How to Explain Your Solution’s Time Complexity Step by Step
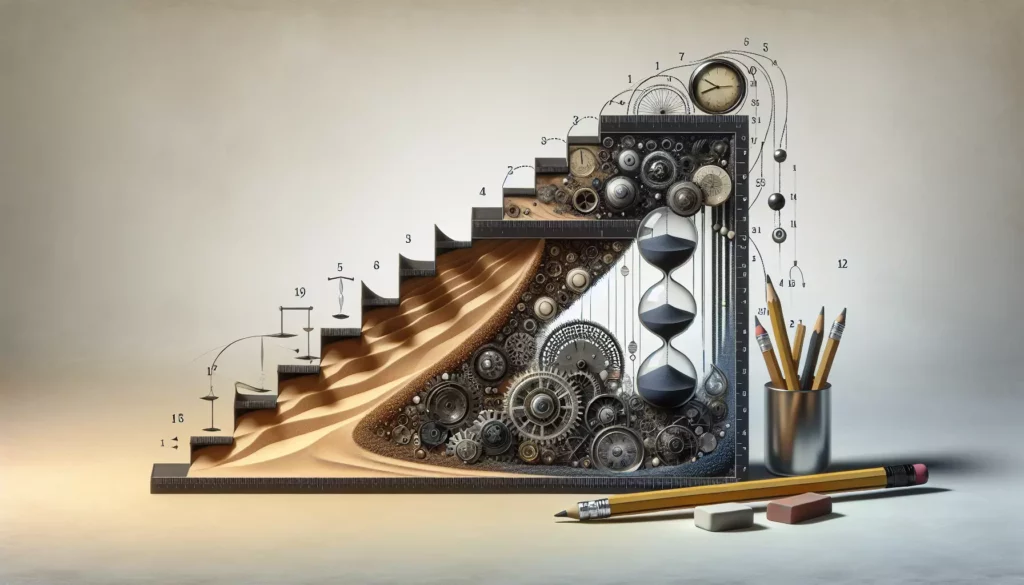
Understanding and explaining time complexity is a crucial skill for programmers, especially when preparing for technical interviews or optimizing algorithms. This comprehensive guide will walk you through the process of analyzing and explaining your solution’s time complexity step by step. By mastering this skill, you’ll be better equipped to tackle coding challenges and impress potential employers.
1. Understand the Basics of Time Complexity
Before diving into the explanation process, it’s essential to have a solid grasp of what time complexity is and why it matters.
What is Time Complexity?
Time complexity is a measure of how the runtime of an algorithm grows as the input size increases. It’s typically expressed using Big O notation, which provides an upper bound on the growth rate of the algorithm’s running time.
Why is Time Complexity Important?
Understanding time complexity helps you:
- Predict how your algorithm will perform with large inputs
- Compare different algorithms and choose the most efficient one
- Optimize your code for better performance
- Demonstrate your problem-solving skills in technical interviews
2. Identify the Key Operations
The first step in explaining your solution’s time complexity is to identify the key operations that contribute to the overall runtime. These are typically the operations that are executed most frequently or have the potential to grow significantly as the input size increases.
Common Operations to Look For:
- Loops (for, while, do-while)
- Recursive function calls
- Built-in methods or functions
- Data structure operations (e.g., inserting into an array or searching a binary tree)
Let’s look at an example to illustrate this step:
def find_max(arr):
max_val = arr[0]
for num in arr:
if num > max_val:
max_val = num
return max_val
In this simple function to find the maximum value in an array, the key operation is the for loop that iterates through each element of the input array.
3. Analyze the Growth Rate
Once you’ve identified the key operations, analyze how they grow as the input size increases. This step involves determining the relationship between the input size and the number of operations performed.
Common Growth Rates:
- Constant time: O(1)
- Logarithmic time: O(log n)
- Linear time: O(n)
- Linearithmic time: O(n log n)
- Quadratic time: O(n^2)
- Exponential time: O(2^n)
For our find_max
function, we can see that the for loop iterates through each element of the input array exactly once. As the size of the input array (n) grows, the number of iterations grows linearly. Therefore, the growth rate is linear, or O(n).
