How to Handle Getting Stuck Halfway Through Your Solution
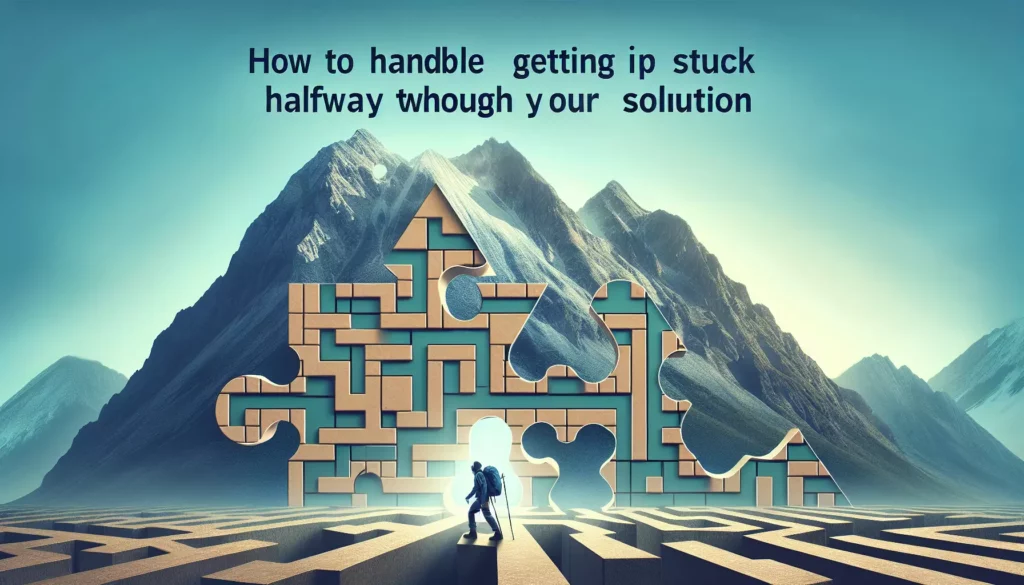
As a programmer, getting stuck halfway through a solution is a common and often frustrating experience. Whether you’re a beginner tackling your first coding challenge or an experienced developer working on a complex project, hitting a roadblock can be disheartening. However, it’s essential to remember that getting stuck is a natural part of the problem-solving process and an opportunity for growth. In this comprehensive guide, we’ll explore effective strategies to overcome these obstacles and continue making progress in your coding journey.
Understanding Why You Get Stuck
Before diving into solutions, it’s crucial to understand why programmers often get stuck midway through solving a problem. Some common reasons include:
- Lack of a clear problem-solving strategy
- Insufficient understanding of the problem requirements
- Gaps in knowledge of programming concepts or language features
- Overwhelm from tackling too much complexity at once
- Fatigue or burnout from extended coding sessions
- Perfectionism leading to analysis paralysis
Recognizing these factors can help you identify the root cause of your struggle and apply the most appropriate strategies to overcome it.
Strategies for Overcoming Coding Roadblocks
1. Take a Step Back and Reassess
When you find yourself stuck, the first step is to take a moment to step back from your code and reassess the situation. This pause allows you to gain a fresh perspective and avoid spiraling into frustration.
- Review the problem statement and requirements
- Examine your current approach and identify where you’re stuck
- Consider if there are alternative ways to tackle the problem
2. Break Down the Problem Further
If you’re feeling overwhelmed by the complexity of your current task, try breaking it down into smaller, more manageable sub-problems. This divide-and-conquer approach can help you make progress and build momentum.
// Example: Breaking down a sorting problem
function sortArray(arr) {
// 1. Divide the array into two halves
// 2. Recursively sort each half
// 3. Merge the sorted halves
}
function mergeSort(arr) {
if (arr.length <= 1) return arr;
const mid = Math.floor(arr.length / 2);
const left = mergeSort(arr.slice(0, mid));
const right = mergeSort(arr.slice(mid));
return merge(left, right);
}
function merge(left, right) {
// Implement merging logic here
}
3. Use Pseudocode or Flowcharts
Sometimes, the act of writing code directly can be limiting. Instead, try expressing your solution in pseudocode or creating a flowchart. This approach allows you to focus on the logic and structure of your solution without getting bogged down in syntax details.
