Understanding the Basics: An Example of Coding for Beginners
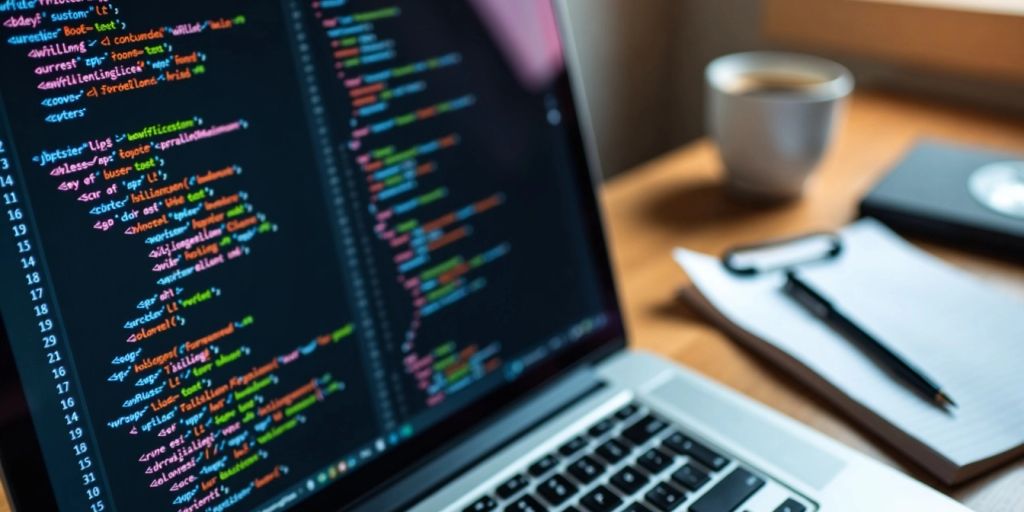
In this article, we will explore the basics of coding and how it can open doors to exciting careers. Whether you’re interested in building websites, apps, or analyzing data, understanding coding is essential. We’ll break down the fundamental concepts, tools, and skills you need to get started on your coding journey.
Key Takeaways
- Coding is a way to communicate with computers, telling them what to do.
- Learning the basics of coding is crucial for understanding more complex programming languages.
- Variables are like containers for data, making it easy to use and refer to information in your code.
- Control structures help your code make decisions based on different conditions.
- Writing clean and organized code is important for collaboration and future reference.
What Is Coding?
Definition of Coding
Coding is the process of writing instructions for computers. It allows us to communicate with machines and tell them what to do. These instructions are called programs, and they can be written in various programming languages like Python, Java, and JavaScript. Each language has its own rules, but they all serve the same purpose: to make computers perform tasks.
Importance of Coding
Learning to code is essential in today’s digital world. Here are some reasons why:
- Career Opportunities: Many jobs require coding skills.
- Problem-Solving Skills: Coding helps develop critical thinking and creativity.
- Empowerment: It allows you to create your own projects, from websites to apps.
How Coding Works
When you write code, it needs to be translated into a language that computers understand, known as machine code. This is done using compilers or interpreters. Here’s a simple breakdown of the process:
- Write the code in a programming language.
- Use a compiler or interpreter to convert it into machine code.
- The computer executes the machine code to perform the desired action.
Coding is not just about writing instructions; it’s about bringing your ideas to life. By learning coding basics, you can unlock a world of possibilities!
Getting Started With Coding
Choosing a Programming Language
When you’re ready to dive into coding, selecting the right programming language is crucial. Here are some popular options:
- HTML/CSS: Great for web development.
- JavaScript: Adds interactivity to websites.
- Python: Known for its simplicity and versatility.
- Java: Widely used in enterprise applications.
Setting Up Your Development Environment
To start coding, you need a proper setup. Here’s how:
- Install a Code Editor: Tools like VS Code or Sublime Text are user-friendly.
- Get Familiar with the Console: Learn basic commands to navigate your system.
- Set Up Version Control: Use Git to manage your code changes.
Basic Coding Tools
Having the right tools can make learning easier. Here are some essentials:
- Code Editors: For writing and editing code.
- Debugging Tools: Help find and fix errors in your code.
- Online Resources: Websites like Codecademy and freeCodeCamp offer tutorials.
Remember, starting with small, specific goals can help you stay motivated. For instance, if you want to build a simple website, focus on HTML and CSS first. This approach will help you build the skills you need and give you a sense of accomplishment.
By understanding these basics, you’ll be well on your way to becoming a proficient coder!