4. Consider Best, Average, and Worst Cases
When explaining time complexity, it’s important to consider different scenarios that may affect the runtime of your algorithm. These scenarios are typically categorized as best-case, average-case, and worst-case complexities.
Best-case Complexity
The best-case complexity represents the scenario where the algorithm performs the minimum number of operations possible. This is often less relevant in practice but can be useful for understanding the algorithm’s behavior in optimal conditions.
Average-case Complexity
The average-case complexity represents the expected performance of the algorithm under typical conditions. This is often the most relevant measure for real-world applications.
Worst-case Complexity
The worst-case complexity represents the scenario where the algorithm performs the maximum number of operations possible. This is often the most important measure for guaranteeing performance bounds.
For our find_max
function, the time complexity remains O(n) for all cases because it always iterates through the entire array, regardless of the input values.
5. Simplify and Express in Big O Notation
After analyzing the growth rate and considering different cases, simplify your analysis and express the time complexity using Big O notation. When simplifying, focus on the dominant term and drop constants and lower-order terms.
Rules for Simplification:
- Drop constants: O(2n) becomes O(n)
- Drop lower-order terms: O(n^2 + n) becomes O(n^2)
- Keep the highest-order term: O(n log n + n) becomes O(n log n)
For our find_max
function, we’ve already determined that the time complexity is O(n) for all cases, so no further simplification is needed.
6. Explain Your Analysis
Now that you’ve completed your analysis, it’s time to explain your reasoning clearly and concisely. A good explanation should include:
- A brief overview of the algorithm’s purpose
- Identification of the key operations
- Analysis of how these operations grow with input size
- Consideration of different cases (if applicable)
- The final time complexity expressed in Big O notation
Here’s an example explanation for our find_max
function:
“The
find_max
function finds the maximum value in an input array. The key operation in this function is the for loop that iterates through each element of the array. As the size of the input array (n) increases, the number of iterations grows linearly. The function always examines every element once, regardless of the input values or their order. Therefore, the time complexity of this function is O(n) in all cases – best, average, and worst.”
7. Provide Examples and Comparisons
To further illustrate your understanding of time complexity, it can be helpful to provide examples or compare your solution to alternative approaches. This demonstrates your ability to think critically about algorithmic efficiency and trade-offs.
For instance, you could compare the find_max
function to other approaches:
def find_max_sorted(arr):
return arr[-1] # Assumes the array is sorted in ascending order
def find_max_recursive(arr):
if len(arr) == 1:
return arr[0]
return max(arr[0], find_max_recursive(arr[1:]))
You could then explain:
“The
find_max_sorted
function assumes the input array is already sorted and simply returns the last element. This has a time complexity of O(1), which is more efficient than our original O(n) solution. However, it requires the additional constraint of a sorted input.The
find_max_recursive
function uses recursion to find the maximum value. While it may seem more elegant, it actually has a worse time complexity of O(n^2) due to the repeated slicing of the array in each recursive call. This demonstrates how a seemingly simple solution can have hidden performance implications.”
8. Consider Space Complexity
While the focus of this guide is on time complexity, it’s also important to consider space complexity when analyzing your solution. Space complexity measures the amount of memory an algorithm uses relative to the input size.
For the find_max
function, we can briefly mention the space complexity:
“In terms of space complexity, the
find_max
function uses only a constant amount of extra space to store themax_val
variable, regardless of the input size. Therefore, its space complexity is O(1).”
9. Practice with Different Algorithms
To become proficient at explaining time complexity, it’s crucial to practice with a variety of algorithms and data structures. Here are some common algorithms and their typical time complexities to help you get started:
Algorithm | Average Time Complexity | Worst Time Complexity |
---|---|---|
Binary Search | O(log n) | O(log n) |
Quick Sort | O(n log n) | O(n^2) |
Merge Sort | O(n log n) | O(n log n) |
Breadth-First Search | O(V + E) | O(V + E) |
Depth-First Search | O(V + E) | O(V + E) |
Where V is the number of vertices and E is the number of edges in a graph.
