Mastering Merge Interval Problems: Essential Techniques for Coding Interviews
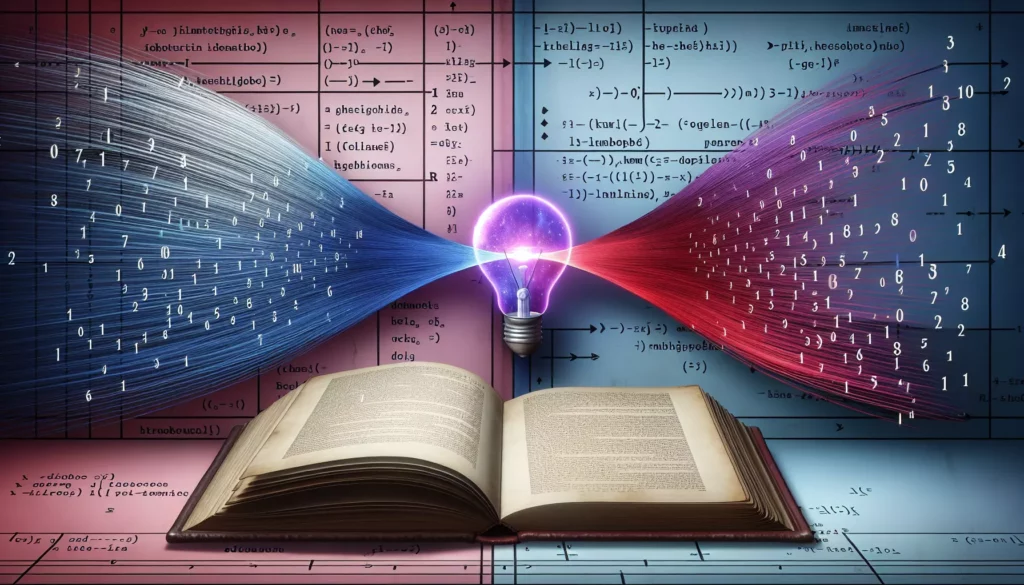
Welcome to AlgoCademy’s comprehensive guide on solving merge interval problems! If you’re preparing for technical interviews at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google) or simply looking to enhance your algorithmic problem-solving skills, you’ve come to the right place. Merge interval problems are a common type of coding challenge that frequently appear in interviews and competitive programming contests. In this article, we’ll dive deep into various techniques and strategies to tackle these problems efficiently.
What are Merge Interval Problems?
Merge interval problems involve working with a set of intervals, each represented by a start and end point. The goal is typically to combine overlapping intervals or perform operations on them. These problems test your ability to think logically, handle edge cases, and implement efficient algorithms.
Here’s a simple example of what an interval might look like:
[1, 3] // An interval from 1 to 3
And here’s a set of intervals:
[[1, 3], [2, 6], [8, 10], [15, 18]]
The challenge often involves merging overlapping intervals, finding intersections, or determining if intervals overlap.
Why are Merge Interval Problems Important?
Merge interval problems are significant for several reasons:
- Real-world applications: These problems have practical applications in various domains, such as scheduling systems, resource allocation, and time management tools.
- Algorithmic thinking: They require you to think critically about data structures and algorithms, enhancing your problem-solving skills.
- Interview frequency: These problems are popular in technical interviews, especially at FAANG companies and other tech giants.
- Complexity analysis: Solving merge interval problems efficiently often involves optimizing time and space complexity, which is a crucial skill for any programmer.
Common Techniques for Solving Merge Interval Problems
Let’s explore some essential techniques that will help you tackle merge interval problems with confidence:
1. Sorting
Sorting is often the first step in solving merge interval problems. By arranging the intervals in a specific order (usually by start time), you can simplify the process of identifying overlaps and merging intervals.