// Pseudocode example for a simple search algorithm
function search(array, target):
for each element in array:
if element equals target:
return index of element
return -1 // Target not found
4. Implement a Simpler Version First
If you’re struggling with a complex implementation, try simplifying the problem and solving a basic version first. This approach can help you understand the core logic and gradually build up to the full solution.
// Simple version of a function to find the maximum number in an array
function findMax(arr) {
let max = arr[0];
for (let i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
// Later, you can optimize or extend this function for more complex scenarios
5. Use Debugging Techniques
Effective debugging can help you identify where your code is going wrong and provide insights into how to fix it. Some useful debugging techniques include:
- Using console.log() or print statements to track variable values
- Setting breakpoints and stepping through your code
- Using a debugger tool in your IDE
- Writing unit tests to verify individual components of your solution
function debugExample(n) {
console.log("Input:", n);
let result = 0;
for (let i = 1; i <= n; i++) {
console.log("Current i:", i);
result += i;
console.log("Current result:", result);
}
console.log("Final result:", result);
return result;
}
debugExample(5);
6. Rubber Duck Debugging
Explaining your code and thought process to someone else (or even an inanimate object like a rubber duck) can help you identify logical errors or overlooked details. This technique, known as rubber duck debugging, forces you to articulate your approach step-by-step, often revealing the source of your problem.
7. Take a Break and Return with Fresh Eyes
Sometimes, the best solution is to step away from your code for a while. Taking a short break, going for a walk, or even sleeping on the problem can help you return with a fresh perspective and renewed energy.
8. Research and Learn
If you’re stuck due to a lack of knowledge or understanding, it’s time to do some research. Look up relevant documentation, tutorials, or examples that can help fill in the gaps in your understanding.
- Official documentation for the programming language or framework you’re using
- Online coding platforms like AlgoCademy for interactive tutorials and exercises
- Stack Overflow for specific coding questions and solutions
- YouTube tutorials or coding channels for visual explanations
9. Use Version Control to Experiment
If you’re hesitant to make significant changes to your code for fear of breaking what already works, use version control systems like Git to create a new branch. This allows you to experiment freely without risking your main codebase.
// Git commands to create and switch to a new branch
git branch experimental-solution
git checkout experimental-solution
// After experimenting, if you want to keep the changes
git checkout main
git merge experimental-solution
// If you want to discard the experimental changes
git branch -D experimental-solution
10. Seek Help from the Coding Community
Don’t hesitate to reach out to the coding community for help. Online forums, coding communities, and platforms like Stack Overflow can provide valuable insights and solutions to your problem.
- Be specific about your problem and what you’ve tried so far
- Share relevant code snippets or a minimal reproducible example
- Be open to feedback and alternative approaches
Developing a Growth Mindset
Getting stuck is not a reflection of your abilities as a programmer but an opportunity for growth. Embrace these moments as learning experiences and chances to improve your problem-solving skills.
- Celebrate small victories and incremental progress
- Reflect on what you’ve learned from each challenging situation
- Keep a log of problems you’ve overcome and solutions you’ve discovered
Preventing Future Roadblocks
While getting stuck is sometimes inevitable, there are steps you can take to minimize the frequency and impact of these situations:
1. Plan Before You Code
Spend time planning your approach before diving into coding. Create an outline or flowchart of your solution to identify potential challenges early.
2. Practice Regularly
Consistent practice helps build your problem-solving skills and familiarity with common coding patterns. Platforms like AlgoCademy offer a structured approach to improving your algorithmic thinking and coding skills.
3. Learn from Others
Study other people’s code and solutions to similar problems. This can expose you to different approaches and techniques you might not have considered.
4. Build a Strong Foundation
Invest time in understanding fundamental concepts and data structures. A solid foundation makes it easier to tackle more complex problems.
5. Stay Updated
Keep up with the latest developments in your programming language and tools. New features or libraries might offer simpler solutions to problems you’re facing.
Conclusion
Getting stuck halfway through a solution is a common experience for programmers of all levels. By applying the strategies outlined in this guide, you can overcome these obstacles and continue making progress in your coding projects. Remember that each challenge you face is an opportunity to learn and grow as a developer.
Platforms like AlgoCademy offer valuable resources and interactive tools to help you develop your problem-solving skills and prepare for technical interviews. By leveraging these resources and maintaining a growth mindset, you can turn moments of frustration into stepping stones towards becoming a more skilled and resilient programmer.
Keep coding, stay curious, and remember that every great programmer has faced similar challenges on their journey. Your ability to persevere and overcome obstacles is what will set you apart in your coding career.