression algorithms, like Huffman coding, use greedy approaches to achieve efficient compression ratios.
Example: Huffman Coding
Huffman coding is a greedy algorithm used for lossless data compression. It assigns variable-length codes to characters based on their frequencies, with more frequent characters getting shorter codes.
import heapq
from collections import defaultdict
class Node:
def __init__(self, char, freq):
self.char = char
self.freq = freq
self.left = None
self.right = None
def __lt__(self, other):
return self.freq < other.freq
def build_huffman_tree(text):
frequency = defaultdict(int)
for char in text:
frequency[char] += 1
heap = [Node(char, freq) for char, freq in frequency.items()]
heapq.heapify(heap)
while len(heap) > 1:
left = heapq.heappop(heap)
right = heapq.heappop(heap)
merged = Node(None, left.freq + right.freq)
merged.left = left
merged.right = right
heapq.heappush(heap, merged)
return heap[0]
def build_codes(node, current_code, codes):
if node is None:
return
if node.char is not None:
codes[node.char] = current_code
return
build_codes(node.left, current_code + "0", codes)
build_codes(node.right, current_code + "1", codes)
def huffman_encoding(text):
root = build_huffman_tree(text)
codes = {}
build_codes(root, "", codes)
encoded_text = ""
for char in text:
encoded_text += codes[char]
return encoded_text, codes
text = "this is an example for huffman encoding"
encoded_text, codes = huffman_encoding(text)
print("Encoded text:", encoded_text)
print("Huffman Codes:", codes)
5. Problems with Optimal Substructure
While optimal substructure is often associated with dynamic programming, some problems with this property can be solved greedily if they also possess the greedy choice property.
Example: Dijkstra’s Algorithm for Shortest Path
Dijkstra’s algorithm is a greedy approach to finding the shortest path in a weighted graph with non-negative edges.
import heapq
def dijkstra(graph, start):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
graph = {
'A': {'B': 4, 'C': 2},
'B': {'D': 3, 'E': 1},
'C': {'B': 1, 'D': 5},
'D': {'E': 2},
'E': {}
}
print(dijkstra(graph, 'A'))
# Output: {'A': 0, 'B': 3, 'C': 2, 'D': 6, 'E': 4}
Limitations and Pitfalls of Greedy Algorithms
While greedy algorithms can be powerful tools in a programmer’s arsenal, they’re not without their limitations:
- Not Always Optimal: Greedy algorithms don’t always produce the best possible solution. They can get stuck in local optima, missing the global optimum.
- Problem-Specific: The effectiveness of a greedy approach heavily depends on the problem structure. What works for one problem might fail for a slightly modified version.
- Difficulty in Proving Correctness: It can be challenging to prove that a greedy algorithm always produces the optimal solution for a given problem.
- Lack of Foresight: By making locally optimal choices without considering the bigger picture, greedy algorithms can sometimes lead to suboptimal overall solutions.
When Not to Use Greedy Algorithms
Understanding when not to use greedy algorithms is just as important as knowing when to use them. Here are some scenarios where greedy approaches might not be the best choice:
1. Problems Without the Greedy Choice Property
If a problem doesn’t exhibit the greedy choice property, a greedy approach may lead to suboptimal solutions.
Example: 0/1 Knapsack Problem
The 0/1 Knapsack problem is a classic example where a greedy approach (like selecting items based on value-to-weight ratio) doesn’t always yield the optimal solution.
def greedy_knapsack(values, weights, capacity):
items = sorted(zip(values, weights), key=lambda x: x[0]/x[1], reverse=True)
total_value = 0
for value, weight in items:
if capacity >= weight:
capacity -= weight
total_value += value
else:
break
return total_value
values = [60, 100, 120]
weights = [10, 20, 30]
capacity = 50
print(greedy_knapsack(values, weights, capacity)) # Output: 220
# Note: The optimal solution is actually 230 (items with values 100 and 120)
2. Problems Requiring Global Optimization
When a problem requires considering the entire input set to make optimal decisions, greedy algorithms may fall short.
Example: Traveling Salesman Problem (TSP)
The TSP is notoriously difficult and a greedy approach (always choosing the nearest unvisited city) often produces suboptimal tours.
def greedy_tsp(graph):
unvisited = set(graph.keys())
tour = []
current_city = next(iter(unvisited))
tour.append(current_city)
unvisited.remove(current_city)
while unvisited:
next_city = min(unvisited, key=lambda city: graph[current_city][city])
tour.append(next_city)
unvisited.remove(next_city)
current_city = next_city
tour.append(tour[0]) # Return to start
return tour
graph = {
'A': {'B': 20, 'C': 42, 'D': 35},
'B': {'A': 20, 'C': 30, 'D': 34},
'C': {'A': 42, 'B': 30, 'D': 12},
'D': {'A': 35, 'B': 34, 'C': 12}
}
print(greedy_tsp(graph)) # Output might be suboptimal
3. Problems with Complex Constraints
When a problem has multiple interrelated constraints, a greedy approach might struggle to satisfy all of them simultaneously.
Example: Job Scheduling with Multiple Resources
Consider scheduling jobs that require multiple resources (e.g., CPU, memory, network). A greedy approach might optimize for one resource but violate constraints on others.
4. Problems Requiring Backtracking
Greedy algorithms make irrevocable decisions. For problems where choices need to be reconsidered, backtracking or dynamic programming might be more suitable.
Example: Subset Sum Problem
Finding a subset of numbers that sum to a target value often requires exploring multiple combinations, which a simple greedy approach can’t handle effectively.
Hybrid Approaches: Combining Greedy with Other Techniques
In some cases, the most effective solution might involve combining greedy algorithms with other techniques:
1. Greedy with Dynamic Programming
Some problems can benefit from a two-phase approach where a greedy algorithm provides an initial solution, which is then refined using dynamic programming.
2. Greedy in Heuristics
For NP-hard problems, greedy algorithms can serve as effective heuristics, providing good (though not necessarily optimal) solutions quickly.
3. Greedy in Approximation Algorithms
Greedy approaches are often used in approximation algorithms, where they can provide solutions with provable bounds on how far they are from the optimal solution.
Conclusion: Mastering the Art of Algorithm Selection
Choosing the right algorithm for a given problem is a crucial skill in computer science and software engineering. While greedy algorithms offer simplicity and efficiency in many scenarios, they’re not a one-size-fits-all solution. The key to mastering algorithm design lies in:
- Understanding the problem’s structure and constraints
- Recognizing the telltale signs that suggest a greedy approach might be effective
- Being aware of the limitations and potential pitfalls of greedy algorithms
- Knowing when to consider alternative approaches or hybrid solutions
By developing this nuanced understanding, you’ll be better equipped to tackle a wide range of algorithmic challenges, whether you’re preparing for technical interviews, solving competitive programming problems, or designing efficient systems in the real world.
Remember, the journey to algorithmic mastery is ongoing. Keep practicing, analyzing different problem types, and exploring the vast landscape of algorithmic paradigms. With time and experience, you’ll develop an intuition for when to reach for the greedy approach in your problem-solving toolkit.