Understanding Variables
What Are Variables?
In programming, variables are like containers that hold data. You can think of a variable as a box that stores a value. For example, in JavaScript, you can use variables to store numbers, strings, objects, and other types of data. This makes it easier to manage and use data in your programs.
Declaring Variables
To create a variable, you need to declare it. This means giving it a name and assigning it a value. For instance:
let myAge = 15;
In this example, myAge
is the variable name, and it holds the value 15
. You can change the value later if needed.
Using Variables in Code
Once you have declared a variable, you can use it throughout your code. Here are some examples of how to use variables:
- Storing Information: You can store user input or data from a database.
- Performing Calculations: You can use variables in math operations, like adding or subtracting.
- Displaying Data: You can show the value of a variable on the screen.
Variable Name | Value |
---|---|
myAge | 15 |
myName | "Zoe" |
Variables are essential in programming because they help you manage and manipulate data easily. They allow you to write flexible and dynamic code that can change based on user input or other factors.
Control Structures in Coding
Control structures are essential in programming as they help your code make decisions. They act like a bouncer at a bar, deciding who gets in based on certain rules. For example, if someone is under 21, they won’t be allowed in. Similarly, control structures allow your program to execute different commands based on specific conditions.
Introduction to Control Structures
Control structures are the rules that guide how your program runs. They help in making choices and executing commands based on those choices. Without them, your code would just run in a straight line without any decision-making.
Types of Control Structures
There are several types of control structures, including:
- Conditional Statements: These allow your program to make decisions. The most common conditional statements in C are the if statement, the else statement, and the switch statement.
- Loops: These let your program repeat actions until a certain condition is met. Common loops include for loops and while loops.
Examples of Control Structures
Here’s a simple example of an if statement in Python:
age = 20
if age >= 21:
print("You can enter the bar.")
else:
print("Sorry, you cannot enter.")
In this example, the program checks the age and decides whether to allow entry based on the condition.
Control structures are vital for creating dynamic and responsive programs. They allow your code to adapt to different situations, making it more powerful and flexible.
Control structures are the backbone of decision-making in programming. They help your code respond to various conditions effectively.
Functions and Their Uses
What Are Functions?
Functions are like little machines in your code. They take some input, do something with it, and then give you an output. Functions in programming are modular units of code designed to perform specific tasks. They help keep your code organized and make it easier to read.
Creating Functions
To create a function, you need to give it a name and tell it what to do. Here’s a simple way to think about it:
- Name your function: This is how you will call it later.
- Define what it does: Write the steps inside the function.
- Call the function: Use its name whenever you need it.
Using Functions in Programs
Using functions can make your coding life easier. Here are some benefits:
- Reusability: You can use the same function multiple times without rewriting the code.
- Organization: Functions help break your code into smaller, manageable pieces.
- Testing: It’s easier to test a small function than a big program.
Benefit | Description |
---|---|
Reusability | Use the same code without rewriting it. |
Organization | Keep your code neat and tidy. |
Testing | Test small parts of your code easily. |
Functions are essential for writing clean and efficient code. They allow you to focus on bigger problems without getting lost in details.
Data Types and Their Importance
Common Data Types
In programming, data types are essential because they help computers understand different kinds of information. Here are some common data types:
- Numeric: Represents numbers, like 5 or 3.14.
- String: A sequence of characters, like "Hello!".
- Boolean: Represents true or false values.
Choosing the Right Data Type
Choosing the right data type is crucial for your program to work correctly. Here are some tips:
- Understand the data: Know what kind of information you are working with.
- Use numeric types for calculations: If you need to do math, use numeric data types.
- Use strings for text: If you are working with words or sentences, use strings.
- Use booleans for conditions: When you need to check if something is true or false, use booleans.
Manipulating Data Types
Manipulating data types means changing or using them in your code. Here are some common operations:
- Concatenation: Joining strings together, like "Hello" + " World" = "Hello World".
- Arithmetic operations: Adding or subtracting numbers, like 5 + 3 = 8.
- Logical operations: Using booleans to make decisions, like checking if a condition is true.
Data types are the building blocks of programming. They help you organize and manage information effectively.
Summary
Understanding data types is vital for coding. They help you define how information is stored and used in your programs. By knowing the different types and how to use them, you can write better code and avoid errors. For more details, you can discover the 10 data types (with definitions and examples) to explore how different data type examples may look and function within programming.
