When to Use a Frequency Counter Pattern: A Comprehensive Guide
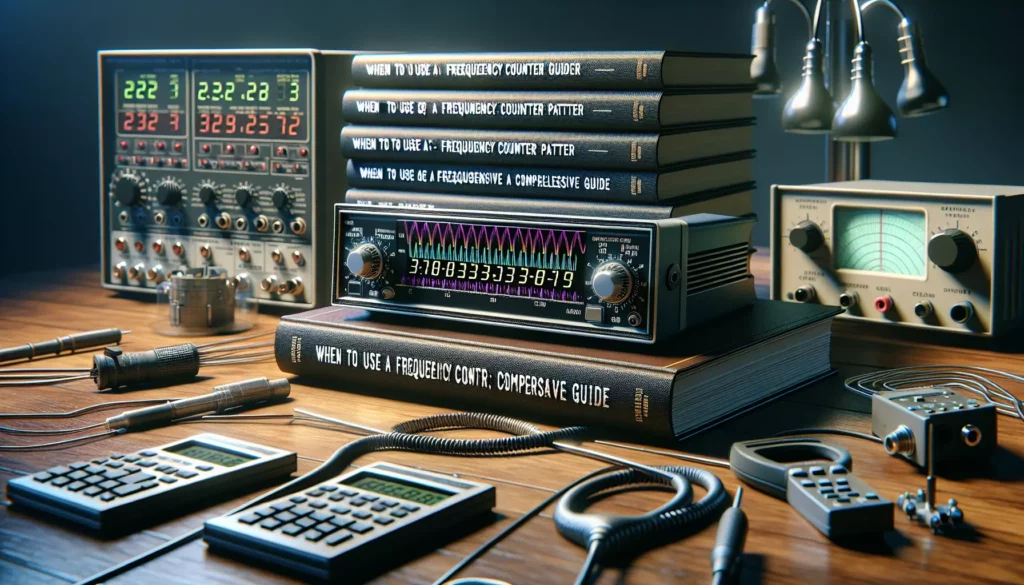
In the world of coding and algorithm design, efficiency is key. As developers, we’re constantly seeking ways to optimize our code and solve problems more effectively. One powerful technique that often comes in handy is the Frequency Counter pattern. But when should you use it, and how can it improve your coding skills? In this comprehensive guide, we’ll explore the ins and outs of the Frequency Counter pattern, its applications, and how it can help you ace those technical interviews at major tech companies.
What is the Frequency Counter Pattern?
Before we dive into when to use the Frequency Counter pattern, let’s first understand what it is. The Frequency Counter pattern is a technique used to compare the frequency of elements in multiple collections (such as arrays or strings) without the need for nested loops. It typically involves using objects or sets to collect frequencies of values, which can then be compared or analyzed.
The main advantage of this pattern is that it can often reduce the time complexity of a solution from O(n^2) to O(n), making it significantly more efficient for larger inputs.
When Should You Use the Frequency Counter Pattern?
Now that we understand what the Frequency Counter pattern is, let’s explore the scenarios where it’s most useful:
1. Comparing Two Collections
One of the most common use cases for the Frequency Counter pattern is when you need to compare two collections (arrays or strings) to determine if they have the same frequency of elements. This could include problems like:
- Checking if two strings are anagrams
- Determining if one array is a subset of another
- Verifying if two arrays have the same elements, regardless of order
Example problem: Write a function to determine if two strings are anagrams.
