Strategies for Solving Tree Traversal Problems: A Comprehensive Guide
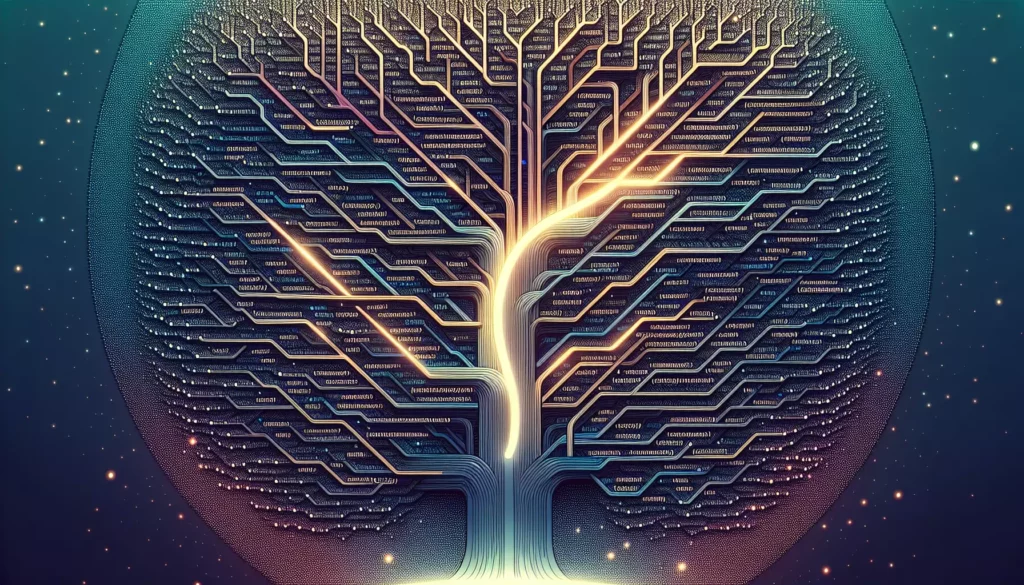
Tree traversal is a fundamental concept in computer science and a common topic in coding interviews, especially for positions at major tech companies. Understanding and mastering tree traversal techniques is crucial for any programmer looking to excel in their career. In this comprehensive guide, we’ll explore various strategies for solving tree traversal problems, providing you with the knowledge and skills needed to tackle these challenges confidently.
Table of Contents
- Introduction to Tree Traversal
- Types of Tree Traversal
- Recursive Approaches
- Iterative Approaches
- Advanced Traversal Techniques
- Common Tree Traversal Problems and Solutions
- Optimization Strategies
- Practice and Resources
- Conclusion
1. Introduction to Tree Traversal
Tree traversal is the process of visiting each node in a tree data structure exactly once. It’s a fundamental operation in computer science and is essential for various applications, including:
- Searching for a specific node
- Printing or processing all nodes in a specific order
- Copying or comparing tree structures
- Evaluating expressions represented by trees
Before diving into specific traversal techniques, it’s important to understand the basic structure of a tree:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
This simple structure represents a binary tree node, but the concepts we’ll discuss can be applied to various types of trees.
2. Types of Tree Traversal
There are three main types of depth-first tree traversal:
- Inorder Traversal: Left subtree, Root, Right subtree
- Preorder Traversal: Root, Left subtree, Right subtree
- Postorder Traversal: Left subtree, Right subtree, Root
Additionally, there’s breadth-first traversal:
- Level Order Traversal: Visit nodes level by level, from left to right
Each of these traversal methods has its own use cases and advantages. Let’s explore how to implement them using both recursive and iterative approaches.
3. Recursive Approaches
Recursive approaches to tree traversal are often the most intuitive and easiest to implement. Let’s look at how to implement each type of traversal recursively.
Inorder Traversal (Recursive)
def inorder_traversal(root):
result = []
def inorder(node):
if node:
inorder(node.left)
result.append(node.val)
inorder(node.right)
inorder(root)
return result
Preorder Traversal (Recursive)
def preorder_traversal(root):
result = []
def preorder(node):
if node:
result.append(node.val)
preorder(node.left)
preorder(node.right)
preorder(root)
return result
Postorder Traversal (Recursive)
def postorder_traversal(root):
result = []
def postorder(node):
if node:
postorder(node.left)
postorder(node.right)
result.append(node.val)
postorder(root)
return result
The recursive approach is elegant and easy to understand, but it can lead to stack overflow errors for very deep trees. That’s where iterative approaches come in handy.
4. Iterative Approaches
Iterative approaches use explicit stacks or queues to traverse the tree, avoiding the potential stack overflow issues of recursive methods. Here’s how to implement each traversal type iteratively:
Inorder Traversal (Iterative)
def inorder_traversal_iterative(root):
result = []
stack = []
current = root
while current or stack:
while current:
stack.append(current)
current = current.left
current = stack.pop()
result.append(current.val)
current = current.right
return result
Preorder Traversal (Iterative)
def preorder_traversal_iterative(root):
if not root:
return []
result = []
stack = [root]
while stack:
node = stack.pop()
result.append(node.val)
if node.right:
stack.append(node.right)
if node.left:
stack.append(node.left)
return result
Postorder Traversal (Iterative)
def postorder_traversal_iterative(root):
if not root:
return []
result = []
stack = [root]
visited = set()
while stack:
node = stack[-1]
if (not node.left and not node.right) or (node in visited):
result.append(node.val)
stack.pop()
else:
visited.add(node)
if node.right:
stack.append(node.right)
if node.left:
stack.append(node.left)
return result
Level Order Traversal (Iterative)
from collections import deque
def level_order_traversal(root):
if not root:
return []
result = []
queue = deque([root])
while queue:
level_size = len(queue)
level = []
for _ in range(level_size):
node = queue.popleft()
level.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
result.append(level)
return result
Iterative approaches are generally more efficient in terms of space complexity, especially for large trees, as they don’t rely on the call stack.