Writing Clean Code
Importance of Clean Code
Writing clean code is essential for any programmer. Clean code is easier to read and understand, which helps both you and others who may work with your code later. When you write code that is clear, it saves time and reduces errors. Here are some reasons why clean code matters:
- Readability: Others can easily understand your code.
- Maintainability: It’s easier to fix bugs or add features.
- Collaboration: Team members can work together more effectively.
Tips for Writing Clean Code
Here are some tips to help you write cleaner code:
- Use meaningful names: Choose names for your variables and functions that describe their purpose.
- Keep functions small: Each function should do one thing and do it well.
- Limit line length: Try to keep your lines of code under 80 characters.
- Comment your code: Use comments to explain complex parts of your code.
- Consistent formatting: Stick to a style guide for indentation and spacing.
Examples of Clean Code
Here’s a simple example of clean code:
# This function adds two numbers
def add_numbers(a, b):
return a + b
In this example, the function name clearly states what it does, and the comment explains its purpose.
Writing clean code is a habit that will benefit you throughout your programming journey. It’s better to start now than to fix messy code later.
Conclusion
By following these guidelines, you can develop the habit of writing clean code. This will not only help you but also make it easier for others to work with your code in the future. Remember, clean code is a sign of a good programmer!
Debugging Your Code
Debugging is a crucial part of coding. It involves finding and fixing errors in your code. Learning how to debug your code can greatly enhance your coding skills. Here are some key points to help you understand debugging better:
Common Coding Errors
When coding, you might encounter several types of errors:
- Syntax Errors: Mistakes in the code that prevent it from running.
- Runtime Errors: Errors that occur while the program is running.
- Logic Errors: The code runs, but it doesn’t produce the expected results.
Debugging Techniques
Here are some effective techniques to debug your code:
- Read Your Code: Go through your code line by line to spot mistakes.
- Use Print Statements: Add print statements to check the values of variables at different points.
- Rubber Duck Debugging: Explain your code to an inanimate object. This can help clarify your thoughts.
Tools for Debugging
Using the right tools can make debugging easier. Here are some popular debugging tools:
Tool Name | Description |
---|---|
Debugger | A built-in tool in many IDEs that allows you to step through your code. |
Linting Tools | These tools check your code for errors before you run it. |
Error Logs | Logs that show errors that occurred during execution. |
Debugging is not just about fixing errors; it’s about understanding your code better.
By mastering debugging, you can improve your coding skills and create better programs. Remember, every coder faces challenges, but with practice, you can overcome them!
Advanced Coding Concepts for Beginners
Introduction to Advanced Concepts
As you dive deeper into coding, you’ll encounter advanced concepts that can enhance your programming skills. Understanding these ideas is crucial for building more complex applications and solving intricate problems.
Object-Oriented Programming
Object-Oriented Programming (OOP) is a programming style that uses objects to represent data and methods. Here are some key points about OOP:
- Encapsulation: Bundling data and methods that operate on that data within one unit, or class.
- Inheritance: Creating new classes based on existing ones, allowing for code reuse.
- Polymorphism: Using a single interface to represent different underlying forms (data types).
Basic Algorithms
Algorithms are step-by-step procedures for solving problems. Here are a few basic types:
- Sorting Algorithms: Organizing data in a specific order (e.g., bubble sort, quick sort).
- Search Algorithms: Finding specific data within a structure (e.g., linear search, binary search).
- Recursive Algorithms: Solving problems by breaking them down into smaller sub-problems.
Understanding these algorithms is essential for efficient coding. They help you write programs that run faster and use less memory.
Conclusion
Mastering these advanced concepts will not only improve your coding skills but also prepare you for real-world programming challenges. Remember, this roadmap will give you a complete guideline to build a strong coding habit and achieve your goal as a software developer!
Practical Examples of Coding
HTML Example
HTML, or HyperText Markup Language, is the standard language for creating web pages. Here’s a simple example:
<!DOCTYPE html>
<html>
<body>
<h1>Hello, world!</h1>
</body>
</html>
This code displays the message "Hello, world!" on a web page. It’s a great starting point for anyone learning to code.
JavaScript Example
JavaScript is a popular programming language used to make web pages interactive. Here’s a basic example:
function greet(name) {
console.log("Hello, " + name + "!");
}
greet("Alice");
In this code, we define a function called greet
that takes a name and prints a greeting. This shows how functions can be used to organize code.
Python Example
Python is known for its simplicity and readability. Here’s a quick example:
# This program adds two numbers
num1 = 5
num2 = 3
sum = num1 + num2
print("The sum is:", sum)
This Python code adds two numbers and prints the result. It’s a straightforward way to see how coding can perform calculations.