function areAnagrams(str1, str2) {
if (str1.length !== str2.length) return false;
const frequencyCounter1 = {};
const frequencyCounter2 = {};
for (let char of str1) {
frequencyCounter1[char] = (frequencyCounter1[char] || 0) + 1;
}
for (let char of str2) {
frequencyCounter2[char] = (frequencyCounter2[char] || 0) + 1;
}
for (let key in frequencyCounter1) {
if (frequencyCounter1[key] !== frequencyCounter2[key]) {
return false;
}
}
return true;
}
console.log(areAnagrams("listen", "silent")); // true
console.log(areAnagrams("hello", "world")); // false
2. Counting Occurrences
The Frequency Counter pattern is excellent for problems that require counting the occurrences of elements in a collection. This can be useful for:
- Finding the most frequent element in an array
- Counting the number of unique elements
- Identifying elements that appear more than a certain number of times
Example problem: Find the most frequent element in an array.
function mostFrequentElement(arr) {
const frequencyCounter = {};
let maxFrequency = 0;
let mostFrequent;
for (let elem of arr) {
frequencyCounter[elem] = (frequencyCounter[elem] || 0) + 1;
if (frequencyCounter[elem] > maxFrequency) {
maxFrequency = frequencyCounter[elem];
mostFrequent = elem;
}
}
return mostFrequent;
}
console.log(mostFrequentElement([1, 2, 3, 2, 1, 3, 2])); // 2
3. Validating Sequences or Patterns
The Frequency Counter pattern can be useful when you need to validate sequences or patterns within a collection. This might include:
- Checking if a string contains all the characters from another string
- Verifying if an array contains a specific sequence of numbers
- Determining if a collection satisfies certain frequency-based criteria
Example problem: Write a function to check if a string contains all the characters from another string.
function containsAllChars(str, chars) {
const frequencyCounter = {};
for (let char of chars) {
frequencyCounter[char] = (frequencyCounter[char] || 0) + 1;
}
for (let char of str) {
if (frequencyCounter[char] > 0) {
frequencyCounter[char]--;
}
}
return Object.values(frequencyCounter).every(count => count === 0);
}
console.log(containsAllChars("hello world", "ol")); // true
console.log(containsAllChars("hello world", "xyz")); // false
4. Optimizing Time Complexity
In general, you should consider using the Frequency Counter pattern when you encounter a problem that would typically require nested loops to solve. By using this pattern, you can often reduce the time complexity from O(n^2) to O(n), making your solution much more efficient for larger inputs.
For example, consider a problem where you need to find if there’s a pair of numbers in an array that sum up to a target value. A naive approach might use nested loops:
function hasPairWithSum(arr, target) {
for (let i = 0; i < arr.length; i++) {
for (let j = i + 1; j < arr.length; j++) {
if (arr[i] + arr[j] === target) {
return true;
}
}
}
return false;
}
// Time complexity: O(n^2)
Using the Frequency Counter pattern, we can optimize this to O(n):
function hasPairWithSum(arr, target) {
const seen = new Set();
for (let num of arr) {
if (seen.has(target - num)) {
return true;
}
seen.add(num);
}
return false;
}
// Time complexity: O(n)
How to Implement the Frequency Counter Pattern
Now that we know when to use the Frequency Counter pattern, let’s discuss how to implement it effectively:
- Choose the right data structure: In most cases, you’ll use an object or a Map to store the frequencies. Use an object when your keys are strings or can be easily converted to strings. Use a Map when you need to use non-string keys or want to maintain insertion order.
- Initialize the frequency counter: Create an empty object or Map to store your frequencies.
- Iterate through the collection: Loop through your array or string, updating the frequency counter for each element.
- Analyze or compare the frequencies: Depending on your problem, you might need to loop through the frequency counter to analyze the results or compare it with another frequency counter.