10. Address Common Pitfalls
When explaining time complexity, be aware of common pitfalls that can lead to incorrect analyses. Some of these include:
Overlooking Hidden Operations
Some operations that seem simple may have non-constant time complexity. For example, using the in
operator to check if an element is in a list takes O(n) time for unsorted lists.
def contains_duplicate(arr):
for num in arr:
if num in arr[arr.index(num) + 1:]:
return True
return False
This function might appear to be O(n) at first glance, but the in
operation inside the loop makes it O(n^2).
Misunderstanding Logarithmic Complexity
Logarithmic time complexity O(log n) often appears in divide-and-conquer algorithms or when the problem size is repeatedly halved. It’s important to recognize these patterns.
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
This binary search implementation has a time complexity of O(log n) because it halves the search space in each iteration.
Forgetting about Nested Loops
Nested loops can quickly increase the time complexity of an algorithm. Always consider how inner loops affect the overall complexity.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
This bubble sort implementation has two nested loops, resulting in a time complexity of O(n^2).
11. Optimize Your Solution
After analyzing and explaining the time complexity of your solution, consider if there are ways to optimize it. This demonstrates your ability to improve algorithms and can be particularly impressive in interview settings.
For example, let’s optimize our earlier contains_duplicate
function:
def contains_duplicate_optimized(arr):
seen = set()
for num in arr:
if num in seen:
return True
seen.add(num)
return False
You could then explain the optimization:
“The optimized version uses a set to keep track of seen numbers. This improves the time complexity from O(n^2) to O(n) because set operations (checking membership and adding elements) have an average time complexity of O(1). The space complexity increases to O(n) to store the set, demonstrating a classic time-space trade-off.”
12. Relate to Real-World Scenarios
To make your explanation more compelling, especially in interview situations, relate the time complexity analysis to real-world scenarios. This shows that you understand the practical implications of algorithmic efficiency.
For instance, when discussing the time complexity of sorting algorithms:
“While quicksort has an average-case time complexity of O(n log n), its worst-case complexity is O(n^2). This could be problematic in real-time systems or when dealing with very large datasets. For example, in a high-frequency trading system where consistent performance is crucial, we might prefer merge sort, which guarantees O(n log n) time complexity in all cases, despite potentially using more memory.”
13. Be Prepared for Follow-up Questions
When explaining time complexity, especially in an interview setting, be prepared for follow-up questions. These might include:
- How would the time complexity change if we modified the input or constraints?
- Can you think of any edge cases that might affect the performance?
- How does the space complexity compare to the time complexity?
- What trade-offs did you consider when choosing this approach?
Being able to answer these questions demonstrates a deep understanding of algorithmic analysis and problem-solving skills.
14. Practice Clear Communication
Explaining time complexity effectively is not just about the technical analysis; it’s also about clear communication. Here are some tips to improve your explanation skills:
- Use simple language and avoid jargon when possible
- Break down complex ideas into smaller, manageable parts
- Use analogies or real-world examples to illustrate concepts
- Be concise, but don’t skip important details
- Practice explaining out loud to improve verbal communication
Conclusion
Explaining your solution’s time complexity is a valuable skill that demonstrates your understanding of algorithmic efficiency and your ability to analyze and optimize code. By following these steps and practicing regularly, you’ll be well-prepared to discuss time complexity in technical interviews and real-world programming scenarios.
Remember that time complexity analysis is just one aspect of algorithm design and optimization. Always consider other factors such as space complexity, readability, and maintainability when evaluating and explaining your solutions. With practice and experience, you’ll develop a intuitive understanding of time complexity and be able to quickly analyze and explain the efficiency of your algorithms.
As you continue to learn and grow as a programmer, keep challenging yourself with new problems and algorithms. Platforms like AlgoCademy offer a wealth of resources and practice problems to help you hone your skills in algorithmic thinking and time complexity analysis. By mastering these concepts, you’ll be better equipped to tackle complex programming challenges and excel in your coding career.