Here’s an example of how you might sort intervals in Python:
intervals = [[1, 3], [8, 10], [2, 6], [15, 18]]
sorted_intervals = sorted(intervals, key=lambda x: x[0])
# Result: [[1, 3], [2, 6], [8, 10], [15, 18]]
Sorting the intervals allows you to compare adjacent intervals easily, which is crucial for many merge interval algorithms.
2. Two-Pointer Technique
The two-pointer technique is handy when working with sorted intervals. You can use one pointer to keep track of the current interval and another to explore potential merges or overlaps.
Here’s a basic implementation of merging overlapping intervals using the two-pointer technique:
def merge_intervals(intervals):
if not intervals:
return []
# Sort intervals by start time
intervals.sort(key=lambda x: x[0])
merged = [intervals[0]]
for current in intervals[1:]:
# Get the last interval in the merged list
last = merged[-1]
# If the current interval overlaps with the last, update the end time
if current[0] <= last[1]:
merged[-1][1] = max(last[1], current[1])
else:
# If not overlapping, add the current interval to the merged list
merged.append(current)
return merged
# Example usage
intervals = [[1, 3], [2, 6], [8, 10], [15, 18]]
print(merge_intervals(intervals))
# Output: [[1, 6], [8, 10], [15, 18]]
This algorithm has a time complexity of O(n log n) due to the sorting step, and a space complexity of O(n) to store the merged intervals.
3. Stack-based Approach
A stack can be an effective data structure for solving certain types of merge interval problems, especially when you need to keep track of the most recent interval and compare it with incoming intervals.
Here’s an example of using a stack to merge overlapping intervals:
def merge_intervals_stack(intervals):
if not intervals:
return []
# Sort intervals by start time
intervals.sort(key=lambda x: x[0])
stack = []
for interval in intervals:
# If the stack is empty or if the current interval doesn't overlap with the stack top,
# simply push the current interval onto the stack
if not stack or interval[0] > stack[-1][1]:
stack.append(interval)
else:
# Otherwise, there is overlap, so merge the current interval with the stack top
stack[-1][1] = max(stack[-1][1], interval[1])
return stack
# Example usage
intervals = [[1, 3], [2, 6], [8, 10], [15, 18]]
print(merge_intervals_stack(intervals))
# Output: [[1, 6], [8, 10], [15, 18]]
The stack-based approach can be particularly useful when you need to handle intervals in a streaming fashion or when the problem involves nested intervals.
4. Line Sweep Algorithm
The line sweep algorithm, also known as the sweep line algorithm, is a powerful technique for solving various geometric problems, including some types of interval problems. The basic idea is to imagine a vertical line sweeping across the plane from left to right, stopping at important points (like start and end points of intervals) to process events.
Here’s an example of using the line sweep algorithm to find the maximum number of overlapping intervals:
from heapq import heappush, heappop
def max_overlapping_intervals(intervals):
# Create events for start and end points
events = []
for start, end in intervals:
events.append((start, 0)) # 0 indicates start event
events.append((end, 1)) # 1 indicates end event
# Sort events by time, with start events before end events if times are equal
events.sort(key=lambda x: (x[0], x[1]))
max_overlaps = 0
current_overlaps = 0
for time, event_type in events:
if event_type == 0: # Start event
current_overlaps += 1
max_overlaps = max(max_overlaps, current_overlaps)
else: # End event
current_overlaps -= 1
return max_overlaps
# Example usage
intervals = [[1, 4], [2, 5], [3, 6], [4, 7]]
print(max_overlapping_intervals(intervals))
# Output: 3
The line sweep algorithm is particularly useful for problems involving finding maximum overlaps, intersection points, or when you need to process intervals in chronological order.
5. Interval Tree
An interval tree is a specialized data structure designed for efficient interval operations, such as finding all intervals that overlap with a given interval. While it’s less common in coding interviews due to its complexity, understanding the concept can be beneficial for certain types of interval problems.