Common Pitfalls and How to Avoid Them
While the Frequency Counter pattern is powerful, there are some common mistakes to watch out for:
1. Forgetting to Handle Edge Cases
Always consider edge cases, such as empty inputs or inputs of different lengths. For example, in our anagram function, we check if the lengths are equal before proceeding:
if (str1.length !== str2.length) return false;
2. Using the Wrong Data Type for Keys
Remember that object keys in JavaScript are always converted to strings. If you need to use numbers or other data types as keys, consider using a Map instead:
const frequencyCounter = new Map();
frequencyCounter.set(42, 1); // Using a number as a key
3. Not Resetting the Counter Between Multiple Uses
If you’re using the same frequency counter for multiple operations, make sure to reset it between uses:
function resetCounter(counter) {
for (let key in counter) {
delete counter[key];
}
}
// Or if using a Map:
frequencyCounter.clear();
4. Overcomplicating Simple Problems
While the Frequency Counter pattern is powerful, it’s not always necessary. For very small inputs or simple problems, a more straightforward approach might be clearer and just as efficient.
Real-world Applications and Interview Questions
Understanding when and how to use the Frequency Counter pattern can be incredibly valuable in real-world applications and technical interviews. Here are some examples of where this pattern might come in handy:
1. Data Analysis and Processing
In data-heavy applications, you might need to analyze large datasets to find patterns or anomalies. The Frequency Counter pattern can be useful for tasks like:
- Identifying the most common words in a text corpus
- Finding duplicate entries in a database
- Analyzing user behavior patterns in web analytics
2. Cryptography and Security
The Frequency Counter pattern can be applied in various cryptography and security-related tasks, such as:
- Analyzing frequency distributions in encrypted messages
- Detecting potential security threats based on unusual patterns of behavior
- Implementing simple encryption/decryption algorithms
3. Common Interview Questions
Many technical interview questions, especially those from major tech companies, can be solved efficiently using the Frequency Counter pattern. Here are a few examples:
Question 1: Valid Anagram
Given two strings s and t, return true if t is an anagram of s, and false otherwise.
function isAnagram(s, t) {
if (s.length !== t.length) return false;
const frequencyCounter = {};
for (let char of s) {
frequencyCounter[char] = (frequencyCounter[char] || 0) + 1;
}
for (let char of t) {
if (!frequencyCounter[char]) return false;
frequencyCounter[char]--;
}
return true;
}
console.log(isAnagram("anagram", "nagaram")); // true
console.log(isAnagram("rat", "car")); // false
Question 2: First Unique Character in a String
Given a string s, find the first non-repeating character in it and return its index. If it does not exist, return -1.
function firstUniqChar(s) {
const frequencyCounter = {};
for (let char of s) {
frequencyCounter[char] = (frequencyCounter[char] || 0) + 1;
}
for (let i = 0; i < s.length; i++) {
if (frequencyCounter[s[i]] === 1) {
return i;
}
}
return -1;
}
console.log(firstUniqChar("leetcode")); // 0
console.log(firstUniqChar("loveleetcode")); // 2
Question 3: Subarray Sum Equals K
Given an array of integers nums and an integer k, return the total number of continuous subarrays whose sum equals to k.
function subarraySum(nums, k) {
const frequencyCounter = { 0: 1 };
let count = 0;
let sum = 0;
for (let num of nums) {
sum += num;
if (frequencyCounter[sum - k]) {
count += frequencyCounter[sum - k];
}
frequencyCounter[sum] = (frequencyCounter[sum] || 0) + 1;
}
return count;
}
console.log(subarraySum([1, 1, 1], 2)); // 2
console.log(subarraySum([1, 2, 3], 3)); // 2
Conclusion
The Frequency Counter pattern is a powerful tool in a developer’s arsenal, particularly useful for optimizing solutions to problems involving collections of data. By understanding when and how to apply this pattern, you can write more efficient code and tackle a wide range of programming challenges.
As you continue to develop your coding skills and prepare for technical interviews, practice implementing the Frequency Counter pattern in various scenarios. Remember, the key to mastering any programming technique is consistent practice and application to diverse problems.
Whether you’re working on a personal project, solving coding challenges on platforms like AlgoCademy, or preparing for interviews with major tech companies, the Frequency Counter pattern can help you write cleaner, more efficient code. Keep exploring, keep practicing, and keep pushing the boundaries of your programming knowledge!