5. Advanced Traversal Techniques
Beyond the basic traversal methods, there are several advanced techniques that can be useful in specific scenarios:
Morris Traversal
Morris Traversal is a technique that allows for inorder traversal using constant extra space. It temporarily modifies the tree structure to traverse without using a stack or recursion.
def morris_inorder_traversal(root):
result = []
current = root
while current:
if not current.left:
result.append(current.val)
current = current.right
else:
predecessor = current.left
while predecessor.right and predecessor.right != current:
predecessor = predecessor.right
if not predecessor.right:
predecessor.right = current
current = current.left
else:
predecessor.right = None
result.append(current.val)
current = current.right
return result
Threaded Binary Trees
Threaded binary trees use the null pointers in leaf nodes to create “threads” that link to the next node in a specific traversal order. This can speed up traversals and eliminate the need for a stack or recursion.
Zigzag Level Order Traversal
This is a variation of level order traversal where we alternate the direction of traversal for each level.
from collections import deque
def zigzag_level_order(root):
if not root:
return []
result = []
queue = deque([root])
left_to_right = True
while queue:
level_size = len(queue)
level = deque()
for _ in range(level_size):
node = queue.popleft()
if left_to_right:
level.append(node.val)
else:
level.appendleft(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
result.append(list(level))
left_to_right = not left_to_right
return result
6. Common Tree Traversal Problems and Solutions
Let’s explore some common tree traversal problems you might encounter in coding interviews and how to solve them:
Problem 1: Validate Binary Search Tree
Determine if a binary tree is a valid binary search tree (BST).
def is_valid_bst(root):
def validate(node, low=float('-inf'), high=float('inf')):
if not node:
return True
if node.val = high:
return False
return (validate(node.left, low, node.val) and
validate(node.right, node.val, high))
return validate(root)
Problem 2: Serialize and Deserialize Binary Tree
Design an algorithm to serialize and deserialize a binary tree.
class Codec:
def serialize(self, root):
if not root:
return 'null'
return f"{root.val},{self.serialize(root.left)},{self.serialize(root.right)}"
def deserialize(self, data):
def dfs():
val = next(values)
if val == 'null':
return None
node = TreeNode(int(val))
node.left = dfs()
node.right = dfs()
return node
values = iter(data.split(','))
return dfs()
Problem 3: Lowest Common Ancestor of a Binary Tree
Find the lowest common ancestor (LCA) of two given nodes in a binary tree.
def lowest_common_ancestor(root, p, q):
if not root or root == p or root == q:
return root
left = lowest_common_ancestor(root.left, p, q)
right = lowest_common_ancestor(root.right, p, q)
if left and right:
return root
return left if left else right
7. Optimization Strategies
When working with tree traversal problems, consider these optimization strategies:
- Choose the right traversal method: Different problems may be more efficiently solved with specific traversal methods. For example, inorder traversal is often used for BST-related problems.
- Use iterative solutions for large trees: To avoid stack overflow errors, use iterative solutions for very deep trees.
- Prune unnecessary branches: In some problems, you can stop traversing certain branches early if they don’t meet specific conditions.
- Use helper functions: Break down complex logic into helper functions to improve readability and maintainability.
- Consider custom tree structures: For specific problems, modifying the tree structure (e.g., adding parent pointers) can simplify traversal.
8. Practice and Resources
To master tree traversal problems, consistent practice is key. Here are some resources to help you improve:
- LeetCode: Offers a wide range of tree-related problems with varying difficulty levels.
- HackerRank: Provides a Data Structures track with tree-related challenges.
- GeeksforGeeks: Offers comprehensive articles and practice problems on tree traversal.
- AlgoExpert: Provides curated list of tree problems often asked in coding interviews.
- Books: “Cracking the Coding Interview” and “Elements of Programming Interviews” both contain sections on trees and traversal.
Remember to not just solve problems, but also to analyze different approaches, time and space complexities, and edge cases for each problem you encounter.
9. Conclusion
Tree traversal is a fundamental skill for any programmer, especially those preparing for technical interviews at top tech companies. By mastering the various traversal techniques – inorder, preorder, postorder, and level order – and understanding both recursive and iterative approaches, you’ll be well-equipped to tackle a wide range of tree-related problems.
Remember that the key to success is not just knowing the algorithms, but also understanding when and how to apply them. As you practice, focus on:
- Identifying the most appropriate traversal method for each problem
- Analyzing the time and space complexity of your solutions
- Considering edge cases and potential optimizations
- Clearly explaining your thought process and approach
With dedication and consistent practice, you’ll develop the skills and confidence needed to excel in tree traversal problems during coding interviews and in your programming career. Keep coding, keep learning, and remember that every problem you solve brings you one step closer to mastery!