Summary of Examples
Language | Example Purpose |
---|---|
HTML | Display a message on a webpage |
JavaScript | Create interactive functions |
Python | Perform calculations |
These examples illustrate the basics of coding in different languages. Coding is a powerful tool that allows you to create and solve problems. As you learn more, you can build more complex programs and applications!
Learning Resources for Coding
Books and Ebooks
Reading books is a great way to learn coding. They often explain concepts in detail and provide exercises to practice. Here are some popular titles:
- "Automate the Boring Stuff with Python" by Al Sweigart
- "Eloquent JavaScript" by Marijn Haverbeke
- "HTML and CSS: Design and Build Websites" by Jon Duckett
Online Tutorials
There are many free online tutorials available that can help you learn coding at your own pace. Some popular platforms include:
- freeCodeCamp
- W3Schools
- Codecademy
Coding Bootcamps
If you want a more structured approach, consider a coding bootcamp. These programs are intensive and can help you learn coding quickly. They often include hands-on projects and mentorship. Some well-known bootcamps are:
- Fullstack Academy
- General Assembly
- Le Wagon
Online Courses
Many websites offer online courses that cover various programming languages. Some popular platforms include:
- Coursera: Offers courses from universities and companies like Google and IBM. Financial aid is available for those who qualify.
- edX: Features courses from top universities, including Harvard.
Video Tutorials
YouTube is a fantastic resource for coding tutorials. Channels like Crash Course Computer Science and Traversy Media provide valuable insights and lessons.
Community and Forums
Joining coding communities can be very helpful. Websites like Stack Overflow and Reddit have active forums where you can ask questions and share knowledge. Engaging with others can enhance your learning experience.
Learning to code is a journey, and there are many paths to success. Explore different resources to find what works best for you!
Career Opportunities in Coding
Jobs That Require Coding
In today’s tech-driven world, coding skills are essential for many jobs. Here are some popular roles:
- Web Developer: Builds and maintains websites.
- Software Engineer: Designs software applications.
- Data Scientist: Analyzes data to help businesses make decisions.
- IT Technician: Supports and manages computer systems.
- Cloud Engineer: Works with cloud computing services.
- DevOps Engineer: Combines software development and IT operations.
- Database Administrator: Manages databases to store and organize data.
- UX Designer: Focuses on user experience in software and websites.
Freelancing as a Coder
Freelancing offers flexibility and the chance to work on various projects. Here are some tips:
- Build a strong portfolio showcasing your work.
- Network with potential clients through online platforms.
- Set competitive rates based on your skills and experience.
- Stay updated with the latest coding trends and technologies.
Future of Coding Careers
The demand for coding skills is growing. As technology evolves, new opportunities will arise in fields like:
- Artificial Intelligence: Creating smart systems.
- Cybersecurity: Protecting data and systems from threats.
- Mobile App Development: Building apps for smartphones.
Coding is not just a skill; it’s a pathway to exciting career opportunities. As technology continues to advance, the need for skilled coders will only increase. Embrace the journey and explore the possibilities!
If you’re looking to kickstart your career in coding, now is the perfect time to take action! Our platform offers hands-on tutorials and resources that can help you build the skills you need to succeed in coding interviews. Don’t wait any longer—visit our website and start coding for free today!
Conclusion
In summary, learning to code is an exciting journey that opens up many doors. While it may seem tough at first, remember that every expert was once a beginner. Take your time to understand the basics, like data types, variables, and control structures. These are the building blocks of programming. Be patient with yourself and practice regularly. As you grow more comfortable with coding, you’ll find it easier to tackle more complex projects. So, keep pushing forward, stay curious, and enjoy the process of creating with code!
Frequently Asked Questions
What is coding?
Coding, or programming, is writing instructions for computers to follow. It allows us to create websites, apps, and software.
Why is learning coding important?
Learning coding helps you understand technology better and opens up many job opportunities.
How do I start coding?
Begin by choosing a programming language, setting up your tools, and practicing with simple projects.
What are variables in coding?
Variables are names that store data. They help programs remember information to use later.
What are control structures?
Control structures are rules that let your program make decisions, like if-else statements.
What are functions and why are they useful?
Functions are reusable blocks of code that perform specific tasks. They help organize your code and make it easier to manage.
What are data types?
Data types define the kind of data a variable can hold, like numbers, text, or lists.
How can I write clean code?
To write clean code, keep it simple, use clear names for variables, and add comments to explain your code.