Here’s a basic implementation of an interval tree node in Python:
class IntervalNode:
def __init__(self, interval):
self.interval = interval
self.max = interval[1]
self.left = None
self.right = None
def insert(root, interval):
if not root:
return IntervalNode(interval)
if interval[0] < root.interval[0]:
root.left = insert(root.left, interval)
else:
root.right = insert(root.right, interval)
root.max = max(root.max, interval[1])
return root
def search_overlapping(root, interval):
if not root:
return []
overlapping = []
if do_overlap(root.interval, interval):
overlapping.append(root.interval)
if root.left and root.left.max >= interval[0]:
overlapping.extend(search_overlapping(root.left, interval))
overlapping.extend(search_overlapping(root.right, interval))
return overlapping
def do_overlap(interval1, interval2):
return interval1[0] <= interval2[1] and interval2[0] <= interval1[1]
# Example usage
intervals = [[1, 3], [2, 4], [5, 7], [6, 8]]
root = None
for interval in intervals:
root = insert(root, interval)
search_interval = [2, 6]
overlapping = search_overlapping(root, search_interval)
print(f"Intervals overlapping with {search_interval}: {overlapping}")
# Output: Intervals overlapping with [2, 6]: [[1, 3], [2, 4], [5, 7]]
While interval trees are powerful, they’re often overkill for most interview problems. However, understanding the concept can help you approach complex interval-related problems more effectively.
Common Pitfalls and How to Avoid Them
When solving merge interval problems, be aware of these common pitfalls:
- Forgetting to sort: Many interval problems require sorted intervals as input. Always consider whether sorting is necessary as the first step.
- Incorrect handling of edge cases: Pay attention to cases like empty input, single intervals, or intervals with the same start and end times.
- Overlooking the importance of endpoints: Be clear about whether intervals are inclusive or exclusive at their endpoints. This can affect how you handle overlaps.
- Inefficient comparisons: Avoid unnecessary comparisons by leveraging the sorted nature of the intervals when applicable.
- Mismanaging memory: Be mindful of creating new interval objects unnecessarily, which can lead to increased space complexity.
Practice Problems
To solidify your understanding of merge interval techniques, try solving these practice problems:
- Merge Overlapping Intervals
- Insert Interval
- Non-overlapping Intervals
- Meeting Rooms II (Maximum Number of Overlapping Intervals)
- Interval List Intersections
You can find these problems on popular coding platforms like LeetCode, HackerRank, or right here on AlgoCademy. Remember to approach each problem methodically:
- Understand the problem statement thoroughly
- Identify the appropriate technique(s) to use
- Plan your approach before coding
- Implement your solution
- Test with various input cases, including edge cases
- Analyze the time and space complexity of your solution
- Look for potential optimizations
Advanced Concepts
As you become more comfortable with basic merge interval problems, consider exploring these advanced concepts:
- Segment Trees: A more advanced data structure useful for range queries and updates on intervals.
- Lazy Propagation: An optimization technique used with segment trees for efficient range updates.
- Fenwick Trees (Binary Indexed Trees): Another data structure useful for certain types of range queries, which can be adapted for some interval problems.
- Sweep Line Algorithm Variations: More complex applications of the sweep line algorithm for geometric problems involving intervals.
Conclusion
Mastering merge interval problems is an essential skill for any programmer preparing for technical interviews or looking to improve their algorithmic problem-solving abilities. By understanding and practicing the techniques we’ve covered – sorting, two-pointer approach, stack-based solutions, line sweep algorithm, and interval trees – you’ll be well-equipped to tackle a wide range of interval-related challenges.
Remember, the key to success is consistent practice and a methodical approach to problem-solving. Don’t be discouraged if you find some problems challenging at first; with time and practice, you’ll develop the intuition to recognize patterns and apply the appropriate techniques efficiently.
Keep coding, keep learning, and don’t hesitate to use AlgoCademy’s resources, including our AI-powered assistance and step-by-step guidance, to help you on your journey to becoming a top-tier programmer. Happy coding!